Python Arithmetic Operators
An arithmetic operator is a mathematical operator that is used to operate on two operands. Based on the operator used, action is performed on the operands, and output is delivered.
Following are the seven Arithmetic operators used in Python: -
- Addition operator
- Subtraction operator
- Multiplication operator
- Division operator
- Modulus operator
- Exponentiation operator
- Floor division operator
Now, let’s dive deeper and understand each of the above-mentioned operators:
1. Addition operator
In python, ‘+’ is used as the addition operator. It takes two operands. Operands can be both numbers positive as well as negative., It is also capable of adding two strings. The addition of string using ‘+’ is called string concatenation.
For Example-
- z = “ABC” + “def” ( string concatenation is performed )
The solution here will be ABCdef.
- x= - 5, y = - 7;
z = x + y; (addition of negative numbers )
Here, z = (-5) + (-7);
So, the answer would be -12.
Example 1: Implementation showing addition operator on two positive numbers.
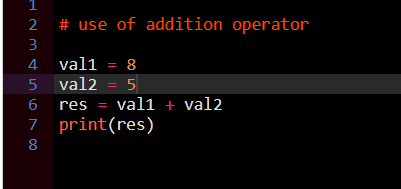
Output:
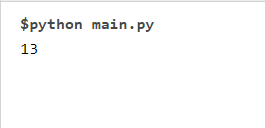
Example 2: Implementation showing addition operator on two strings.
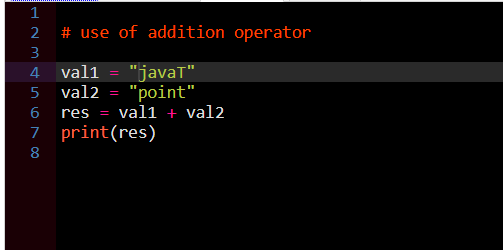
it simply adds both the operands and gives out the result.
Output:
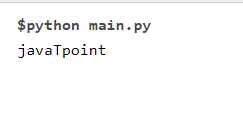
Example 3: Implementation showing addition operator on two negative numbers.
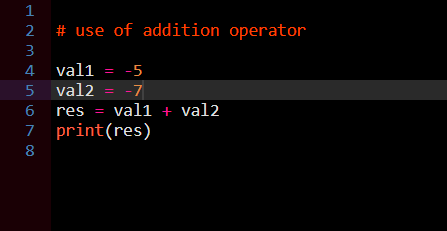
Output:
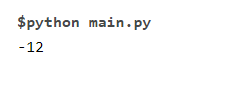
2. Subtraction operator
‘-’ is used as the subtraction operator in python. It also takes two operands which should be a number positive or negative. It is not capable of supporting string operations.
For Example-
- x = -9, y=4
z = x - y ;
Here, z = (-9)- 4;
So, the answer would be -13
Example 1: Implementation showing the usage of subtraction operator
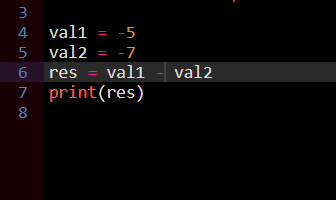
Output:
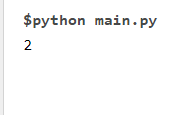
Example 2: Implementation showing the usage of subtraction operator on two strings.
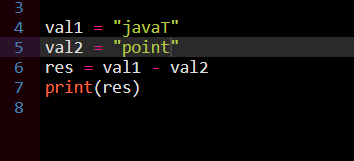
Output:
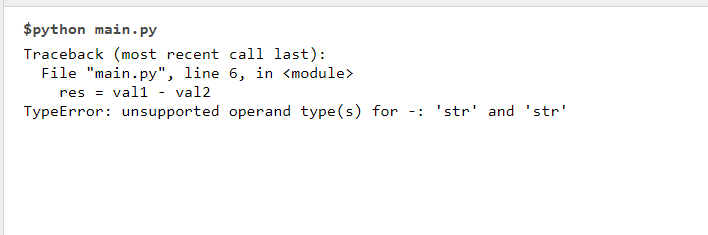
The above code throws an error as the subtraction operator is not capable of performing string operations.
3. Multiplication operator
In python, ‘*’ is used as a multiplication operator. It finds the product of two desired numbers.
For Example-
x = 6, y = 5;
z = x * y
Here, 5 * 6;
So, the answer would be 30.
Example 1: Implementation showing the usage of multiplication operator on two strings
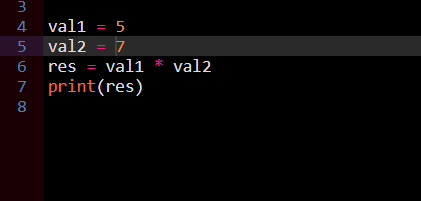
Output:
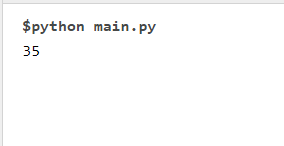
4. Division operator
In python, ‘/ ’ is used as a division operator. It gives out the quotient on dividing value 1 with value 2 or vice versa.
For Example-
x = 34, y = 5;
z = x / y;
Here, 34 / 5;
So, the answer would be 6.
Example 1: Implementation showing the usage of division operator on two positive integers.
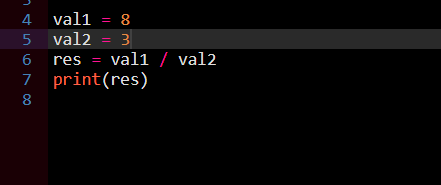
Output:
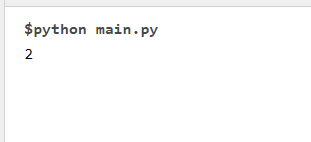
5. Modulus operator
In python, ‘ % ’ is used as a modulus operator. It gives out the remainder when value 1 divides the value 2.
For Example-
x = 24, y = 5;
z = x % y;
Here, 20 % 5
So, the answer would be 4.
Example 1: Implementation showing the usage of modulus operator on two positive integers.
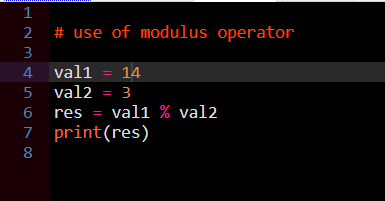
Output:
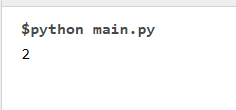
6. Exponentiation operator
‘**’ is the exponentiation operator used in Python. This operator is used to raise the first operand to the power of the second.
For Example-
x = 3, y=2;
z = x ** y;
Here, z = 3**2 (that is 3^2)
So, the answer would be 9.
Example 1: Implementation showing the usage of an exponential operator on two positive integers.
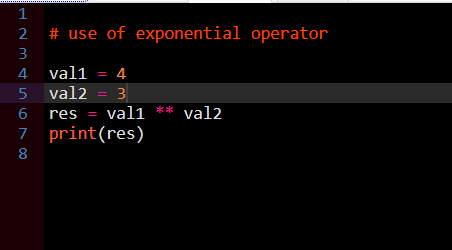
Output:
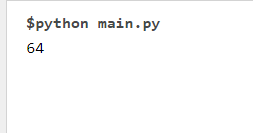
7. Floor division operator
In Python, ‘ // ’ is used to perform the floor division. It is used to calculate the floor of the quotient when the first operand is divided by the second.
For Example-
x = 5, y = 2;
z = x // y;
Here, 5//2
the answer would be 2.
Example 1: Implementation showing the usage of an exponential operator on two positive integers.
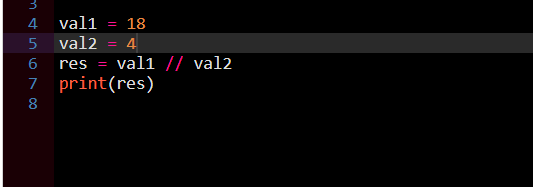
Output:
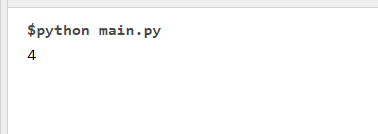
Summary:
We have seen all the seven arithmetic operators supported in python and we have also seen its implementations on python IDE.
These operators are not much different from mathematical operators used outside the programming world.