Screen Rotation app Using Tkinter in Python
Tkinter:
The standard Python technique for building Graphical User Interfaces (GUIs) is Tkinter, which is included in all popular Python distributions. The only framework included in the Python standard library is this one. On top of Tk, this Python framework serves as a thin object-oriented layer that provides access to the Tk toolkit. The Tk toolkit is a cross-platform set of "graphical control elements," also called widgets, used to build application interfaces. This framework gives Python users a quick and easy way to build GUI elements with the Tk toolkit's widgets. In a Python application, Tk widgets can create buttons, menus, data fields, etc.
Once created, these graphic elements can be connected to or work with other widgets, features, functionality, processes, or data.
Tkinter bridges the gap between Python's execution model and Tcl's, built around cooperative multitasking. Like Python, Tcl is an interpreted dynamic programming language. Although it is an overall programming language that can be used independently, it is most frequently used in C programs as a scripting motor or as an interaction with the Tk toolbox. The Tcl library has a C interface that enables users to add custom Tcl or C commands, run Tcl commands and scripts, and manage one or more Tcl interpreter instances. Each interpreter has a queue for events that can be used to send and process events.
Syntax:
from tkinter import *
from tkinter import ttk
Advantages of Tkinter:
This promotes flexibility in handling GUI incidents, which makes the code base run more smoothly. Qt is more than just a framework; it uses a variety of native platform APIs for networking, database development, and other uses. Through a unique API, it gives them direct access. Using a wide range of software and regular spot APIs for network, database design, and other functions, Qt is more than just a framework. Through a unique API, it gives them direct access. Different UI elements: Qt offers several widgets, including icons or menus, all created with a fundamental interface for all supported systems. Various sources for learning Among the most often used UI frameworks for Python is PyQt. Thus, it's simple to browse a variety of information.
Disadvantages of Tkinter:
Tkinter doesn't have any advanced widgets. There isn't a tool like Qt Designer for Tkinter in it. Its user interface is unreliable. Tkinter can be challenging to debug at times. It's not entirely in Python. Classes in PyQt5 lack Python-specific documentation. PyQt has a long learning curve since understanding every aspect requires much effort. Advanced widgets are not included in Tkinter. There isn't a tool like Qt Design for Tkinter in it. It lacks a natural feel and appearance.
CODE:
from tkinter import *
import rotatescreen
def Screen_rotation(temp):
screen = rotatescreen.get_primary_display()
if temp == "up":
screen.set_landscape()
elif temp == "right":
screen.set_portrait_flipped()
elif temp == "down":
screen.set_landscape_flipped()
elif temp == "left":
screen.set_portrait()
master = Tk()
master.geometry("100x100")
master.title("Screen Rotation")
master.configure(bg='light grey')
result = StringVar()
Button(master, text="Up", command=lambda: Screen_rotation(
"up"), bg="white").grid(row=0, column=3)
Button(master, text="Right", command=lambda: Screen_rotation(
"right"), bg="white").grid(row=1, column=6)
Button(master, text="Left", command=lambda: Screen_rotation(
"left"), bg="white").grid(row=1, column=2)
Button(master, text="Down", command=lambda: Screen_rotation(
"down"), bg="white").grid(row=3, column=3)
mainloop()
CODE EXPLANATION:
Import all the required modules which are necessary for screen rotation. Add user define function for rotating screen using if and elif statements. Creating tkinter object() with geometry() , title(), configuration() also add variable classes in tkinter().Now create buttons to change orientation using button() and use grid for position. We use primary display keyword to rotate the content by using the modules imported.
OUTPUT:
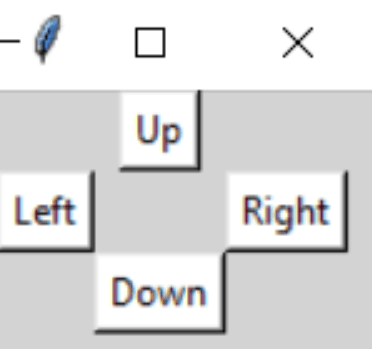