Standard GUI Unit Converter using PyQt5 in Python
GUI:
A graphical interface (GUI) is a user interface that lets users interact with electronic devices like computers and smartphones by using menus, icons, and other visual cues (graphics). In contrast to text-based interfaces, which only display data and commands as text, GUIs visualize information and related user controls. A cursor, trackball, stylus, or thumb on a touch screen are all pointing devices that can be used to communicate with GUI representations. The first text interface between a human and a computer operated using keyboard input and a prompt.
PyQt5:
PyQt5 is one of several solutions that Python offers for creating GUI applications. Cross-platform GUI toolkit PyQt5 is a collection of Python interfaces for Qt version 5. With this library's capabilities and convenience, one can quickly create an interactive computer program. Front-end and Back-end make up a GUI application. In order to speed up development & allow more time to be spent on back-end tasks, PyQt5 has developed a tool called "QtDesigner" that allows one to drag and drop elements to create the front end. Riverbank Computing is the company in charge of developing and maintaining PyQt.
Understanding the Unit Conversion:
The unit conversion process entails several steps involving addition, subtraction, or multiplication by a conversion factor. Additionally, rounding and choosing the appropriate number of substantial digits may be included in the procedure. Various conversion units are used to measure various properties.
Unit conversion is required when attempting to solve various mathematical puzzles. For instance, if a rectangle's length is given in feet, but its width is given in meters, we must convert the units to make them uniform before finding the rectangle's perimeter. As a result, we must understand the idea of unit conversion.
- Measuring Weight
- Measuring Capacity
- Measuring Length
- Measuring Temperature
Conversion of Units Measurements:
The different types of unit measurements are:
- Length
- Area
- Volume
- Weight
- Temperature
In the early stages of learning, some non-standard units are used to introduce kids to units and measurements without requiring them to read any scales. Since reading any scale is a tedious task in and of itself, the introduction of non-standard measures enables the child to concentrate on ideas like heavy, light, long, short, and more before progressing to the next stage of measuring using the standard units.
- So, we calculate the unit conversions in this code using PyQt5 with the imported libraries which are present.
CODE:
Final Code:
from PyQt5 import QtCore, QtGui, QtWidgets
class Ui_UnitConverter(object):
def setupUi(self, UnitConverter):
UnitConverter.setObjectName("UnitConverter")
UnitConverter.resize(728, 601)
self.centralwidget = QtWidgets.QWidget(UnitConverter)
self.centralwidget.setObjectName("centralwidget")
self.verticalLayout = QtWidgets.QVBoxLayout(self.centralwidget)
self.verticalLayout.setContentsMargins(10, 10, 10, 10)
self.verticalLayout.setSpacing(0)
self.verticalLayout.setObjectName("verticalLayout")
self.dropShadowFrame = QtWidgets.QFrame(self.centralwidget)
self.dropShadowFrame.setStyleSheet("QFrame{\n"
" background-color: rgb(44, 50, 86);\n"
" color: rgb(200, 200, 200);\n"
"}")
self.dropShadowFrame.setFrameShape(QtWidgets.QFrame.StyledPanel)
self.dropShadowFrame.setFrameShadow(QtWidgets.QFrame.Raised)
self.dropShadowFrame.setObjectName("dropShadowFrame")
self.label = QtWidgets.QLabel(self.dropShadowFrame)
self.label.setGeometry(QtCore.QRect(190, 50, 311, 41))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(18)
self.label.setFont(font)
self.label.setStyleSheet("color: rgb(79, 199, 255);")
self.label.setAlignment(QtCore.Qt.AlignCenter)
self.label.setObjectName("label")
self.lineEdit = QtWidgets.QLineEdit(self.dropShadowFrame)
self.lineEdit.setGeometry(QtCore.QRect(50, 200, 161, 31))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(7)
font.setBold(True)
font.setWeight(75)
self.lineEdit.setFont(font)
self.lineEdit.setStyleSheet("QLineEdit {\n"
"background-color: rgb(115, 199, 255);\n"
"border: none;\n"
"border-radius: 9px;\n"
"}")
self.lineEdit.setText("")
self.lineEdit.setObjectName("lineEdit")
self.lineEdit_2 = QtWidgets.QLineEdit(self.dropShadowFrame)
self.lineEdit_2.setGeometry(QtCore.QRect(360, 200, 161, 31))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(7)
font.setBold(True)
font.setWeight(75)
self.lineEdit_2.setFont(font)
self.lineEdit_2.setStyleSheet("QLineEdit {\n"
"background-color: rgb(115, 199, 255);\n"
"border: none;\n"
"border-radius: 9px;\n"
"}")
self.lineEdit_2.setText("")
self.lineEdit_2.setObjectName("lineEdit_2")
self.comboBox_1 = QtWidgets.QComboBox(self.dropShadowFrame)
self.comboBox_1.setGeometry(QtCore.QRect(210, 200, 121, 31))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setBold(True)
font.setWeight(75)
self.comboBox_1.setFont(font)
self.comboBox_1.setStyleSheet("QComboBox {\n"
"\n"
" background-color: rgb(44, 50, 86);\n"
" color: rgb(79, 199, 255);\n"
" border: none;\n"
" border-radius: 9px;\n"
" text -align: center;\n"
"}\n"
"")
self.comboBox_1.setObjectName("comboBox_1")
self.comboBox_1.addItem("Second[s]")
self.comboBox_1.addItem("Millisecond[ms]")
self.comboBox_1.addItem("Minute[m]")
self.comboBox_1.addItem("Hour[h]")
self.comboBox_1.addItem("Day[D]")
self.comboBox_1.addItem("Year[y]")
self.comboBox_2 = QtWidgets.QComboBox(self.dropShadowFrame)
self.comboBox_2.setGeometry(QtCore.QRect(520, 200, 131, 31))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setBold(True)
font.setWeight(75)
self.comboBox_2.setFont(font)
self.comboBox_2.setStyleSheet("QComboBox {\n"
"\n"
" background-color: rgb(44, 50, 86);\n"
" color: rgb(79, 199, 255);\n"
" border: none;\n"
" border-radius: 9px;\n"
" text -align: center;\n"
"}\n"
"")
self.comboBox_2.setObjectName("comboBox_2")
self.comboBox_2.addItem("Second[s]")
self.comboBox_2.addItem("Millisecond[ms]")
self.comboBox_2.addItem("Minute[m]")
self.comboBox_2.addItem("Hour[h]")
self.comboBox_2.addItem("Day[D]")
self.comboBox_2.addItem("Year[y]")
self.comboBox_3 = QtWidgets.QComboBox(self.dropShadowFrame)
self.comboBox_3.setGeometry(QtCore.QRect(260, 450, 191, 31))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(14)
font.setBold(True)
font.setWeight(75)
self.comboBox_3.setFont(font)
self.comboBox_3.setLayoutDirection(QtCore.Qt.LeftToRight)
self.comboBox_3.setStyleSheet("QComboBox {\n"
"\n"
" background-color: rgb(44, 50, 86);\n"
" color: rgb(79, 199, 255);\n"
" border: none;\n"
" border-radius: 9px;\n"
" text -align: center;\n"
"}\n"
"")
self.comboBox_3.setEditable(False)
self.comboBox_3.setObjectName("comboBox_3")
self.comboBox_3.addItem("TIME")
self.label_2 = QtWidgets.QLabel(self.dropShadowFrame)
self.label_2.setGeometry(QtCore.QRect(90, 170, 81, 21))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(18)
self.label_2.setFont(font)
self.label_2.setStyleSheet("color: rgb(247, 19, 255);")
self.label_2.setAlignment(QtCore.Qt.AlignCenter)
self.label_2.setObjectName("label_2")
self.label_3 = QtWidgets.QLabel(self.dropShadowFrame)
self.label_3.setGeometry(QtCore.QRect(390, 170, 81, 21))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(18)
self.label_3.setFont(font)
self.label_3.setStyleSheet("color: rgb(247, 19, 255);")
self.label_3.setAlignment(QtCore.Qt.AlignCenter)
self.label_3.setObjectName("label_3")
self.label_4 = QtWidgets.QLabel(self.dropShadowFrame)
self.label_4.setGeometry(QtCore.QRect(300, 390, 91, 41))
font = QtGui.QFont()
font.setFamily("Segoe UI")
font.setPointSize(18)
self.label_4.setFont(font)
self.label_4.setStyleSheet("color: rgb(247, 19, 255);")
self.label_4.setAlignment(QtCore.Qt.AlignCenter)
self.label_4.setObjectName("label_4")
self.verticalLayout.addWidget(self.dropShadowFrame)
UnitConverter.setCentralWidget(self.centralwidget)
self.retranslateUi(UnitConverter)
QtCore.QMetaObject.connectSlotsByName(UnitConverter)
def retranslateUi(self, UnitConverter):
_translate = QtCore.QCoreApplication.translate
UnitConverter.setWindowTitle(_translate("UnitConverter", "MainWindow"))
self.label.setText(_translate("UnitConverter",
"<html><head/><body><p>MY <span style=\" font-weight:600;\">UNIT CONVERTER</span></p></body></html>"))
self.label_2.setText(_translate("UnitConverter",
"<html><head/><body><p><span style=\" font-size:10pt; font-weight:600;\">FROM:</span></p></body></html>"))
self.label_3.setText(_translate("UnitConverter",
"<html><head/><body><p><span style=\" font-size:10pt; font-weight:600;\">TO:</span></p><p><br/></p></body></html>"))
self.label_4.setText(_translate("UnitConverter",
"<html><head/><body><p><span style=\" font-size:16pt; font-weight:600;\">TYPE:</span></p></body></html>"))
if __name__ == "__main__":
import sys
app = QtWidgets.QApplication(sys.argv)
UnitConverter = QtWidgets.QMainWindow()
ui = Ui_UnitConverter()
ui.setupUi(UnitConverter)
UnitConverter.show()
sys.exit(app.exec_())
CODE EXPLANATION:
Importing the modules which are to be required that, are Qtcore, Qtwidgets, and some more for the best extraction of the model, then introducing a function called unit converter with an object to create an instance with its dimensional size, central widget, vertical layout, shadow frames to get an attractive output. We add items to the content like seconds, milliseconds, minute, hour, day, and year, and adds widgets Combobox from self, drop shadow frame, and geometry with QtCore and QRect with some dimensions of 260,450,191,31 with a font style of Segoe UI. Setting layout direction from left to right with a background color, border, border radius, and text-aligned to the center. We use Html to and CSS to get the output clear and attractive with significant colors with the module.
OUTPUT:
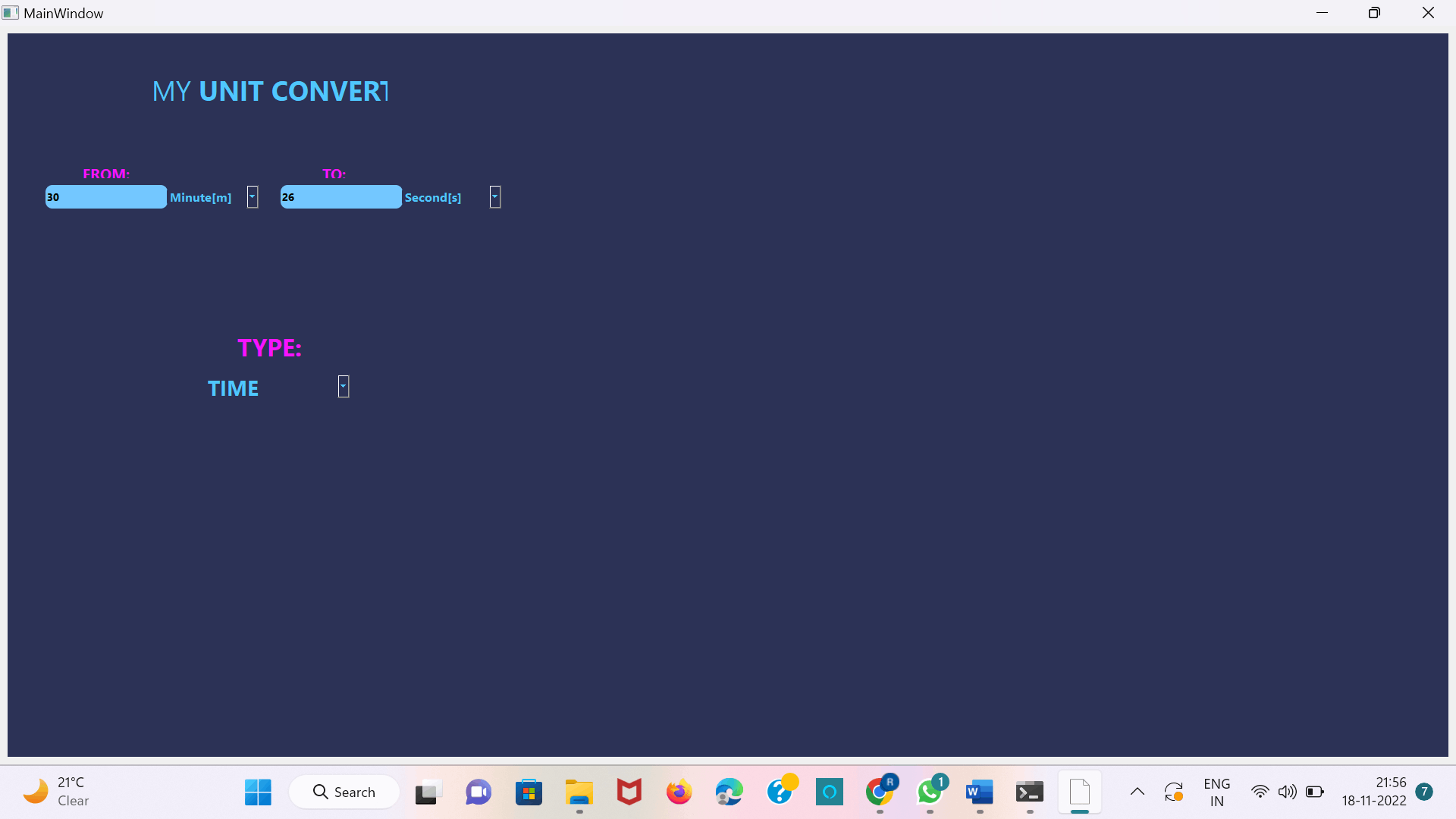