Image to NumPy Arrays in Python
Image is a simple process of working model confined to machine learning, Python works with the image in the data format of width and height i.e. Images are excelled into NumPy arrays structure in height and channel format.
We need to import two different modules for this process and those are to be followed by:
- NumPy
- Pillow
NumPy:
NumPy by default was brought up from the versions 3.x, NumPy comes with the Python wholly but if it is not present, we can install it from cmd by using the command:
pip install NumPy
This works with the arrays and has functions for image classification too.
Pillow:
Pillow is an image editor tool which is been explicitly installed in the new versions of Python. In the Python version Python3, the library pillow is just nothing but an advanced and upgraded version of PIL. If it is not present in the main idle, we can install by using the command:
pip install pillow
As the versions of the pillow are not up to date if we want to check the version of pillow, we jus need to use the command
Import PIL
Print (‘Installed pillow version:’, PIL. __version__)
The first step is to load image by using the path directory assigning to it and importing the image which is of PNG format. We need to focus on the image class because it is the main source of PIL. OPEN() function is used to display up image and load the digital content in it and retrieve the data as of pixel format.
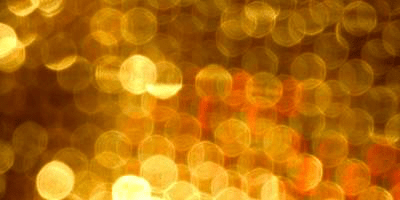
Sample image is imported using the code below.
Code:
From PIL import Image
#sample.png is the name of the image that we have uploaded in the current directory or we need
#To give the path
Image=Image. Open(‘Sample.png’)
#
PNG
(300, 150)
RGB
After importing the image we need to convert the image into NumPy array and Python contains many modules and they contain API which is used to convert the image to NumPy array.
Python NumPy module in itself provides and it contains the methods and they are followed as:
- asarray()
- NumPy. Array ()
- Image.fromarray()
Using asarray():
It is a function that is implemented to convert PIL images into NumPy arrays module. This function is used converts the given input to an array. Array makes a duplicate copy of the input we given but the asarray will not make the copy until its unnecessary.
Code:
#Import the necessary libraries
From PIL import Image
From NumPy import asarray
#Loading the given image and converting to numpy array
Image = Image. Open(‘Sample.png’)
#Asarray () function is used to load and convert PIL images into NumPy arrays respectively
NumPy data = asarray(image)
#<class ‘NumPy. Nd array’>
Print (type (NumPy data))
#Shape
Print (NumPy data. shape)
Output:
<class ‘numpy.ndarray’>
(200, 400, 3)
Using NumPy. Array ():
NumPy array function is used to create arrays in Python by manipulating Python structures like lists and tuples into arrays or by using intrinsic NumPy creation in Python.
And the second stage of deployment of code:
from PIL import Image
Import NumPy
Image = Image. Open(“Sample.png”)
np_image = NumPy. Array(img)
print(np_image.shape)
Output:
(200, 400, 3)
Image.fromarray():
from array function is implemented to make image memory form an image object which exports the array by providing the required path and file name respectively.
Code:
import numpy as np
from PIL import image
Array = np.arrange(0, 73280, 1, np.uint8)
Array = np.reshape(array, (1024, 720))
im = Image.fromarray(array)
im.save(“filename.jpeg”)
We can use the function imageio. imwrite () function to save NumPy arrays as an image and we can also deploy the SciPy and matplotlib modules to implement the images to numpy array.
We can also convert NumPy array to back its image.
The image.fromarray() function is used to convert the image to array and comes with the handmade manipulation functions and numpy.ndarray image data.
from PIL import Image
from numpy import asarray
#Load the image
Image=Image. open(‘Kolkata.jpeg’)
#Converting the image into numpy array
data = asarray(image)
Print(type(data))
#shape resizing
Print (data. Shape)
#creating pillow image
Image2=Image.fromarray(data)
Print(type(image2))
#concludimg the details
Print (image2.mode)
Print (image2.size)
Output:
<class ‘numpy. ndarray’>
(450,800,3)
<class ‘PIL.Image.Image’>
RGB
(800,450)