Reverse a Number in Python
Python is an Object-Oriented high-level language. Python has an English-like syntax, which is very easy to read and write codes. Python is an interpreted language which means that it uses an interpreter instead of the compiler to run the code. The interpreted language is processed at the run time thus takes less time to run. Python is also interactive, so we can directly run programs in the terminal itself.
There are some basic and standard questions which are very popular are asked in almost every interview. The concept of these questions is important and can be used in multiple other questions. One of these questions is to Reverse a Number in Python.
In this question, we will be given a number in integer format and the task is to return the reversed number. We are going to solve these questions with three approaches:
- Iteration
- Recursion
- String Slicing
1. Reverse a number by iteration:
We will take the input as an integer and store it in a variable. Then we run a while loop and store the last digit of the given number in a different variable and then remove that digit from the original number. At last, we will print the reversed number.
Before writing the actual code itself it is advised to write the pseudo code or algorithm for the problem. After writing and understanding the algorithm it becomes very easy to execute the code.
Let us try to write the pseudo code first:
Pseudo Code:
-
Input : Number
-
Initialize rev_num = 0
-
Loop while Number>0
-
Digit = Number%10
-
rev_num = (rev_num*10) + Digit
-
Number = Number/10
-
-
Print Number
Now, writing the code for this pseudocode in Python is quite easy for us:
Code:
# Input the number to be reversed
number = int(input("Enter a number: "))
# Initiate the rev_num as 0
rev_num = 0
# Implement the logic in while loop while Number > 0
while(number>0):
digit = number % 10
rev_num = (rev_num * 10) + digit
number = number//10
# print rev_num
print("The reverse number is : {}".format(rev_num))
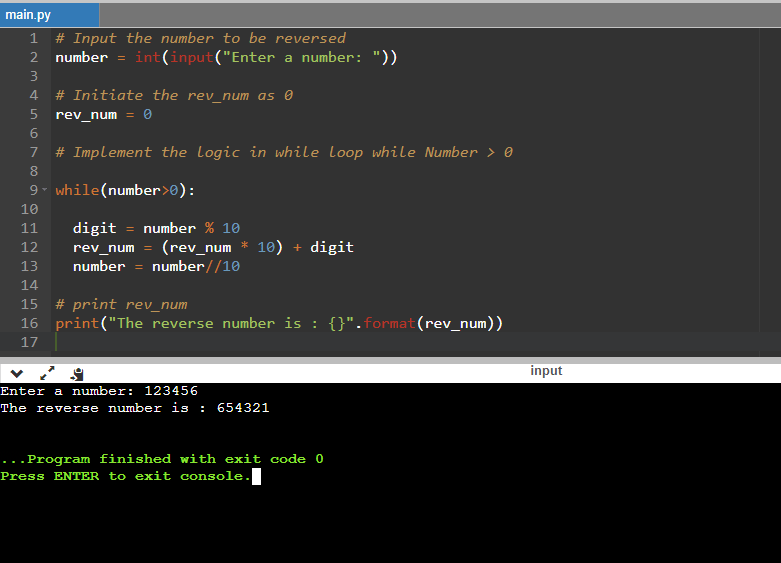
Explanation:
In the first line of the code, we have taken the input of the number. After that, we have initialized a variable rev_num as 0 in this variable we are going to store our answer.
The main logic starts from the while loop, run the while loop till the number is greater than 0.
Inside the while loop first, we get the last digit of the number by taking the remainder after dividing the number by 10.
Then we add the digit to the rev_num variable and also shift it to left in each iteration.
Then divide the number by 10 to get the second last digit in the next iteration.
This loop will run until the number is greater than 0. And finally, we print the rev_num.
We will better understand this program by taking an example and looking at each iteration step by step:
Number = 12345, rev_num = 0
First Iteration
Digit = number%10 è 12345%10 = 5
Rev_num = (rev_num*10) + digit
Rev_num = (0*10) + 5 = 5
Number = number//10 => 12345//10 = 1234
Second Iteration
After the first iteration, we have number = 1234 and rev_num = 5, since the number is greater than 0, while the loop will run again:
Digit = number%10 è 1234%10 = 4
Rev_num = (rev_num*10) + digit
Rev_num = (5*10) + 4 = 54
Number = number//10 => 1234//10 = 123
Third Iteration
After the second iteration, we have number = 123 and rev_num = 54, since the number is greater than 0, while the loop will run again:
Digit = number%10 è 123%10 = 3
Rev_num = (rev_num*10) + digit
Rev_num = (54*10) + 3 = 543
Number = number//10 => 123//10 = 12
Fourth Iteration
After the second iteration, we have number = 12 and rev_num = 543, since the number is greater than 0, while the loop will run again:
Digit = number%10 è 12%10 = 2
Rev_num = (rev_num*10) + digit
Rev_num = (543*10) + 2 = 5432
Number = number//10 => 12//10 = 1
Fifth Iteration
After the second iteration, we have number = 1 and rev_num = 5432, since the number is greater than 0, while loop will run again:
Digit = number%10 è 1%10 = 1
Rev_num = (rev_num*10) + digit
Rev_num = (5432*10) + 1 = 54321
Number = number//10 => 1//10 = 0
Sixth Iteration
After the second iteration, we have number = 0 and rev_num = 54321, since the number is 0, while the loop will not run and the code will just print the rev_num.
Print(rev_num)
54321
2. Recursive approach
The next approach to print the reverse of a number is by using recursion. Recursion is a method of solving a problem when the problem is defined in terms of itself.
The logic will be the same, instead of in this approach we will define a function and then call the function with the number//10.
Let us look at the code:
number = int(input("Enter the number: "))
rev_num = 0 # initial value is 0. It will hold the reversed number
def reversesum_recur(number):
global rev_num # We can use it out of the function
if (number > 0):
Digit = number % 10
rev_num = (rev_num * 10) + Digit
reversesum_recur(number // 10)
return rev_num
rev_num = reversesum_recur (number)
print(“Reverse of entered number is ", rev_num)
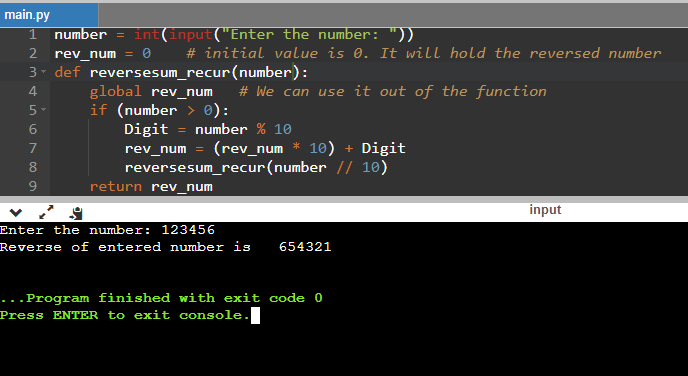
3. String Slicing
The third approach is to use slice slicing, this method can only be used in Python. This is one of the advantages of Python, that it provides some efficient techniques. With the help of slicing, we can access a range of elements and can also choose where to start and where to end and in which order.
Code:
number = int(input(“Enter the number: ”))
number = str(number)
print(number[::-1])
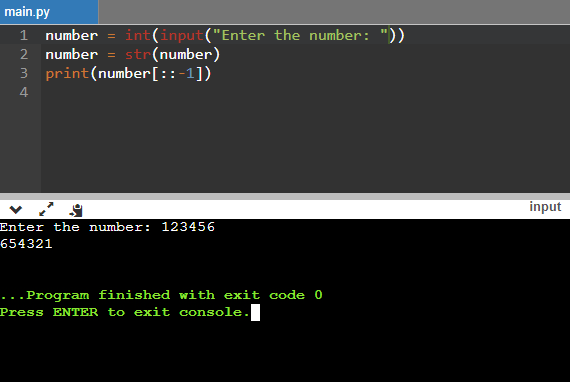
In this code, first, we have converted the integer number to a string. Then we have used slicing, the syntax for the slicing is start:stop:step . We have not passed anything to start and stop so it will take the whole string. The step is specified as -1, which means it will process the strip backward.
These are the methods to reverse a number in python. The most important task is to understand the logic behind the code, once you do that you can write code in every language.