Commands in Python
In this tutorial, we will see some of the widely used python commands along with their syntaxes and examples. To make Python more user-friendly, developers have provided these commands to ease using the python language.
Now, let us move ahead with our topic.
1. Print command
To print any output, calculated result, or the specified text on the screen or even on other output devices, this print command is used. It can be used to print objects of types including string, tuple, integer, etc.
Syntax – print(object)
Here, the object refers to the text or the value the programmer wants to display.
Example: The following code illustrates the use of a print statement. Here, 4 different objects, i.e., int, string, list, and tuple are being printed.
#Program to understand the usage of print statements
# integer is being printed
x=78
print (x)
# string is being printed
print ("Bonjour, World!")
# list is being printed
l=[110, 34, 567, 321]
print(l)
# tupule is being printed
t= (13, 67)
print(t)
Output:
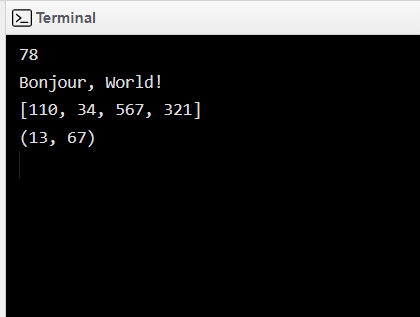
2. pip install command
It is a package manager command written in python. It is taken into account to install and manage the installed software packages. It can install any software packages from the python package index, a public repository available online.
Syntax – pip install package-name
Example: The following figure shows how to install any packages in python using the pip command. Here, pandas are being installed in Windows operating system.
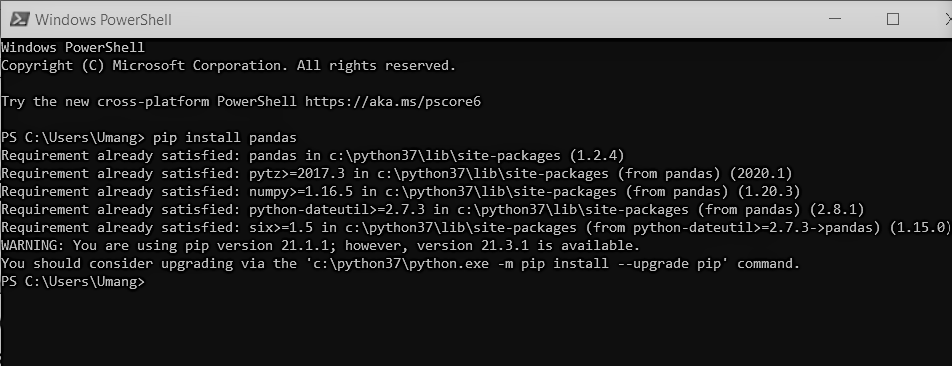
3. type command
To check the type or class of an object, the type command is taken into account.
Syntax: type(object)
Here, the object refers to the value whose type is to be checked.
Example: The following code illustrates the utility of type command. Here the objects - list, dictionary and tuple are taken into account.
# Python3 code to understand the utility of type() function
# Class of list type
class ListType:
ListNo = [16, 23, 38, 43, 59]
# it Will print the object type of existing class ListType
print(type(ListNo))
# Class of dict type
class DictType:
DictNo = {1:'Computer', 2:'programming',
3:'is', 4:'undoubtedly', 5: ‘interesting’}
# It will print the object type of existing class DictType
print(type(DictNo))
# Class of tuple type
class TupleType:
TupleNo = ('Java', 'T', 'point', ‘learning’, ‘website’)
# It will print the object type of existing class TupleType
print(type(TupleNo))
# Creating object of each class
d = DictType()
l = ListType()
t = TupleType()
Output:
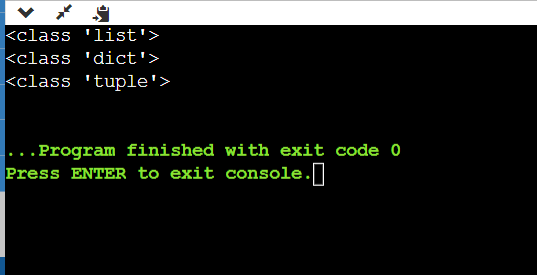
4. Input command
To take input from users, the input command is taken into account.
The flow continues as long as the user doesn’t press any key.
Syntax: input(message)
Here, the message inside the parenthesis refers to the text that programmers intend to show to the user.
Example 1: The following code illustrates the usage of command input() is in python. Here, the two integers are being taken as input.
# Python program to understand the use of input() command
val1 = input("Enter the 1st value: ")
print(val1)
val2 = input("Enter the 2nd value: ")
print(val2)
Output:
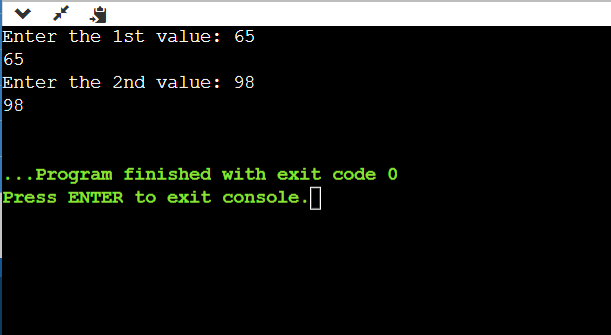
Example 2: The following program shows how to check the type of inputted value. Here, integer and string are considered.
# Program to check the type of inputted value in Python
x = input ("Enter any number :")
print(x)
s = input("Enter any name : ")
print(s)
# Printing type of input value
print ("type of number", type(x))
print ("type of name", type(s))
Output:
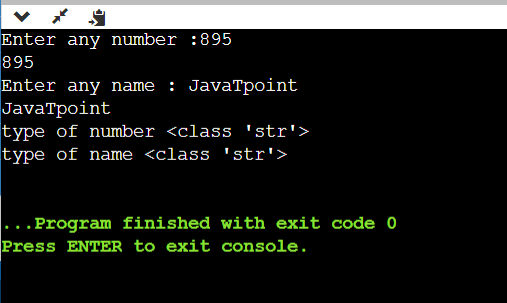
5. len command
To fetch the total number of items present in an object, length() or len() commands are used.
It returns the total no. of characters present if the input object is a string.
It returns the total no. of elements present if the input object is a list or a tuple.
It returns an error if the input object is an integer.
Syntax: len(object)
Here, the object inside parenthesis refers to the target object whose length is to be found.
Example: This example shows the usage of the len command. Here, three objects String, tuple and list are being considered.
# Python program to illustrate the use of the len() method
# with string
str = "javatpoint"
print(len(str))
# with tuple
tpl = (12,72,39)
print(len(tpl))
# with list
lst = [11,27,32,45]
print(len(lst))
Output:
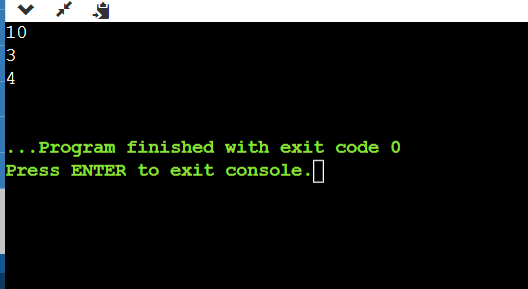
6. Range command
To create an order of integers that starts from 0 (start value is 0 by default) and ends before n.
Here n is not included in the created numbers.
This command is mostly employed in the case of loops.
Syntax: range (start value, stop value, step value)
Here,
The start value marks the starting of the range, it is 0 by default.
Stop value marks that no. before which user wants to terminate the loop.
The step value marks the count of increments in the given range.
Example:
# Python Program to understand range () command
# the numbers less than 20 are getting printed
for i in range (20):
print (i, end=" ")
print ()
#Here, the range command is being used for iteration
lst = [10, 20, 30, 40]
for i in range (len (lst)):
print (lst[i], end=" ")
print ()
# sum of first 20 natural number is being performed
sm = 0
for i in range (1, 21):
sm = sm + i
print("The Sum of first 20 natural number :", sm)
Output:
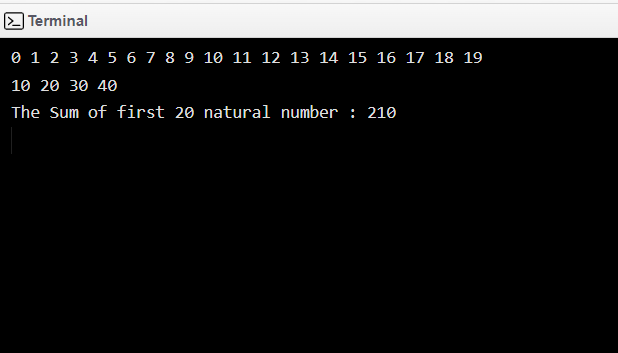
7.Round command
To round a number to specified correctness in decimal digits, the round command is taken into account. That means the round command can be used to round off the no. when there are so many digits after decimal in a float point number. Here, one can mention the no. of digits after the decimal point that is to be kept.
Syntax: round (number, digits)
Here,
The number refers to the no. that has to be rounded off.
Digits refer to the no. of digits that has to be kept after the decimal point.
Example 1: When only 1 argument is present. In the case of int, no rounding off but in the case of float, values get rounded off to the nearest number. If the value is close is less than 5, it gets rounded off to that no. only but if the value is greater than and equal to 5, it gets rounded off to the succeeding number.
# The program to understand round command in python when one argument is present
# for integer datatype
print (round (156))
print (round (16))
print (round (46))
# for float point datatype
print (round (86.6))
print (round (51.5))
print (round (51.4))
Output:
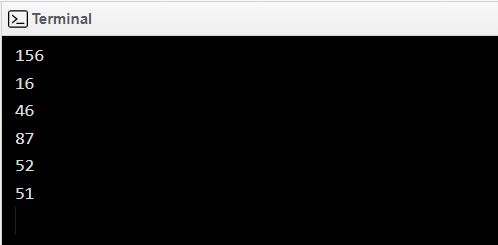
Example 2: When both the arguments are present, the value gets rounded off as per the no. of digits specified in the 2nd argument.
#program to understand round command in python
# when the last second digit is =5
print (round (3.6715, 3))
# when the last second digit is >=5
print (round (4.6726, 2))
# when the last second digit is <5
print (round (8.63973, 4))
Output:
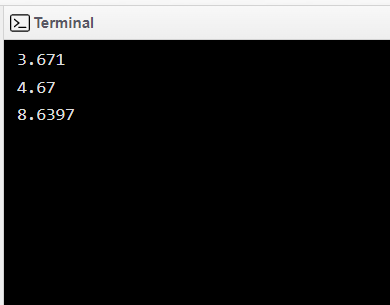
8.Loop command
While and for are two primitive loop commands used in python language.
Here, we will try to understand the working of both of them.
while loop
It is a looping command that gets executed as long as the condition is true.
Syntax:
Condition of while:
statements
update iterator
Example 1: The code here illustrates the working of the while loop
# Python program to understand while loop
Counter=0
While (counter < 10):
Counter= counter + 1
Print(“JavaTpoint)
Output:
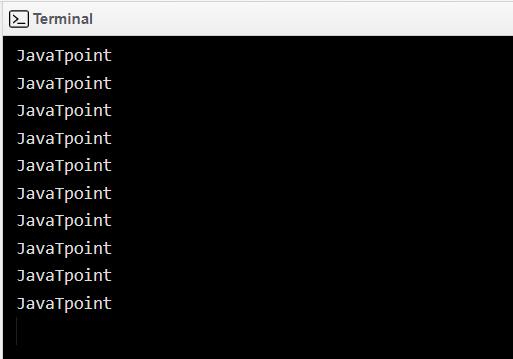
Example 2: The code shows the working of the while loop
# Python program to understand a single statement while block
counter = 0
while (counter < 5): counter += 1; print("Bonjour World")
Output:
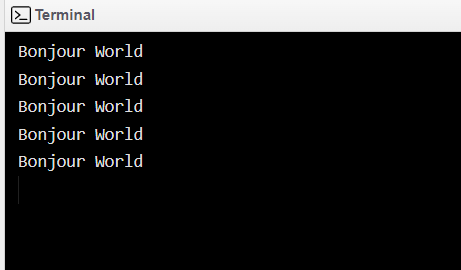
For loop
It is a looping statement that is used to execute a statement for the given no. of times as long as the condition is true.
Syntax:
for x in sequence:
statements
Example 1: Now, let us see the code to understand the code better.
#python program to understand the working of for loop
hillstation = ["Nainital", "Darjeeling", "gangtok", "Manali"]
for x in hillstation:
print (x)
Output:
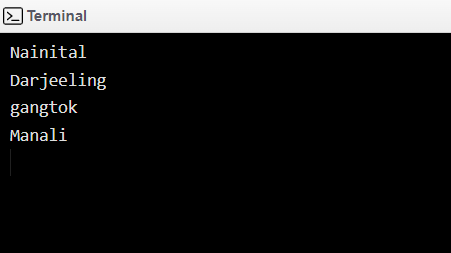
Example 2: Now, let us understand the code to understand the code better
# A python program to illustrate the for loop which use a break statement
Hillstations = ["Nainital", "Darjeeling", "Gangtok", "Ooty", "Manali"]
for x in Hillstations:
print (x)
if x == "Ooty":
break
Output:
Here, the hill stations till Ooty gets printed as the loop encounters a break statement after that.
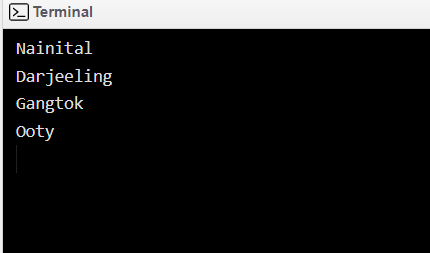
Let us now learn some string commands used in python.
- isalnum()– To check if all the characters of a given string are either alphabets or numbers, the isalnum command is used. (alphabet and numbers are together called alphanumeric characters)
It returns a Boolean value after checking all the characters of the string.
Syntax: stringname.isalnum()
Example:
# Python program to illustrate the usage of isalnum command
# string contains either alphabet or number
Val1 = “Newyear2023”
Print(val1.isalnum())
# string contains whitespace
Val2 = “New Year 2023”
print(val2.isalnum()
Output:
val1 contains the alphabet as well as numbers so the output is true.
val2 contains the white space character along with alphabet and number so the output is false.
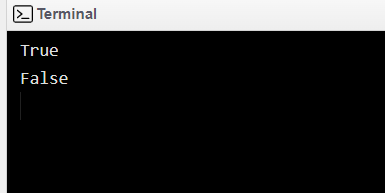
- capitalize() - Toconvert the first character of the string to uppercase if it is written in lowercase, the capitalize command is taken into account. It will leave the string as it is if the first character of the string is uppercase or an integer or any special character.
Syntax: stringname.capitalize()
Example:
# Python program to illustrate the usage of capitalize command
string = "JAVATPOINT is very useful website"
# it converts the first character of the string into uppercase and others to lowercase
capitalized_string = string.capitalize()
print (capitalized_string )
Output:
The first character of the string here is J which remains as it is while the other characters get converted into a lower case.
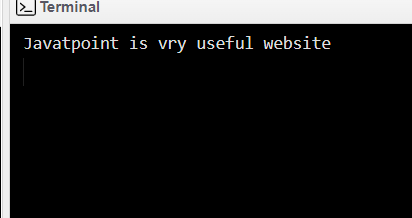
- find() -To search for a substring present in a string, the find() command is taken into account. It returns the index of the foremost occurrence of the substring if it is present else it returns -1.
Syntax: string.find(substring)
Here,
Substring marks that part of a string that is to be found in a larger string
Example:
#python program to illustrate the find command
message = 'programming is really interesting as it enhances the thinking ability’
# checking the index of 'it'
Print (message.find('it'))
# checking the index of 'thinking'
Print (message.find ('thinking'))
Output:
The code is written to understand the use of the find command.
The word which is to be found and its index is given as output.
Here, the index of ‘it’ is 36 which is shown in the output, and the index of the word ‘thinking’ is 52 which is given as output.
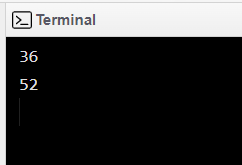
- center() -To line up a string in the center, using a stated character (which is by default a space) as the fill character.
Syntax: string.center (length, character)
Here,
Length marks the maximum length of the string
Character marks the character that is to be inserted
Example:
#python program to illustrate center command
string = "India is a great country"
# returns the centered padded string of length 24
string1 = string.center(50, '*')
print(string1)
Output:
The code illustrates the usage of the center command. Through this command, asterisks get inserted at the left end as well as at the right end keeping our string in the center.
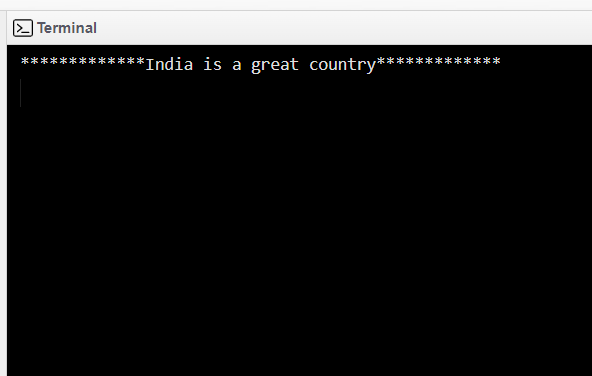
s
- count() - To return the sum of occurrences of a substring in a string object, the count function is taken into account.
Syntax: stringname.count (substring, start index, end index)
Here,
Substring marks the string which we want to search in the main string.
The start index marks the starting index of the substring which we want to search.
The end index marks the last index of the substring which we want to search.
Example 1: When start and end indexes are not provided.
#python program to understand the usage of count command
string = 'javaTpoint is a popular website among engineers'
# number of occurrences of character 'i'
print('Number of occurrence of character i:', string.count('p'))
# number of occurrences of character 'a'
print('Number of occurrence of character a:', string.count('a'))
Output:
The code here illustrates the usage of the count command. Here, the no. of occurrence of characters p and a are being calculated. Here, its occurrence is being checked in the whole string rather than the part of the string.
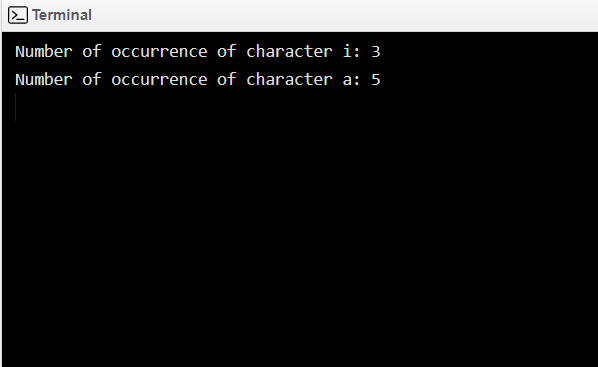
Example 2: When substring, start and end indexes are present.
# defining the string
string = "java is a good language, isn't it?"
substring = "o"
# counting after first 'o' and before the last 'o'
count = string.count(substring, 5, 45)
# printing the count of occurrence of o
print("The count of character o is:", count)
Output:
In this code, the usage of command count is illustrated when starting as well as ending indexes are provided.
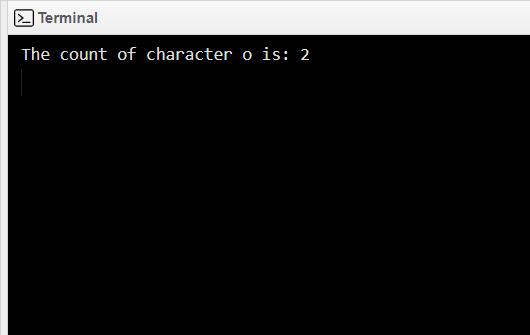
List commands in python
Let us see some of the list commands used in python.
1. append()
To add an element at the end of a list, the append command is taken into account.
Syntax: list.append (element)
The list marks the list object in which one wants to add an element.
An element marks the new item that one wants to add to the list.
Example:
tourist_place = ['Darjeeling', 'ooty', 'Nainital', 'Silliguri']
print(tourist_place)
tourist_place.append("Shimla")
print(tourist_place)
Output: In this example, the element Shimla is being added to the list named tourist_place
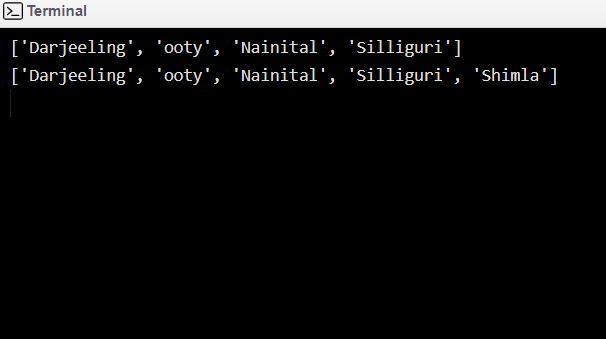
2. copy()
To create a new copy of the list object, the copy command is taken into account. A new list object is returned.
Syntax: list.copy()
Example –
#python program to illustrate the copy command
tourist_place = ['Darjeeling', 'ooty', 'Nainital', 'Silliguri']
print(tourist_place)
x = tourist_place.copy()
print(x)
Output –
In this example, the content of one list named tourist_place is being copied to another list named x.
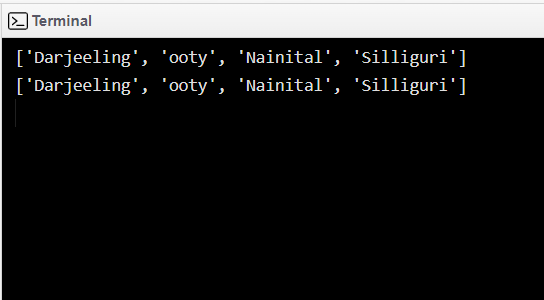
3. insert()
To add an element at a specified position in the list object, the insert command is taken into account. It takes two parameters position and an element.
Syntax: list_name.insert(location, element)
Example –
# creating a list of odd numbers
odd_numbers = [21, 33, 75, 57, 25, 43]
print('Old List:', odd_numbers)
# inserting 41 at index 5
odd_numbers.insert(5, 41)
print('New List:', odd_numbers)
Output –
In this example, a new element is inserted in the list named odd_numbers with the aid of the insert command.
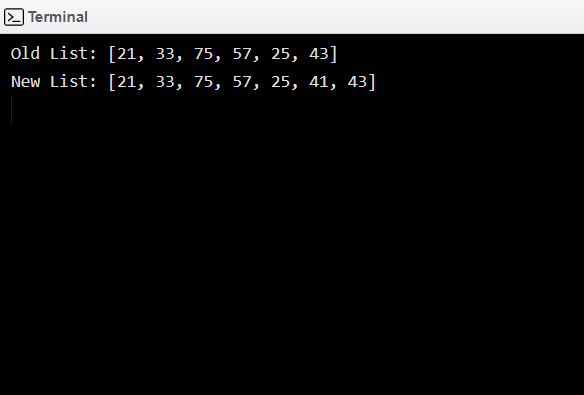
location refers to the position where one wants to insert a new element. The element will always get inserted at the end when one gives a position greater than the number of elements in the list.
element refers to the new element that needs to be added to the list.
4. pop()
To eliminate an element from a specified location in the list, the pop command is taken into account. The element is returned after removing it from the list.
Syntax: listname.pop(location)
location refers to the position in which one wants to remove the element from the list.
Example-
# Creating a list of odd numbersof odd numbers
odd_numbers = [23, 63, 35, 87, 90, 67, 54]
# Removing the element at index 5
popped_element = odd_numbers.pop(5)
print('Popped Element:', popped_element)
print('List after updatation:', odd_numbers)
Output:
Here, the element presents at index 5 is popped from the list named odd_numbers.
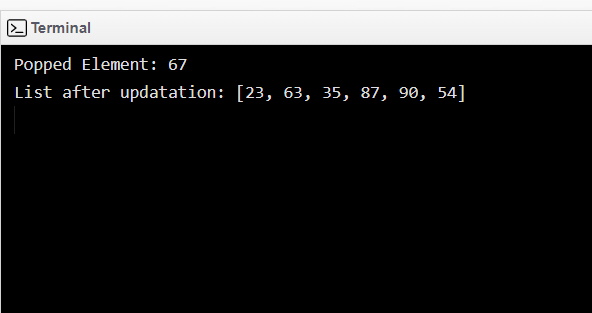
5. reverse()
To reverse the order of all elements in the list, the reverse command is taken into account. the original list object is modified but nothing is returned.
Syntax: list.reverse()
#Python program to reverse the strinf
tourist_places = [‘Darjeeling’, ‘Ooty’ ‘Nilgiri’, ‘Rajgir’, ‘Agra’]
tourist_places.reverse()
Print(tourist_places)
Output:
Here, the elements present in a list named tourist_places are reversed using the reverse command.
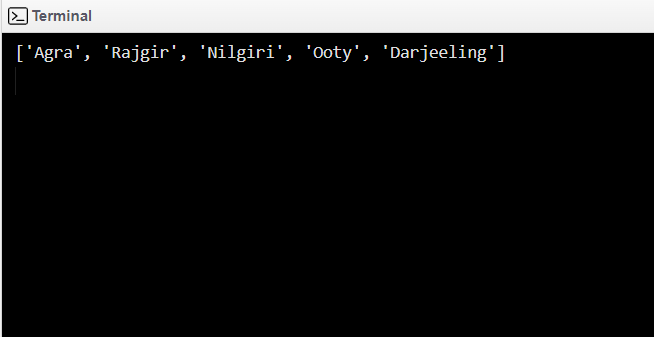
To sort the elements of the list in ascending order by default, the sort command is taken into account.
6. sort()
Syntax: list.sort()
Example:
#Python program to illustrate the sort command
tourist_places = [‘Darjeeling’, ‘Ooty’ ‘Nilgiri’, ‘Rajgir’, ‘Agra’]
print(“Before sorting:”)
print(tourist_places)
print(“After sorting:”)
Print(tourist_places)
Output:
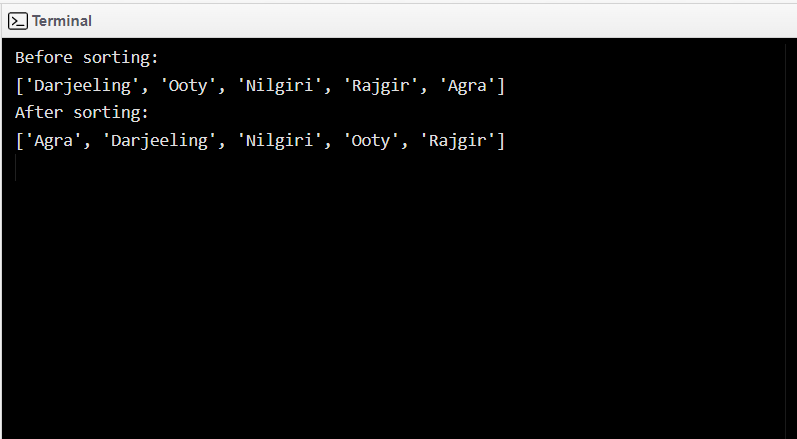
Tuple commands in python
Now, let us discuss some commands of tuples.
1.. count():
To count the existence of an element in the tuple, the count method is taken into account.
Syntax: tuple.count(element)
An element marks that element that is to be counted.
#python program to understand count command in tuple
# tuple of vowel
vowels = ('a', 'e', 'i', 'o', 'i', 'u', 'o', 'i')
# count of element 'o'
count = vowels.count('o')
# printing the count of 'o'
print('The count of o is:', count)
# count of element 't'
count = vowels.count('t')
# printing the count of 't'
print('The count of t is:', count)
Output:
Here, the occurrence of vowel o is 2 and the occurrence of vowel t is 0
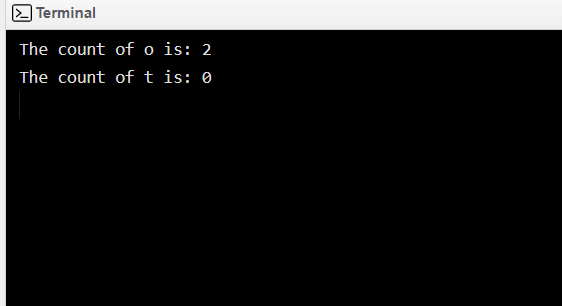
2. index ()
To find the index of the first occurrence of an element, the index () method is taken into account. It will raise a ValueError if the searched element is not found in the whole tuple.
Syntax: tuple.index(element)
element refers to the item that is to be searched.
Example:
#python program to understand the index command used in tuple
animals = ['cat', 'giraffe', 'goat', 'tiger', 'dog', 'rabbit', 'horse']
tourist_place = ['Darjeeling', 'Agra', 'ooty', 'Nainital', 'Silliguri']
# get the index of 'ooty'
index1 = animals.index('dog')
index2 = tourist_place.index('ooty')
print(index1)
print(index2)
Output:
Here, the dog is present at the 4th position in tuple named animals and the Ooty is present at the 2nd position in a tuple named tourist_places.
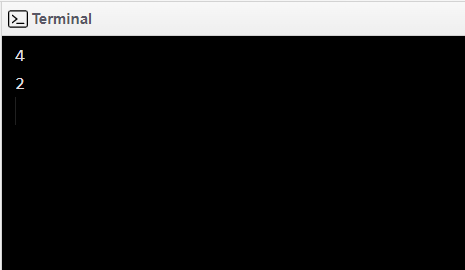