How to create a list in Python?
How to create a list in python
Python is known for its versatility and its data structures help us to perform different kinds of operations on various datasets irrespective of their datatype.
In this article, we will briefly discuss the features of the list, the operations we can perform and the methods we can apply.
Let's start by defining what the list is?
List – It is a sequence of elements enclosed in square brackets where each element is separated with a comma.
Syntax of a list is-
a=[1,2,4.5,’Python’,’Java’]
We can print the list and check its type using-
print(a) print(type(a))
The list is mutable which means we can change its elements.
Accessing the value of lists:
The values inside a list can be accessed using slicing.
The syntax for the same is-
print(list_name[start:end:step])
For example-
a=[1,2,4.5,'Python','Java'] print(a) print(type(a)) print(a[0]) print(a[1:]) print(a[1:4]) print(a[1::2])
INPUT-

OUTPUT-

WAYS TO CREATE A LIST
Let us look at the three different ways of creating a list in Python-
- Declaring a list
The first way of creating a list is the one we understood in the example which is given at the beginning of this article-
list = [2,3,’C’,7.5,’C++’]
- The second way to create a list is-
a=list() a=[2,3,'C',7.5,'C++'] print("Type of a is {}".format(type(a))) print(a)
INPUT-
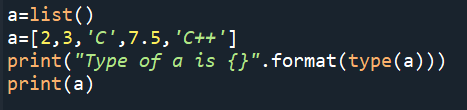
OUTPUT-

- The next way we will discuss creating a list in python is using-
a=[ ]
INPUT-

OUTPUT-

Updating our list –
The list that we are using for our understanding is-
list = [2,3,’C’,7.5,’C++’]
Now we will look at the methods that can update the values in our list.
So, the first way to update a list is to change a particular value using its index-
Here we will change the third value of our list which is the string 'C'. Since the indexing starts from 0, we will update the value by writing-
list[2]=’Java’ print(list)
INPUT-
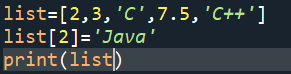
OUTPUT-

2. Using the append() method.
The append() method adds a value at the end of the string.
This can be done by-
list = [2,3,’C’,7.5,’C++’] list.append(‘Java’) print(list)
INPUT-

OUTPUT-

The last method that we will discuss here is adding a value using the insert() method.
The only thing that we must keep in our mind while using this method is here we must specify two things-
- The value that we want to add to the list.
- The position at which we want the value to be added.
So, in the given list we will insert the string value ‘Java’ at the fourth position, we will write the following program to implement the same-
list = [2,3,’C’,7.5,’C++’] list.insert(3,’Java’) #specifying index and value print(list)
INPUT-

OUTPUT-

List Operations-
Now we will learn about some basic list operations that make programming in python interesting.
Following is our list and we will apply these operations to it.
list = [2,3,’C’,7.5,’C++’]
- len- the len operation tells us about the length of our list or we can also say that it returns the number of elements present in our list.
print(len(list))
- Concatenation or Joining two lists – We can join two lists using the “+” operator.
list1=[1,4,'Practice',9.5,'Programming'] print(list+list1)
- Multiplication or Repetition – If we want a certain string value to be repeated, we can use “*” operator.
list=[2,3,'C',7.5,'C++'] a=list[2] print(a*2)
- Membership Operator – The membership operators that we can use in the list are 'in' and 'not in'. They check whether a particular element is present in the list or not.
list=[2,3,'C',7.5,'C++'] print(2 in list) print(7.5 not in list)
- Maximum/Minimum – The ‘max’ and ‘min’ operation returns the maximum and minimum element of the list.
l1=[23,11,45,66,77] print("Maximum in the list is {}".format(max(l1))) print("Minimum in the list is {}".format(min(l1)))
- sorted – The sorted operation returns a new list in which all the elements are present in ascending order.
l1=[23,11,45,66,77] print("Sorted order of list is {}".format(sorted(l1)))
- list – The list operation returns each iterable in a list.
list1=list("Programming") print("Iterables of list are {}".format(list1))
List Methods
- append() – This method inserts an element at the end of the list.
Example – list.append(obj)
- count() – It tells the occurrence of an element in the list.
Example – list.count(obj)
- index() – The lowest index of an element is returned when we apply this method to our list.
Example-list.index(obj)
- insert() – This method inserts an element at the specified position.
Example-list.insert(index,obj)
- reverse() – This method reverses the elements of our list.
Example-list.reverse().
- sort()-This method sorts the elements of our list.
Example-list.sort()
- pop()-This method removes the element from the specified position in the list.
Example-list.pop([index]).