Add a key-value pair to dictionary in Python
In programming, data type defines the type of value that a variable can hold. With help of these, we can perform various mathematical, logical, or relational operations on that particular variable. Similarly, Dictionary is also a data type in the programming language.
What is a Dictionary?
It is a collection of unordered data. Unlike other data types, it does not hold a single value as its element, it holds elements in the format of key-value pair, where keys and values are separated by ‘:’ and two keys are separated by a comma. A dictionary can have identical values but it cannot store identical keys. While creating a dictionary we should keep in mind that keys are case sensitive i.e., both ‘day’ and ‘Day’ will be considered as different keys as the first letter of both words is in a different case (small letter and capital letter).
How to create a Dictionary?
# Creating a Dictionary
dic1={1: 'One', 2: 'Two', 3: 'Three'}
print("\nDictionary with the use of Integer Keys: ")
print(dic1)
# Creating a Dictionary with different keys
dic1={'name': 'Amit', 1: [08, 01, 20, 04]}
print("\nDictionaryusingdifferent Keys: ")
print(dic1)
# Creating a Dictionary using the dict() method
new=dict({11: 'Eleven', 12: 'Twelve', 13:'Thirteen'})
print("\nDictionaryusingdict() function: ")
print(new)
# Creating a Dictionary with each item as a Pair
new1=dict([(20, 'Twenty'), (22, 'Twenty-Two')])
print("\nDictionaryusing paired items: ")
print(new1)
Output:
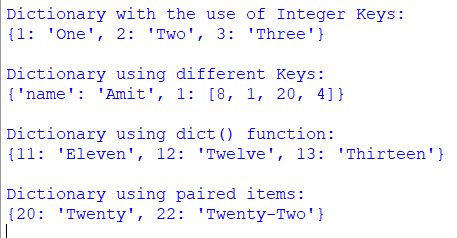
What are keys in a Dictionary?
As we know, a dictionary holds the data in the form of key-value pair, and between every key-value pair, there rests a colon “:”, and these pairs are divided by commas “,”.
Keys are unique whereas values can be the same in a dictionary. In a dictionary, it is compulsory that, the keys should be of immutable data type, but values can be of any type.
Properties of keys in a Dictionary
- Duplicate keys are not allowed, as keys have to be unique. In this case, if same keys are entered, then the key entered at last is considered.
For example:
dict={'Name':'Aadi','Age':18,'Blood_Group' :'A+', 'Name':'Amit'}
print("dict['Name']: ",dict['Name'])
Output:

As we can see in this example when we entered multiple keys with the same name, then the key entered at last is considered and when we executed the program, we have received output as “Amit”.
- Declared keys has to be immutable, i.e., for declaring keys, strings, numbers, or tuples can be used but other methods cannot be used. For example:
dict = {['Name']: 'Zara', 'Blood_Group' : 'A+', 'Age': 7}
print ("dict['Name']: ", dict['Name'])
After executing the above code we get the following output:

Unlike keys, values in dictionaries have no hard and fast rules. They can be any user-defined objects, standard objects, or arbitrary objects.
Difference between updating and adding a new key-value pair in a Dictionary:
- While updating a dictionary, the value of the pre-existing key is updated, no new element is added or deleted and the size of the dictionary remains the same. A dictionary can be updated in two ways:
Method 1:
It can be updated using the update function.
For example:
- # A program in python to update a dictionary using an update
- function
- # Dictionary with three items
- dict1={'X': 'Good', 'y': 'Day',}
- dict2={'Y': 'Morning'}
-
- # Dictionary before updating
- print("Dictionary before updating:")
- print(dict1)
-
- # Updating the value of key 'Y'
- dict1.update(dict2)
- print("Dictionary after updating:")
- print(dict1)
Output:
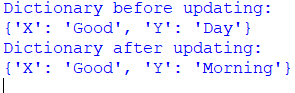
Method 2:
The value of existing can also be updated directly.
For example:
- defchkKy(dict, key):
- ifkey indict.keys():
- dict['a'] = 550
- else:
- print("Key does not exist in the dictionary.")
- dict={'a': 600, 'b':50, 'c':400}
- print("Dictionary before updating:\n", dict)
-
- key ='a'
- chkKy(dict, key)
- print("Dictionary after updating:\n", dict)
Output:
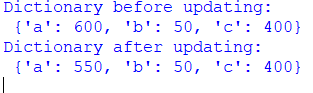
- While adding a new key-value pair to a dictionary, single or multiple new pairs are added and therefore the size of the dictionary also changes.
For example:
• dict={'Name':'Zaid','Age':17,'Class':'Elevents'}
• print("Dictionary before adding new elements: \n",dict)
• # Add new key-value pairs
• dict['School']="DAV Public School"
• dict['Blood Group']="O-"
• dict['Date of Birth']="5 June 2000"
• print("\nDictionary after adding new elements: \n",dict)
Output:

Some built-in Dictionary Methods and Functions
Some of the Built-in methods are:
Methods | Description |
dict.clear() | This method deletes all the elements from the dictionary dict. |
dict.copy() | This method gives back a shallow copy of the dictionary dict. |
dict.formkeys() | This method creates a new dictionary using keys from seq and values set to value. |
dict.get(key, default = None) | This method returns the value of the entered key or returns default if the key is not present in the dictionary. |
dict.has_key(key) | This method is used to check whether a key is present in a dictionary or not. |
dict.items() | This method is used to return the list of the dictionary’s (key-value) tuple pairs. |
dict.keys() | This method is used to return the list of keys present in a dictionary. |
dict.values() | This method is used to return the list of values present in a dictionary. |
dict.update(dictn) | This method is used to add other dictionary elements to the first dictionary. |
dict.setdefault(key, default = None) | This method is similar to the “dict.get()” method, but it sets “dict[key] = default” if the key is not present in the dictionary. |
Some of the built-in dictionary functions are:
Functions | Description |
cmp(d1, d2) | This function is used to compare two dictionaries. |
len(dict) | This function is used to return the total length of the dictionary, i.e., the total no of items present in it. |
str(dict) | This function is used to produce a printable representation of a dictionary. |
type(variable) | This function is used to check the type of the variable passed in this function. |