Difference between Package and Module in Python
What are Python modules?
A file with the “.py” suffix that includes Python or C executable code is known as a module. Multiple Python commands and expressions make compose a module. We can use pre-defined variables, functions, and classes thanks to modules. This shortens the development process and makes it possible to reuse code.
Making a Python Module
We may create a module by entering some code in a file and giving it the.py extension.
Create a Python module called pythoneg.py.
Python module illustration
def display()
print(“Python”)
if__name__=”__main__”:
display()
Output:
Python
Python Module Importing
Using the import keyword, we may import a module.
Python modules import illustration
import python
.display()
Output:
Python
Using the asterisk * operator, we may import each object in a module.
from python import *
display()
Output:
Python
Like that, we can import a single module function.
from pythonimport display
display()
Output:
Python
While importing a module, we may also alias it.
import python as pg
pg.display()
Output:
Python
Python's dir()
Dir() is a built-in function in Python. It takes the name of a module as input and gives output a sorted list of all the characteristics and functions found in the given module.
Python example of dir()
import python
print(dir(python))
Output:
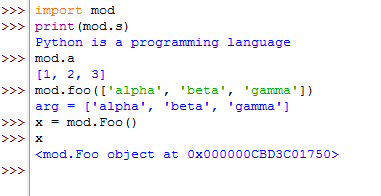
How to Import a Module in Python
Python starts by looking at the current directory before importing a module. The PYTHONPATH variable's list of directories is then searched if the module isn't found in the current directory. It then moves on to the default directory if it is still unable to locate the directory. If the module cannot be found in any directories, it raises a ModuleNotFoundError. We can view every directory in the PYTHONPATH variable using the following code.
A Python PYTHONPATH variable example
from os, import environ, paths
print(environ['PYTHONPATH'].split(pathsep))
Output:

Python packages
A Python package builds a hierarchical directory structure containing several modules and sub-packages to provide an environment for developing applications. They are merely a collection of related modules and packages.
A Package's Creation and Import
We must establish a directory containing a module and __init .py file to construct a Python package. Assume we have included the previously built module pythongeeks.py in a website package. We can import the website package by combining the import keyword with the dot operator.
For example: To import a package using Python
import website.python
python.display()
Output:
Python
We can alias while importing, just like with modules.
For example: To import a package using Python
import website. python as pg
.display()
Output:
Python
However, only the immediate modules of a package (and not the sub-packages) are imported when we import a package. If you attempt to access them, an Attribute Error will be raised.
Python Packages vs. Modules
Some of the differences between modules and packages are as follows:
- A Module is an a.py file containing Python code, but a Package is a directory containing many modules and sub-packages.
- A package must have a __init .py file to be created. When it comes to developing modules, there is no such requirement.
- Using the asterisk (*) operator, we can import every object in a module at once but not every module in a package.
A Python "module" includes a unit namespace, locally extracted variables, and some parsed functions like:
- Variables and constants
- any value, fresh or old
- Definitions of properties for classes
- Typically, a module corresponds to a single file.
- In the user interface library, a debugging tool.
Some frequently used technologies enable programmers to create new platforms with modules for improved code execution. Additionally, during runtime, this installs and distributes packages throughout the library.
Modules, packages, and functions
The idea is the same for modules, functions, and packages. They strive to make reusing code as simple as they can. Even though they behave and appear differently, their fundamental concept is the same.
A function in Python is composed of several statements and expressions. A Python module is made up of many Python functions, while a Python package is made up of several Python modules. This is demonstrated