Python Comment Block
In this tutorial, we will see what comment blocks mean in Python. Further, we will see the commenting methods supported in Python. We will understand the topics deeply with the aid of a few examples.
Through Programming, creativity and the level of thinking of one’s mind get reflected. Good programming is the one that is written in easy-to-understand language, as well as whose debugging can be done quickly. One should be able to reuse it whenever they want in the future. Commenting is a good way of showcasing the thought flow, which can later help understand the intention behind the particular line of code.
Understanding the comment
Commenting is done to make the code more readable, and it helps the third person to understand the code without much difficulty. It would be not easy to understand the code in the future if commenting is not done. Python takes # into account to write the comment.
In other programming languages, including JavaScript, Java, and C++, which consider the following /*... */ for multiline comments, there is no built-in mechanism for multiline comments in Python.
In Python, multiple lines are commented using hash ( # ).
Now, let us understand the meaning and difference between good and bad comments.
Considering the below example of a bad comment (not self-explanatory) -
a = 78 # assigning ‘a’ the value of 78
b = 62 # assigning ‘b’ a value of 62
The following example shows a more descriptive comment which is more beneficial.
gst10 = 1.10 # defining a GST of 10%
gst20 = 1.20 # defining a GST of 20%
Python offers two ways of commenting –
1. Single line comment – This comment begins with a hash character (#) and is followed by text that contains added explanations.
Let us understand this kind of comment through an example.
Example 1:
# Defining the total marks
Total_marks = 850
Example 2:
# defining the overall structure of the product with default values
product = {
}
Example 3:
# it is a comment
print(“bonjour world!”)
Example 4:
#print("It is a good place to live in")
print("It is a good place to live in!")
Output:
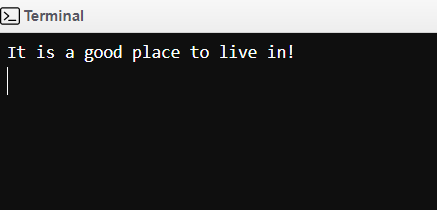
Example 4: Comments can be positioned even at the end of a line in the following manner:
print(("It is a good place to live in!")#it is a comment
Output:
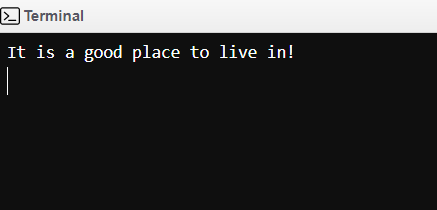
It is entirely vague for write the comment in a proper text format.
#print(“bonjour world!”)
print(“bonjour world!”)
Output:
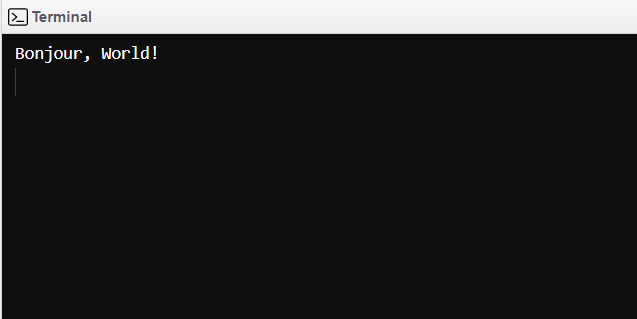
2. Multiline comments – A python is accomplished in understanding a single line comment and the total comment block. Python does not offer a separate operator; instead, the following methods achieve it.
Through the utility of Multiple Hashtags (#)
In Python, one can consider multiple hashtags (#) to write multiline comments. Here, each line will be regarded as a separate single-line comment.
Example:
# Python program to understand
# the syntax and working of
# multiline comments
print("Multiline comments using multiple hashtags")
Output:
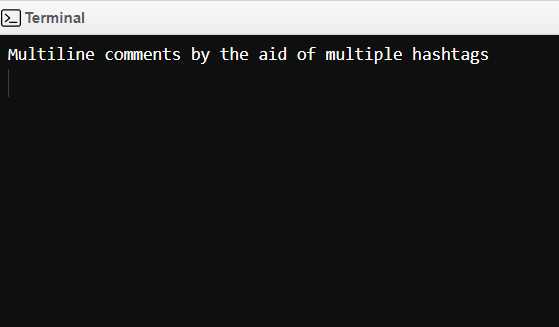
Using String Literals
One can use string literal as a comment as Python neglects the string literals that are not assigned to a variable so that we can use these string literals as a comment. A single-line comment in Python begins with the hashtag (#) symbol without any white spaces between them and ends at the end of the line.
Example 1: Using a single quote
'This particular line shall be neglected by Python language.'
Explanation: On executing the above code, we won’t receive any output, so a single quote can be used to comment out a statement in Python.
Example 2: Using triple quotes.
""" Python program to demonstrate
multiline comments"""
print("Multiline comments using triple quotes"
Output:
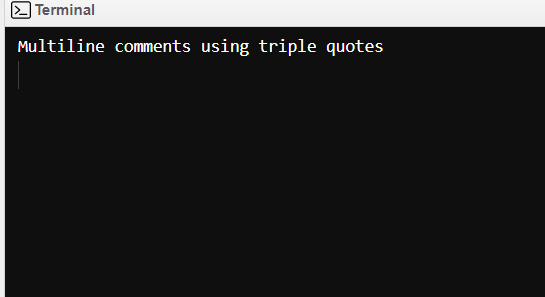
Officially, Python doesn't explicitly support multiline comments, so some consider the following options.
Version 1 of Python combines single-line comments.
# LinuxThingy version 1.6.5
#
# Constrains:
#
# -t (--text): display the text interface
# -h (--help): display this help
Version 2 is moderately simpler than version 1. It is intended to be used for the creation of documentation, but in addition, it can also be used for multiline comments.
“ “ “
LinuxThingy version 1.6.5
Constraints:
-t (--text) : display the text interface
-h (--help) : display this help
“ “ “
It should be noted that the latter version needs to be bounded within special quotation marks (" ") to work instead of hash characters.
Syntax:
# This is a "block comment" or a "multiline" comment in Python,
# which is constructed
# out of several
# single-line comments.
# Isn’t it marvellous, yeah?
Example 1:
#This is a comment
#written in
#more than one line
print(“This is a good place to live in !”)
Output:
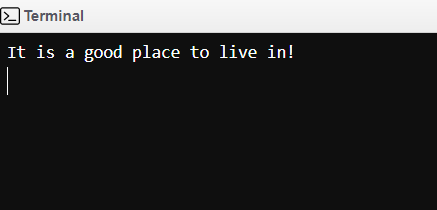
Example 2:
"""
This is a comment
written in
more than just one line
"""
print("Hello, World!")
Output:
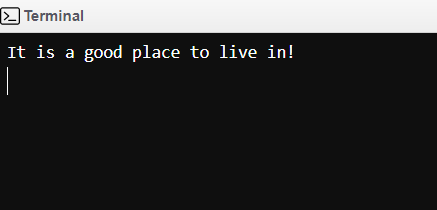
3. Documentation string
The documentation strings allow associating human-readable documentation with Python modules, functions, classes, and methods. They are not the same as source code comments.
More about them:
A docstring can either be a single-line or multiline comment. In a single-line comment, only one line is used to describe the code, and in the case of multiple lines, more than one line is used.
A docstring begins with an uppercase and ends with a period.
Now let us understand the documentation string with the aid of an example.
Example:
def addition(a, b, c):
"""adds the value of a, b and c""" return a+b+c
# Print the docstring of multiply function
print(addition.__doc__)
Output:
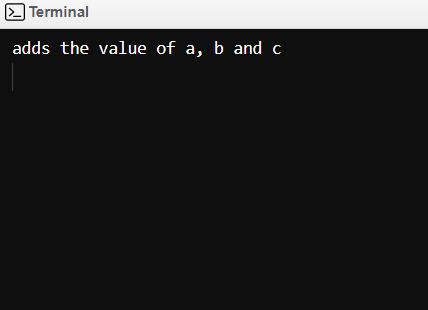
Common Practice
It is common to begin a python file with a specific line of comments. These lines prove helpful for the programmer as it contains the description of the project.
Most programming languages, such as C, Java, etc., use syntax for block comments that consist of numerous lines of text.
/*
This is a block comment.
It encompasses multiple lines
of code.
Good, that?
*/
Now, let us see the usage of comments in the actual program.
Example 1: Program to see the calendar of the given month and year.
# Python Program to show the calendar of the given month and year
# importing the calendar module
import calendar
y1 = 2022 # year
m1 = 10 # month
# To take month and year as input from the user
# y1 = int(input("Enter the year: "))
# m1 = int(input("Enter the month: "))
# displaying the calendar
print(calendar.month(y1, m1))
Example 2: Program to find the L.C.M of two numbers entered by the user.
# Python Program to calculate the L.C.M. of two numbers entered by the user
def calculate_lcm(x1, x2):
# choosing the larger number among the two
if x1 > x2:
larger = x1
else:
larger = x2
while(True):
if((larger % x1 == 0) and (larger % x2 == 0)):
lcm = larger
break
larg
er += 1
return lcm
num1 = 54
num2 = 24
print("The L.C.M. of the number is", calculate_lcm(num1, num2))
Summary:
In this tutorial, we have learned how to comment in Python. We saw various ways of commenting in Python.
It is not that difficult to write suitable comments in Python. One can easily do it with the power of understanding. Commenting in python is not that complicated, and just the power of endurance is needed. It supports all of us trying to comprehend the code, including the programmer himself, for when they visit their own code again. It becomes more accessible for the user to understand the code with the aid of comments.
We conclude the article with the hope that you understood the comment in python and learned how to use the comment in python.
We hope that the advice we have given you here makes creating better comments and documentation in your code more manageable.