Python Parser
Introduction :
Parsing is referred to as the processing and translation of a Python program into machine language. In general, we could indeed say that the command parse is used to separate the given program code into a manageable chunk of code for the purpose of analyzing the proper syntax. The Python programming language comes with a built-in module called parse that acts as an interface between the internal parser and compiler. Using this module, a python program can edit small pieces of code and produce an executable program from the parse tree of the modified python code. A different module in Python called argparse is used to parse command-line options.
How Python Parse Works:
In this article, parsing, the process of converting data into the required format, is primarily accomplished using the Python parser. Due to the fact that data obtained from various applications may have different data formats that aren't appropriate for the particular application, parsing is required in these circumstances. As a result, parsing is generally understood to be the conversion of data from one format to another. Lexer and a parser are the two components of a parser, though occasionally, only parsers are used.
Python can parse data in a number of ways, including using the parser module, regular expressions, string methods like split() and strip(), pandas, and reading CSV files into text using read.csv. There is also the idea of argument parsing, which refers to the use of the Python module argparse to parse data with one or even more arguments from the command line or terminal. Other modules, such as the getopt, sys, and argparse modules, are available when working with argument parsings. Let's now look at a Python parser demonstration. The creation of parsers in Python is also possible with the aid of a few tools, like parser generators and the parser combinators library.
Several Python parser examples:
Let's examine the example below to see how the parser module is employed to parse the provided expressions.
Sample code 1 :
import parser
print("Python program to demonstrate the parser module")
print("\n")
exp = "3 + 6"
print("The parsing expression that is provided is as follows:")
print(exp)
print("\n")
print("The result of parsing of the given expression is:")
st = parser.expr(exp)
print(st)
print("\n")
print("Conversion from the parsed object to the code object")
code = st.compile()
print(code)
print("\n")
print("The evaluated result for the given expression is as follows:")
result = eval(code)
print(result)
Output:
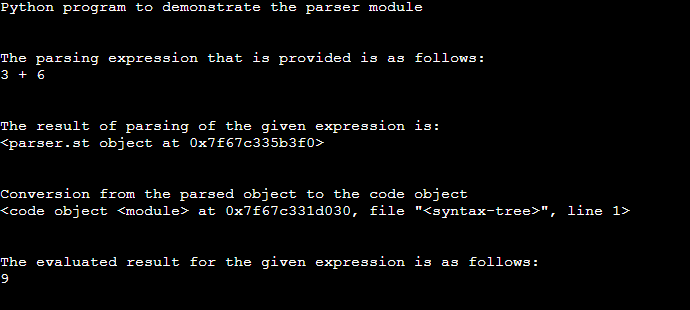
In the above-mentioned program, we must first import the parser module before declaring an expression that needs to be calculated and parsed using the parser.expr() function. The given expression can then be evaluated using the eval() function.
In Python, we occasionally receive data that is in date-time format and is either in text or CSV format. Python, therefore, offers the parse dates() function to parse such formats into appropriate date-time formats. Imagine that we have a CSV file with data in it, but the time details are divided by commas, making it difficult to read. In this case, we would use the parse_dates() function, but first, we would need to import pandas because this function is a part of pandas.
Using the argparse module in Python, which is very user-friendly for the command-line interface, we could also parse command-line options and arguments. Suppose we want to run Unix commands through a Python command-line interface. For example, the ls command lists all the directories on the current drive and requires a variety of arguments. To build such a command-line interface, we use Python's argparse module.
Therefore, in order to create a command-line interface in Python, we must first import the argparse module. Next, using the ArgumentParser() function provided by the argparse module, we create an object for holding arguments. Finally, we can add arguments to the ArgumentParser() object we just created and execute any commands via the Python command line. The help command is the only one that can be run for free, so keep that in mind. In order to create a command line interface using an argparse module, here is a small piece of Python code.
import argparse
After using ArgumentParser() to create an object, we can now use the parser.parse_args() function to parse the arguments.
parser = argparse.ArgumentParser()
parser.parse_args()
We can use add_argument() to add the arguments and pass the argument to this function, for example, parser.add_argument(" ls "). So, let's look at a brief example below.
Sample code 2:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("ls")
args = parser.parse_args()
print(args.ls)
Output:
Since we cannot use any other commands in the programme above, the output can be seen in the screenshot below. However, if we have an argparse module, we can run the following commands in the Python shell.

$ python ex_argparse.py --help
usage: ex_argparse.py [-h] echo
Positional Arguments:
echo
Optional Arguments:
-h, --help show this help message and exit
$ python ex_argparse.py Educba
Educa
Conclusion:
In this article, we draw the conclusion that Python has a concept for parsing. We learned how straightforward the parsing process is. In general, parsing is the process of cutting up a lengthy string of one type of format into smaller pieces so that it can be converted to a different format when it is needed. Python offers a variety of methods for doing this, including split() and strip() for strings and pandas for converting CSV files to text format. We learned that by using the Python argparse module, we could also use a parser module as a command-line interface for running commands. In the previous section, we learned how to use argparse and how to use the Python terminal to execute commands.