Application to get live USD/INR rate Using Tkinter in Python
Tkinter:
The standard Python technique for building Graphical User Interfaces (GUIs) is Tkinter, which is included in all popular Python distributions. The only framework included in the Python standard library is this one. On top of Tk, this Python framework serves as a thin object-oriented layer that provides access to the Tk toolkit. The Tk toolkit is a cross-platform set of "graphical control elements," also called widgets, used to build application interfaces. This framework gives Python users a quick and easy way to build GUI elements with the Tk toolkit's widgets. In a Python application, Tk widgets can create buttons, menus, data fields, etc.
Once created, these graphic elements can be connected to or work with other widgets, features, functionality, processes, or data.
Tkinter bridges the gap between Python's execution model and Tcl's, built around cooperative multitasking. Like Python, Tcl is an interpreted dynamic programming language. Although it is an overall programming language that can be used independently, it is most frequently used in C programs as a scripting motor or as an interaction with the Tk toolbox. The Tcl library has a C interface that enables users to add custom Tcl or C commands, run Tcl commands and scripts, and manage one or more Tcl interpreter instances. Each interpreter has a queue for events that can be used to send and process events.
Syntax:
from tkinter import *
from tkinter import ttk
Advantages of Tkinter:
This promotes flexibility in handling GUI incidents, which makes the code base run more smoothly. Qt is more than just a framework; it uses a variety of native platform APIs for networking, database development, and other uses. Through a unique API, it gives them direct access.
Disadvantages of Tkinter:
Tkinter doesn't have any advanced widgets. There isn't a tool like Qt Designer for Tkinter in it. Its user interface is unreliable. Tkinter can be challenging to debug at times. It's not entirely in Python.
The libraries imported were:
- Tkinter
- BeautifulSoup
BeautifulSoup:
A Python package called Beautiful Soup can extract data from XML and HTML files. It provides natural means of traversing, searching, and altering the parse tree in conjunction with your preferred parser. Programmers frequently save days or hours of labour thanks to it. There is a tonne of unstructured data and information readily available today. The readily accessible data can be challenging to read at times. Web scraping is a highly helpful technique for turning unstructured information into data that is simpler to read and analyse, regardless as to how the data is currently available. In other words, web scraping is one method for gathering, arranging, and analysing this massive amount of data. Therefore, let's first define web-scraping. Data extraction, copying, and filtering are all just parts of the scraping process. Web-scraping refers to the process of collecting information or streams from the internet. The process of extracting data from the web is called web scraping, also known as information extraction or web harvesting. In essence, web scraping gives programmers a mechanism to gather and examine internet data.
The Beautiful Soup is a Library for python that bears the same name as a poem by Lewis Carroll from "Alice's Adventures in Wonderland." A Python tool called "Beautiful Soup" helps organise and arrange complicated web data by repairing terrible HTML and presenting it to us in clearly navigable XML structures by parsing the undesirable material, as the name implies.
CODE:
from tkinter import *
import requests
from bs4 import BeautifulSoup
def getdata(url):
r = requests.get(url)
return r.text
def get_info():
try:
htmldata = getdata("https://finance.yahoo.com/quote/usdinr=X?ltr=1")
soup = BeautifulSoup(htmldata, 'html.parser')
mydatastr = ''
for table in soup.find_all("div", class_="D(ib) Va(m) Maw(65%) Ov(h)"):
mydatastr += table.get_text()
list_data = mydatastr.split()
temp = list_data[0].split("-")
rate.set(temp[0])
inc.set(temp[1])
per_rate.set(list_data[1])
time.set(list_data[3])
result.set("success")
except:
result.set("Opps! something wrong")
master = Tk()
master.configure(bg='light grey')
result = StringVar()
rate = StringVar()
per_rate = StringVar()
time = StringVar()
inc = StringVar()
Label(master, text="Status :", bg="light grey").grid(row=2, sticky=W)
Label(master, text="Current rate of INR :",
bg="light grey").grid(row=3, sticky=W)
Label(master, text="Increase/decrease by :",
bg="light grey").grid(row=4, sticky=W)
Label(master, text="Rate change :", bg="light grey").grid(row=5, sticky=W)
Label(master, text="Rate of time :", bg="light grey").grid(row=6, sticky=W)
Label(master, text="", textvariable=result,
bg="light grey").grid(row=2, column=1, sticky=W)
Label(master, text="", textvariable=rate,
bg="light grey").grid(row=3, column=1, sticky=W)
Label(master, text="", textvariable=inc, bg="light grey").grid(
row=4, column=1, sticky=W)
Label(master, text="", textvariable=per_rate,
bg="light grey").grid(row=5, column=1, sticky=W)
Label(master, text="", textvariable=time,
bg="light grey").grid(row=6, column=1, sticky=W)
b = Button(master, text="Show", command=get_info, bg="Blue").grid(row=0)
mainloop()
CODE EXPLANATION:
Importing all the required modules to get processed[winapps]. Apps method is attached for output. Use set() method to set the data like the name of the application, version of the application, installation date of the application, publisher of the application. The uninstalling string required for the application and set background using configure()[light grey] ,create variable classes in tkinter[name , version , publisher , install_date , publisher, uninstall_string then create label for each information using label() these labels() are positioned by using grid() method[select row as per the design. We also create entry box to enter the application using entry(). In entry box we also insert buttons, rows , columns , checkbox and lot many.
OUTPUT:
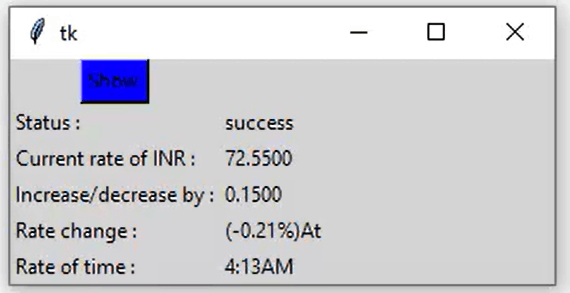