Collections in python
In this tutorial, we will see what is collection in python. Further, we will see in-depth the different kinds of collections in python.
Collections
Collections in python are the built-in module used to store the groups of data together in a container. The collection module provides different categories of containers to ease the work of the user.
Some of the containers provided in python are-
- Counters
- OrderedDict
- DefaultDict
- ChainMap
- NamedTuple
- DeQue
- UserDict
- UserList
- UserString
Now, let’s start discussing each one of the above one by one.
Counters
It acts as a data holder. It is considered a subclass of the dictionary. It stores the count of the elements in an unordered dictionary, where the key represents the element, and the value is represented by the count of that particular element. It keeps a record of the number of times a certain value has been added.
It is required to import the module to be able to use the counter.
Syntax to import
from collections import Counter
Implementation
# A Python program to show three ways to create Counter
from collections import Counter
# creation with sequence of items
print(Counter(['X','Y','X','Y','F','X','Y','Z','Z','Y']))
# creation with dictionary
print(Counter({'F':1, 'X':3, 'Y':4, 'Z':1,}))
# creation with keyword arguments
print(Counter(F=3, X=3, Y=4, Z=1))
Output
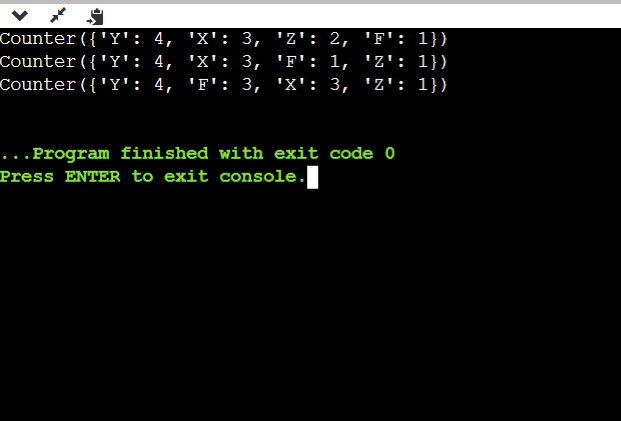
Explanation: The image shows the output when a counter is created using three different methods.
OrderedDict
The meaning of this container is self-explanatory. It is an OrderedDict which is also one of the sub-classes of the dictionary. It recollects the order in which the keys were inserted in the dictionary.
It is required to import the following module to be able to use OrderedDict.
Syntax to import
from collections import OrderedDict
Implementation
# A Python program to show the how an OrderedDict works
from collections import OrderedDict
print("This is a Dictionary:\n")
dt = {}
dt['v'] = 1
dt['x'] = 2
dt['y'] = 3
dt['z'] = 4
for key, value in dt.items():
print(key, value)
print("\n This is an Ordered Dict:\n")
odt = OrderedDict()
odt['v'] = 1
odt['x'] = 2
odt['y'] = 3
odt['z'] = 4
for key, value in odt.items():
print(key, value)
Output
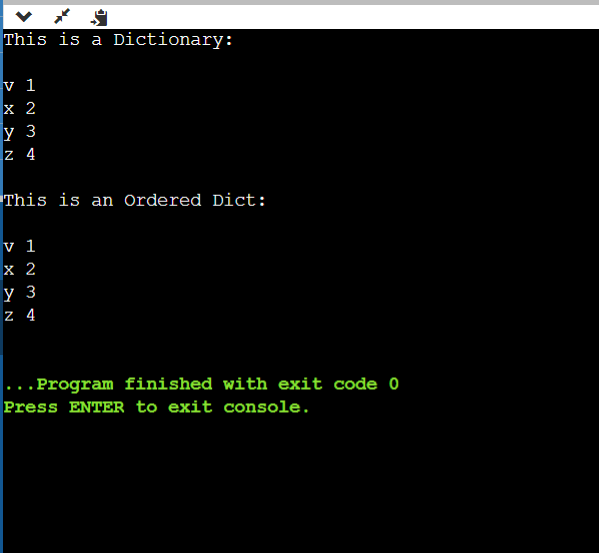
Explanation: The image shows the output when an ordered Dict is created to show how it works.
DefaultDict
To solve the keyerror problem arising in a dictionary, defaultdict comes into action.
It is also one of the containers of the collection modules in python. To be precise, it is a subdivision of dictionary class.
It is required to import the following module to be able to use DefaultDict.
Syntax
from collections import defaultdict
Implementation
# Python program to show how the defaultdict works
from collections import defaultdict
defaultdict_demo = defaultdict(list)
defaultdict_demo['first'].append(1)
defaultdict_demo['second'].append(2)
defaultdict_demo['first'].append('1')
defaultdict_demo['third']
print(dict(defaultdict_demo.items()))
Output
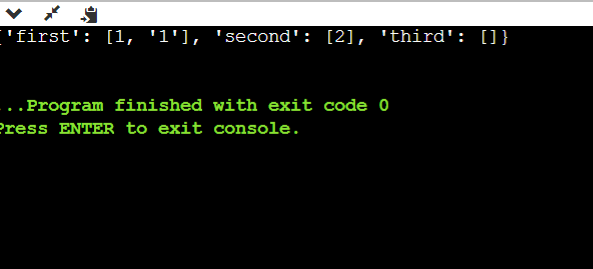
Explanation: The image shows the output showing the utility of defaultdict
Instead of throwing an error for the third key. It shows the list by default.
ChainMap
A ChainMap is another built-in container that encapsulates several dictionaries into a single component and yields a list of dictionaries.
The following syntax is being used to import chainMap.
Syntax
import collections from chainMap
Implementation
# Python program to show the working of ChainMap
from collections import ChainMap
dt1 = {'x': 8, 'y': 7}
dt2 = {'p': 5, 'q': 8}
dt3 = {'d': 35, 'f': 66}
# Defining the chainmap
cm = ChainMap(dt1, dt2, dt3)
print(cm)
Output
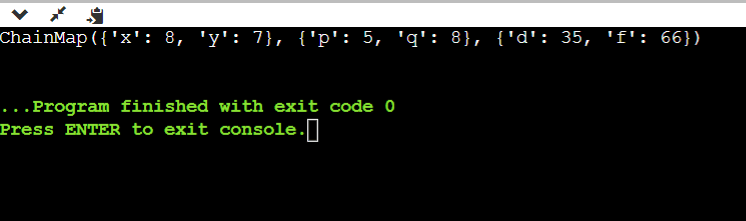
Explanation: This image shows three dictionaries in output. This is the utility of chainMap. It allows to creation of multiple dictionaries.
It also allows to add multiple new dictionaries through new_child() method.
Implementation
# Python code to demonstrate ChainMap and
# new_child()
import collections
# initialization of dictionaries
dt1 = { 'x' : 1, 'z' : 2 }
dt2 = { 'c' : 3, 'e' : 4 }
dt3 = { 'r' : 5 }
# Initialization of ChainMap
chainmap = collections.ChainMap(dt1, dt2)
# printing of chainMap
print ("All the ChainMap contents are : ")
print (chainmap)
# using new_child() to add new dictionary
chainmap1 = chainmap.new_child(dt3)
# printing of chainMap
print ("Displaying new ChainMap: ")
print (chainmap1)
Output
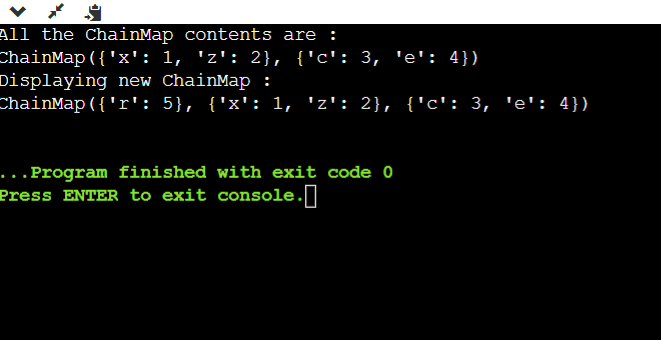
Explanation: This image shows the output of the code written above.
In the First chainmap, the contents of the first two dictionaries, dt1 and dt2, are shown.
In the second chainmap, the third dictionary dt3 has been added through the new_child method.
NamedTuple
It is another class under the module collection.
It is also a tuple with additional functionalities. Unlike ordinary tuples, it returns a tuple object with names for each position. It is also similar to dictionaries in python but, it offers additional features. It contains keys along with their equivalent values, just like dictionaries. It allows access to values through keys like in dictionaries, and besides that, it also allows to access values through an index that is not provided in usual dictionaries.
Like all other containers, it is also required to import modules before using namedtupule.
syntax:
from collections import namedtupule
Implementation:
# Python code to illustrate the working of namedtuple()
from collections import namedtuple
# namedtuple() is being declared
Employee = namedtuple('Employee' ,['name', 'age', 'eid'])
# Adding the values
E = Employee('NandaDevi' , '25', '1741990')
# Accessing using index
print ("The Employee age using index is : ",end ="")
print (E[1])
# Accessing using the name
print ("The Employee name using keyname is : ",end ="")
print (E.name)
Output:
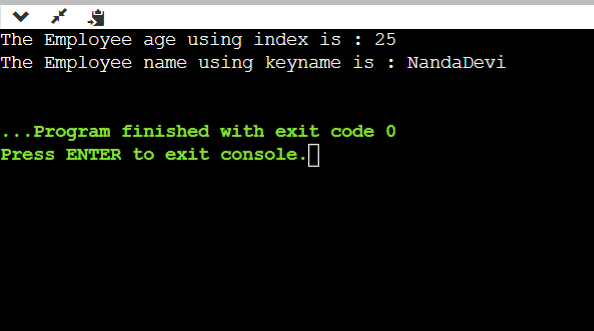
Explanation: This image contains the output of the code written above. The first line shows the output when the value is accessed through the index, and the second line shows when the value is accessed through the name of the key.
Deque
It is an acronym for Doubly Ended Queue. It comes under collection in python. It does an operation similar to the list but with a faster speed. It takes lesser time to pop and push elements from either side of the container. Time complexity here is O(1) and in the case of the list, it is O(n).
The dequeue function takes the list as an argument.
Like all other containers, it is also required to import modules before using Deque.
syntax:
from collections import deque
Let’s understand how the append function works in the deque.
Implementation
# Python code to illustrate the working of append(), appendleft() functions in deque
from collections import deque
# initialization of deque
dq = deque([15,22,38])
# inserting an element at the right end using append()
# here,48 is inserted at the end of the deque
dq.append(48)
# modified deque is being printed.
print ("The deque obtained after appending at right is: ")
print (dq)
# to insert an element at the right end using appendleft()
# Here,87 gets inserted at the beginning of deque
dq.appendleft(87)
# modified deque is being printed
print ("The deque obtained after appending at left is: ")
print (dq)
Output:
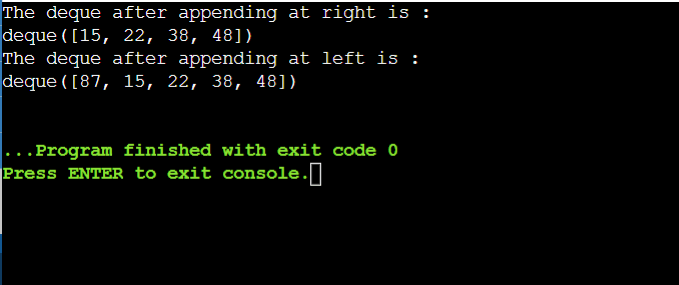
Explanation: The image shows the output of the code written above. The first line of the code gives the deque when values are appended at the right and the second line shows the output when values are appended at the left end.
Now, let’s understand how the pop operation works in the deque.
# Python code to illustrate the working of
# pop(), and popleft() functions
from collections import deque
# initialization of deque
dq = deque([67, 21, 87, 72, 36, 49])
# deleting an element from the right end using pop()
# deletes 49 from the right end of the deque
dq.pop()
# modified deque is being printed
print ("The deque obtained after deleting from right is: ")
print (dq)
# deleting element from left end using popleft()
# deletes 67 from the left end of deque
dq.popleft()
# printing modified deque
print ("The deque obtained after deleting from left is: ")
print (dq)
Output:
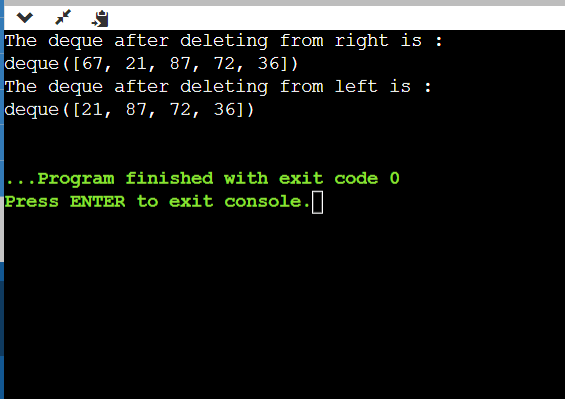
Explanation: The image shows the output of the code written above. The first line of the code gives the deque when the value (here 49) is popped from the right end and the second line shows the output when the value (here 67 ) is popped from the left end.
UserDict
It is another container just like a dictionary. This acts as a cover around a dictionary. It is a way of adding new features to the existing dictionary. This container is used when user wants to create their dictionary with added functionality.
Syntax:
from collections import UserDict
Implementation
# Program to illustrate UserDict
from collections import UserDict
dt = {'x':17,
'y': 25,
'z': 39}
# Creating an UserDict container
userDt = UserDict(dt)
# Printing the created UserDict
print(userDt.data)
# Creating an empty UserDict
userDt = UserDict()
print(userDt.data)
Output:
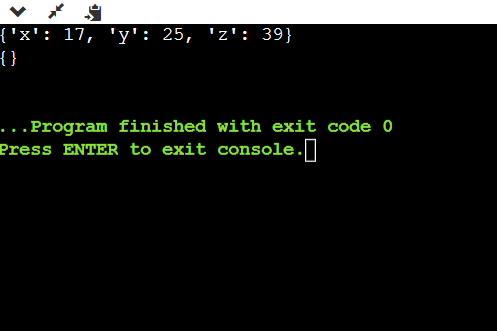
Explanation: The first line shows the created dictionary, and the second line is the empty dictionary created by the user.
Implementation to understand userdict better.
# Python program to illustrate userdict
from collections import UserDict
# Creating a Dictionary in which deletion is not allowed
class Dict1(UserDict):
# Function to stop deletion from dictionary
def __del__(self):
raise RuntimeError("Deletion is not allowed")
# Function to stop pop from the dictionary
def pop(self, s = None):
raise RuntimeError("Deletion is not allowed")
# Function to stop the pop item from Dictionary
raise RuntimeError("Deletion is not allowed")
# Driver's code
dt = Dict1({'x':15,
'y': 24,
'z': 33})
print("unmodified Dictionary")
print(dt)
dt.pop(1)
Output:
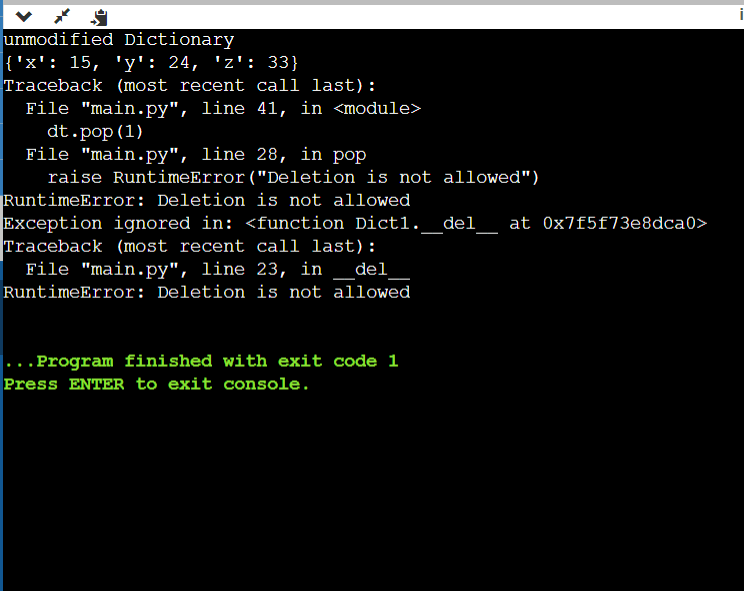
UserList
The UserList is another container available in the collection module of python. This class acts as a cover or a wrapper around the lists. It can store similar as well as dissimilar types of data together. Such collections are of great use when user wants to create a list of their own with some added functionalities.
Here also, it is required to import the module first to be able to use UserList.
Syntax:
from collections import UserList
Now, let us see some programs to understand the nature of the UserList container in Python.
Implementation 1:
This Program is just to understand the working of UserList
#Program to illustrate the working of user list in python
from collections import UserList
lt1 = [1 , 2 , 3 , 4 , 5]
user_list1 = UserList(lt1)
print("The UserList is :")
print(user_list1.data)
Output:
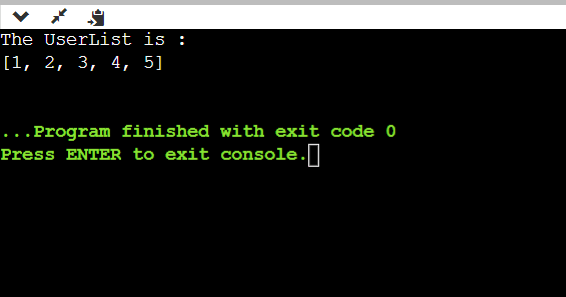
Explanation: This image shows the output of the code written above. It shows how the UserList is created in Python.
Implementation 2:
#python program to illustrate the wrapper class in UserList in
from collections import UserList
class mydata(UserList):
def pop(self, s = None):
raise RuntimeError("Deletion is not allowed")
mylist1 = mydata([10 , 20 , 30])
print("Initial list :")
print(mylist1)
mylist1.append(75)
mylist1.append(45)
print("List obtained after insertion :")
print(mylist1)
mylist1.pop()
Output:
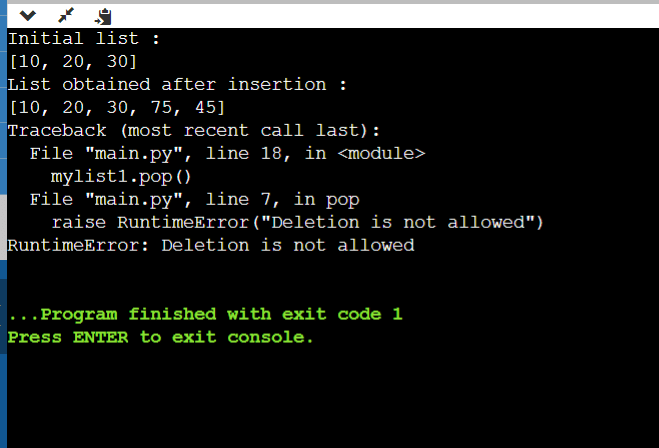
Explanation: This image shows the output for the program written above. Here, the UserList acts as a wrapper class for a customized list named mylist1 and allows users to add the function of their own. Here, the functionality ‘deletion not possible’ is added.
UserString
The UserList is another container in the dictionary that acts as a cover around a string. This class is useful when one wants to create a string with added functionalities. This class is capable of taking any argument as input and further converting it into a string.
Here also, it is mandatory to import the module to be able to use UserString.
Syntax:
from collections import UserString
Now, let us see some programs to understand the nature of UserString.
Implementation 1:
An Example where a number is being used to create an UserString.
#Importing the UserString module
from collections import UserString
#Initializing the variable
data = 738
ud = UserString(data)
print("Original data : " + ud.data)
#string concatenation is performed and the value is printed thrice
print("resultant data :")
print(ud.data + ud.data + ud.data)
Output:
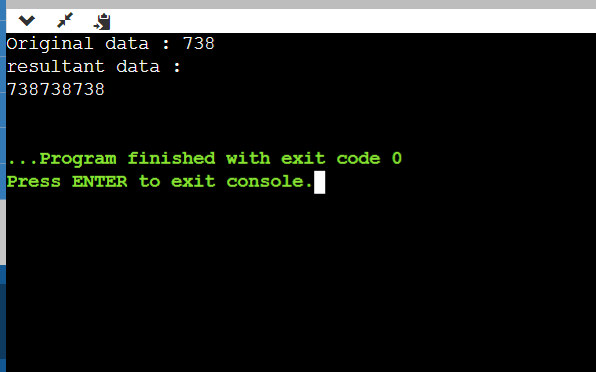
Explanation: This image shows the output of the code written above. Here, the number 738 is converted into a string using UserString, and concatenation is applied.
Implementation 2:
An example where an UserString is being created, which acts as a packaging class for a modified string object. Thus, it allows the user to add and replace the character in a string.
#Importing the UserString
from collections import UserString
# Defining a class
class Stringex(UserString):
def append(self, s):
self.data += s
def rep(self, s):
self.data = self.data.replace(s, "*")
s = Stringex("java")
print("Initial String:", s.data)
# Calling the append function
s.append(" is fun")
print("String after append function is performed:", s.data)
# Replacement of one character with the other
s.rep("a")
print("String received after replacement:", s.data)
Output:
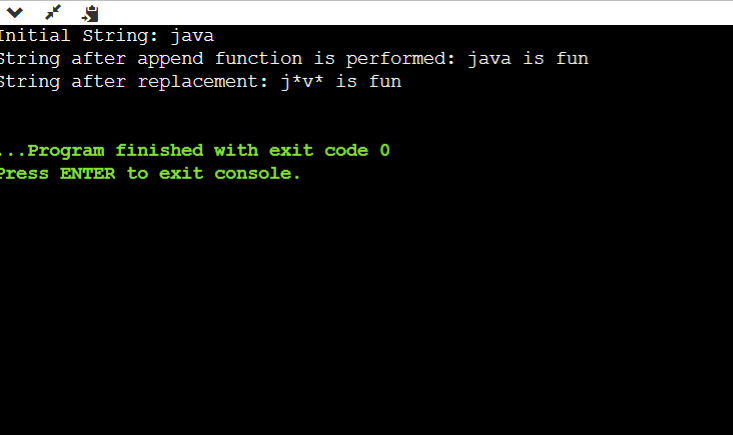
Explanation: This image contains the output of the code written above. The first line shows the string on which we are working. The second line shows the string after some new words get added. The third line shows the string in which character a is replaced with ‘*’.
Implementation 3:
An example where an UserString is being created which acts as a packaging class for a modified string object. Thus, it allows the user to add and delete the character in a string.
#Importing the UserString container
from collections import UserString
#Defining the class stringdemo
class stringex(UserString):
def append(self, a):
self.data = self.data + a
def remove(self, s):
self.data = self.data.replace(s, "")
National_Parks =' Betla Kaziranga Valmiki Periyar Silent_valley Gir Sunderban Udaipur'
np = stringex(National_Parks)
print("Entered Strings : "+np)
print("string after modification : ")
for i in ['Betla','Gir']:
np.remove(i)
print(np)
Output:
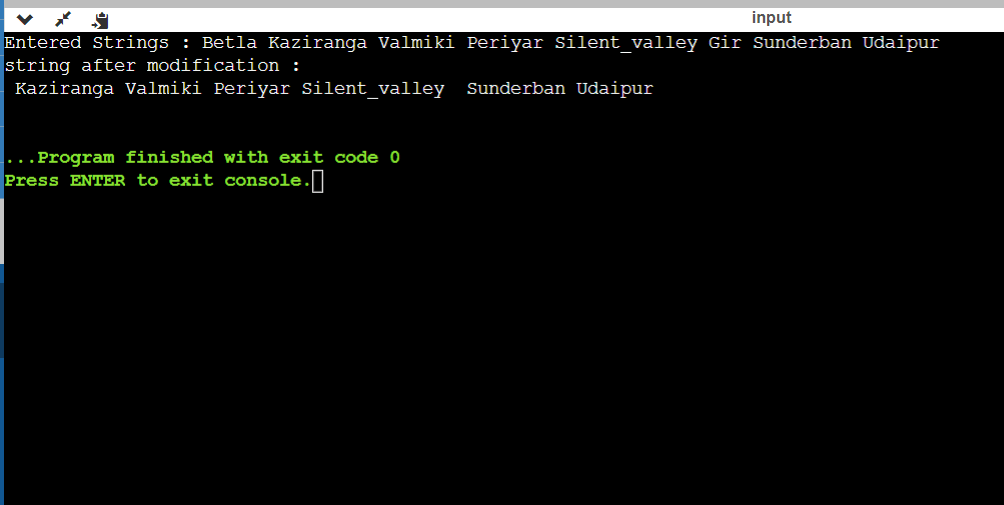
Explanation: This image contains the output of the code written above. Here, the first line shows the string on which we are performing the operation the and second string is obtained after Gir and Betla have been deleted from the string.
Summary:
In this tutorial, we realized the importance of 9 containers available in the module collection in python. We started studying the meaning of the word Collection and ended up understanding each container of collection in detail with the aid of certain examples.