Classes & Objects in Python
Classes and Objects are used in most modern programming languages, mainly in Object-oriented Programming languages.
What is meant by Object Oriented Programming language?
Usage of objects and their components in order to create a software program is known as Object-oriented Programming language. More shortly, it is known as OOP.
(or)
The name itself specifies what it is actually. A language that is completely oriented with Objects and Components of objects is known as an Object-oriented programming language. Ex: Python, C++, Java.
As we have already discussed, Python is also such type of programming, i.e., Object-oriented programming. Classes and objects can be created fully, only in Object-Oriented Languages.
What is a class?
A class is a blueprint with a similar functionality where objects can be created or declared. A class is generally created using the keyword "class".
Syntax for creating a class:
class ClassName:
Example program for creating a class:
class Program:
n= 20
print(n)
Output:
10
What do a class contain?
A class consists of Objects, Attributes, Methods and Constructors.
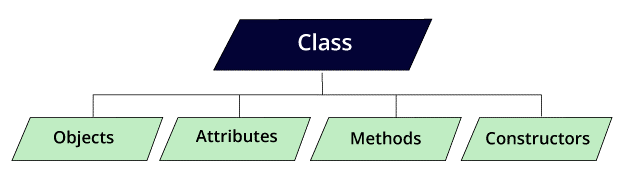
Why do we use classes?
The classes allow logical grouping of the data and function to make understanding and implementation easier. Here, data is nothing but Attributes. Functions are nothing but methods. A method is a function associated with its specific class.
Classes also allow reusability. Small changes can lead to different implementations and different outputs. This is the main use of class. The program can be changed easily for better implementations while using classes.
Let's discuss classes and their components with a real-time example.
Consider a class consisting of 60 students. Every student has their own identity. They have different names, different ages, different genders, belong to different regions, etc. There are many aspects that should be considered and makes every student unique. Every student might not be similar.
Some might function sharp, some might be low, some might be hyper active, etc. So, here class will be “Student”. Attributes are “name”, “age”, “gender”, “region”, etc. Methods are “sharp()”, “low()”, “hyperactive()”, etc. Objects will be “student1”, “student2”, “student3”, “student4”, ………. “student59”,” student60”.
What is an Object?
An instance of a class is known as an Object. An object is declared within the class to call any of the methods or access the attributes present in that particular class. Therefore, the creation of an object in a class is known as the Instantiation of the class.
Syntax for creating an Object:
#object is being created
obj1 = ClassName()
The one mentioned above is the basic structure/syntax of the creation of objects. This is insufficient for creating objects in a particular class. We are supposed to use the __init__() functionto create objects in a class.
__init__ () function:
It is a built-in function that should be used if we want to create or declare objects within the class. In other words, we can say that the __ init __() function helps in the initiation of the class. All the operations that are supposed to be done while or after initiating an object are done by the __ init __() function. The keyword “self” must be given as a parameter within the function parenthesis. The same object will be called when a method is called along with the parameters. That's the indication of self as a parameter.
Ex: When the object started calling the parameterized method, i.e.,
object.method( par1, par2),
then, the actual thing that happens is Class.method(object,par1, par2). The indication of the object within the parameters is “self”.
Syntax for __init__ () function:
class ClassName:
def __init__(self,parameter1,parameter2,parameter3,.........,parametern):
self.parameter1=parameter1
self.parameter2=parameter2
.......
self.parametern=parametern
obj1 = ClassName()
Example program using init() and self:
class Student:
def __init__(self,name,age,gender):
self.name=name
self.age=age
self.gender=gender
obj1=Student("Sathwik","20","Male")
print(obj1.gender)
print(obj1.name)
Output:
Male
Sathwik
Let's try a similar example without using self.
Example program using init() without self:
class Garage:
def __init__(obj1,company,colour):
obj1.company=company
obj1.colour=colour
obj1=Garage("Swift","Black")
print("The car "+obj1.colour+" "+obj1.company+" is out for delivery.")
print("You are pleased to collect it from the garage.")
Output:
The car Black Swift is out for delivery.
You are pleased to collect it from the garage.
Explanation:
As we all know that the "self" represents the object created within the class, we have used the same object instead of “self” in the second example. We got the output without any errors. Initially, the object is initiated using the init method inside the class, and the object is declared after assigning parameters. Printing the statements gave no errors, as-like the first example.
Example program which defines Creation of Class, Creation of Object, usage of init function and usage of self:
class Employee:
def __init__(self,name,gender,salary,age,place):
self.name=name
self.gender=gender
self.salary=salary
self.age=age
self.place=place
obj1=Employee("Sannah","She","50000","23","Hyderabad")
print(obj1.name+" is earning "+obj1.salary+" per month.")
print(obj1.name+" is "+obj1.age+" years old.")
print(obj1.gender+" is from "+obj1.place)
Output:
Sannah is earning 50000 per month.
Sannah is 23 years old.
She is from Hyderabad
Explanation:
Initially, a class named Employee is created in order to note the details of Employees working in that company. Init method is defined or initiated considering the parameters, i.e., name, gender, salary, age, place and (default parameter) self. All the parameters are converted into attributes, and then instantiation of object obj1 is implemented. The values have been given to the parameters within the object creation and then allowed to print the statements.
Operations that can be performed 1) Using objects and 2) On objects:
- Modify values of attributes:
If the initial value of an attribute is set to a value, then using objects, we can change or modify the value of that attribute for that particular instance. It can be done by using objects.
Syntax:
class Numbers:
def __init__(self,name):
self.name=name
number=2
obj1=Numbers("Hello")
obj1.number=6
print("My name is "+obj1.name)
print("The changed number is ")
print(obj1.number)
Output:
My name is Hello
The changed number is
6
Explanation:
Initially, the value of "number" is set to 2 inside the init method of the class Numbers. Outside the method, we changed the value of " number" using the object and set it to 6. After changing it, we have allowed it to be printed. From the output, we can say that the value of the attribute has been changed.
- Deletion of Objects:
The syntax is very simple. The objects can be deleted by using the keyword "del". This operation can be performed anywhere after the object has been initiated in the code.
Syntax:
del object_name
Example program to determine the deletion of an object:
class Places:
def __init__(self,name):
self.name=name
obj1=Places("Hyderabad")
del obj1
Output:
Traceback (most recent call last):
File "d:\downloads hdd\2-2\test.py", line 7, in <module>
print("I live in "+obj1.name)
NameError: name 'obj1' is not defined
Explanation:
Initially, the object is created under the class Places. A string value for the name parameter “Hyderabad” is passed through that object. But the object is deleted right after that and allowed to print. As the print statement is given after the object deletion, an error has been raised, i.e., NameError defining that the desired object is not found. Let us check out the output of the same program without object deletion.
class Places:
def __init__(self,name):
self.name=name
obj1=Places("Hyderabad")
#Changing the delete statement into comment and checking for the output
#del obj1
print("I live in "+obj1.name)
Output:
I live in Hyderabad
- Delete Attributes or Object properties:
Like the deletion of objects, the attributes can also be deleted anywhere in the program after declaration.
Syntax:
del object_name. attribute
Example program determining the deletion of Attributes using objects:
class Hi:
def __init__(self,name):
self.name=name
number=20
obj1=Hi("Nikki")
del obj1.number
print("The number present in the class Hi is ")
print(obj1.number)
Output:
Traceback (most recent call last):
File "d:\downloads hdd\2-2\test.py", line 6, in <module>
del obj1.number
AttributeError: number
Explanation:
Initially, the object is created under the class Hi. A string value for the name parameter “Nikki” is passed through that object. Along with the valued parameter, an attribute “number” is also declared before initiating the object. But the attribute is deleted concerning the object right after being initiated and allowed to print. As the print statement is given after the attribute deletion, an error has been raised, i.e., AttributeError, which defines that the desired attribute is not found. Let us check out the output of the same program without attribute deletion.
class Hi:
def __init__(self,name):
self.name=name
number=20
obj1=Hi("Nikki")
#changing the delete statement into comment and checking for the output
#del obj1.number
print("Hi, my name is "+obj1.name)
print("The number present in the class Hi is ")
print(obj1.number)
Output:
Hi, my name is Nikki
The number present in the class Hi is
20