Method Overloading in Python
Overloading is a capability of a method and operator to behave differently according to the nature of parameters.
Overloading is popular because it provides a good number of advantages:
- Reusability of code.
- Reduces the time required in writing the same code repeatedly.
- Debugging becomes easier.
- Decreases complication and raises clarity of code.
Python supports two types of overloading: -
- Operator overloading
- Function or Method Overloading
Operator Overloading
Use of arithmetic operators to provide special meaning to the operators. E.g.- ‘+’ can be used to add string and float besides int.
Other arithmetic operators like -, *, /, % can also be overloaded.
Method Overloading
Whenmore than one methodor functionhas an identicalname, but a dissimilar parameter list which results in varying method signatures, is termed method overloading.
Two or more no. of methods which are also called functions, can participate in method overloading based on the succeeding three criteria:
- Quantity of arguments
- Forms of arguments
- Order of arguments.
The basic syntax of a function:
Method1(int a) {
}
Method2(int a, int b){
}
Method Overloading is provided by most object-oriented programming languages such as C++, Java.
Python does not support method overloading by default, but it can be achieved in two ways which we will see in the upcoming part.
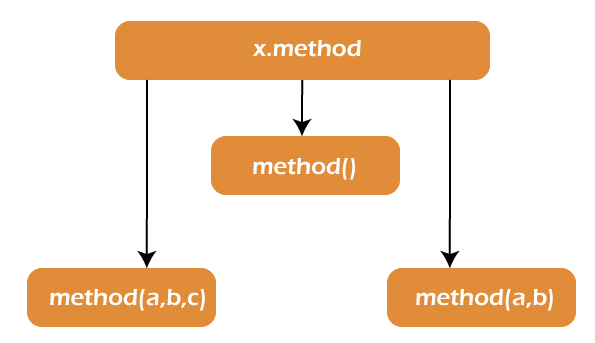
The issue with method overloading in Python
Multiple methods can also be overloaded here but only the newest defined method can be used making, other methods vague.
Here’s an example to understand the issue better.
# sum method 1 accepts two arguments and gives out their sum
def sum (a1, a2 ):
sm = a1 + a2
print(sm)
# sum method 2 accepts two arguments and gives out their sum
def sum (a1, a2, a3, a4):
sm = a1 + a2 + a3 + a4
print(sm)
# sum method 3 accepts three arguments and gives out their sum
def sum (a1, a2, a3):
sm = a1 + a2 + a3
print(sm)
# line below shows an error if it is not commented
# sum (7, 8)
# sum (44, 21, 6, 9)
# This line will call the third sum method
sum (8, 7, 9)
OUTPUT:
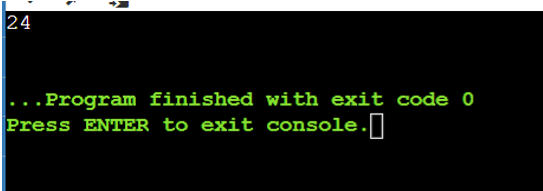
Explanation: Here, we can see the starting two-sum method has not been used. Only the newest one is in action. So, this would not fulfill our purpose.
We have got two ways to resolve this issue. Here we begin to see both of them one by one.
METHOD 1
# Function to input multiple arguments
def sum(data_type, *args):
# if datatype is int
# initialize answer as 0
if data_type =='int':
result = 0
# if datatype is str
# initialize answer as ''
if data_type =='str':
result =''
# Traversing through the arguments
for i in args:
# This will perform addition if the
# arguments are int. Or concatenation
# if the arguments are str
result = result + i
print(result)
# Integer data
sum('int', 45, 86)
sum('int', 78, 87)
# String data
sum('str', 'Hello ', 'developers')
sum('str', 'Hello ' , 'aspirants')
OUTPUT:
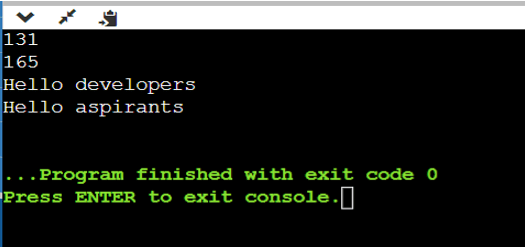
Explanation: This method solves the issue, but the usage of if…else statements increase the no. of lines of code which makes the code complex. Thus, it is not an effective way of method overloading.
METHOD 2
Using the Multiple Dispatch Decorator tool, which is of great utility in python.
Prerequisite:
The multiple dispatch Decorator tool has to be installed.
pip3 install multiple dispatch
Let’s understand through an example-
from multipledispatch import dispatch
#passing one parameter
@dispatch(int, int)
def addition(first, second):
sum = first + second
print(sum);
#passing two parameters
@dispatch(int, int, int)
def addition(first, second, third):
sum = first + second + third
print(sum);
#passing values of float datatypes
@dispatch(float, float, float)
def addition(first, second, third):
sum = first + second + third
print(sum);
#passing values of float and int datatypes
@dispatch(float, int, int, float)
def addition(first, second, third):
sum = first + second + third
print(sum);
#calling addition method with 3 arguments of int datatype
addition(7,8,9)
#this will give an output of 24
#calling addition method with 3 arguments of float datatype
addition(4.8,2.2,3.0)
# this will give output of 10.0
#calling addition method with 4 arguments of float and int datatype
addition(5.0, 3, 6, 5.0)
# this will give an output of 19.0
Although, Python doesn’t provide a default method of overloading, it can be altered to use method overloading by using the Multiple dispatch decorator tool.