Amazon rekognition using python
Amazon recognition service is an AI service which is an image labelling API (Application programming interface). In this article, we shall learn to use the AWS python boto3 module to invoke Amazon Rekognition API to get the results out of the invocation.
We shall develop a python code which reads an example image using the AWS python boto3 module, which provides us with our required results, i.e., the labels in the picture.
In simple words, we can define Amazon recognition as an API that helps us to identify and differentiate various objects or people in a given image.
The Amazon Rekognition service web page (https://aws.amazon.com/rekognition/.) provides various features such as content moderation (detects inappropriate, potentially unsafe, unwanted content across images and videos), face compare and search (compares two faces in two pictures and determines the similarities in the faces), face detection and analysis (detects faces that appear in an image or video and can also recognize features such as specs, facial hair, open eyes, etc.), labels (detects all the objects in pictures and scenes in videos), custom labels (detects custom labels such as brand logos using machine learning algorithms), text detection (extracts distorted and skewed text from images and videos), streaming video events detection (detects objects, people, beings etc. from live streaming videos) etc.
Let us now see how to label a given image using Amazon Rekognition using python. First of all, create an S3 bucket in the Amazon Rekognition interface. Then, please select an image that you want to label and add it to the s3 bucket. Now, implement the following code
import boto3 # first import boto3 on your IDE
def detect_labels( photo , bucket ) :
client = boto3.client( ' rekognition ' )
response = client.detect_labels( Image = { ' S3Object ' : { ' Bucket ' : bucket , ' Name ' : photo } } , MaxLabel = 10 )
print( ' Detected labels for ' + photo )
print( )
for label in response[ ' Labels '] :
print( " Label : " + label[ ' Name '] )
print( " Confidence : " + str( label[ ' Confidence ' ] ) )
print( " Instances : " )
for instance in label[ ' Instances ' ] :
print( " Bounding box " )
print( " Top : " + str( instance[ ' BoundingBox ' ][ ' Top ' ] ) )
print( " Left : " + str( instance[ ' BoundingBox ' ][ ' Left ' ] ) )
print( " Width : " + str( instance[ ' BoundingBox ' ][ ' Width ' ] ) )
print( " Height : " + str( instance[ ' BoundingBox ' ][ ' Height ' ] ) )
print( " Confidence : " + str( instance[ ' BoundingBox ' ][ ' Confidence ' ] ) )
print( )
print( " Parents " )
for parent in label[ ' Parents ' ] :
print( " " + parent[ ' Name ' ] )
print( " - - - - - - - - - - - - - - - - - - " )
return len( response[ ' Labels ' ] )
def main() :
photo = ' img.jpg ' # provide the image address
bucket = ' amazonrekognition-cqpocs '
label_count = detect_labels( photo , bucket )
print( " Labels detected : " + str( label_count ) )
if __name__ == " __main__ " :
main()
After writing the code in your IDE, go to the console and type 'aws configure' to log in to your AWS account. Then, provide the details asked by your console. Then, run your code using local invocation. You will see that your console successfully returns an output providing each and every label in your image along with its confidence, instances, and parent information. Thus, you have successfully completed your Amazon rekognition using python for the identification of labels.
Example input:
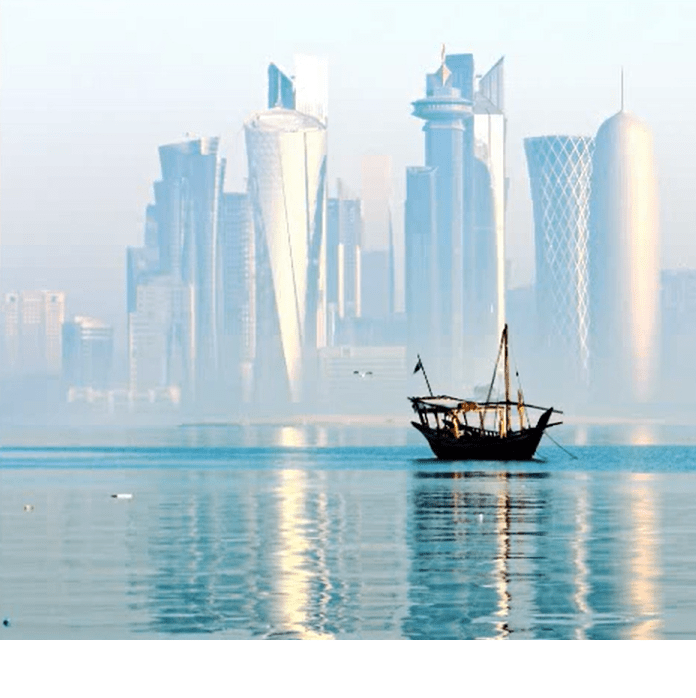
Output:
Label: Watercraft
Confidence: 99.46809387207031
Instances:
Parents: Vehicle
Transportation
--------------------------
Label: Vehicle
Confidence: 99.46809387207031
Instances:
Parents: Transportation
--------------------------
Label: Transportation
Confidence: 99.46809387207031
Instances:
Parents:
--------------------------
Label: Boat
Confidence: 98.43656158447266
Instances: Bounding box
Top: 0.38169410824775696
Left: 0.311278020095825
Width: 0.1282336711883545
Height: 0.1624620407819748
Confidence: 98.43656158447266
Parents:
Vehicle
Transportation
--------------------------
Label: Water
Confidence: 98.2005615234375
Instances:
Parents:
--------------------------
Label: City
Confidence: 82.61547088623047
Instances:
Parents:
Urban
--------------------------
Label: Urban
Confidence: 82.61547088623047
Instances:
Parents:
----------------------
Label: Office Building
Confidence: 81.8774642944336
Instances:
Parents:
-------------------------
Label: Back
Confidence: 81.69802856445312
Instances:
Parents:
--------------------------
Label: High Rise
Confidence: 77.49475860595703
Instances:
Parents:
Similarly, we can perform other recognitions also using the Amazon recognition interface using simple python codes.