Is Python Case Sensitive
Case sensitivity is the mode of dealing with the written alphabet. The cases of the alphabet are examined and based on these words are being treated. The uppercase and lowercase letters or characters are considered dissimilar ways.
E.g. - ADMINISTRATION and administration are considered different words in any case-sensitive platform. The former contains all uppercase characters while the latter contains all lowercase characters.
It means we cannot enter and use uppercase and lowercase letters or characters interchangeably. Nowadays, the case sensitivity feature is widely being used. Passwords, email addresses, etc. are case sensitive.
In Computer World, the case sensitivity feature is becoming popular. Many Programming languages are sensitive to cases. C, C++, JAVA, RUBY, and PYTHON are their examples. JavaScript which is a scripting language is also case sensitive.
Some languages are case unresponsive. FORTRAN, BASIC, PASCAL, and PHP (scripting language used in web development) are some of them. The case of character does not affect them.
There exists a standard code UNICODE (Universal Character Encoding) for every character existing today.
They too assign separate codes for lowercase and uppercase alphabet.
Let’s dive deeper into understanding case sensitivity in python.
PYTHON is one of the popular case-sensitive programming languages. It means it treats two words written in different cases differently.
BONJOUR and bonjour are interpreted differently. Here, the case of each character is different from the others.
Bonjour and bonjour are interpreted in python differently. Even the difference in one character creates a vast difference.
Python checks characters present at the same index in both strings. It compares the Unicode values of the character starting with index 0 to the last index. After comparison, it returns the precise boolean value as per the used operator.
Illustration –
- print ("Civil" == " Civil " )
Output- True
Since UNICODE values of both words are the same. - print ("civil " < " Civil " )
Output- True
Since the UNICODE value of B is more than b - print ("civil " > " Civil " )
Output- False
Since the UNICODE value of b is less than B - print ("Civil " != " Civil " )
Output- True
Since UNICODE values of both words are different.
There are a set of predefined rules in programming languages that are to be kept in mind to avoid an error.
The function name should always begin with a lowercase character. If the function name contains multiple words, then they can be separated with an underscore.
A variable name cannot start with an uppercase character.
Example 1
Function1( ) {
int Ar;
}
Explanation: The snippetwritten above will produce an error as the naming convention has been violated.
Example 2
Function1( ) {
int Ar;
}
Explanation: The codewritten here will not produce an error as the naming convention has not been violated.
Illustration of case sensitivity issue
# problem with variable names of different cases
emp_first_name = 'Jessica'
emp_last_name = 'Ted'
print ('My name is ' + emp_First_Name + ' ' + EMP_last_Name )
OUTPUT:
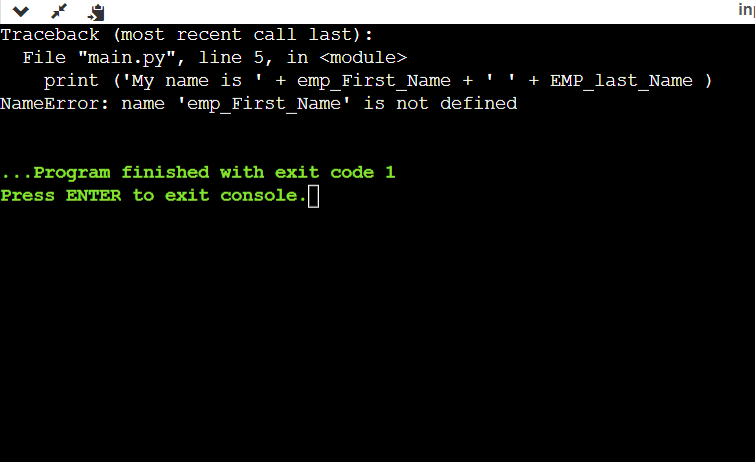
Explanation: This code results in an error.
The called variables are emp_First_Name and EMP_last_Name
The declared variables are emp_first_name and emp_last_name
The cases of the above variables do not match.
# resolving the case sensitivity problem when variable names of different cases are used
emp_first_name = 'Jessica'
emp_last_name = 'Ted'
print ('Employee name is ' + emp_first_name + ' ' + emp_last_name )
OUTPUT –
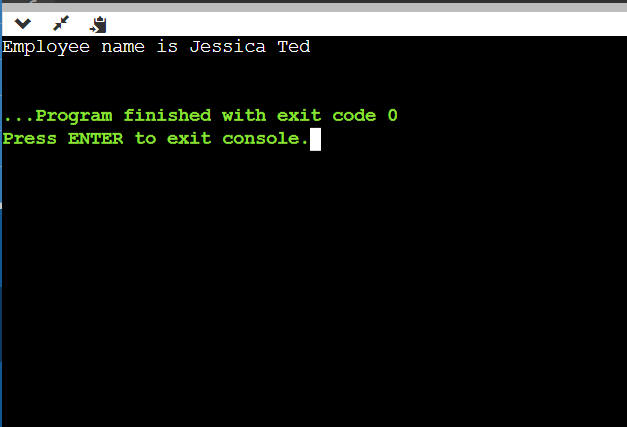
Explanation: This code works correctly and produces the desired output.
In Python, there are some functions to escape the case problem.
.lower( ) - This function can be taken into account to convert all uppercase characters into equivalent lowercase characters.
.upper( ) – This function can be taken into account to convert all lowercase characters into equivalent uppercase characters.
Illustration of the above functions –
# String comparison using .lower( ) function
str1 = ' PYTHON is an interesting programming language'
str2 = ' python is an interesting programming language'
evaluation = str1.lower() == str2
print(evaluation)
OUTPUT:
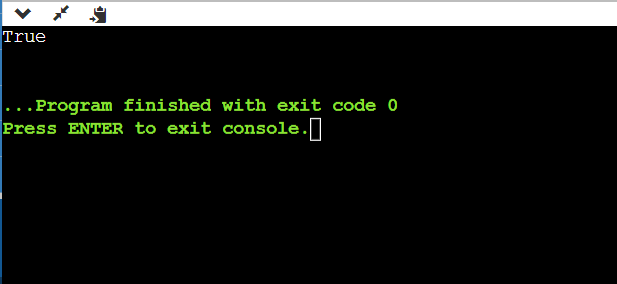
Explanation: Upper case PYTHON in str1 gets converted into lowercase python thus both become equal and there exists no difference between them.
#String comparison using .upper( ) function
str1 = ' JAVA is an interesting programming language'
str2 = ' JAVA is an interesting programming language'
evaluation = ( str1.upper() == str2 )
print(evaluation)
OUTPUT:
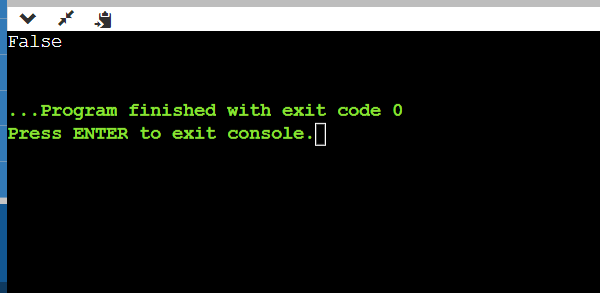
Explanation: Lower case PYTHON in str1 gets converted into uppercase python thus both become equal and there exists no difference between them.