_dict_ in Python
An unordered collection of data values known as a dictionary can be used in Python to store data values similar to a map. Dictionaries can also store a key: value pair, in contrast to other data types, which can only store a single value for each element. An option to increase the dictionary's efficiency is Key-Value.
Regarding attribute access, most users only have experience with the dot "." (as in x.any attribute).
Attribute access, very simply, is the process of acquiring an object that is associated with the one you currently possess. Without getting too deep into the specifics, it might appear to someone who uses Python to be extremely straightforward. For this very straightforward operation, there are numerous activities taking place in the background.
Introduction to _dict _ :
Each module has a unique attribute called dict that holds the module's symbol table. An item's (writable) attributes are kept in a dictionary or another mapping object.
Every Python object contains a property denoted by the symbol __dict__. Additionally, this object possesses all of the attributes that have been listed for it. __dict__ can also be referred to as a mapping proxy object. By giving a class object the __dict__ property, we can use the dictionary.
Syntax:
object.__dict__
Code 1:
# class 'vegetables' is defined here
class vegetables:
def __init__(self): # constructor here
# keys initialized here
self.Carrot = 'Orange'
self.Ladyfinger = 'Green'
self.Tomato = 'Red'
def Show(self):
print("The Dictionary from object fields belongs to the class vegetables :")
# object vegetable of class vegetables here
vegetable= vegetables()
# calling Show function
vegetable.Show()
# calling the attribute __dict__ on vegetables
# making it print it
print(vegetable.__dict__)
Output:
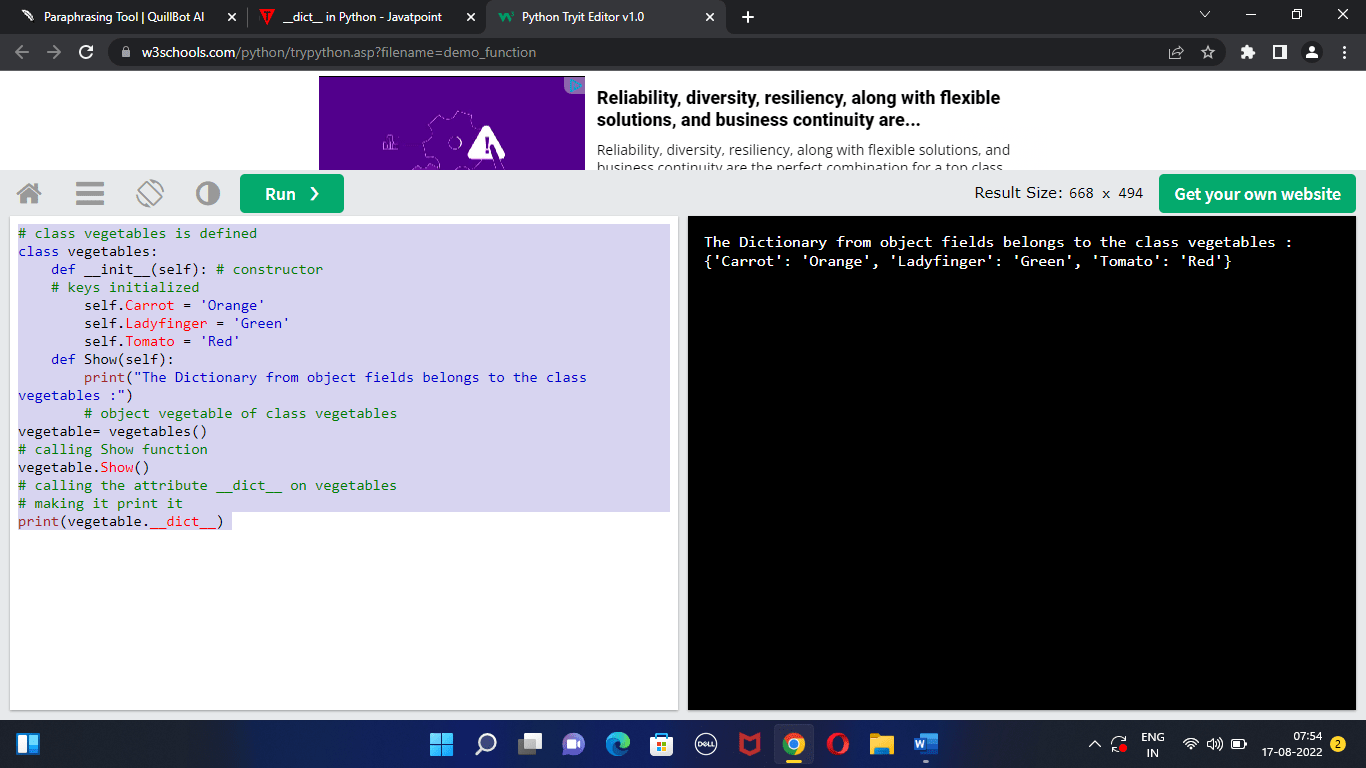
Code 2:
class Grade1_Class:
def __init__(self,Name,age):
self.Name = Name
self.age = age
def feature(self):
if self.age == "7":
return True
else:
return False
Object1 = Grade1_Class('Anika','7')
print(Object1.__dict__)
Output:
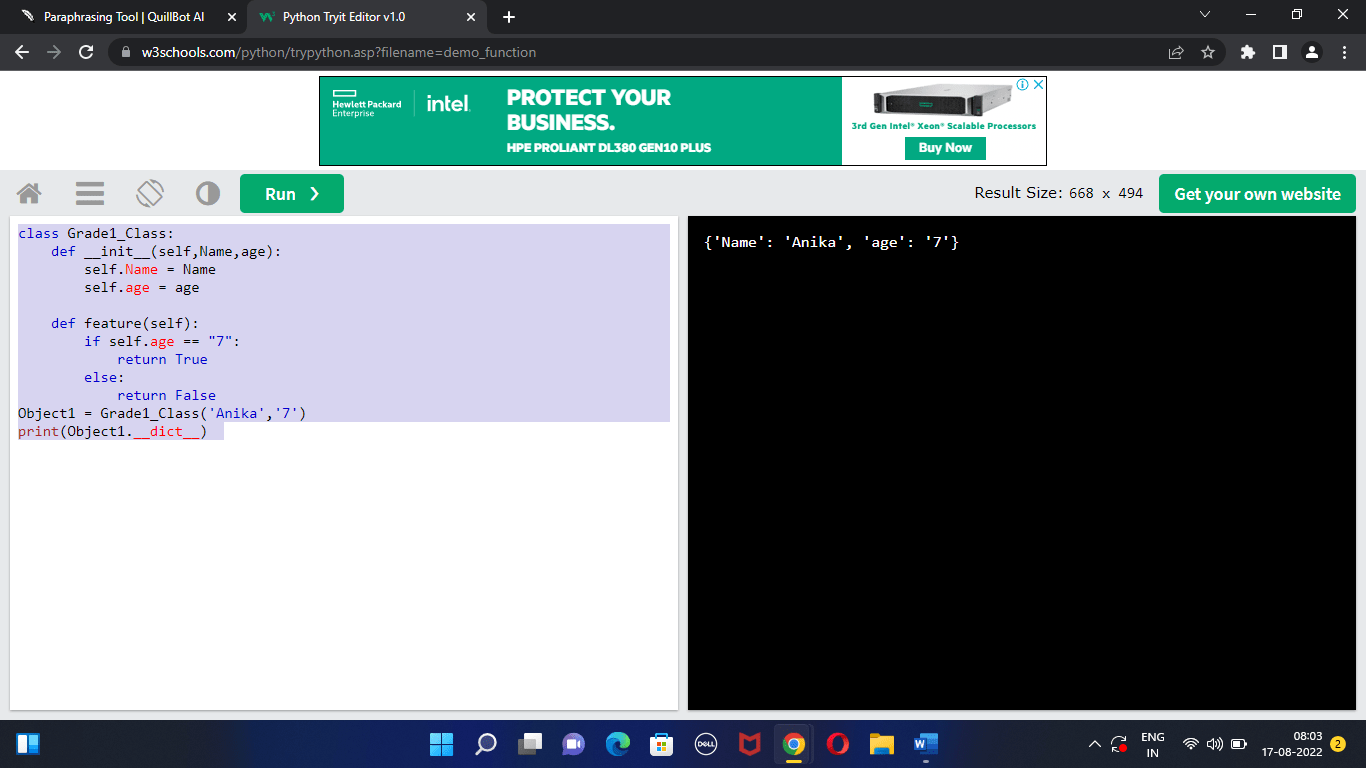
Using Python's Dictionary Without Using __dict__ :
To Create a Dictionary:
Python allows you to create dictionaries from lists of entries by enclosing them in curly brackets and separating them with commas. Dictionary pairs consisting of a key and a key:value pair are saved as pairs, each of which is stored separately. Values in dictionaries can be any data and duplicated, unlike keys, which cannot be repeated and must be immutable.
In curly brackets, the structure is enclosed, the elements are separated by commas, and each key and value are separated by colons (:). A dictionary without any elements is possible.
The dictionary's contents can be of any type, but its keys must be immutable, such as integers, tuples, or strings.
Code 3:
# Creating a Dictionary here
dictionary = {1: 'I', 2: 'Love', 3: 'Myself'}
print("\nCreating a Dictionary by using Integer Keys : ")
print(dictionary)
# Creating a Dictionary
# various Mixed keys here
dic1= {'Address': 'Rajasthan', 7: [1, 2, 3, 4]}
print("\nCreating a Dictionary by using Mixed Keys : ")
print(dic1)
Output:
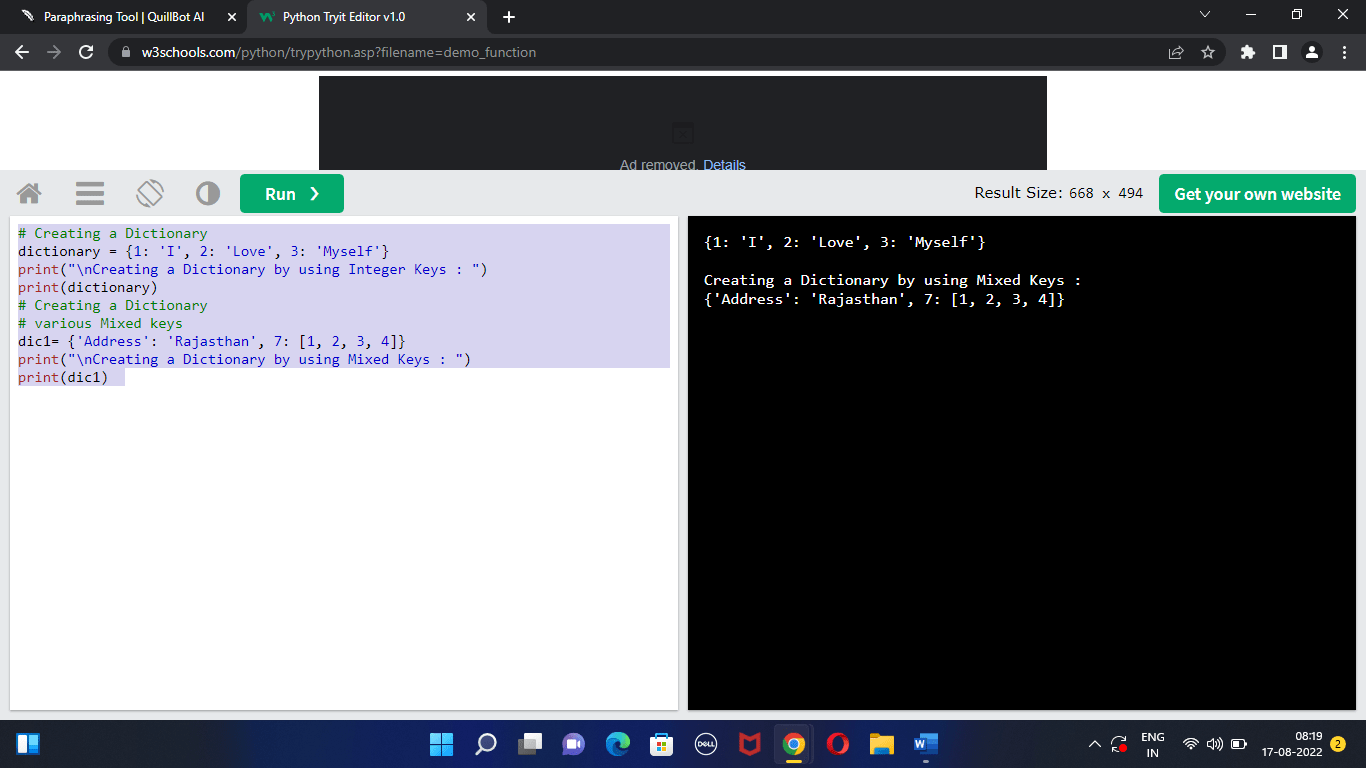
Note: The built-in method dict() can also be used to create dictionaries. Remember a dictionary will be empty if two curly braces are simply combined.
Code 4:
# Creating - an empty Dictionary
Edict = {}
print("An Empty Dictionary: ")
print(Edict)
# Creating Dictionary - using the dict() method
dictionary = dict({1: 'One', 2: 'Two', 3: 'Three'})
print("\nCreating a Dictionary by using the dict() method : ")
print(dictionary)
# Creating a Dictionary - using each item as a different Pair
dict2 = dict([(1, 'First'), (2, 'Second')])
print("\nCreating a Dictionary by using each item as a different pair : ")
print(dict2)
Output:
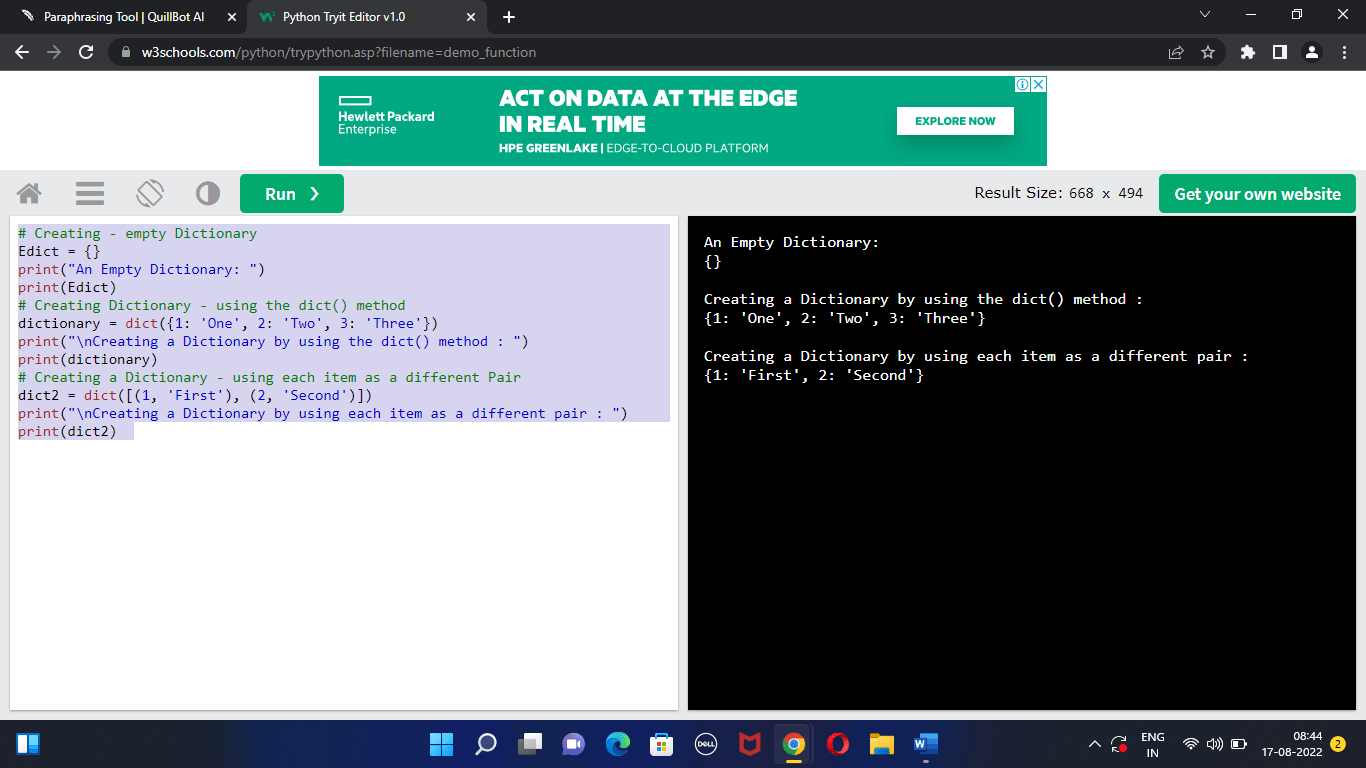
Adding new Elements to it:
A Python dictionary can be expanded with elements using a number of different methods. Combining the key and value results in Dict[Key] = "Value," which may be used to add one value at a time to a dictionary. An existing value in a Dictionary can be changed using the built-in update() function. An existing dictionary can also be expanded using nested key values.
It should be noted that when a value is added if the key-value pair already exists, the value is updated. If not, the dictionary receives a new key and value.
Code 5:
Dict1 = {} # Empty Dictionary
# Adding only one element at a time
Dict1[0] = 'Nice'
Dict1[1] = 'To'
Dict1[2] = 'Cook'
print("\nDictionary after the addition of some elements: ")
print(Dict1)
# A group of values are added to a certain Key.
Dict1['Grade'] = 7, 8, 9
print("\nDictionary after the adding a set of values to a key: ")
print(Dict1)
# Updating the existing Key's Value
Dict1[2] = 'Dance'
print("\nDictionary after Updated key value: ")
print(Dict1)
# Adding Nested Key value
Dict1[3] = {'Nested' :{'A' : 'Sing', 'B' : 'Play'}}
print("\n After Addition of a Nested Key our dictionary looks like: ")
print(Dict1)
Output:
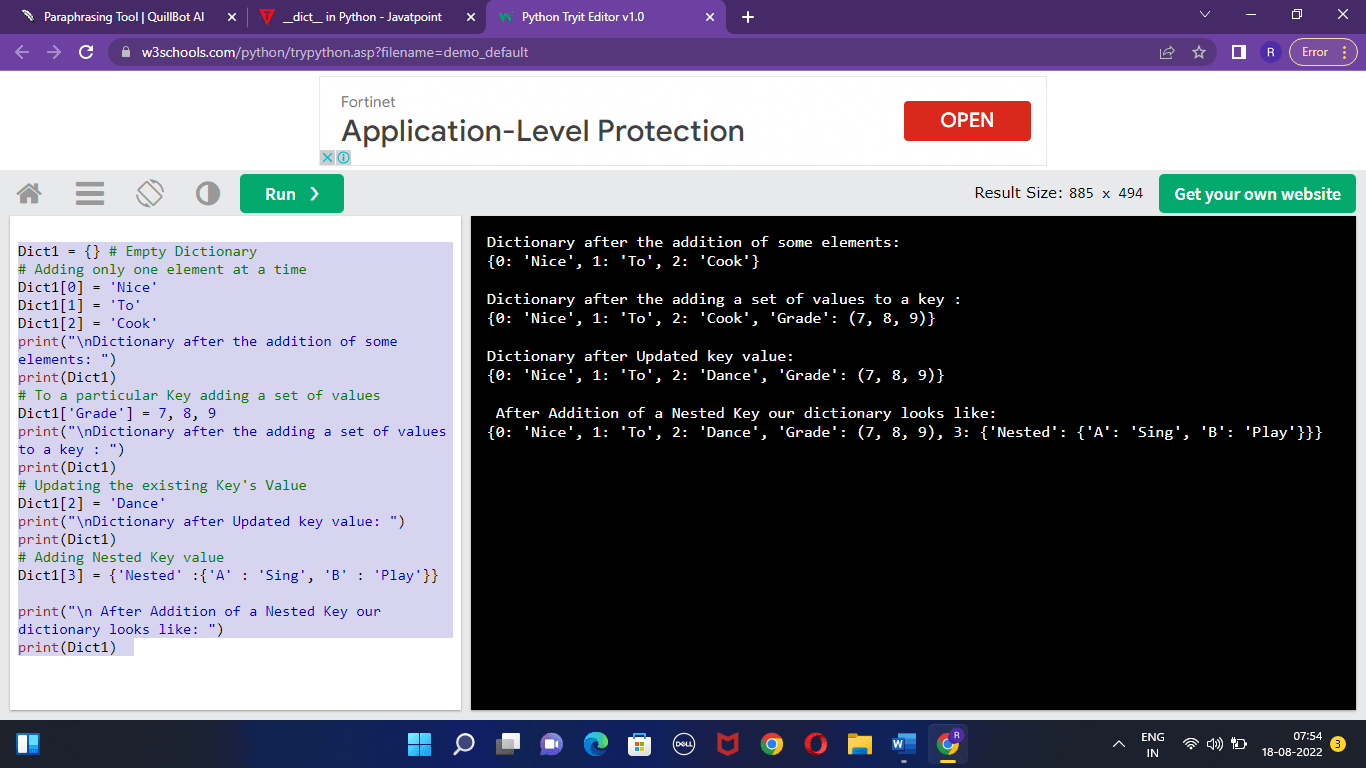
Dictionary Elements Accessing:
A dictionary employs keys, whereas other data structures require indexing to access values. Keys can be used in square brackets [ or with the get() method.
If a key is entered using square brackets [], KeyError is produced if the dictionary does not include the key. In contrast, if the key cannot be found, the get() function returns None.
Code 6:
# Dictionary is created
dictionary = {1: 'My', '2': 'Name', 3: 'is', 4: 'Ruzela'}
# using key - # accessing an element
print("Accessing an element using the key:")
print(dictionary['2'])
print("Accessing some other element using the key:")
print(dictionary[1])
# using the get() method - accessing an element
print("Accessing an using the get() method:")
print(dictionary.get(4))
print("Accessing another using the get() method:")
print(dictionary.get(3))
Output:
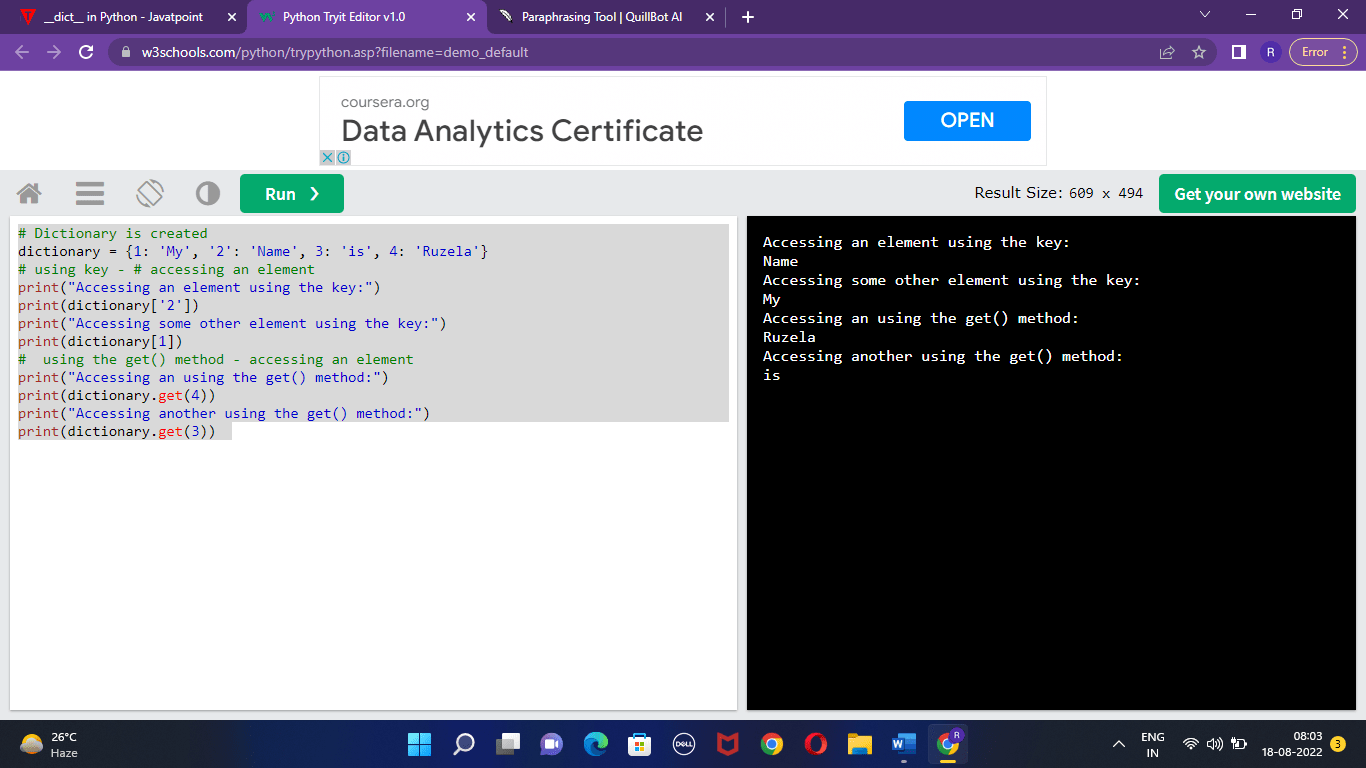
To obtain the value of a key that already exists in the nested dictionary, we can use the indexing [] technique.
Code 7:
# Dictionary created
Dictionary = {'dict1': {1: 'Apple'},
'dict2': {2: 'Orange'}}
# Accessing the elements using the key
print(Dictionary['dict1'])
print(Dictionary['dict1'][1])
print(Dictionary['dict2'][2])
Output:
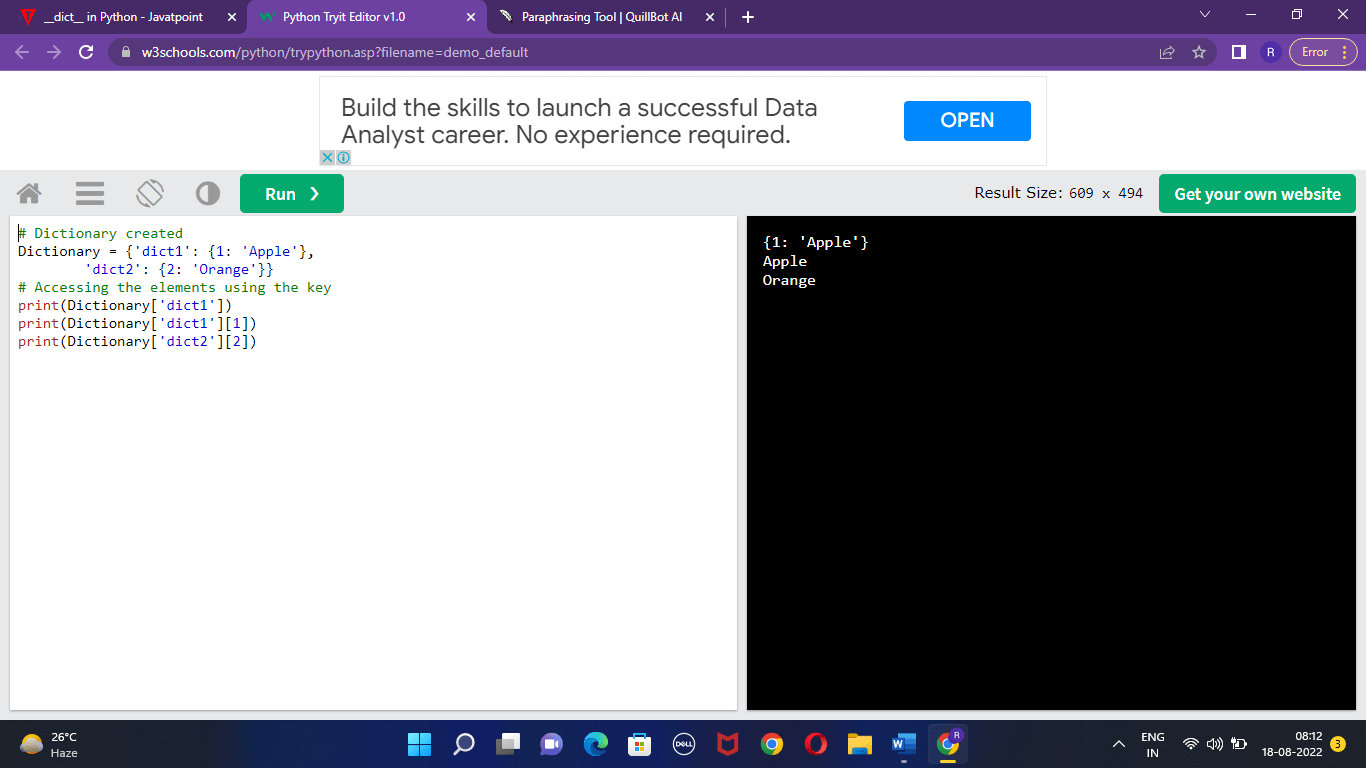
Differentiating between a List and a Dictionary
Lists and dictionaries are two examples of data structures that are fundamentally different. A list can contain an ordered sequence of elements such that we can index into it or iterate over it. Lists can be altered after they have been created since they are a changeable type. The Python dictionary implements hash tables and key-value storage. It doesn't require any particular order and calls for hashable keys. Additionally, key lookups are completed quickly.
The Following Characteristics are Found in a List's Elements:
- Unless otherwise specified, they remain in the existing order (for instance, by sorting the list).
- They could be of any kind or even a mix of several kinds.
We can access them by using numerical (zero-based) indexes.
The Following are the Features of Dictionary Elements:
- There are values and keys for each entry.
- There is no warrant for orders.
- To access elements, key values are needed.
- Key values may be of any hashtable type (other than a dict), and types may be concatenated.
- Any value type is acceptable, including other dicts, and types can be merged.
Note: When we have a collection of unique keys and values, we use a dictionary; however, when we have an ordered collection of items, we use a list.
Code 8: Code Containing Some Build-In Dictionary Methods:
# Dictionary created
dict1={1:"My",2:"Name",3:"is",4:"Ruzela"}
dict2=dict1.copy() #copy method
print(dict2)
dict1.clear() #clear method
print(dict1)
print(dict2.items()) #items method
print(dict2.keys()) #keys method
print(dict2.get(1)) #get method
dict2.pop(4) #pop method
print(dict2)
dict2.popitem() #popitem method
print(dict2)
print(dict2.values()) #values method
dict2.update({4:"Rupsha"}) #update method
print(dict2)
Output:
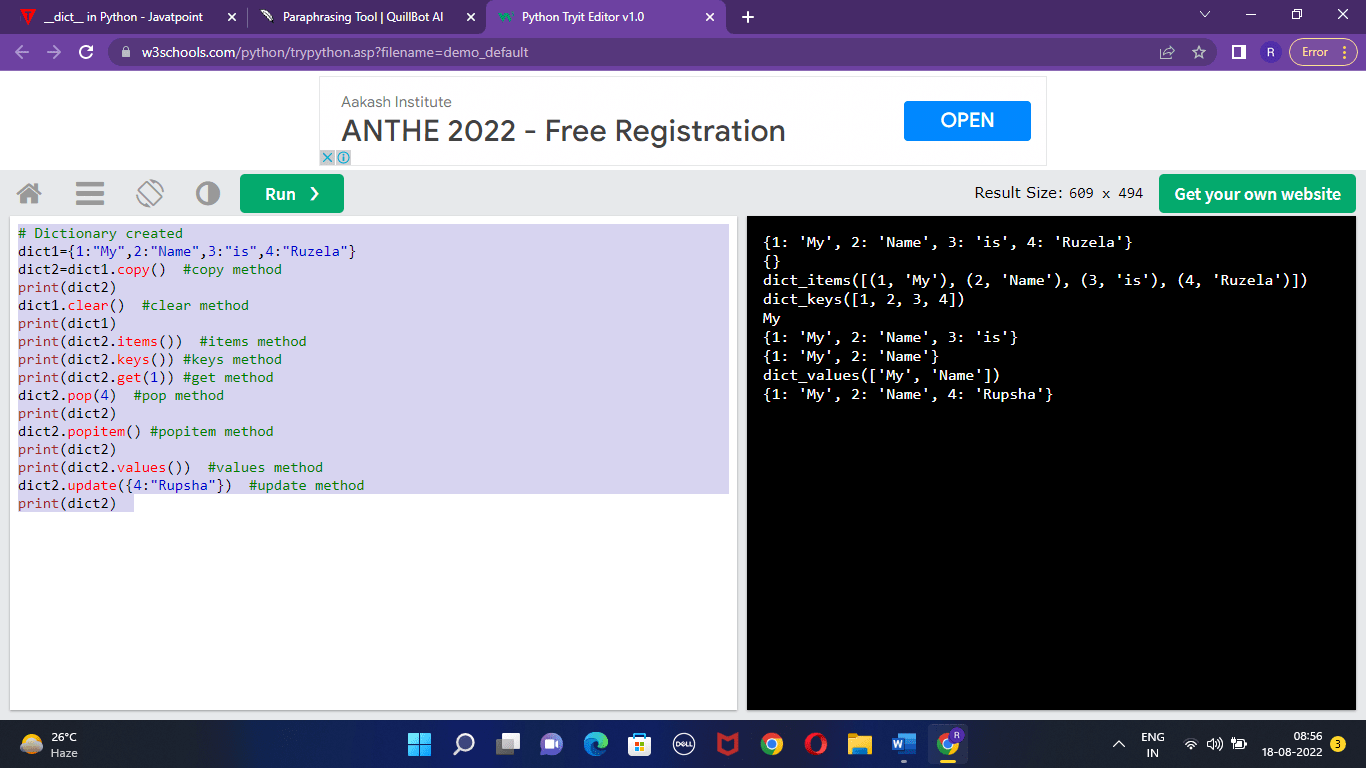
Conclusion:
- Dictionaries are a type of data structure used in computer languages to store information that is somehow connected.
- Each module has a special attribute called __dict__.
- The symbol table for the module is located in __dict__.
- A mapping object contains the attributes of an element.
- In Python, each object has a property denoted by the symbol __dict__.
- The term "mapping proxy object" is another name for the object __dict__.
- Keys and Values are the two terms for a Python dictionary's two parts.
- You might not receive your data back in the exact order you supplied because dictionaries don't maintain their data in any particular order.
- Keys will only have one component.
- Values may take the form of integers, lists, lists within lists, etc.
- The dictionary's keys must be immutable, such as integers, tuples, or strings, but its contents can be of any type. Duplication of keys is not permitted; each key may only be used for one entry.
- Since dictionary keys are case-sensitive, the same key name spelt differently in Python dictionaries is viewed as a separate key.