Accessing Key-value in Dictionary in Python
A Python word reference is an assortment of key-esteem matches where each key is related to worth. Worth in the key-esteem pair can be a number, a string, a rundown, a tuple, or considerably another word reference. As a matter of fact, you can involve a worth of any substantial kind in Python as the worth in the key-esteem pair. A key in the key-esteem pair should be unchanging. As such, the key can't be changed, for instance, a number, a string, a tuple, and so on.
Python utilizes the wavy supports {} to characterize a word reference. Inside the wavy supports, you can put zero, one, or many key-esteem matches.
The accompanying model characterizes an unfilled word reference:
empty_dict = {}
Normally, you characterize an unfilled word reference before a circle, either for a circle or a while circle. Also, inside the circle, you add key-esteem matches to the word reference.
To find the sort of a word reference, you utilize the sort() capability as follows:
empty_dict = {}
print(type(empty_dict))
Ouptut:

The accompanying model characterizes a word reference with some key-esteem matches:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
The individual word reference has five key-esteem coordinates that address the primary name, last name, age, most loved tones, and dynamic status.
Getting to values in a Dictionary
To get to a worth by key from a word reference, you can utilize the square section documentation or the get() strategy.
1) Using square section documentation
To get to a worth related with a key, you place the vital inside square sections:
dict[key]
The following shows how to get the values associated with the key first_name and last_name in the person dictionary:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
print(person['first_name'])
print(person['last_name'])
Output:

2) Using the get() method
If you attempt to access a key that doesn’t exist, you’ll get an error. For example:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
ssn = person['ssn']
Error:
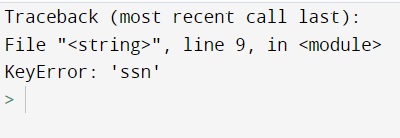
To avoid this error, you can use the get() method of the dictionary:
Person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
ssn = person.get('ssn')
print(ssn)
Output:

There is no such thing as on the off chance that the key, the get() technique returns None as opposed to tossing a KeyError. Note that None no method worth exists. The get() technique likewise returns default esteem when the key doesn't exist by, passing the default worth to its subsequent contention.
The accompanying model returns the '000-00-0000' string if the ssn key doesn't exist in the individual word reference:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
ssn = person.get('ssn', '000-00-0000')
print(ssn)
Output:

Adding new key-value pairs
Since a word reference has a powerful design, you can add new key-esteem matches to it whenever. To add another key-esteem pair to a word reference, you determine the name of the word reference followed by the new key in square sections alongside the new worth. The accompanying model adds another key-esteem pair to the individual word reference:
person['gender'] = 'Female'
Modifying values in a key-value pair
To change a worth related with a key, you determine the word reference name with the vital in square sections and the new worth related with the key:
dict[key] = new_value
The accompanying model changes the worth related with the age of the person dictionary:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
person['age'] = 26
print(person)
Output:

Removing key-value pairs
To eliminate a key-esteem pair by a key, you utilize the del explanation:
del dict[key]
In this language structure, you determine the word reference name and your desired key to eliminate.
The accompanying model eliminates the key 'active' from the person’s dictionary:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 26,
'favorite_colors': ['blue', 'green'],
'active': True
}
del person['active']
print(person)
Output:

Looping through a dictionary
To look at a word reference, you can utilize a for the circle to emphasize its key-esteem coordinates, or keys, or values.
1) Looping all key-value pairs in a dictionary
Python word reference gives a strategy called items() that profits an item which contains a rundown of key-esteem matches as tuples in a rundown.
For instance:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
print(person.items())
Output:

To emphasize overall key-esteem matches in a word reference, you utilize a for a circle with two variable keys and worth to unload each tuple of the rundown:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
for key, value in person.items():
print(f"{key}: {value}")
Output:
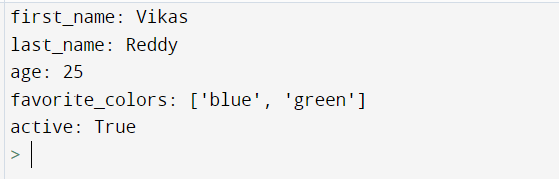
2) Looping through all the keys in a dictionary
Some of the time, you simply need to circle through all keys in a word reference. For this situation, you can utilize a circle with the keys() technique.
The keys() strategy returns an item that contains a rundown of keys in the word reference.
For instance:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
for key in person.keys():
print(key)
Output:
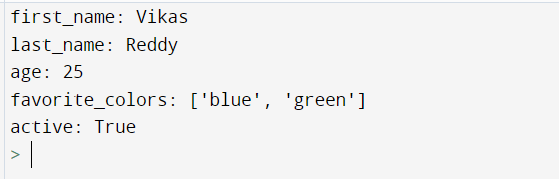
In fact, looping through all keys is the default behaviour when looping through a dictionary. Therefore, you don’t need to use the keys() method.
The following code returns the same output as the one in the above example:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
for key in person:
print(key)
Output:
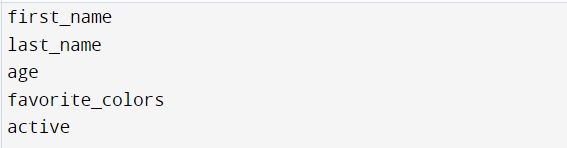
3) Looping through all the values in a dictionary
The values() method returns a list of values without any keys. To loop through all the values in a dictionary, you use a for loop with the values() method:
person = {
'first_name': 'Vikas',
'last_name': 'Reddy',
'age': 25,
'favorite_colors': ['blue', 'green'],
'active': True
}
for value in person.values():
print(value)
Output:
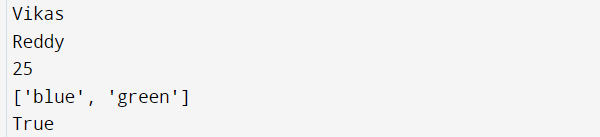
Outline
- A Python word reference is an assortment of key-value matches, where each key has a related worth.
- Utilize square sections or get() strategy to get to a worth by its vital.
- Utilize the del proclamation to eliminate a key-value pair by the key from the word reference.
- Use for loop to emphasize over keys, values, and key-value matches in a word reference.