How to Call a Function in Python
How To Call a Function in Python
Functions are the well-defined and structured piece of code that is used to implement specific functionality. Calling a function in python is the best way to reduce the size of code. Whenever we talk about using 'functions' in a code, we have to mention the following three things-
- Function declaration
- Function definition
- Function calling
The reason behind why python is the most commonly used language among developers is its readability and how it simplifies complex programs.
So, when we are using functions in our python program, we just need to define and call it.
Let’s look at an example where we will see how functions are called in python.
def add(a,b): #function to add two numbers return a+b x=10 y=20 print(add(x,y)) #function call
The above example shows how we can add two numbers in Python using functions.
The way to define functions in Python is-
def function_name(parameters):
return _______
- The keyword ‘def’ is used to define a function in Python
- After that, we must define the name of our function.
- And then specify the number of parameters involved in our function.
- Finally, we will write the expression required for desired result or output preceded with the keyword return.
In the example given above, we have made a function that adds two numbers.
We have defined the function using the 'def' keyword, named our function like add, and specified the parameters a and b that will hold the values for further computation.
Since the function 'add' will give us the addition of two numbers as the output, we will write this statement as return a+b.
Now the next step is to take the values from the user to check how our function works-
The values from the user can be taken in two ways-
- Assign the variables a value like-
x=10 y=20
- Ask the user to input the values of these variables using the ‘input’ function like-
x=int(input(“Enter value of x: ”)) y=int(input(“Enter value of y: ”))
Once we receive the values for our variables, we can call the function 'add' and display the result using the print function.
When the function is invoked, the control of the program passes to the called function, executes the statements/computes the values (in case of a mathematical expression) and then the control again comes back to the calling function.
Let’s look at some points we must keep in mind while declaring a function-
- The function name is given so that it can be identified uniquely in a function.
- Make sure you place a colon outside the parentheses in which you have specified the parameters.
- If your function is meant to simply print a statement or a set of statements, then you need not write anything in the parentheses.
For example-
def message(): print("Hello All") message() message()
- The indentation should be proper in the code block.
- The number of arguments at the time of calling the function must be the same as specified in the function definition.
- The return statement should be present within the function.
- Once a value is returned from a function, it instantly marks the exit of that function.
LAMBDA FUNCTIONS
Lambda or anonymous functions are the functions that are not declared with the keyword ‘def’ and are a one-line version of our function.
They are created with the help of keyword lambda and contain a single line.
Let’s understand this with the help of an example-
add=lambda a,b: a+b print(“Addition =”,add(2,4))
In the above example, the lambda function gives the sum of two parameters a and b, the output is evaluated with the help of the expression a+b and then the result is displayed.
Some Properties of Lambda Functions-
- There is no need to give a function name in this case.
- It returns a single value which is obtained by evaluating an expression.
- There is no need to explicitly mention the return statement.
- Since they are a one-line version, they cannot have multiple statements or expressions.
- They can’t access global variables.
Let's have a glance at some examples of functions-
- Program to add two numbers using functions
def sum(x,y): res=x+y print("Sum of {} and {} is {}".format(x,y,res)) x=int(input("Enter the value of x: ")) y=int(input("Enter the value of y: ")) sum(x,y)
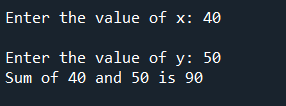
- Program to find cube of a number
cube=lambda x:x*x*x print("Cube is {}".format(cube(9)))
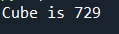