Application to Search Installed Application using Tkinter in Python
Tkinter:
The standard Python technique for building Graphical User Interfaces (GUIs) is Tkinter, which is included in all popular Python distributions. The only framework included in the Python standard library is this one. On top of Tk, this Python framework serves as a thin object-oriented layer that provides access to the Tk toolkit. The Tk toolkit is a cross-platform set of "graphical control elements," also called widgets, used to build application interfaces. This framework gives Python users a quick and easy way to build GUI elements with the Tk toolkit's widgets. In a Python application, Tk widgets can create buttons, menus, data fields, etc.
Once created, these graphic elements can be connected to or work with other widgets, features, functionality, processes, or data.
Tkinter bridges the gap between Python's execution model and Tcl's, built around cooperative multitasking. Like Python, Tcl is an interpreted dynamic programming language. Although it is an overall programming language that can be used independently, it is most frequently used in C programs as a scripting motor or as an interaction with the Tk toolbox. The Tcl library has a C interface that enables users to add custom Tcl or C commands, run Tcl commands and scripts, and manage one or more Tcl interpreter instances. Each interpreter has a queue for events that can be used to send and process events.
Syntax:
from tkinter import *
from tkinter import ttk
Advantages of Tkinter:
This promotes flexibility in handling GUI incidents, which makes the code base run more smoothly. Qt is more than just a framework; it uses a variety of native platform APIs for networking, database development, and other uses. Through a unique API, it gives them direct access.
Disadvantages of Tkinter:
Tkinter doesn't have any advanced widgets. There isn't a tool like Qt Designer for Tkinter in it. Its user interface could be more reliable. Tkinter can be challenging to debug at times. It's not entirely in Python.
Other Python GUI frameworks exist, but Tkinter is part of the standard library. Tkinter offers several benefits. Since the code is cross-platform, it functions equally well on Windows, macOS, and Linux. Applications made with this framework seem to belong on the system being used because Tkinter creates visual elements using native operating system components. Tkinter is generally easy to use and less expensive than competing frameworks. This makes it an enticing solution for individuals who want to create a GUI in Python, especially when producing something cross-platform and quickly functional is the main priority and when a current polish is unnecessary.
To search for an application from the installed applications, we need to install some libraries, which are tkinter, winapps are predefined to give detailed information about the version, installed data, publisher, and uninstall string in the output.
CODE:
from tkinter import *
import winapps
def app():
for item in winapps.search_installed(e.get()):
name.set(item.name)
version.set(item.version)
Install_date.set(item.install_date)
publisher.set(item.publisher)
uninstall_string.set(item.uninstall_string)
master = Tk()
master.configure(bg='light grey')
name = StringVar()
version = StringVar()
Install_date = StringVar()
publisher = StringVar()
uninstall_string = StringVar()
Label(master, text="Enter App name : ",
bg="light grey").grid(row=0, sticky=W)
Label(master, text="Name : ",
bg="light grey").grid(row=2, sticky=W)
Label(master, text="Version :",
bg="light grey").grid(row=3, sticky=W)
Label(master, text="Install date :",
bg="light grey").grid(row=4, sticky=W)
Label(master, text="publisher :",
bg="light grey").grid(row=5, sticky=W)
Label(master, text="Uninstall string :",
bg="light grey").grid(row=6, sticky=W)
Label(master, text="", textvariable=name,
bg="light grey").grid(row=2, column=1, sticky=W)
Label(master, text="", textvariable=version,
bg="light grey").grid(row=3, column=1, sticky=W)
Label(master, text="", textvariable=Install_date,
bg="light grey").grid(row=4, column=1, sticky=W)
Label(master, text="", textvariable=publisher,
bg="light grey").grid(row=5, column=1, sticky=W)
Label(master, text="", textvariable=uninstall_string,
bg="light grey").grid(row=6, column=1, sticky=W)
e = Entry(master, width=30)
e.grid(row=0, column=1)
b = Button(master, text="Show", command=app, bg="Blue")
b.grid(row=0, column=2, columnspan=2, rowspan=2, padx=5, pady=5,)
mainloop()
CODE EXPLANATION:
Importing all the required modules to get processed [winapps]. Apps method is attached for output. Use the set() method to set the data like the name of the application, version of the application, installation date of the application, and publisher of the application. The uninstalling string required for the application and set background using configure()[light grey], create variable classes in tkinter[name, version, publisher, install_date, publisher, uninstall_string then create a label for each information using a label() these labels() are positioned by using grid() method[select row as per the design. We also create an entry box to enter the application using entry(). In the entry box, we insert buttons, rows, columns, checkboxes, and many more.
OUTPUT:
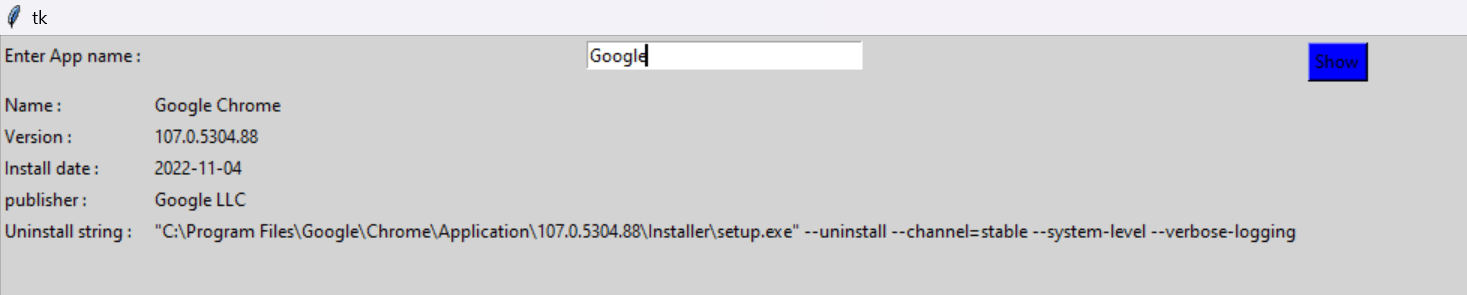