Python Indentation
Overview
The spaces at the start of a code line are known as an indentation in Python programming. Indentation is only used for readability in other programming languages like C, C++, etc., but in Python, it is a fundamental principle that must be obeyed while producing python code; otherwise, the Python interpreter will issue an Indentation Error.
You should have some familiarity with the following Python programming subjects in order to grasp this one:
- Syntax in python
- Python comments
- Python variable scope
Article's focus
- We will learn about Python indentation in this topic.
- With the help of some instance codes, we shall learn a few indentation principles.
- The benefits and drawbacks of indentation will next be covered.
What is Python's indentation?
The leading whitespace (spaces and tabs) before every statement in Python is known as indentation. The fact that indentation in Python serves purposes other than improving code readability explains why it is crucial.
Statements with an identical number of whitespaces before them and the same indentation level are treated by Python as a single code block. Therefore, a block of code in Python is a collection of statements with the same indentation level, or the same amounts of leading whitespaces, as opposed to languages like C, C++, etc. where a block of code is represented by curly braces {}.
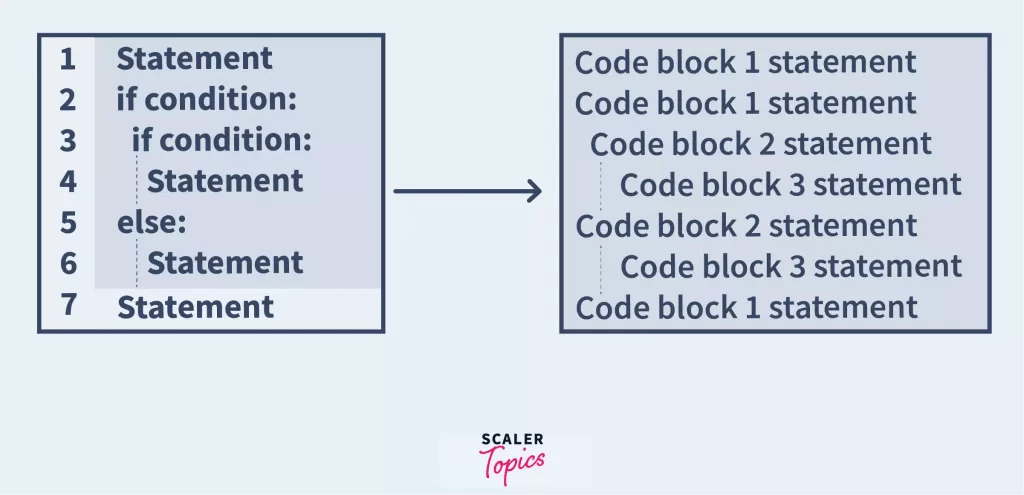
Observations that can be drawn from the above figure include the following:
- According to the figure above, statements on lines 1, 2, and 7 all belong to a single block that has the zero or lowest level of indentation because they are all on the same level of indentation and have the same number of whitespaces before them. Statements 3 and 5 form another block at the first level of indentation after being indented by one space. Statements 4 and 6 are similarly indented two spaces, forming a block at the second level of indentation.
- Statements 3 and 5 are indented one step below the line 2 statement, which is an if statement; as a result, they form a single block. Since line 2 contains an if statement, the block that is indented below the if serves as the statement's body. As a result, lines 2, 3, 4, and 6 that are indented below line 2 are all included in the body of the if statement.
- Now that we are aware that the statements on lines 3, 4, and 6 make up the body of the if statement on line 2, we may go on. Let's examine the indentation in terms of them. As a single block (block2 from the interpretation), which contains the statements at 3 and 5, they are uniformly indented and will be performed one at a time.
- As is well knowledge, the statement on line 4 constitutes the body of the if statement on line 3, and similarly, the statement on line 6 constitutes the body of the else statement on line 5.
- Indentation aids in defining blocks and indicating to which statements each block belongs in this way.
- If the condition is tested and returns true, control then moves within the body of the if statement, bringing statements 3, 4, and 5 into play. The execution begins on line 1 and is followed by the statement at line 2.
- Currently, line 3's statement is being executed. If the condition is being evaluated and returns true, line 4 is then being executed, and control then moves to line 7. If the condition at line 3 returns false, control moves to a different statement at line 5, after which line 6 and the statement at line 7 are executed.
- Currently, line 3's statement is being executed. If the condition is being evaluated and returns true, line 4 is then being executed, and control then moves to line 7. If the condition at line 3 returns false, control moves to a different statement at line 5, after which line 6 and the statement at line 7 are executed.
- The control skips lines 3, 4, 5, and 6 and moves to the statement at line 7 if the condition at line number 2 returns false.
Examples
Example 1: The Python code snippet below shows how to properly indent code.
name = 'Amit'
if name == 'Amit':
print('Hello Amit')
print(‘How are you?’)
print('Welcome to our world!')
else:
print('Who are you?')
print('What are you doing?')
print('Have a nice day!')
Output:
Hello Amit
How are you?
Welcome to our world!
Have a nice day!
Explanation
- Amit is given the name variable in the first statement.
- Now that the condition if name == "Amit": has been evaluated and has returned true, the indented next two words below the if the condition is executed. The three sentences that are executed inside the body are print("Hello Amit"), print("How are you?"), and print("Welcome to our world!").
- The otherwise clause is skipped after the statement is executed and control is passed to the next expression, print("Have a nice day!"), which is also executed.
- The statements in this program's if and else bodies are indented.
Example 2: An instance of properly indented code can be found below.
j = 1
while(j <= 7):
print("the value is " + str(j))
j = j + 1
Output:
The value is 1
The value is 2
The value is 3
The value is 4
The value is 5
The value is 6
The value is 7
Explanation
- In the first statement, variable j is initialized to 1.
- The body of the whole is now run because the condition while (j = 7) is true. All of the lines that are indented under the while loop make up the while's body.
- As a result, the commands print (“The value is” + str(j)) and j = j + 1 are executed.
- Up until the while condition returns false, this operation is repeated.
- Here, the while statement's body and the statements print ("The value is" + str(j)) and j = j + 1 are both uniformly indented to form a block.
Python Code Indentation Techniques
Let's use an easy instance to demonstrate how to indent Python code
For instance: Determine whether a given integer is even or odd, and output neither even nor odd if the value is zero.
Let's go over the strategy step-by-step:
- Assigning the input number to an integer variable comes first.
- To determine whether the input integer is 0, create an if-else block.
- Write another if-else (inner if-else) block within the if block to examine the condition number % 2 == 0 if it is not zero.(For a given number, this is the even check.)
- Print that the number is even if the condition number % 2 == 0 is true.
- The number in the else block print is odd if the condition number % 2 == 0 is false.
- If the value is zero, the statement that the given number would be neither odd nor even is printed inside the outer otherwise block.
Program
num = 50
if(num != 0):
if(num % 2 == 0):
print("A number given is Even. ")
else:
print("A number given is Odd.")
else:
print("The given number is neither Odd nor Even.")
Output:
A number given is Even.
Explanation
- Numbers 1, 3, and 8 are part of a single block at the 1st level of the indentation line.
- Line 1 is the first line to be executed, and line 3 comes next.
- The if condition is tested at line 3, and when the expression num!= 0 yields True, control is transferred to the block of statements under the if at line 3, which is indented.
- At the if block
- Since lines 4 and 6 are indented on the same level (the 2nd indentation level), they form a single block.
- Line 4's if condition is examined, and since 50% 2 == 0 it returns. True, the if statement at line 4 takes control. Control shifts to line 5 as a result and the print command is executed.
- Control late moves to the statement below line 9 after skipping the else blocks within the inner if-else and the outside if-else.
How are Avoid Python Indentation Mistakes
- If you omit the indentation, Python will generate an indentation error. For instance, the code following would raise an IndentationError: anticipated a block with indents wrong:
Incorrect Indentation (Error):
if( 1 == 1):
print("It is test code")
Correct Indentation:
if( 1 == 1):
print("It is test code")
- For the same block of code that is indented, there ought to be an equal amount of whitespaces. Python will otherwise raise the IndentationError: Unexpected Indent error.
Incorrect Indentation (Error):
if( 1 == 1):
print("It is test code")
print("It is test code_1")
Correct Indentation:
if( 1 == 1):
print("It is test code")
print("It is test code_1")
- On the first line of code, indentation is not permitted. IndentationError: Unexpected Indent will be thrown by Python.
Incorrect Indentation (Error):
if( 1 == 1):
print("It is test code")
print("It is test code_1")
Correct Indentation:
if( 1 == 1):
print("It is test code")
print("It is test code_1")
- A statement that is attached to an indented expression should be present; for example, the if statement is attached to the block of statements that are all indented below. This holds true for Python's for, while, classes, functions, etc. This point is illustrated in the example below.
Incorrect Indentation(Error):
if( 1 == 1):
print("It is test code")
print("It is test code_1")
Due to the last print statement's indentation not matching any other indentation, the aforementioned program will produce the error "IndentationError: unindent doesn't match any external indentation level" (no attaching external statement). This will cause an error in the software.
Correct Indentation:
if( 1 == 1):
print("It is test code")
print("It is test code_1")
Here, the indentation of the final print expression matches that of the print statement immediately following the if block. As a result, the if statement is the external attaching statement in this case.
Rules for Python Indentation
- Python's standard for indentation spaces is four. The user has complete discretion over the number of gaps, though. But to indent a statement, you need at least one space.
- Python code cannot contain an indentation on the first line.
- Python requires indentation to define blocks of statements.
- In a block of code, the space’s number must be constant.
- In Python, whitespaces are chosen over tabs for indentation. Additionally, use only whitespace or tabs to indent; combining tabs and whitespaces can result in incorrect indentation.
Advantages of Python Indentation
- Code indentation improves readability, while indentation in Python is mostly used to denote block structures.
- Python can cut the number of lines of code and avoid missing {and} errors and other problems that can occasionally appear in C and C++.
Drawback of Indentation in Python
- Code must be gently indented with the appropriate amount of whitespace, making sure to retain the uniformity of the whitespaces inside a single block. Python code sometimes has a large number of lines, making it laborious if the indentation is accidentally distorted.
- Writing Python code, especially many lines of code, maybe a laborious effort without the assistance of decent editors or Ide that assist with the indentation because we must type the indentation for each line.
Conclusion
The article's conclusion is now at hand. Knowing how the indentation works is the first level before you can begin creating Python code. Python indentation is a fundamental notion for any new Python programmer.