Python Bitwise Operators
When used with variables and objects in an expression, operators are tokens that carry out calculations. The variables and items to which the computation is applied are known as operands. As a result, an operator needs some operands to work with.
The operators and their function are briefly described in the following lists. However, we shall concentrate on Bitwise Operators in this article.
- Unary Operators
Unary operators are those that only have one operand to work with. Unary Operators are listed below:- + Unary Plus
- - Unary Minus
- ~ Bitwise Compliment
- not Logical Negation
- Binary Operators
Binary operators are those that operate on two operands. Some binary operators are as follows:- Arithmetic Operators
- Subtraction
- + Addition
- * Multiplication
- / Division
- // Floor Division
- % Modulus/ Remainder
- ** Raise to the power/ Exponent
- Bitwise Operators
- & Bitwise AND
- ^ Bitwise Exclusive OR (i.e., XOR)
- | Bitwise OR
- Shift Operators
- << Shift Left
- >> Shift Right
- Identity Operators
- is -> is the identity same?
- is not -> is the identity not same?
- Relational Operators
- < Less than
- < Greater than
- <= Less than or Equal to
- >= Greater than or Equal to
- == Equal to
- != Not equal to
- Assignment Operators
- = Assignment
- /= Assign Quotient
- += Assign Sum
- *= Assign Product
- %= Assign Remainder
- -= Assign Difference
- **= Assign Exponent
- //= Assign Floor Division
- Logical Operators
- and Logical AND
- or Logical OR
- Membership Operators
- in whether variable is in the sequence
- not in whether variable not in sequence
Now let’s dig in detail about Python Bitwise Operators.
- Arithmetic Operators
- Python Bitwise Operators
In Python, bitwise operations are carried out on integers using bitwise operators. In order to carry out Bitwise operations In Python on integers, bitwise operators can be used. The Bitwise Operator is called so because its operations are carried out bit by bit once the integers are converted to binary. The outcome is then shown in decimal form.
One thing to pin-point is that, only integers are supported by Python's bitwise operators.
The Bitwise Operators and their Functions are Briefly Described in the Following List:- & Bitwise AND
- | Bitwise OR
- ^ Bitwise XOR
- ~ Bitwise NOT
- >> Bitwise Right Shift
- << Bitwise Left Shift
Syntax:
& -> a & b
| -> a | b
^ -> a ^ b
~ -> ~ a
>> -> a>>
<< -> <<b
Here, a and b are integers.
- Bitwise AND Operator( &)
It returns 1 in the case where the bits present are both 1; otherwise, returns 0.
For Example:
x = 4 (In Binary -> 0100)
y = 10 (In Binary -> 1010)
x & y = 0100 & 1010
= 0000 = 0 (decimal)
- Bitwise OR Operator ( | )
Returns 1 if either bit is 1, otherwise 0.
For Example:
x = 4 (In Binary -> 0100
y = 10 (In Binary -> 1010)
x | y = 0100 | 1010
= 1110 = 14 (decimal)
- Bitwise Not Operator ( ~ )
One's complement is returned of the number.
For Example:
x = 4 (In Binary -> 0100)
~ x = ~ 4 = ~ 0100
= - (0100 + 1)
= - 5 (decimal)
- Bitwise XOR Operator ( ^ )
If one of the bits is 1 and the other is 0, returns true; otherwise, returns false.
For Example:
x = 4 (In Binary 0100)
y = 10 (In Binary 1010)
x ^ y = 4 ^ 10 = 0100 ^ 1010
= 1110 = 14 (decimal)
Code:
x = 10
y = 4
print("x & y =", x & y) # bitwise AND operation
print("x | y =", x | y) # bitwise OR operation
print("~x =", ~x) # bitwise NOT operation
print("x ^ y =", x ^ y) # print bitwise XOR operation
Output:

Shift Operator
These operators shift the bits of a number to the left or right, multiplying or dividing it by two, accordingly. In the program,sometimes we need to multiply or divide a number by it and this is the case where we can use Shift Operators.
1.Bitwise left shift
Shifts the number's bits to the left, filling vacancies on the right with 0 as a result. Multiplying a number by a power of two has a similar effect.
For Example1:
x = 5 = 0000 0101 (In Binary)
x << 1 = 0000 1010 = 10
x << 2 = 0001 0100 = 20
For Example 2:
y = -10 = 1111 0110 ( In Binary)
y<< 1 = 1110 1100 = -20
y << 2 = 1101 1000 = -40
2. Bitwise right shift
Shifts the bits of the number to the right, filling the vacancies on the left with 0 (or 1 if the value is negative). The result is similar to dividing a number by a power of two.
For Example 1:
x = 10 = 0000 1010 ( In Binary)
x >> 1 = 0000 0101 = 5
For Example 2:
x = -10 = 1111 0110 ( In Binary)
x >> 1 = 1111 1011 = -5
Code:
a = 20
b = -15
print("a >> 1 =", a >> 1)# bitwise right shift operator --> print
print("b >> 1 =", b >> 1)#bitwise right shift operator --> print
a = 15
b = -8
print("a << 1 =", a << 1)#bitwise left shift operator --> print
print("b << 1 =", b << 1)#bitwise left shift operator --> print
Output:
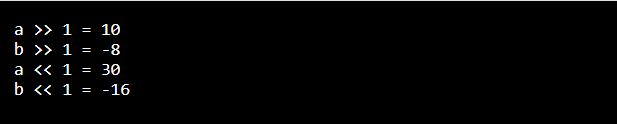
Overloading of the Bitwise Operator
Overloading an operator refers to giving them more meaning than their established operational meaning. For instance, the + operator can be used to combine two lists, join two strings, and add two integers.
It is feasible due to the fact that the int and str classes override the '+' operator. When the same built-in operator or method operates differently for objects of different classes, this is referred to as operator overloading.
A basic example of Bitwise operator overloading is shown below:
Code:
class OperOver():
def __init__(self, value):
self.value = value
def __and__(self, obj):
print("And operator overloaded")
if isinstance(obj, OperOver):
return self.value&obj.value
else:
raise ValueError("Must be a object of class OperOver")
def __or__(self, obj):
print("Or operator overloaded")
if isinstance(obj, OperOver):
return self.value | obj.value
else:
raise ValueError("Must be a object of class OperOver")
def __xor__(self, obj):
print("Xor operator overloaded")
if isinstance(obj, OperOver):
return self.value ^ obj.value
else:
raise ValueError("Must be a object of class OperOver")
def __lshift__(self, obj):
print("lshift operator overloaded")
if isinstance(obj, OperOver):
return self.value<<obj.value
else:
raise ValueError("Must be a object of class OperOver")
def __rshift__(self, obj):
print("rshift operator overloaded")
if isinstance(obj, OperOver):
return self.value&obj.value
else:
raise ValueError("Must be a object of class OperOver")
def __invert__(self):
print("Invert operator overloaded")
return ~self.value
if __name__ == "__main__":
a = OperOver(12)
b = OperOver(32)
print(a & b)
print(a | b)
print(a ^ b)
print(a << b)
print(a >> b)
print(~a)
Output:
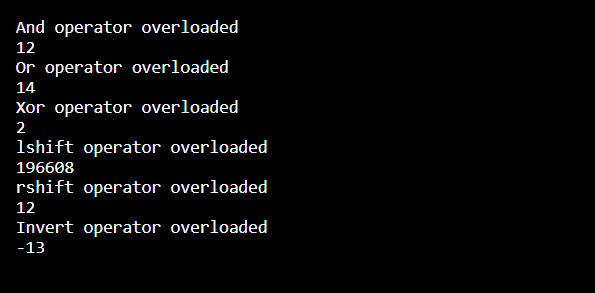