Write a String in Python
Python is an object-oriented high-level programming language. Python has dynamic semantics and has high-level built-in data structures which support dynamic typing and dynamic binding. Python provides rapid development. It has an English-like syntax that is very easy to write and understand, which also reduces the maintenance cost. Python is an interpreted language which means that it uses an interpreter instead of the compiler to run the code.
The interpreted language is processed at the run time thus takes less time to run. Python also has third parties modules and libraries which encourage development and modifying the code.
While working in Python, we need to store data or export some and import some data to the files. The output of a program is not permanent, and it gets destroyed as soon as we close the program, so what if we want to store the output? So, in that case, file handling comes to help us.In this article, we will discusshow to write in text files in Python.
Python provides an in-built function to read and write files, so it is simple to handle files in Python. We can handle two types of files in Python, which are:
- Text Files: These are the default text files. In these files, every line of the text is terminated with a special character called End of Line (EOL). It is '\n' by default in normal text files.
- Binary Files: These files do not have any End of Line characters, and the data is stored in binary language, which the machine understands.
Writing in Text files.
To write in a text, we use some steps. The steps to writing a string in a file using Python are:
- Step 1: The first step is to open the file in WRITE or APPEND mode with the help of the open() function in Python.
- Step 2:The next step is to write in the file using the write() or write lines() functions by passing the string.
- Step 3: After everything is done, we can finally close the file using the close() functions.
Now, we will discuss these steps in detail
Opening a File
The syntax for opening the file using the open() function in Python is:
f = open(path_to_file, mode)
Like many functions, the open() function also accepts many arguments, but the mandatory ones are just two, which are:
- path_to_file: The path_to_file parameter is used to specify the path of the file on which we want to write the text on.
- Mode: The mode parameter is used to assign the permission we want to open the file, e.g., Write, Read, etc.
In this case, we want to write a text file, so the mode we can use are:
- w: w mode is used to open the text file with WRITE permissions so that we can write on it. It creates the file if it does not already exist. If we open an existing file, then the data we write gets over-written to it.
- The file is created if it does not exist already
- . a: a mode is used to open the file with APPEND permission to append the text we want to write. The data we write is appended at the end of the file.
Writing in a File
After opening a file with the open() function, we get a file object returned by the open function. To write the text on the file, we can use the two functions available in this file object which are written () and write lines().
With the write() function, we can write a string to a file, and with the writelines() function, we can write multiple strings by using the list of strings in a file.
We can also pass iterable objects like tuples or a set of strings and not just a list to the writelines() function.
After writing a line in a file, we will need to add a new line character manually.
To add the new line character in a text file we can use the code below:
f.write('\n')
f.writelines('\n')
Writing Text files examples.
Writing using write() function
Below is the example of how we can use the write() function to write a string in a file in Python.
strings = ['Example 1', 'How to write strings in text file in Python']
with open('Example1.txt', 'w') as file:
for line in strings:
file.write(line)
file.write('\n')
Output
After running the above code, a new file called Example1.txt will be created with the following content:
Example1
How to write strings in the text file in Python
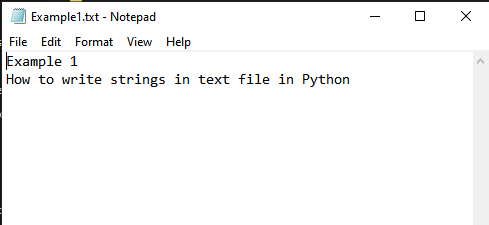
Note: If the Example1.txt already exists, then this code will overwrite the strings to the original file.
Writing using write lines() function
write lines() function is used to write multiple lines in a text file using Python. Below is the code of using the writelines() function:
strings = ['Example2', 'How to write multiple text lines in file in Python']
with open('readme.txt', 'w') as file:
file.writelines(strings)
Output
The output of the above code will be:
Example2How to write multiple text lines in a file in Python
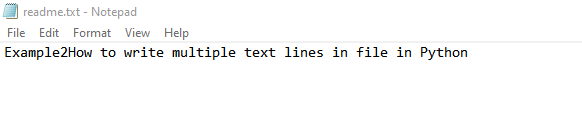
If we want to add every string in the list in a new line, we have to add the new line character in the code manually.
strings = ['Example 3', 'How to write multiple text lines in file in Python']
with open('readme.txt', 'w') as file:
file.writelines('\n'.join(strings))
Output
The output will be a text file with every string in a new line.
Example 3
How to write multiple text lines in a file in Python
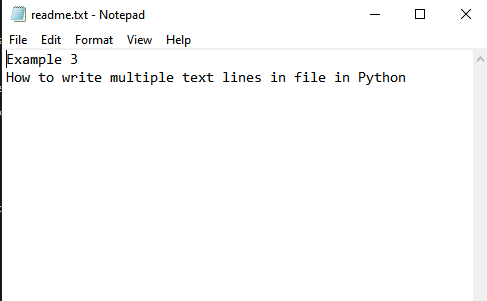
As you can see, the write mode has overwritten the previous text in the file.
Appending Text File
Let us append some more lines to the file we created in Example 3. To append the strings in a file, we need to open the file with append permission. Let us see the code for appending strings:
multiple_lines = ['', 'Example 4', 'Append new lines' ]
with open('readme.txt', 'a') as file:
file.writelines('\n'.join(multiple_lines))
Output
After running the file, the list multiple_lines will get appended to the file readme.txt
Example 3
How to write multiple text lines in a file in Python
Example 4
Append new lines
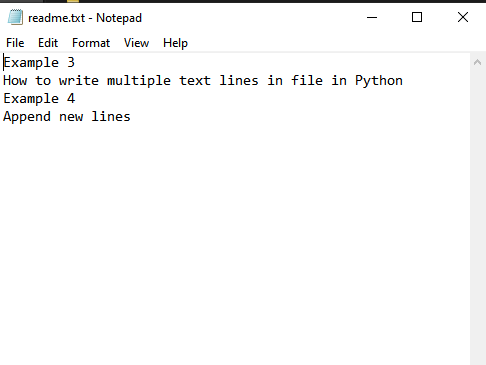