Python Syntax Error Invalid Syntax
Python is renowned for its simple and direct syntax. However, we might come across some things that Python doesn't allow if we are learning Python for the very first time or if we already have a strong foundation in another programming language. This guide can assist us if we have ever encountered a SyntaxError while trying to execute Python code. We will encounter typical instances of Python's incorrect syntax throughout this article and discover how to fix the problem.
Invalid Syntax in Python:
Our Python code will be segmented by the interpreter before being translated into Python byte code and executed when we run it. During this initial phase of programme execution, also referred to as the parsing phase, the Python interpreter will detect any improper syntax. Our Python code may have contained instances of invalid syntax if the interpreter is unable to parse it successfully. The interpreter will make an effort to demonstrate where the error occurred.
It can be annoying to receive a Syntax error when we are first learning Python. Python will make an effort to assist us in locating the offending syntax in our code. However, the traceback it offers might be a little unclear. Sometimes the code it refers to is absolutely perfect.
If our code is syntactically stable, we might encounter other exceptions that are not Syntax errors. In Python, invalid syntax cannot be handled like other exceptions. Even if we attempted to enclose code with improper syntax in a try and except block, the interpreter would still throw a SyntaxError.
SyntaxError Exception and Traceback:
Incorrect syntax in Python code will cause the interpreter to raise a SyntaxError exception and produce a traceback with some useful information to aid in error diagnosis. Here is some Python code that uses the improper syntax:
Sample code:
ages = {'ram': 25,'ravi': 25'rahul': 30}
print(f'rahul is {ages["rahul"]} years old.')
Output:

The dictionary literal on line 1 contains an error in syntax that can be seen. ravi, the second entry, lacks a comma. The following traceback will appear if we have attempted to execute this code as it is.
Keep in mind that in line 1 is where the error is found in the traceback message. The Python interpreter is making an effort to identify the problematic syntax. It is only able to pinpoint the location of a problem, though. We should start working our way backwards through the code once we receive a SyntaxError traceback, and the script that the traceback is pointing to appears to be alright.
In the above-mentioned example, depending on what comes after the comma, it is acceptable to omit the comma. For instance, line 1's missing comma after "rahul" is not problematic. The interpreter, however, can only direct us to the first thing it cannot understand once it comes across something that doesn't make any sense.
We can locate the incorrect syntax in our code by looking at a few components of a SyntaxError traceback:
- The name of the file where the improper syntax was found.
- The code line(line number) and replicated line where the problem was found.
- The spot in the code that has a problem is indicated by a caret (^) on the line below the replicated code.
- The error message that follows the exception of type SyntaxError may contain details that will help us identify the issue.
In the above-mentioned example, "main.py" was the given file name, line 1 was indicated, and the caret (^) was placed on the final quotation of the dictionary key rahul. Even if the SyntaxError traceback doesn't identify the true issue, it will identify the first instance where the interpreter failed to understand the syntax.
There are two additional exceptions that Python may raise. Although they go by different names, these are similar to SyntaxError:
- IndentationError
- TabError
Both of these exceptions derive from the Syntax error class, but they present unique indentation-related situations. When our code's indentation levels don't line up, an Indentation error is generated. When our code uses both spaces and tabs in the same file, a TabError is thrown.
Usual Syntax Issues:
Knowing the cause of the SyntaxError and how to correct the Python code's improper syntax are helpful when we encounter one for the first time. We can find a few of the most frequent causes of SyntaxErrors as well as solutions in the sections that follow.
Using the assignment operator (=) incorrectly
In a number of circumstances, Python prevents us from assigning values to objects. Assigning to function calls and literals are a couple of examples. We can see a few examples that try to do this in the code block below, along with the SyntaxError tracebacks that result:
len('abhinav') = 7
Output:
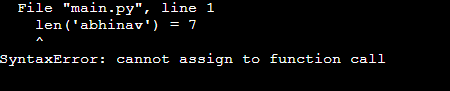
'hi' = 1
1 = 'hi'
Output:
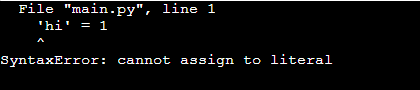
The first illustration tries to give the len() call the value 7. In this situation, the SyntaxError message is very beneficial. It informs us that a function call cannot have a value assigned to it.
To allocate a string and an integer to literals, see the second and third examples. For other literal values, the same rule applies. Once more, the traceback messages show that the issue arises when we try to give a literal value.
print(len('abhinav') == 7)

Most of the time, we might want to double-check to see if the statement shouldn't be a Boolean expression instead when Python informs us that we are trying to assign something that cannot be assigned to. This problem might also arise if we try to give a Python keyword a value, which we will learn how to do in the following section.
Missing, misspelt, or misused Python Keywords:
Python keywords are a group of protected words with unique meanings. We cannot use these words in our code as variables, identifiers, or function names. They are an integral part of the language and may only be applied in situations where Python permits it.
There are three typical mistakes people make when using keywords:
- Using an incorrect keyword
- Lack of a keyword
- Misuse of a keyword
A SyntaxError is returned if a keyword in our Python code is misspelt. Here's what happens, for instance, if we spell the keyword incorrectly:
fro i in range(11):
Output:
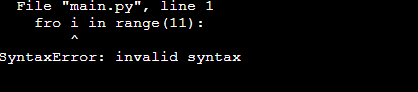
SyntaxError: Invalid Syntax is the message, but that is not very helpful. The traceback identifies the initial point at which Python could identify a problem. Make sure that all of our Python keywords are spelt correctly to resolve this kind of error. When we completely ignore keywords, this is another problem with keywords.
for i range(10):
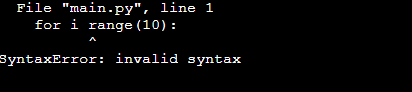
Once more, the exception message is not particularly helpful, but the traceback does make an effort to direct us in the right direction. The in keyword is lacking from the for loop syntax, as we can see if we step back from the caret.
Additionally, we can abuse a protected Python keyword. Keep in mind that there are only a few circumstances in which keywords are appropriate. Our Python code will have invalid syntax if we use them improperly. The use of continue or break outside of a loop is a typical illustration of this. When we are implementing things and accidentally move logic outside of a loop, this can happen very easily during development:
names = ['ram', 'ravi', 'rahul']
if 'ravi' in names:
print('ravi is found')
break
Output:
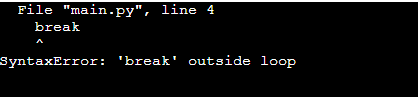
names = ['ram', 'ravi', 'rahul']
if 'ravi' in names:
print('ravi is found')
continue
Output:

pass = True
Output:
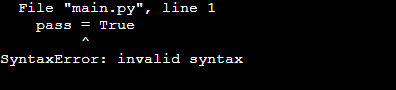
def pass():
Output:
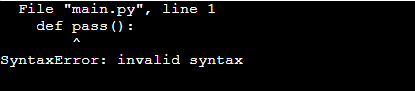
We will encounter a SyntaxError and the "invalid syntax" message once more if we attempt to give a value to pass or define a new function with the name pass.
Because the Python code appears to be valid on the surface, it might be a little more difficult to fix. Consider checking the variable or function name we want to use against the keyword list for the Python version we are using if our code appears to be correct, but we are still getting a SyntaxError.
With each new release of Python, the list of protected words has evolved. For instance, we could use the word await as a variable or function name in Python 3.6, but as of Python 3.7, it has been added to the list of keywords. Now, if our code is for Python 3.7 or later, attempting to use await as a variable or function name will result in a SyntaxError.
Python 2's print is a keyword, so we can't give it a value. However, it has a built-in function in Python 3 that accepts values. Whatever the version of Python we are using, we can execute the following code to view the list of keywords:
import keyword
print(keyword.kwlist)
Output:

Missing quotation marks, brackets, and parentheses:
In Python code, a missing or misplaced closing parenthesis, quote, or bracket is frequently the root of invalid syntax. These can be challenging to find in blocks of longer than one line that contain multiple lines of nested parentheses. Python's tracebacks can be used to identify mismatched or missing quotes:
word = 'don't'
Output:
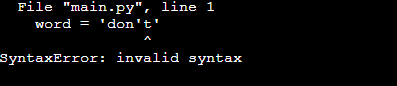
The traceback in this case, indicates the invalid code, which has following a having closed single quote. We have two options for resolving this:
- Put a backslash (\) to escape the single quote ('don\'t').
- Instead, enclose the entire string in double quotes (i.e. "don't")
- Forgetting to close a string is another frequent error. Both single-quoted and double-quoted strings have the same circumstance and traceback:
statement = "This is an unclosed string
Output:

This time, the traceback's caret directly points at the error code. A little more detailed and useful in identifying the issue is the SyntaxError message, "EOL while scanning string literal". This indicates that an open string wasn't closed before the Python interpreter reached the end of a line (EOL). End the string with a quote that corresponds to the one we used to start it in order to correct this. This would be a double quote in this instance (").
Python syntax errors can also result from statements within an f-string lacking quotes:
#officestaff ages
ages = {
'ram': 24,
'ravi': 24,
'rahul': 43
}
print(f'rahul is {ages["rahul]} years old.')
Output:

The closing double quote from the key reference is absent from this instance's reference to an ages dictionary inside the printed f-string. The traceback that results is as follows:
Python recognises the issue and informs us that it can be found inside the f-string. The error "unterminated string" also identifies the issue. In this instance, the caret only designates the start of the f-string.
This may not be as useful as when the caret indicates the problematic portion of the f-string, but it does help us focus our search. Somewhere inside of that f-string is an unterminated string. All of us need to do is figure out where. Make sure that the all internal f-string quotes and brackets are present in order to solve this issue.
The situation for missing parentheses and brackets is largely the same. Python will recognise and flag an error if, for instance, the closing square bracket [ ] is omitted from a list. However, there are a few varieties of this. The first would be to omit the final bracket from the list:
# missing.py
def foo():
return [1, 2, 3
print(list())
Output:

We will be informed that there is a problem with the call to print() when we run this code:
In this case, Python interprets the list as having the three elements 1, 2, and 3 print(list()). Because there isn't a comma or bracket separating 3 from print(list()), Python groups them together as the third element of the list. Python uses whitespace to group things logically.
Another option is to omit the closing square bracket and add a trailing comma after the final item in the list:
# missing.py
def foo():
return [1, 2, 3,
print(list())
Output:

In contrast to the previous example, where 3 and print(list()) were combined as one element, here, a comma is used to separate the two. The fourth element of the list is now the call to print(list()), and Python ends the file without the closing bracket. Python reached the End Of the File (EOF), but it was intending something else, according to the traceback.
Python was looking for a closing bracket (]) in this example, but the recurring line and caret aren't particularly helpful. Python finds missing brackets and parentheses with difficulty. Sometimes the only option is to start at the caret and work our way back until we can figure out what's missing or off.
Making a dictionary syntax error:
We already saw that leaving the comma off of a dictionary element could result in a SyntaxError. The equals sign (=), rather than the colon, is another example of improper syntax when using Python dictionaries:
age = {'ram'=25}
Output:
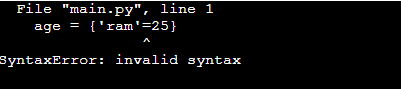
This error message is not helpful once more. However, the repeated line and caret are very useful. They are specifically mentioning the problematic character.
If we mistake Python syntax for that of other programming languages, problems of this nature are frequently encountered. We will also notice this if we mistake defining a dictionary for calling dict(). We could correct this by inserting a colon in place of the equals sign. We can also use dict() instead.
The incorrect use of indentation:
There are two Syntax Error subclasses that specifically address indentation problems:
- Indentation Error
- Indentation Error
Python uses whitespace instead of the curly braces that other programming languages use to indicate code blocks. This means that Python anticipates predictable behaviour from the whitespace in our code. If there is a line in a code segment that has the incorrect number of spaces, it will raise an Indentation Error:
# indentation error in Python
def foo():
for i in range(11):
print(i)
print('done')
Output:

It might be difficult to see, but in the line 5 is, only two spaces are indented. The for loop, which is four spaces over, should be in line with it. Fortunately, Python can quickly identify this as a problem and let us know what it is.
However, there is some ambiguity present as well. Is the print("done") line supposed to come before or after the for loop? The following error will appear when we run the code mentioned above:
The traceback appears very similar to the Syntax Error traceback, but it is actually an Indentation Error. Also very helpful is the error message. We are informed that the line's indentation level does not correspond to any other indentation level. In other words, even though print("done") is indented two spaces, Python is unable to locate any other lines of code that are indented to the same degree. By trying to make sure the code corresponds with the desired level of indentation, we can quickly fix this.
The second kind of Syntax Error is called Tab Error, and it appears whenever a line has either tabs or spaces for indentation while the rest of the file has the other. Prior to Python pointing it out to you, this might go unnoticed.
It might appear as though all the lines are at the same level if our tab size is the same width as that of the number of spaces in each indentation level. However, Python will flag this as a problem if one line is indented with spaces while the other is indented with tabs:
Instead of 4 spaces, line 5 is indented with a tab in this instance. Depending on our system settings, this code block may appear to be completely correct to us or appear to be completely incorrect.
Python, on the other hand, will catch the problem right away. It might be useful for us to see an example of how the code appears under various tab width settings before running the code and see what Python will report is incorrect:
tabs 4 # Sets the width of the shell tab to four spaces.
1 # indentation.py
2 def foo():
3 for i in range(11)
4 print(i)
5 print('done')
6
7 foo()
tabs 8 # sets the width of the shell tab to 8 spaces (standard)
1 # indentation.py
2 def func():
3 for i in range(11)
4 print(i)
5 print('done')
6
7 foo()
tabs 3 # sets the width of the shell tab to 3 spaces.
1 # indentation.py
2 def foo():
3 for i in range(11)
4 print(i)
5 print('done')
6
Observe how the 3 examples above display differently from one another. The majority of the code uses four spaces for each level of indentation, but in all three examples, line 5 uses a single tab. Depending on the tab width setting, the width of tab changes:
- The print statement would then appear to be outside of the for loop if the width of the tab is 4. At the conclusion of the loop, the console will print "done."
- The print statement would then appear to be inside the for loop if the width of tab is 8, which is typical for many systems. 'd one' will be displayed on the console after each number.
- The print statement appears out of place if the width of the tab is 3. Line 5 in this instance does not correspond to any indentation level.
Instead of the typical SyntaxError, notice the TabError. Python provides us with a beneficial error message and highlights the problematic line. It makes it clear that the same file uses a combination of spaces and tabs for indentation.
Making sure that either spaces or tabs, but not both, are used on every line in a single Python code file will solve this problem. The correction for the code blocks above would be to eliminate the tab and substitute four spaces, which will print "done" when the for loop is complete.
Defining a function and Calling it:
In Python, when defining or calling functions, we might encounter invalid syntax. If we use a semicolon rather than a colon at the end of a function definition, for instance, we will see a Syntax Error:
def fun();
Output:
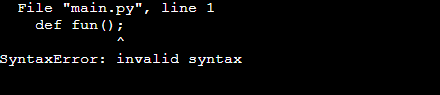
The caret pointing directly to the problematic character in the traceback is very helpful. By substituting a colon for the semicolon, we can fix this Python syntax error.
Additionally, keyword arguments must be used in the correct order in both function calls and function definitions. Positional arguments are always followed by keyword arguments. A SyntaxError will appear if this ordering is not followed:
fun(a=1, 2)
Output:

Once more, the error is very helpful in this situation because it clearly explains what is completely mistaken with the line.
Conclusion:
We have seen what details the Syntax Error traceback provides in this article. Additionally, we are familiar with numerous examples of typical Python syntax errors and their fixes. This will not only expedite our workflow but also improve our code review skills.
Try to use an IDE (Integrated Development Environment) that recognises Python syntax and offers feedback when we are writing code. Many of the examples of invalid Python code from this article should highlight the problematic lines before we even run our code if we paste them into a reliable integrated development environment (IDE).
It can be frustrating to receive a Syntax Error while learning Python, but at least we now know how to decode traceback messages as well as what kinds of Python syntax errors we might encounter. The following time we encounter a Syntax Error, we will be more prepared to address the issue quickly.