Write Dictionary to CSV in Python
Python is an Object-Oriented high-level language. Python has an English-like syntax, which is very easy to read and write codes. Python is an interpreted language which means that it uses an interpreter instead of the compiler to run the code. The interpreted language is processed at the run time thus takes less time to run. Python is also interactive, so we can directly run programs in the terminal itself.
Comma Separated Files are very common while working with data in Python. They store data in a tabular form in which values are separated by commas. We can easily transfer data with CSV files to software like Google Sheets, Microsoft Excel. We can easily handle CSV files in Python with the in-built CSV module.
In this port, we are going to discuss how to write a dictionary to CSV using Python.
Writing CSV files
We can easily write and create CSV files using an in-built module called CSV in Python. The CSV.writer() function is used to write the CSV files. We can also CSV.DictWriter() class to write dictionary into a CSV file.
Using csv.Writer()
The CSV module contains the writer function that is used to write in CSV files. We can write anything from a single row, lists to dictionaries. The syntax for the function is:
csv.writer
(csvfile, dialect='excel', **fmtparams)
This function returns a writer object which converts the data into a delimited string. Here, argument csvfile is a CSV file that has WRITE permission. The other parameter is dialect. Dialect is an optional parameter used to set parameters specific to a CSV dialect.
The functions writerow() and wroterows() are used to write rows in a CSV sheet.
Code to Write CSV
import CSV
field_names = ['No', 'Student', 'Class']
students = [
{'No': 1, 'Student': 'Rahul', 'Class': 'Tenth'},
{'No': 2, 'Student': 'Rohan', 'Class': 'Eleventh'},
{'No': 3, 'Student': 'Rishik', 'Class': 'Twelfth'},
{'No': 4, 'Student': 'Ravi', 'Class': 'Tenth'},
{'No': 5, 'Company': 'Rajneesh', 'Class': 'Eleventh'},
]
with open('Example1.csv', 'w') as csvfile:
writer = csv.writer(csvfile)
for dicton in students:
for key, value in dicton.items():
writer.writerow([key, value])
Output
The following content will be present in a file called Example1.csv after running the CSV:
No | 1 |
Student | Rahul |
Class | Tenth |
No | 2 |
Student | Rohan |
Class | Eleventh |
No | 3 |
Student | Risk |
Class | Twelfth |
No | 4 |
Student | Ravi |
Class | Tenth |
No | 5 |
Company | Rajneesh |
Class | Eleventh |
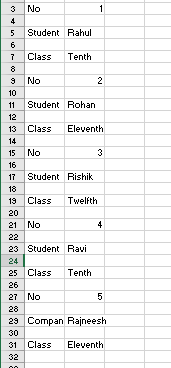
As you can see, this is not the ideal output. We do not want our data in this format most of the time. To get the desired output, we have to use another class instead of CSV.writer().
But first, let us understand the above code.
- In the first line of code we have imported the module csv.
- The second line defines the fieldnames which are the column heading for the csv file.
- After that we have defined our data which we want to write in the csv file. The data is in the dictionary format. The data looks like this:
students = [
{'No': 1, 'Student': 'Rahul', 'Class': 'Tenth'},
{'No': 2, 'Student': 'Rohan', 'Class': 'Eleventh'},
{'No': 3, 'Student': 'Rishik', 'Class': 'Twelfth'},
{'No': 4, 'Student': 'Ravi', 'Class': 'Tenth'},
{'No': 5, 'Company': 'Rajneesh', 'Class': 'Eleventh'},
]
- In the next line of code, we have opened the Example1.csv file in WRITE mode as the csvfile.
- Then we have used the csv.writer() function to write the CSV file, which returns a writer object.
- Then we are first writing the header of the CSV file, which is stored in a list called field_names, and then we are looping through our list of dictionaries one by one and writing the data to the CSV file.
Using csv.DictWriter()
As we discussed earlier, we have to use the CSV.DictWriter() to write the dictionary in a CSV file in the ideal format. The syntax of the DictWriter class is:
class
csv.DictReader
(file, fieldnames=None, restkey=None, restval=None, dialect='excel', *args, **kwds)
This returns an object which maps the data in each row of the dictionary to the given key defined by the fieldnames parameter.
The fieldnames are the optional parameter. If not defined, the first row of the file will be the field name for the file.
All other arguments are optional.
Code to Write CSV
import CSV
field_names = ['No', 'Student', 'Class']
students = [
{'No': 1, 'Student': 'Rahul', 'Class': 'Tenth'},
{'No': 2, 'Student': 'Rohan', 'Class': 'Eleventh'},
{'No': 3, 'Student': 'Rishik', 'Class': 'Twelfth'},
{'No': 4, 'Student': 'Ravi', 'Class': 'Tenth'},
{'No': 5, 'Company': 'Rajneesh', 'Class': 'Eleventh'},
]
with open('Names.csv', 'w') as csvfile:
writer = csv.DictWriter(csvfile, fieldnames = field_names)
writer.writeheader()
writer.writerows(students)
Output
Running this code will create a CSV file named Names.csv with the following content:
No | Student | Class |
1 | Rahul | Tenth |
2 | Rohan | Eleventh |
3 | Risk | Twelfth |
4 | Ravi | Tenth |
5 | Rajneesh | Eleventh |
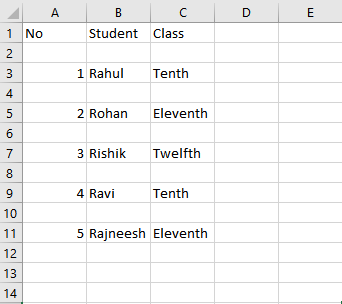
Let us explain the above code line by line:
- In the first line of code we have imported the module csv.
- The second line defines the fieldnames which are the column heading for the csv file.
- After that we have defined our data which we want to write in the csv file. The data is in the dictionary format. The data looks like this:
students = [
{'No': 1, 'Student': 'Rahul', 'Class': 'Tenth'},
{'No': 2, 'Student': 'Rohan', 'Class': 'Eleventh'},
{'No': 3, 'Student': 'Rishik', 'Class': 'Twelfth'},
{'No': 4, 'Student': 'Ravi', 'Class': 'Tenth'},
{'No': 5, 'Company': 'Rajneesh', 'Class': 'Eleventh'},
]
- In the next line of code, we have opened the Names.csv file in WRITE mode as the csvfile.
- Then we have used the DictWriter class to write the CSV file, which returns a writer object.
- Then we are writing the column header with the function writeheader() and then the actual data with the writerows method.