Assignment Operators in Python
The prime usage of Assignment Operators is to assign values to variables.
These are taken into account to do operations on values and variables. There are some special symbols in python to perform arithmetic, logical, and bitwise computations. Operands are the values on which operators perform operations.
Following are the assignment operators used in python:
- Assign: operator used =
- Add and Assignment: operator used +=
- Subtract and Assignment: operator used -=
- Multiply AND Assignment: operator used *=
- Divide and Assignment: operator used /=
- Modulus and Assignment: operator used % =
- Divide (floor) and Assignment: operator used //=
- Bitwise AND and Assignment: operator used &=
- Bitwise XOR and Assignment: operator used !=
- Bitwise Right Shift and Assignment: operator used ^=
- Bitwise right shift Assignment: operator used >>=
- Bitwise left shift Assignment: operator used <<=
Let’s understand each of the above operators in detail
Assignment operator:
The utility of this operator is to simply assign the value present on the right side of the operator to the operand present on the left side.
Syntax:
z = x + y
Example: Showing the implementation of add, and assignment operator
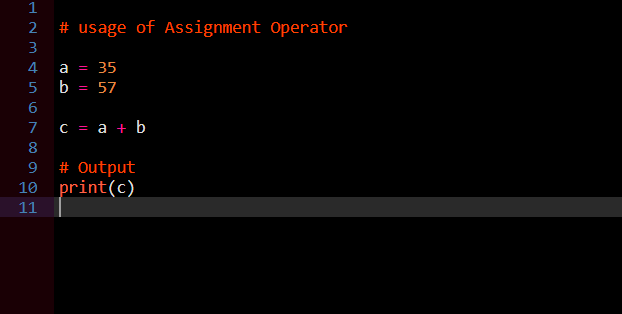
Output:
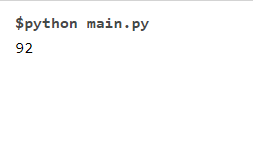
Add and Assignment operator:
The usefulness of this operator is to assign the result to the left operand after calculating the sum of values present on left and right side of the operator.
Syntax:
x + = y
Example 1: Showing the implementation of add and assignment operator
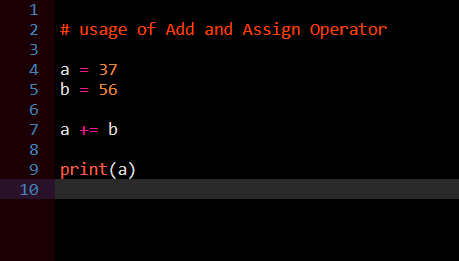
Output:
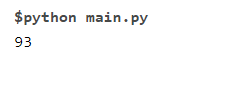
Example 2: Showing the implementation of add and assignment operator
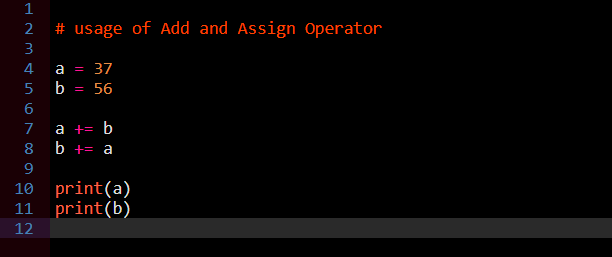
Output:
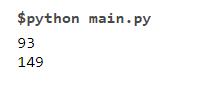
Subtract and Assignment:
The usefulness of this operator is to assign the result to the left operand after subtracting the values present on the right side.
Syntax:
x -= y
Example: Showing the implementation of subtract and assignment operator
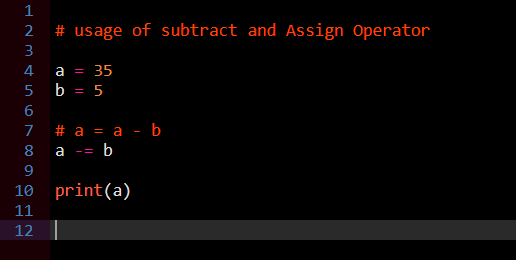
Output:
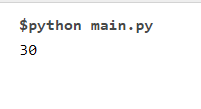
Multiply and Assignment:
The usefulness of this operator is to assign the result to the left side of the operator after obtaining the result on multiplying values present on the left and right sides of the operator.
Syntax:
x *= y
Example: Showing the implementation of multiply and assignment operator
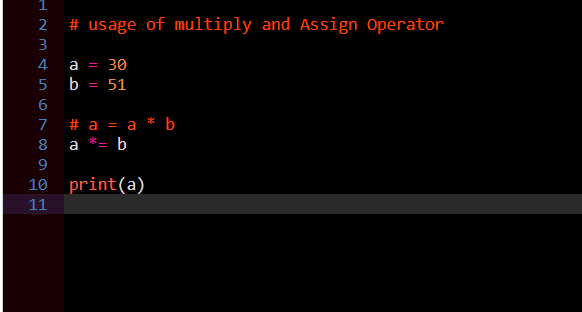
Output:
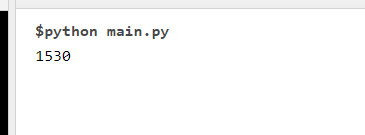
Divide and Assignment:
The utility of this operator is to assign the result in the variable present on the left side of operator. Here, the result is obtained after dividing the operand present on left side with the right operand.
Syntax:
x /= y
Example: Showing the implementation of divide and assignment operator
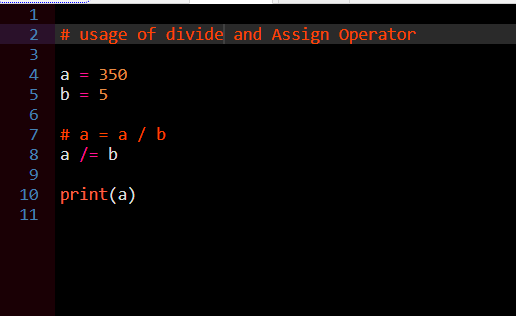
Output:
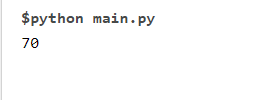
Modulus and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after finding out the modulus by dividing the left operand with the right one.
Syntax:
x %= y
Example: Showing the implementation of modulus and assignment operator
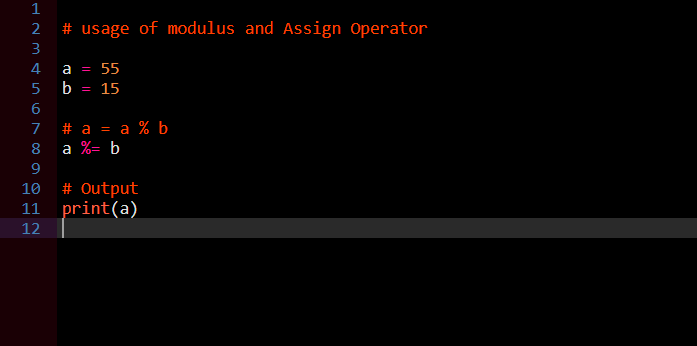
Output:
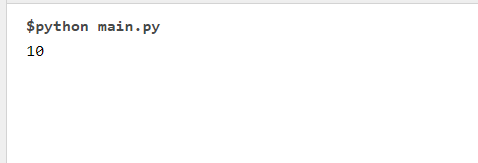
Divide (floor) and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after finding out the floor value by dividing the left operand with the right one.
Syntax:
x //= y
Example: Showing the implementation of divide(floor) and assignment operator
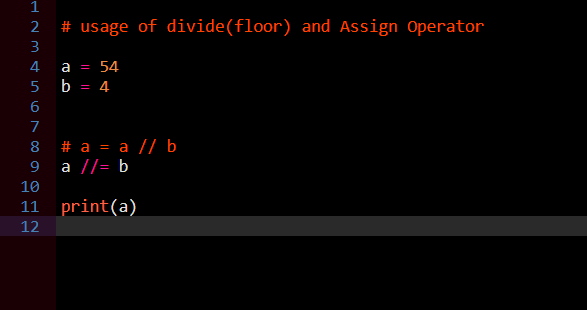
Output:
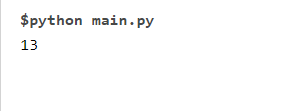
Exponent and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the exponent by raising the power to the left operand.
Syntax:
x **= y
Example: Showing the implementation of exponent and assign operator
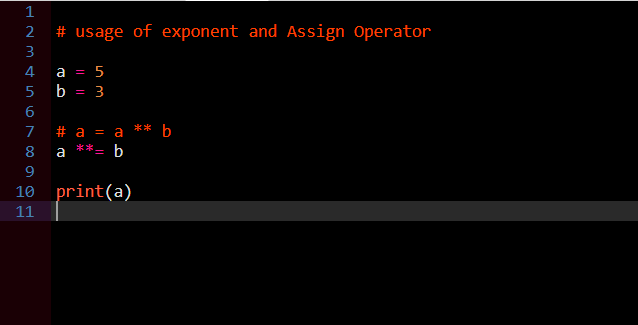
Output:
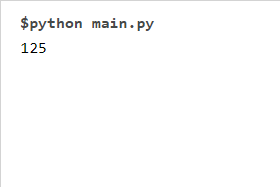
Bitwise AND and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the Bitwise AND.
Syntax:
x &= y
Example: Showing the implementation of Bitwise add and assignment operator
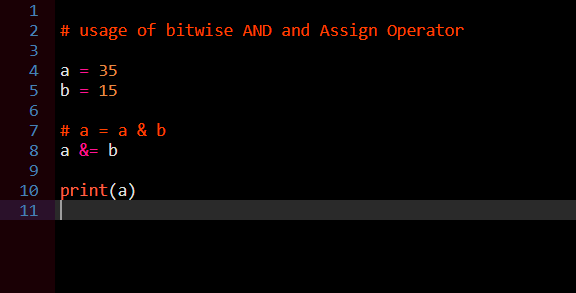
Output:
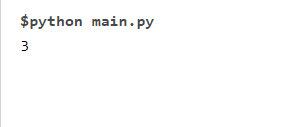
Bitwise OR and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the Bitwise OR.
Syntax:
x |= y
Example: Showing the implementation of bitwise OR and assignment operator
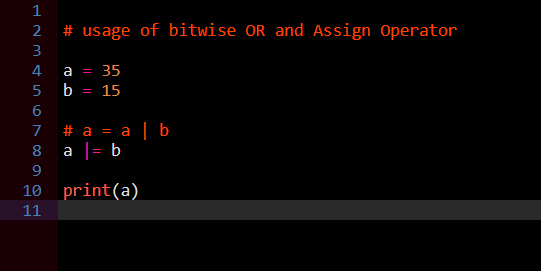
Output:
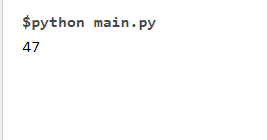
Bitwise XOR and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the Bitwise XOR.
Syntax:
x ^= y
Example: Showing the implementation of bitwise XOR and assign operator
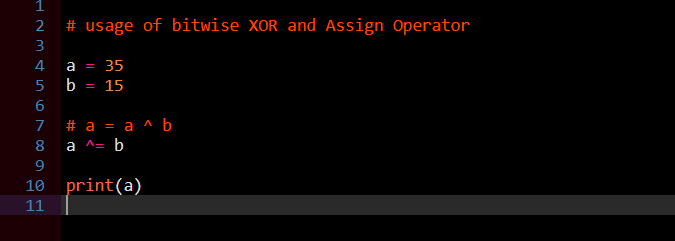
Output:
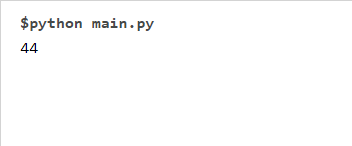
Bitwise Right Shift and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the Bitwise Right Shift.
Syntax:
x >>= y
Example: Showing the implementation of bitwise right shift and assign operator
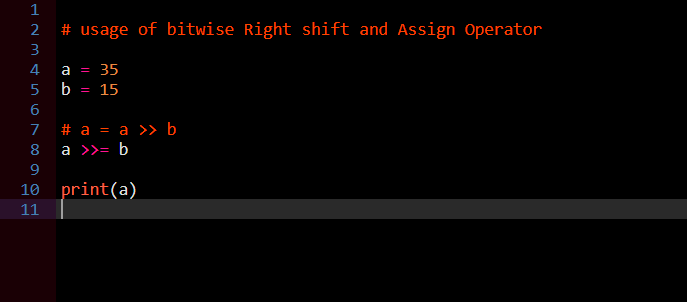
Output:
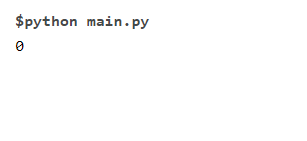
Bitwise Left Shift and Assignment:
The utility of this operator is to assign the result to the operand present on the left side of the operator after calculating the Bitwise Left shift.
Syntax:
x <<= y
Example: Showing the implementation of bitwise left shift and assign operator
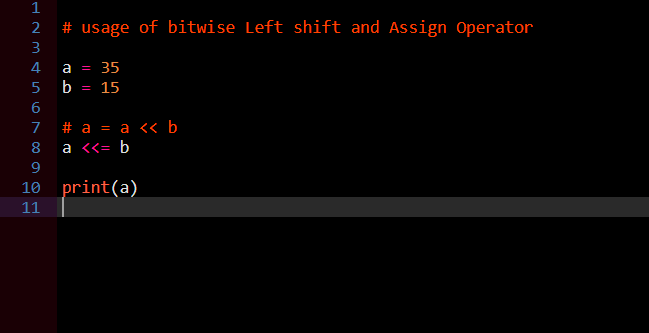
Output:
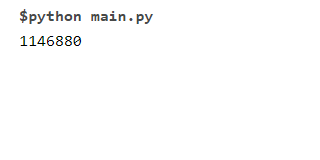