How To Compare Two Strings In Python
In this article, we will discuss how to compare two strings in Python.
So, before that, let’s have a quick revision on “What are strings?”
Strings are a sequence of characters that are denoted using inverted commas ''. They are immutable which means they cannot be changed once declared. The distinction between the two strings can be understood using id().
Examples of a string are-
a=’Bill Gates’
x=’Moscow’
In the first example, we will see how we can compare two strings in Python using relational operators.
We have used the following relational operators in our program-
- == - This relational operator is used to compare whether the given two values are equal or not. For example, if we provide the value 2 to variables a and b and then check whether they are equal or not using ‘==’, it will display the result as True, in case of dissimilar values, the result is False.
- >,< - These relational operators are used to denote the greater or the smaller value. For example, a>b means a is greater than b, and a<b means a is less than b. On providing values a=3 and b=4 when these operators are applied then, a>b will display the result as False whereas a<b will display the result as True.
- != - This relational operator stands for ‘not equal to’. For example, if the values of a and b are 2 and if we apply a!=b, it will display the result as ‘False’ because both the values are equal whereas if a and b hold different values then it will give the result as True.
Following program illustrates how the user has provided two strings and then they are compared using relational operators.
#using relational operator
a="Tutorials"
b="tutorials"
print(a==b)
print(a>b)
print(a<b)
print(a!=b)
INPUT-
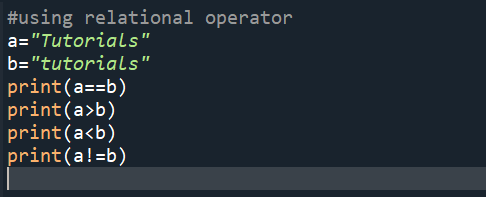
OUTPUT-
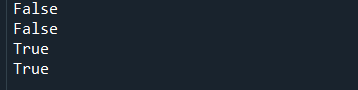
In the output, we can observe that the expected results are displayed after the comparison.
The second example is based on the same idea but here instead of two we have provided four strings.
The four strings are – “Python”, “interesting”, “programming”, “Language”. In the program given below, we have compared str1 with str2 and str3 and str4.
You can try and compare str1 with the other strings as well.
Let us see what happens when we execute the given program-
#using relational operators
str1="Python"
str2="interesting"
str3="programming"
str4="language"
print(str1==str2)
print(str3==str4)
print(str1>str2)
print(str1<str2)
print(str3>str4)
print(str3<str4)
INPUT-
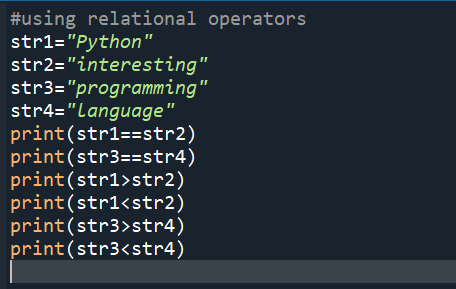
OUTPUT-
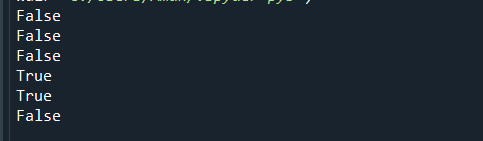
In the output, we can observe the expected results are displayed when str1 is compared with str2 and str3 is compared with str4.
In the next method of comparing strings, we will see how the two keywords 'is' and 'is not in Python help us to proceed on our objective.
In the given program, we have assigned the string values to objects a and b and then compared them using these keywords.
The next thing that we did in the program was assign the value of a to c and then printed their addresses and after that, we compared all the three objects a,b and c.
The following program illustrates the same.
Let’s see what happens when we execute it!
#using is and is not operator
a="Tutorials"
b="tutorials"
print(a is b)
print(a is not b)
c=a
print(id(a))
print(id(c))
print(a is c)
print(b is c)
print(a is not c)
print(b is not c)
INPUT-
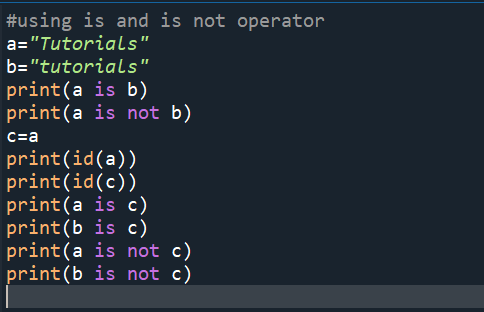
OUTPUT-
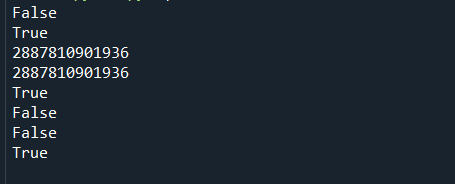
In the output, we can observe the following things-
- The result is displayed based on the comparison of and b.
- The addresses come out to be the same because in Python similar values point to a common address.
- Then the results based on the comparison of a,b and c are displayed.
The second example is based on the same method but here we have taken three strings str1, str2, and str3 respectively.
We have assigned the string values to the objects str1, str2 and str3 and then compared them using the keywords ‘is’ and ‘is not’.
The next thing that we did in the program was assign the value of str2 to str4 and then printed their addresses and after that, we compared all the four objects str1,str2,str3, and str4.
The following program illustrates the same.
Let’s see what happens when we execute it!
#using relational operators str1="Python" str2="interesting" str3="programming" print(str1 is str2) print(str1 is not str2) print(str1 is str3) print(str2 is str3) str4=str2 print(id(str2)) print(id(str4)) print(str2 is str4) print(str1 is str4) print(str1 is not str4) print(str2 is not str4)
INPUT-
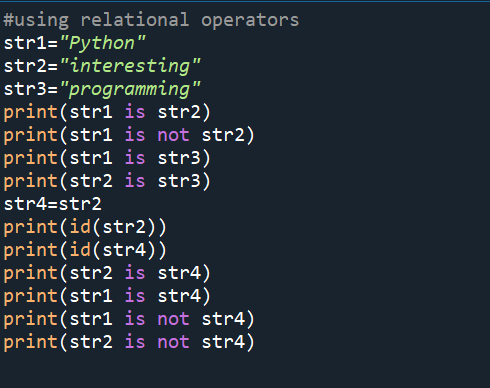
OUTPUT-
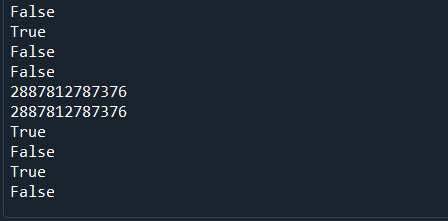
In the output, we can observe the following things-
- The result is displayed based on the comparison of str1,str2, and str3.
- The addresses come out to be the same because in Python similar values point to a common address.
- Then the results based on the comparison of str1,str2,str3, and str4 are displayed.
In our last example of this article, we have defined a function that takes the length of the string as its range, checks whether the index of each element lies within it and if the condition is fulfilled, the declared counters c1 and c2 are incremented.
Once the function is defined, we call the function by passing strings as the parameters.
Following program illustrates the same.
#defining function def str_comp(str1,str2): c1=0 c2=0 for i in range(len(str1)): if str1[i]>="0" and str1[i]<="9": c1+=1 for i in range(len(str2)): if str2[i]>="0" and str2[i]<="9": c2+=1 return c1==c2 print(str_comp("Tutorials","tutorial")) print(str_comp("Examples","example09")) print(str_comp("Python","python90"))
INPUT-
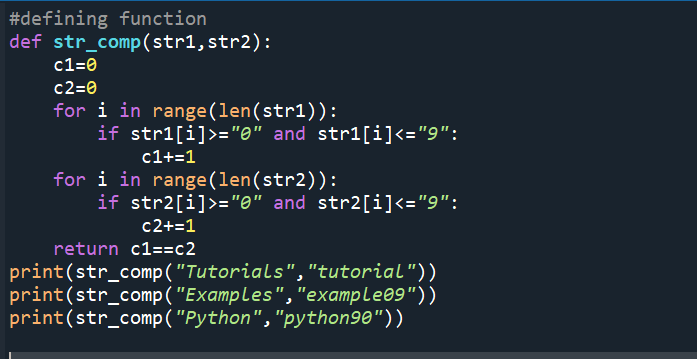
OUTPUT-
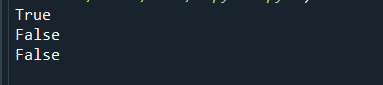
Since the function returns whether the strings are equal or not, in the output we can see the expected results.
So, in this article, we discussed various methods of comparing strings in Python.