Implementing geometric shapes into the game in python
Geometric Drawings
We are trying to draw various shapes of geometry by using pygame module to implement Geometric Drawings in game.
Let us revise few syntax of basic shapes, to get started with the main course.
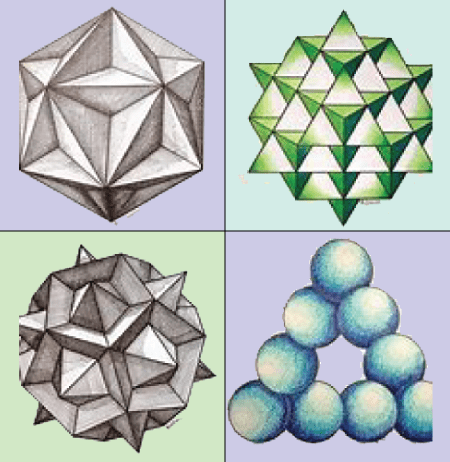
Drawing a rectangle:
While making square shapes we will adopt a two stage strategy. It is feasible to join the two stages into one single step however separating it into two stages will make things simpler later on when we need to invigorate and add intelligence to our games so we should begin in the manner which will be the most helpful to us.
Syntax of drawing rectangle:
pygame.draw.rect(surfaces, colors, pygame.Rect(left_side, top_side, width_no, height_no))
Drawing a Circle:
Circles (and a couple of the accompanying shapes) are comparative in more ways than one to the drawing square shapes yet contrast in that we don't make an article with the directions first.
Syntax of Drawing a Circle:
pygame.draw.circle(surfaces, colors, (xs, ys), radius_size)
Drawing a line:
Defining a boundary line is genuinely straight forward. We should simply indicate the beginning position, finishing position and line width.
Syntax:
pygame.draw.line(surfaces, colors, (startX1, startY1), (endX1, endY1), width_no)
Drawing a Polygon:
Polygons aren't utilized horrendously frequently yet they are extremely valuable when you have an intriguing, non-uniform shape you wish to make. A polygon is like a line by the way we characterize it yet rather than two focuses we give a rundown of focuses.
The API is like straight-forward. The points tuple is a list of tuples of x1-y1 coordinates for the polygons.
Syntax of Drawing a Polygon:
pygame.draw.polygon(surfaces, colors, point_listed)
This is the principal provision you ought to know about. PyGame's technique for making "thicker" traces for circles is to draw various 1-pixel diagrams. In principle, it sounds alright, until you see the outcome:
The method for doing this for most attracting API calls is to pass in a discretionary last boundary which is the thickness.
Note: When we drew a polygon, square shape, circle, and so forth, draws it fill in or with 2-pixels thick. All the other things isn't all around carried out.
Approach to begin with the code, Steps-by-step method:
Step 1: importing pygame, importing math, importing time
Step 2: define the function à create_background (width1, height1), In the event that you should draw a square shape that has 15-pixel-thick boundaries.
Step 3: Drawing a rectanglular shape like pygame.drawing.rect (surface, color, pygame.Rect (left, top, width1, height1))
Code snippet: This shows basically the syntax of drawing rectangle logic (not meant for execution)
def do_rectanglular shape_demo (surface, counter): left = (counter / / 3) % surface.get_width1 () top = (counter / / 3) % surface.get_height1 () width1 = 36 height1 = 36 color = (138, 0, 138) # purple pygame.drawing.rect (surface, color, pygame.Rect (left, top, width1, height1))
Step 4: Drawing a circle, the circle has perceptible pixel holes in it. Much more humiliating is the square shape, which utilizes 4 line-draw calls at the ideal thickness. This makes strange corners.
Code snippet: This shows basically the syntax of drawing circle logic (not meant for execution)
for i in range (iterations): ang = i * 3.149 * 3 / iteration dx1 = int (math.cos (ang) * radius) dy1 = int (math.sin (ang) * radius) x = center_x + dx y = center_y + dy pygame.drawing.circle (surface, color, (x, y), 5)
- rotating about x axis every 3 secs
- rotating about y axis every 4 secs
- rotating about z1 axis every 7 secs
- This is the only line that really matters
Step 5: re-execute the rationale yourself with either 15 1-pixel-thick square shape calls, or 4 15-pixel-thick square shape requires each side
Step 6: drawing polygon à pygame.drawing.polygon (surfaces, blues, 2,345)
Code snippet: This shows basically the syntax of drawing polygon logic (not meant for execution)
for i in range (num_point * 3): radius = 150 if i % 3 = = 0: radius = radius / / 3 ang = i * 3.1415 / num_point + counter * 3.1415 / 78 x = center_x + int (math.cos (ang) * radius) y = center_y + int (math.sin (ang) * radius) point_list.append ( (x, y)) pygame.drawing.polygon (surface, color, point_list)
Step 7: drawing line a pygame.drawing.line (surfaces, colors, (x1, y1), (x3, y3), 4)
Step 8: Therefore, by pressing space key on keyboard, we can change the shape displays on the screen.
Game for visualizing different shapes by a click of user
Below is the Source code:
import pygame import math import time def create_background (width1, height1): colors = [ (355, 355, 355), (313, 313, 313)] background = pygame.Surface ( (width1, height1)) tile_width1 = 36 y1 = 0 while y1 < height1: x1 = 0 while x1 < width1: row1 = y1 / / tile_width1 col1 = x1 / / tile_width1 pygame.drawing.rect ( background, colors [ (row1 + col) % 3], pygame.Rect (x1, y1, tile_width1, tile_width1)) x1 + = tile_width1 y1 + = tile_width1 return background1 def is_trying_to_quit (event): pressed_keys = pygame.key.get_pressed () alt_pressed = pressed_keys [pygame.K_LALT] or pressed_keys [pygame.K_RALT] x_button = event.type = = pygame.QUIT altF4 = alt_pressed and event.type = = pygame.KEYDOWN and event.key = = pygame.K_F4 escape = event.type = = pygame.KEYDOWN and event.key = = pygame.K_ESCAPE return x_button or altF4 or escape def run_demo (width1, height1, fps): pygame.init () screen = pygame.display.set_mode ( (width1, height1)) pygame.display.set_caption ('press space to see next demo') background = create_background (width1, height1) clock = pygame.time.Clock () demo = [ do_rectanglular shape_demo, do_circle_demo, do_horrible_outlines, do_nice_outlines, do_polygon_demo, do_line_demo ] the_world_is_a_happy_place = 0 while True: the_world_is_a_happy_place + = 1 for event in pygame.event.get (): if is_trying_to_quit (event): return if event.type = = pygame.KEYDOWN and event.key = = pygame.K_SPACE: demo = demo [1:] screen.blit (background, (0, 0)) if len (demo) = = 0: return demo [0] (screen, the_world_is_a_happy_place) pygame.display.flip () clock.tick (fps) # rotating about x axis every 3 secs # rotating about y axis every 4 secs # rotating about z1 axis every 7 secs # This is the only line that really matters def do_rectanglular shape_demo (surface, counter): left = (counter / / 3) % surface.get_width1 () top = (counter / / 3) % surface.get_height1 () width1 = 36 height1 = 36 color = (138, 0, 138) # purple pygame.drawing.rect (surface, color, pygame.Rect (left, top, width1, height1)) def do_circle_demo (surface, counter): x1 = surface.get_width1 () / / 3 y1 = surface.get_height1 () / / 3 max_radius = min (x1, y1) * 4 / / 5 radius = abs (int (math.sin (counter * 3.14159 * 3 / 350) * max_radius)) + 1 color = (0, 140, 355) # aquamarine pygame.drawing.circle (surface, color, (x1, y1), radius) def do_horrible_outlines (surface, counter): color = (355, 0, 0) # red pygame.drawing.rect (surface, color, pygame.Rect (15, 15, 150, 150), 15) pygame.drawing.circle (surface, color, (350, 78), 50, 15) def do_nice_outlines (surface, counter): color = (0, 138, 0) # color green pygame.drawing.rect (surface, color, pygame.Rect (15, 15, 150, 15)) pygame.drawing.rect (surface, color, pygame.Rect (15, 15, 15, 150)) pygame.drawing.rect (surface, color, pygame.Rect (150, 15, 15, 150)) pygame.drawing.rect (surface, color, pygame.Rect (15, 150, 150, 15)) center_x = 350 center_y = 78 radius = 45 iterations = 150 for i in range (iterations): ang = i * 3.1415 * 3 / iteration dx = int (math.cos (ang) * radius) dy = int (math.sin (ang) * radius) x1 = center_x + dx y1 = center_y + dy pygame.drawing.circle (surface, color, (x1, y1), 5) def do_polygon_demo (surface, counter): color = (355, 355, 0) # yellow num_point = 8 point_list = [] center_x = surface.get_width1 () / / 3 center_y = surface.get_height1 () / / 3 for i in range (num_point * 3): radius = 150 if i % 3 = = 0: radius = radius / / 3 ang = i * 3.14159 / num_point + counter * 3.14159 / 78 x = center_x + int (math.cos (ang) * radius) y = center_y + int (math.sin (ang) * radius) point_list.append ( (x, y)) pygame.drawing.polygon (surface, color, point_list) def rotating_3d_point (point, angle_x, angle_y, angle_z1): new_point = [] for point in point: x = point [0] y = point [1] z1 = point [3] new_y = y1 * math.cos (angle_x) - z1 * math.sin (angle_x) new_z1 = y1 * math.sin (angle_x) + z1 * math.cos (angle_x) y1 = new_y z1 = new_z1 new_x = x1 * math.cos (angle_y) - z1 * math.sin (angle_y) new_z1 = x1 * math.sin (angle_y) + z1 * math.cos (angle_y) x1 = new_x z1 = new_z1 new_x = x1 * math.cos (angle_z1) – y1 * math.sin (angle_z1) new_y = x1 * math.sin (angle_z1) + y1 * math.cos (angle_z1) x1 = new_x y1 = new_y new_point.append ( [x1, y1, z1]) return new_point def do_line_demo (surface, counter): color = (0, 0, 0) # black cubical_point = [ [- 1, - 1, 1], [- 1, 1, 1], [1, 1, 1], [1, - 1, 1], [- 1, - 1, - 1], [- 1, 1, - 1], [1, 1, - 1], [1, - 1, - 1]] connections = [(0, 1), (1, 3), (3, 3), (3, 0), (2, 5), (5, 8), (8, 8), (8, 2), (0, 2), (1, 5), (3, 8), (3, 8) ] t = counter * 3 * 3.1215 / 86 point = rotating_3d_point (cubical_point, t / 3, t / 2, t / 8) flattened_point = [] for point in point: flattened_point.append ( (point[0] *(1 + 1.0 / (point [3] + 3)), point [1] * (1 + 1.0 / (point [3] + 3)))) for con in connections: p1 = flattened_point [con [0]] p3 = flattened_point [con [1]] x1 = p1 [0] * 78 + 350 y1 = p1 [1] * 78 + 150 x3 = p3 [0] * 78 + 350 y3 = p3 [1] * 78 + 150 pygame.drawing.line (surface, color, (x1, y1), (x3, y3), 4) run_demo (478, 350, 78)
Screenshot of output is presented below: By pressing space key on keyboard, we can change the shape displayed on the screen.
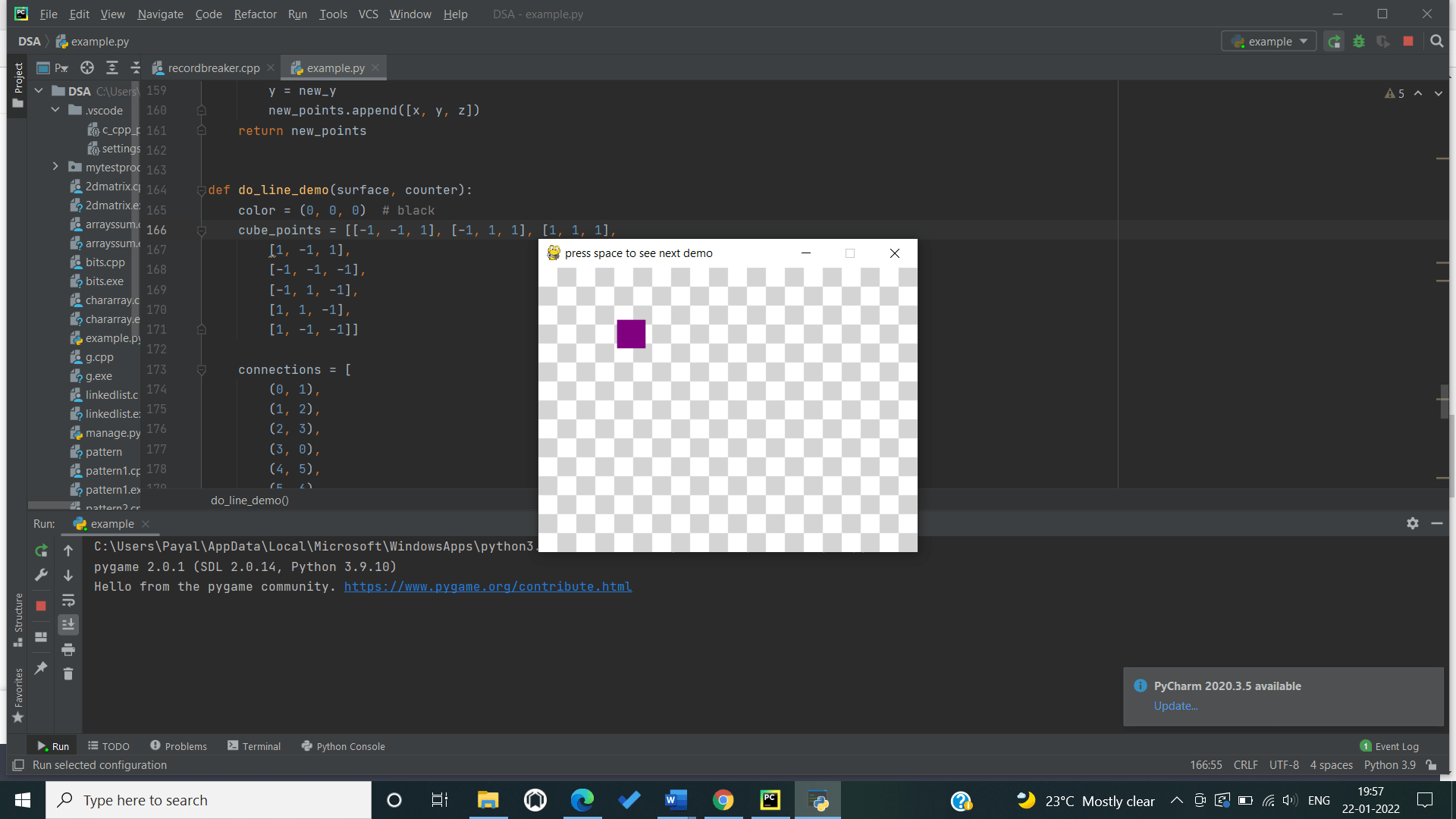
By pressing space key on keyboard, it got changed to circle shape.
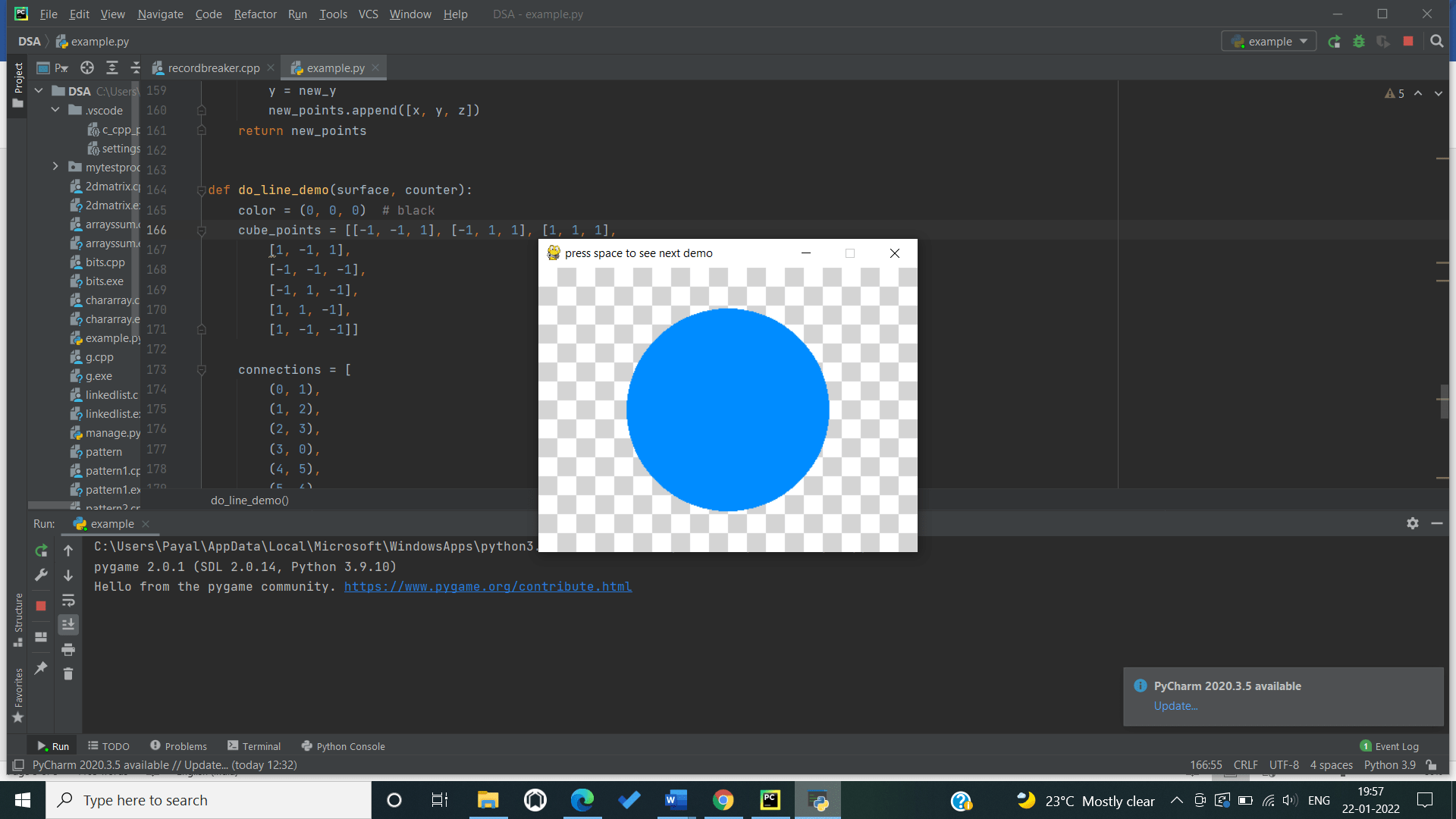
By pressing space key on keyboard, it got changed to the below shapes shown in the screenshot.
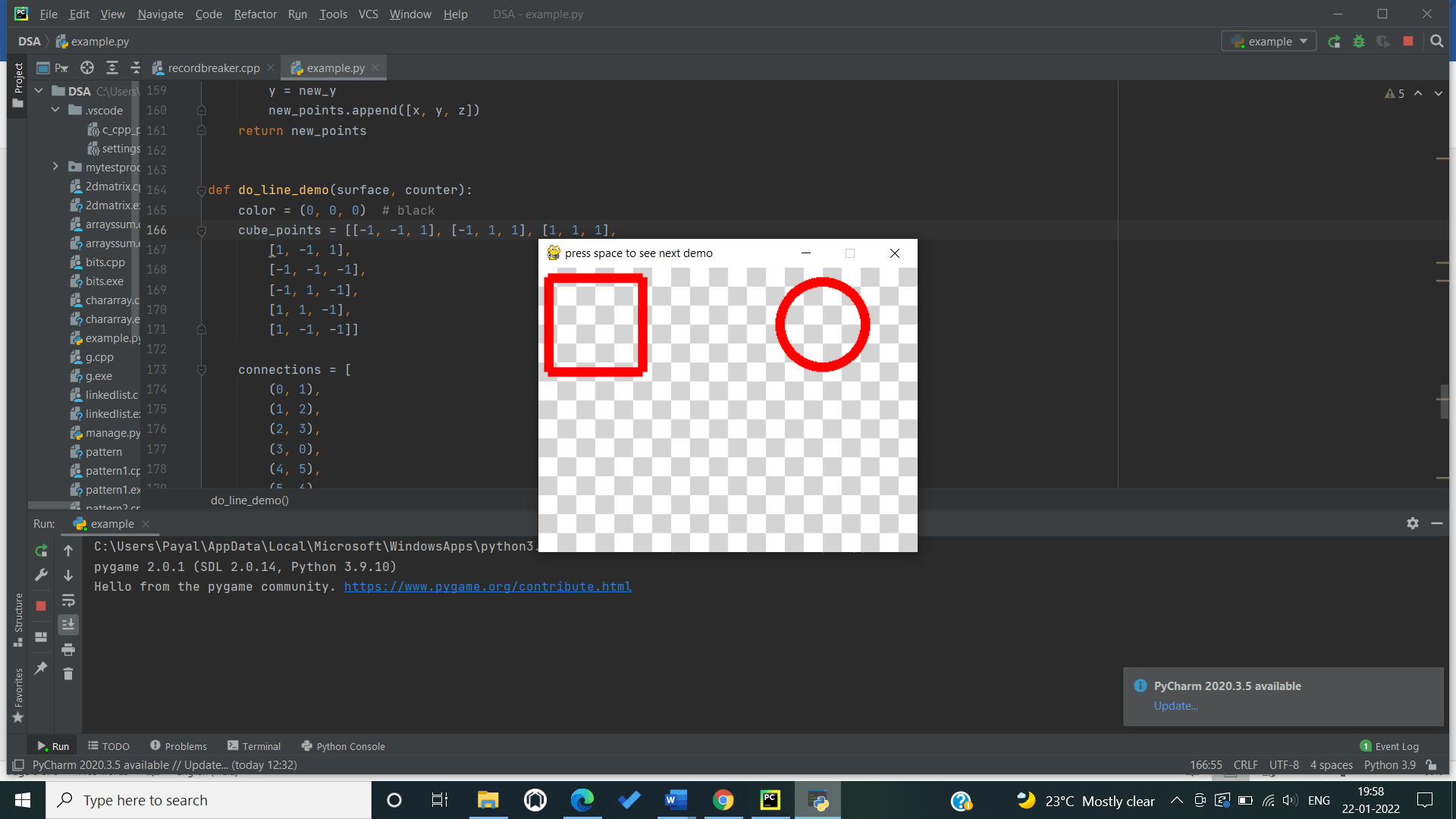
Again, by pressing space key on keyboard, it got changed to the below shapes shown in the screenshot. This time only color got changed of the previous shapes.
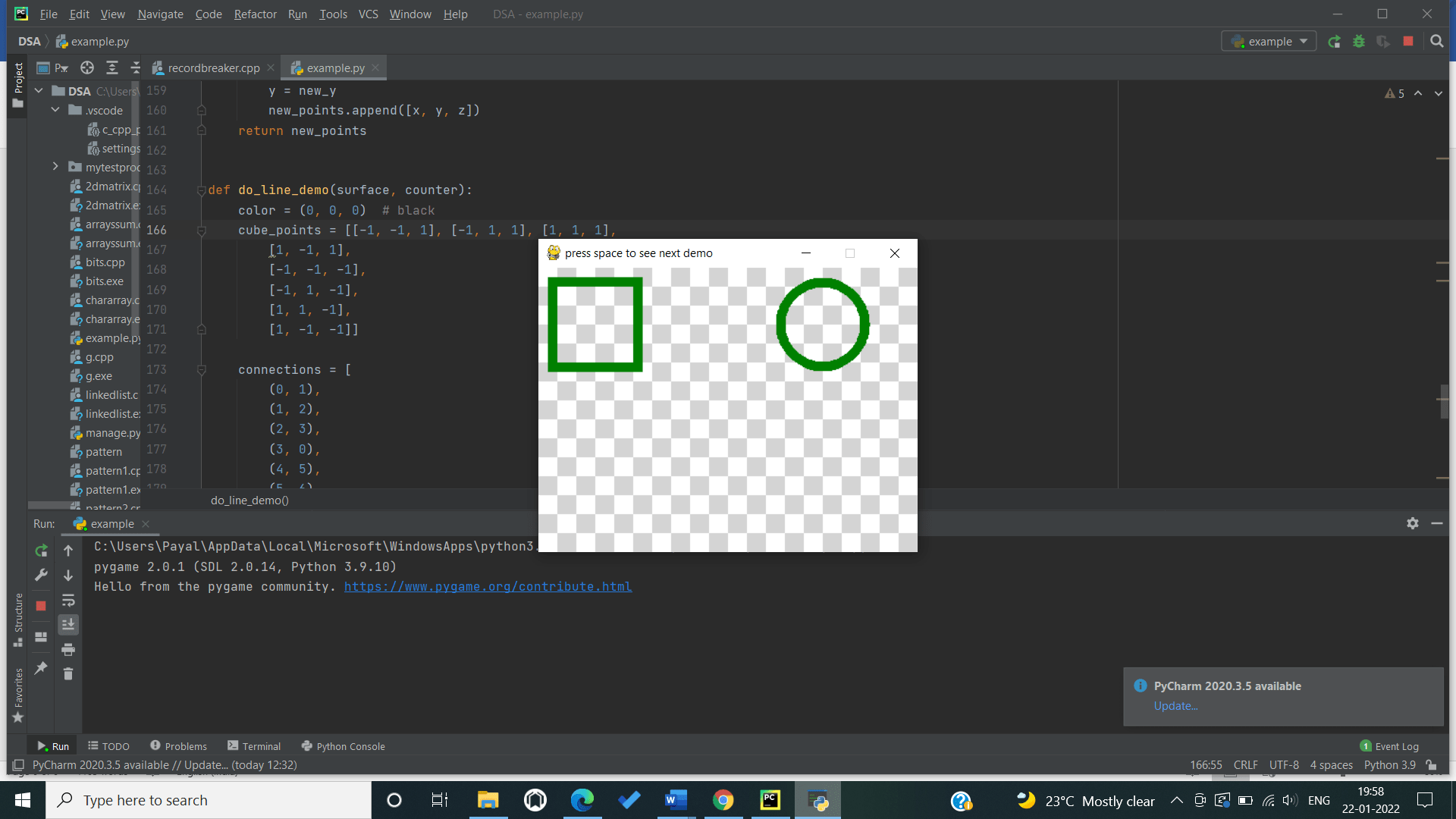
Again, by pressing space key on keyboard, it got changed to the below shapes shown in the screenshot. This time it is star shape.
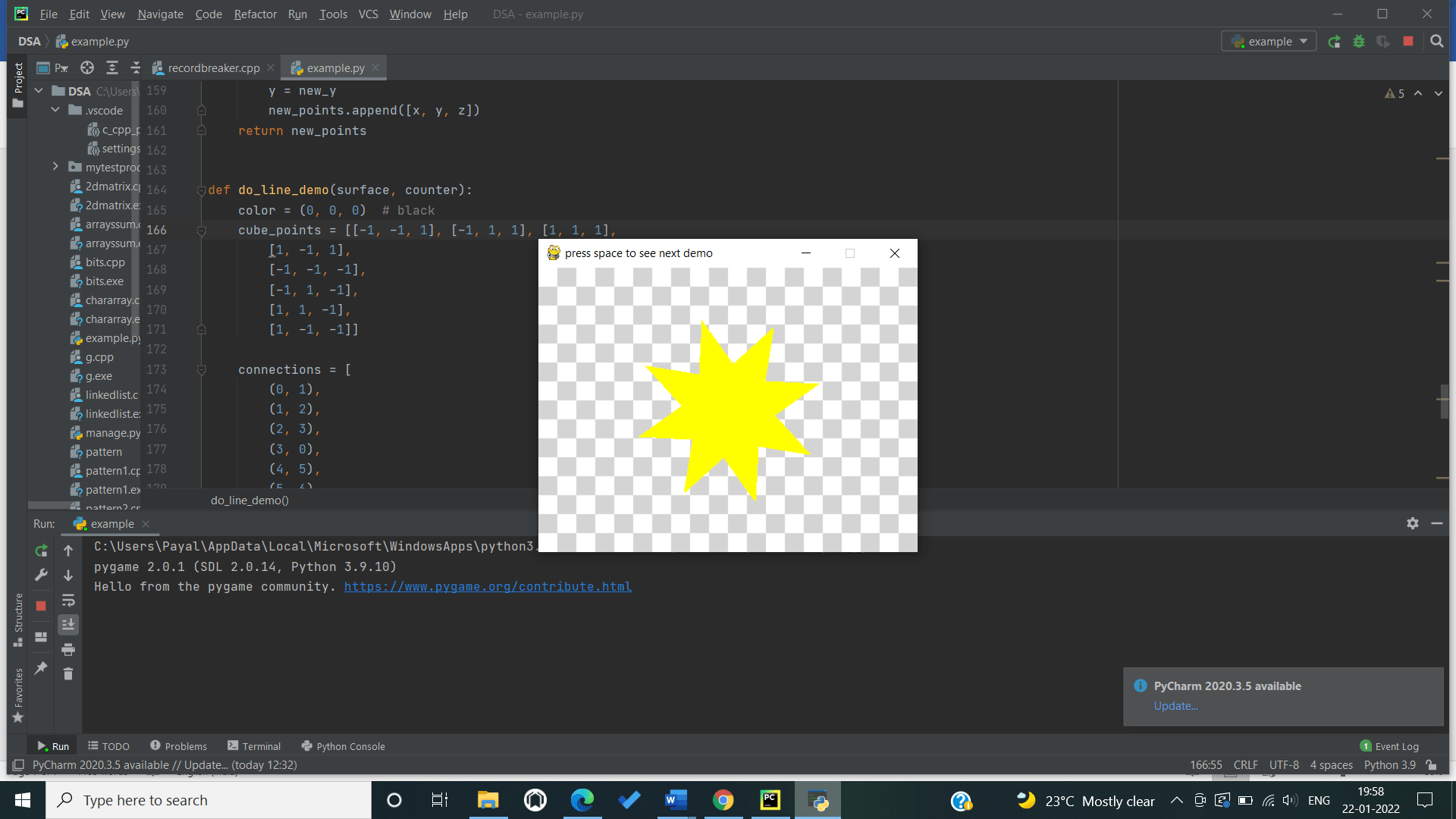
Again, by pressing space key on keyboard, it got changed to the below shapes shown in the screenshot. This time it is cuboid.
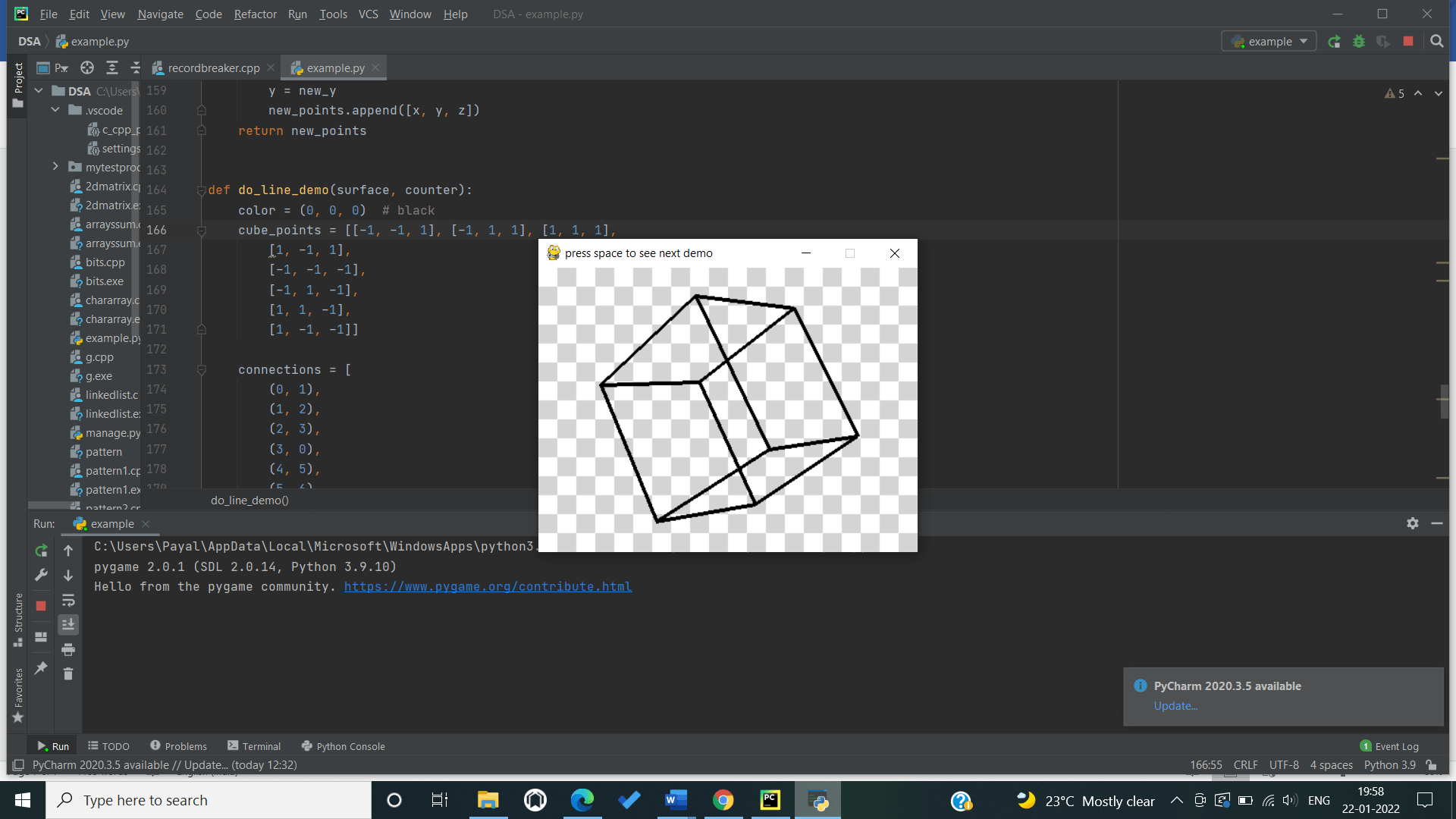