Text detection using Tkinter in Python
Tkinter:
The standard Python technique for building Graphical User Interfaces (GUIs) is Tkinter, which is included in all popular Python distributions. The only framework included in the Python standard library is this one. On top of Tk, this Python framework serves as a thin object-oriented layer that provides access to the Tk toolkit. The Tk toolkit is a cross-platform set of "graphical control elements," also called widgets, used to build application interfaces. This framework gives Python users a quick and easy way to build GUI elements with the Tk toolkit's widgets. In a Python application, Tk widgets can create buttons, menus, data fields, etc.
Once created, these graphic elements can be connected to or work with other widgets, features, functionality, processes, or data.
Tkinter bridges the gap between Python's execution model and Tcl's, built around cooperative multitasking. Like Python, Tcl is an interpreted dynamic programming language. Although it is an overall programming language that can be used independently, it is most frequently used in C programs as a scripting motor or as an interaction with the Tk toolbox. The Tcl library has a C interface that enables users to add custom Tcl or C commands, run Tcl commands and scripts, and manage one or more Tcl interpreter instances. Each interpreter has a queue for events that can be used to send and process events.
Syntax:
from tkinter import *
from tkinter import ttk
Advantages of Tkinter:
This promotes flexibility in handling GUI incidents, which makes the code base run more smoothly. Qt is more than just a framework; it uses a variety of native platform APIs for networking, database development, and other uses. Through a unique API, it gives them direct access.
Disadvantages of Tkinter:
Tkinter doesn't have any advanced widgets. There isn't a tool like Qt Designer for Tkinter in it. Its user interface is unreliable. Tkinter can be challenging to debug at times. It's not entirely in Python.
- The lexicon- and rule-based sentiment analysis tool VADER (Valence Aware Dictionary and sEntiment Reasoner) is customized precisely to sentiments expressed on social media. VADER makes use of a variety of. A sentiment lexicon is a collection of linguistic elements (such as words) that are often classified as either negative or positive depending on their semantic orientation. VADER informs us of the positive and negative scores and only informs us of the positive and negative scores but also the sentimentality of each score.
CODE:
import time
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from tkinter import *
import tkinter.messagebox
from nltk.sentiment.vader import SentimentIntensityAnalyzer
class analysis_text():
def center(self, toplevel):
toplevel.update_idletasks()
w = toplevel.winfo_screenwidth()
h = toplevel.winfo_screenheight()
size = tuple(int(_) for _ in
toplevel.geometry().split('+')[0].split('x'))
x = w/2 - size[0]/2
y = h/2 - size[1]/2
toplevel.geometry("%dx%d+%d+%d" % (size + (x, y)))
def callback(self):
if tkinter.messagebox.askokcancel("Quit",
"Do you want to leave?"):
self.main.destroy()
def setResult(self, type, res):
if (type == "neg"):
self.negativeLabel.configure(text =
"you typed negative comment : "
+ str(res) + " % \n")
elif (type == "neu"):
self.neutralLabel.configure( text =
"you typed comment : "
+ str(res) + " % \n")
elif (type == "pos"):
self.positiveLabel.configure(text
= "you typed positive comment: "
+ str(res) + " % \n")
def runAnalysis(self):
sentences = []
sentences.append(self.line.get())
sid = SentimentIntensityAnalyzer()
for sentence in sentences:
ss = sid.polarity_scores(sentence)
if ss['compound'] >= 0.05 :
self.normalLabel.configure(text =
" you typed positive statement: ")
elif ss['compound'] <= - 0.05 :
self.normalLabel.configure(text =
" you typed negative statement")
else :
self.normalLabel.configure(text =
" you normal typed statement: ")
for k in sorted(ss):
self.setResult(k, ss[k])
print()
def editedText(self, event):
self.typedText.configure(text = self.line.get() + event.char)
def runByEnter(self, event):
self.runAnalysis()
def __init__(self):
self.main = Tk()
self.main.title("Text Detector system")
self.main.geometry("600x600")
self.main.resizable(width=FALSE, height=FALSE)
self.main.protocol("WM_DELETE_WINDOW", self.callback)
self.main.focus()
self.center(self.main)
self.label1 = Label(text = "type a text here :")
self.label1.pack()
self.line = Entry(self.main, width=70)
self.line.pack()
self.textLabel = Label(text = "\n",
font=("Helvetica", 15))
self.textLabel.pack()
self.typedText = Label(text = "",
fg = "blue",
font=("Helvetica", 20))
self.typedText.pack()
self.line.bind("<Key>",self.editedText)
self.line.bind("<Return>",self.runByEnter)
self.result = Label(text = "\n",
font=("Helvetica", 15))
self.result.pack()
self.negativeLabel = Label(text = "",
fg = "red",
font=("Helvetica", 20))
self.negativeLabel.pack()
self.neutralLabel = Label(text = "",
font=("Helvetica", 20))
self.neutralLabel.pack()
self.positiveLabel = Label(text = "",
fg = "green",
font=("Helvetica", 20))
self.positiveLabel.pack()
self.normalLabel =Label (text ="",
fg ="red",
font=("Helvetica", 20))
self.normalLabel.pack()
myanalysis = analysis_text()
mainloop()
CODE EXPLANATION:
Importing all the required modules which are Time, Pandas, numpy, matplotlib, tkinter Adding a main function in the program to update, winfo_screenwidhth, winfo_screenheight, dimensions including geometry. Next calculate comments in vader analysis. Create a main window using main() method that are Title, geometry, resizable, protocol, focus. Adding addition item on window that is hidden entry button.
OUTPUT:
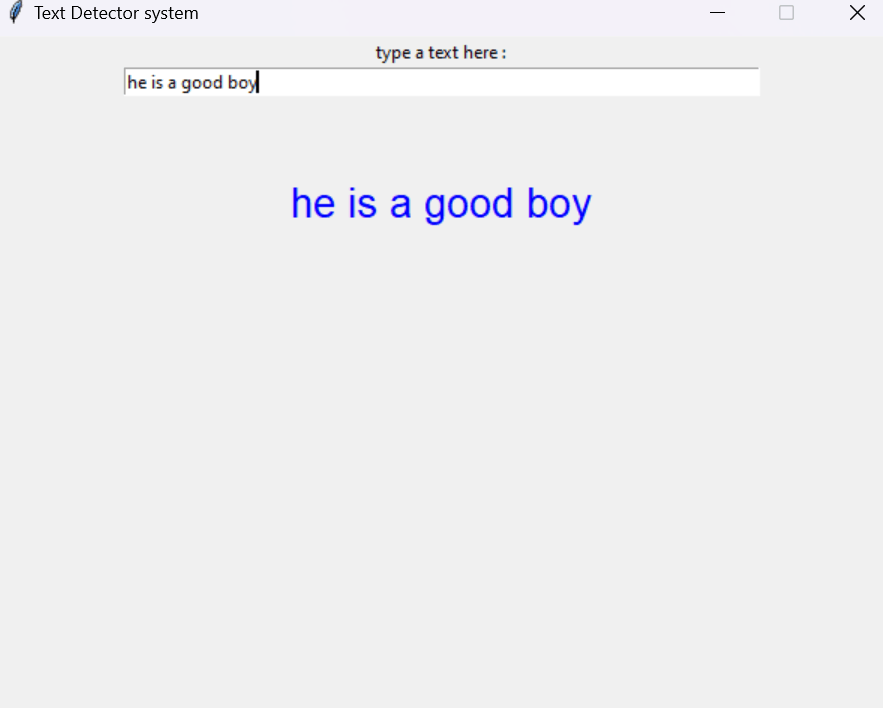