Pointers in Python
In this tutorial, we will study what pointers are and if they have any utility in python.
Now, let us understand what pointers are
Pointers
Pointers are special variables used to store the addresses of another variable. It is generally used in programming languages such as C and C++. These are very useful but are quite complicated. They are represented using an asterisk ‘*’.
Syntax to declare a pointer in C:
datatype *a1
For Example - int *a1;
float *b1
The syntax to assign the address of a variable to a pointer is:
datatype a1, *a2;
a2=&a1;
For Example – int a1, *a2;
a2=&a1;
Four types of pointers are there in C and C++
- Null Pointers
- Void Pointer
- Dangling pointers
- Wild pointers
Pointers in Python
Python does not support pointers.
Keeping python user-friendly has been the main idea behind its development. Python has provided several in-built libraries just to ease the work of users. Adding pointers in python would add to the complexities, which would go against the idea of python development. Thus, pointers are not supported in python.
Although python does not support pointers explicitly, still, users can get the benefits of pointers in python. There are various ways to put on pointers in python.
We will see two of them here:
- Usage of mutable types as pointers
- Usage of Python custom objects
By the Usage of mutable types as pointers
void add_one(int *a) {
*a += 1;
}
In the above snippet of C language, the code receives a pointer to an integer(*a) and then increases the value by 1.
The below code shows the main function to carry out the add_one function operation on an integer.
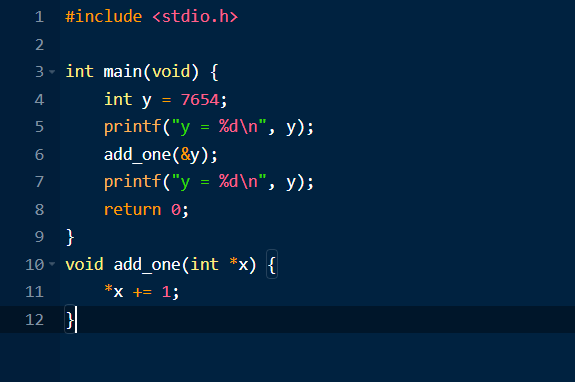
Explanation: In the above code, value = 7654 has been assigned to y, and that value is printed first. Further, this value is incremented by one, and the modified value is printed.
Output:
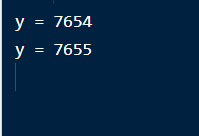
Explanation: Foremost y contains an unmodified value and the second y contains the modified value.
Now, let’s try to achieve the same in python.
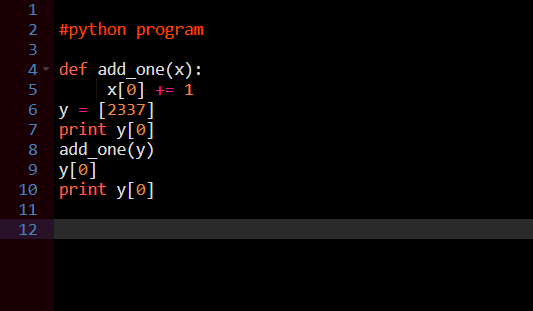
Explanation: In the above code, a mutable list has been used to do the increment.
In this case, the add_one(x) function accesses the unmodified value i.e., 2337, and increments its value by one. Finally, we get a modified value at last.
Thus, we have achieved our desired task without using pointers, as in C.
The modification was possible because of the use of the list, which is of mutable data type.
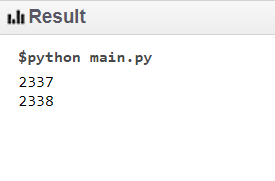
This figure shows the output of the above python code.
Explanation: Here,the first line contains the unmodified value, and the second line contains the modified values.
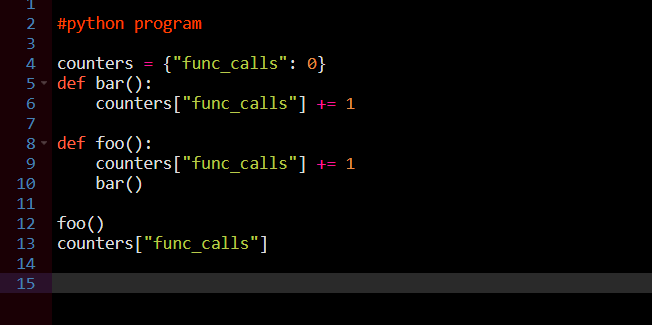
Explanation - In the above example, we have used the dictionary named counter, which keeps a record of the overall quantity of function calls. When the function named foo() is called, the counter value increases by 2 because the dictionary is a mutable data type.
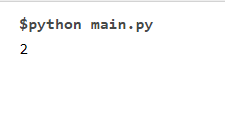
Explanation: it shows the output of the python code written above.
By the usage of Python custom objects
The dictionary
option is a great way to compete with pointers in Python, but sometimes it gets monotonous to recall the key name used. If the dictionary has been used in various parts of the application it gets even more difficult. We can use a custom class instead of a dictionary to overcome this issue.
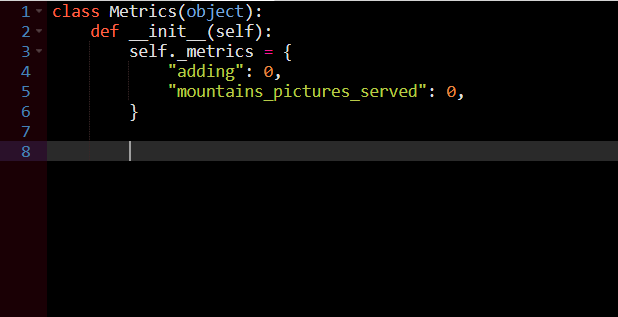
Explanation: The above code expresses a Metrics
class that still uses a dictionary
to hold the real data, which is in the _metrics
member variable. This will give the changeability needed by the user. It is now needed to be able to access these values.
It can be performed through properties:
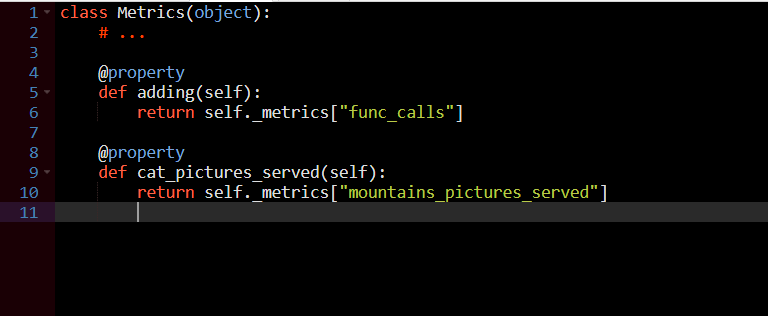
Explanation: The code here is making use of @property. The @property
decorator here allows accessing functions - adding
and mountains_pictures_served
.
Now, we will create an object that is the instance of the pointer class defined above.
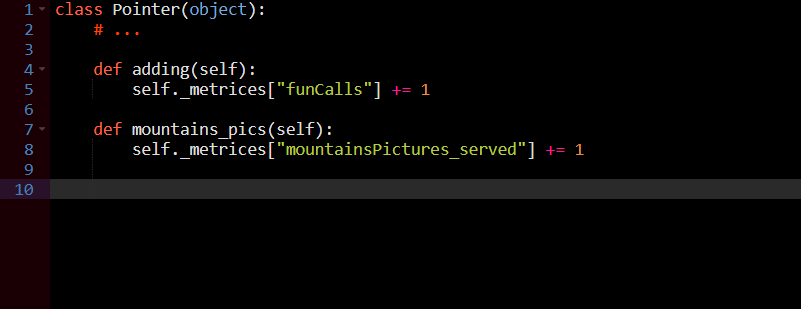
Explanation: The code written above shows the creation of an object of the pointer.
Here also, the values are getting incremented.
Two new functions have been used:
- adding()
- mountains_pics()
These two functions are capable of modifying the values in the metrics dict
. Now we have created a class that can be modified just like the pointers get modified.
Here, one can access both of these functions adding() and mountains_pic() to simulate pointers in Python.
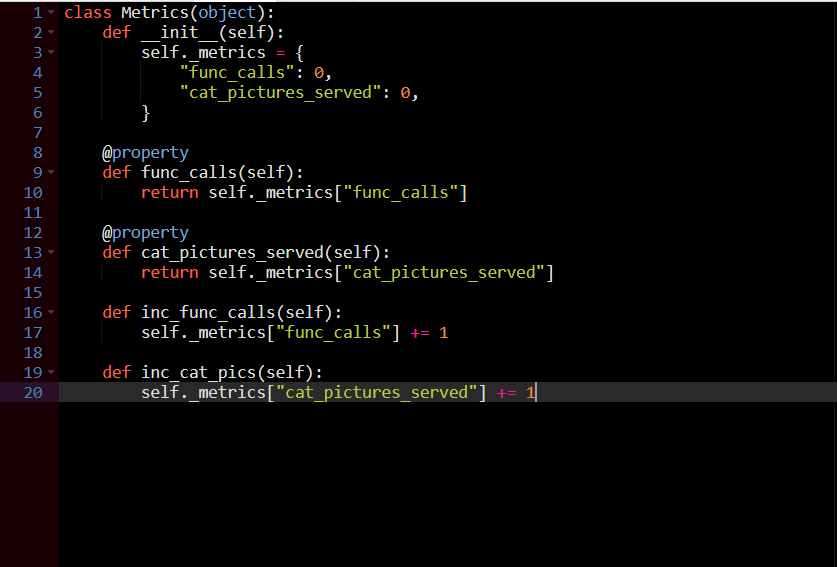
Explanation: The above code contains the full code of the Metrics class.