Struct Module in Python
What is a structure in Python?
An entity that holds data in form of a structure is called a data structure. In Python, the data structure includes tuples, lists, dictionaries, and sets.
To use data structures in Python, we use the struct module. Though this module is available in Python 3.x, and cannot be used in older versions.
Functions in struct module
There are multiple functions available in the struct module that can be easily used after importing this module. The following functions are available in this module
struct.pack()
This function is used to print the string that contains the provided values in the format declared. (The formatting string is a mechanism that is used to specify the format of data while packing and unpacking). To make this mechanism work, the values passed should be passed according to the format, or else an error is raised.
Syntax:
struct.pack(format_string, a1, a2, a3, ....)
Example:
import struct
# Here, format ‘i’ is int in C type
# and the format 'iii' shows 'int intint’.
val=struct.pack('iii',1,2,3)
print(val)
# Here, format ‘i’ is int in C type
#and format ‘l’ is long in C type
# and the format 'hhl' shows 'short short long'
val=struct.pack('hhl',1,2,3)
print(val)
Output:
b'\x01\x00\x00\x00\x02\x00\x00\x00\x03\x00\x00\x00'
b'\x01\x00\x02\x00\x00\x00\x00\x00\x03\x00\x00\x00\x00\x00\x00\x00'
struct.unpack()
This function is used to print the data that is unpacked according to the format specified. It returns the data in the format of a tuple with the size depending on the values passed in the struct.pack() function during packing.
Syntax:
struct.unpack(formatstring, name of string)
Example:
import struct
# Here, '?' stands for BOOL, 'h' stands for short, 'i' stands for
#int and 'l' stands for long
val=struct.pack('?hil', True, 3, 8, 668)
print(val)
# The struct.unpack() function is used to return tuples
# Variables a1, a2, a3, ... are returned as elements of the tuple
tpl=struct.unpack('?hil', val)
print(tpl)
# Here, q stands for long long int and f stands for float
val=struct.pack('qf', 8, 5.3)
print(val)
tpl=struct.unpack('qf', val)
print(tpl)
Output:
b'\x01\x00\x03\x00\x08\x00\x00\x00\x9c\x02\x00\x00\x00\x00\x00\x00'
(True, 3, 8, 668)
b'\x08\x00\x00\x00\x00\x00\x00\x00\x9a\x99\xa9@'
(8, 5.300000190734863)
struct.calcsize()
This function is used to calculate and return the size of the structure corresponding to the given format. This is an important function, as struct.pack() and struct.unpack(), they both require buffer and offset values, and this function is useful for them.
Syntax:
struct.calcsize(format)
Example 1:
import struct
val=struct.pack('?hil', True, 3, 8, 678)
print(val)
# Returning the size of the structure
sze=struct.calcsize('?hil')
print(sze )
sze=struct.calcsize('qf')
print(sze )
Output:
b'\x01\x00\x03\x00\x08\x00\x00\x00\xa6\x02\x00\x00\x00\x00\x00\x00'
16
12
Example 2
import struct
val=struct.pack('bi', 56, 0x12131415)
print(val)
sze=struct.calcsize('bi')
print(sze )
val=struct.pack('ib', 0x12131415, 56)
print(val)
sze=struct.calcsize('ib')
print(sze )
Output:
b'8\x00\x00\x00\x15\x14\x13\x12'
8
b'\x15\x14\x13\x128'
5
Note: The ordering of format characters can have an impact on the size.
Exception struct.error()
The exception struct error tells us the problem with the argument passed; this kind of error is raised when any wrong argument is passed.
Example:
from struct import error
print(error)
Output:
<class 'struct.error'>
Note: This is used only in exception handling, to show the error that occurred.
struct.pack_into()
This function is used to pack the data into the buffer.
Syntax:
struct.pack_into(frmt, bfr, ofst, a1, a2, a3, ...)
Here,
- frmt: The format for the data type
- bfr: The writable buffer which starts at offset (optional)
- a1, a2, a3, ... :The valuesthat are passed
struct.unpack_from()
This function is used to unpack data, and it returns tuples.
Syntax:
struct.unpack_from(frmt, bfr, [ofst = 0])
Here,
- frmt: The format for the data type
- bfr: The writable buffer which starts at offset (optional)
Example:
import struct
# To create a string buffer, ctypes are required
import ctypes
# Thecalcsize() function is used to calculate the size of the format
siz=struct.calcsize('hhl')
print(siz)
# The buffer 'bfr' is created
bfr=ctypes.create_string_buffer(siz)
# The struct.pack() function packs the data
# The struct.unpack() function returns the data after unpacking
a=struct.pack('hhl', 4, 4, 5)
print( a)
b=struct.unpack('hhl', x)
print( b )
# The struct.pack_into() function is used topack data into the buffer,
#and it does not return any value
# The struct.unpack_from() function is used to unpack data from the
# thebuffer,and it returns the unpacked values in form of a tuple
struct.pack_into('hhl', bfr, 1, 3, 3, 4)
c=struct.unpack_from('hhl', bfr, 0)
print( c )
Output:
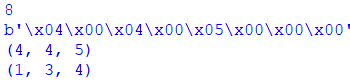