Find whether the given stringnumber is palindrome or not
Problem statement
Sam is found of playing with strings. One day he thought of finding whether a string is a palindrome or not. He wanted to develop a computer process to check whether the given string is palindrome or not. As a programmer, your task is to develop a code that takes a string input and checks whether it is palindrome or not.
Approach
If you know what a palindrome is, you can easily solve this question. A palindrome is a set of sequence of character, which looks similar from either side. It means that if you take a particular sequence and write it from left to right or from right to left, if both the written sequences are the same, then it is a palindrome.
You can solve this by reversing the given string and comparing it with the original string. If both the strings are the same, then you can return true or else false. For this, you need to declare an empty string and traverse the original string from the right and store each character into an empty string. After the completion of string traversal, you need to compare the original string with the new string and return the result.
Example 1.
import java.io.*;
import java.util.*;
public class Main
{
public static void main (String args [])
{
Scanner sc1 = new Scanner (System.in);
System.out.println("Enter the string to check whether it is palindrome or not:");
String palindromeString = sc1.next();
// input the string
String duplicateString = ""; // duplicate string
int n = palindromeString.length(); // length of the string
for (int i = n - 1; i >= 0; i--)
{
duplicateString = duplicateString + palindromeString.charAt (i);
// reversing the string
}
// comparing both string
if (palindromeString.equalsIgnoreCase (duplicateString)
{
System.out.println("The given string"+palindromeString+" is palindrome.");
}
else
{
System.out.println("The string "+palindromeString+"is not palindrome.");
}
}
}
Output
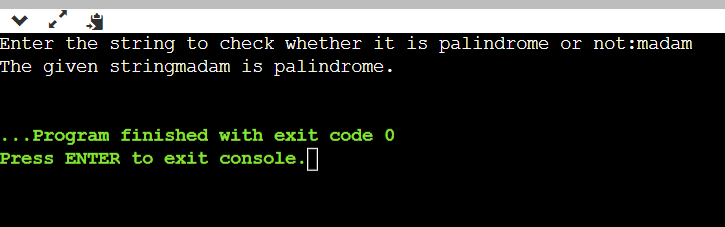
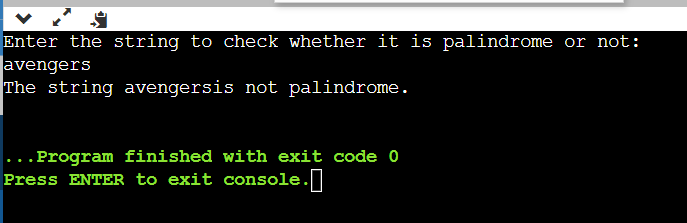
Example 2: If input is in the number form.
import java.io.*;
import java.util.*;
class Main {
public static void main (String [] args) {
Scanner sc1= new Scanner (System.in);
System.out.println("enter the number to check whether it is palindrome or not");
int Palindromenumber = sc1.nextInt(); // input the integer
int revNum = 0, remainder = 0;
// storing the input number to orgNum
int orgNum = Palindromenumber;
// getting thr reverse of orgNum
// storing it to revNum
while (Palindromenumber != 0) {
remainder = Palindromenumber % 10 ;
revNum = revNum * 10 + remainder ;
Palindromenumber /= 10 ;
}
// check if revNum and orgNumNum are equal
if (orgNum == revNum) {
System.out.println(orgNum + " is a Palindrome.");
}
else {
System.out.println(orgNum + " is not a Palindrome.");
}
}
}
Output
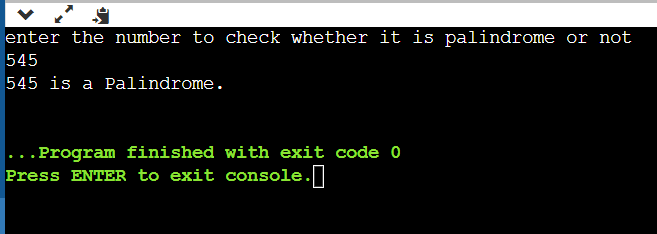
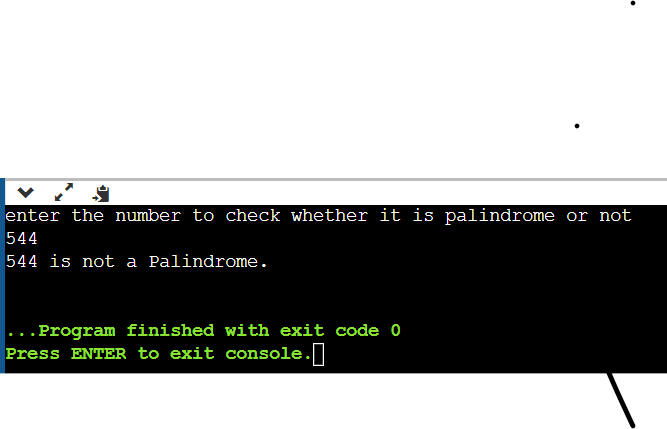