Loan Calculator using PyQt5 in Python
In the following tutorial, we will learn how to build a Loan Calculator application using the PyQt5 library in the Python programming language.
So, let's get started.
Introduction to the code:
The heading label for the calculator's name is created, followed by a label and line edit pair for the interest rate. The label specifies what the user must type, while the line edit facilitates text entry. To make a pair for the sum and the year, in the same way, build a calculator push button, design a label that shows the expected monthly payment, and finally, create a label that shows the sum calculated. We started by importing all of the necessary modules into our software. We imported the QtWidgets, QtCore, QtGui, and sys files. The function Object() { [native code] } for initialising our function was then added to a new class that we had built. The window's title, width, and height are then set. We also established the geometry of the window. The widgets were then all displayed. Then, we developed a different function where we would create the heading and different labels as well as set their properties. The headline for the loan calculator was then set, along with its position, geometry, font characteristics, and colour effects. The further steps are included in code explanation part to give a brief on the code.
CODE:
from PyQt5.QtWidgets import *
from PyQt5 import QtCore, QtGui
from PyQt5.QtGui import *
from PyQt5.QtCore import *
import sys
class Window(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Python ")
self.w_width = 400
self.w_height = 500
self.setGeometry(100, 100, self.w_width, self.w_height)
self.UiComponents()
self.show()
def UiComponents(self):
head = QLabel("Loan Calculator", self)
head.setGeometry(0, 10, 400, 60)
font = QFont('Times', 15)
font.setBold(True)
font.setItalic(True)
font.setUnderline(True)
head.setFont(font)
head.setAlignment(Qt.AlignCenter)
color = QGraphicsColorizeEffect(self)
color.setColor(Qt.darkCyan)
head.setGraphicsEffect(color)
i_label = QLabel("Annual Interest", self)
i_label.setAlignment(Qt.AlignCenter)
i_label.setGeometry(20, 100, 170, 40)
i_label.setStyleSheet("QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}")
i_label.setFont(QFont('Times', 9))
self.rate = QLineEdit(self)
onlyInt = QIntValidator()
self.rate.setValidator(onlyInt)
self.rate.setGeometry(200, 100, 180, 40)
self.rate.setAlignment(Qt.AlignCenter)
self.rate.setFont(QFont('Times', 9))
n_label = QLabel("Years ", self)
n_label.setAlignment(Qt.AlignCenter)
n_label.setGeometry(20, 150, 170, 40)
n_label.setStyleSheet("QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}")
n_label.setFont(QFont('Times', 9))
self.years = QLineEdit(self)
onlyInt = QIntValidator()
self.years.setValidator(onlyInt)
self.years.setGeometry(200, 150, 180, 40)
self.years.setAlignment(Qt.AlignCenter)
self.years.setFont(QFont('Times', 9))
a_label = QLabel("Amount", self)
a_label.setAlignment(Qt.AlignCenter)
a_label.setGeometry(20, 200, 170, 40)
a_label.setStyleSheet("QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}")
a_label.setFont(QFont('Times', 9))
self.amount = QLineEdit(self)
onlyInt = QIntValidator()
self.amount.setValidator(onlyInt)
self.amount.setGeometry(200, 200, 180, 40)
self.amount.setAlignment(Qt.AlignCenter)
self.amount.setFont(QFont('Times', 9))
calculate = QPushButton("Compute Payment", self)
calculate.setGeometry(125, 270, 150, 40)
calculate.clicked.connect(self.calculate_action)
self.m_payment = QLabel(self)
self.m_payment.setAlignment(Qt.AlignCenter)
self.m_payment.setGeometry(50, 340, 300, 60)
self.m_payment.setStyleSheet("QLabel"
"{"
"border : 3px solid black;"
"background : white;"
"}")
self.m_payment.setFont(QFont('Arial', 11))
self.y_payment = QLabel(self)
self.y_payment.setAlignment(Qt.AlignCenter)
self.y_payment.setGeometry(50, 410, 300, 60)
self.y_payment.setStyleSheet("QLabel"
"{"
"border : 3px solid black;"
"background : white;"
"}")
self.y_payment.setFont(QFont('Arial', 11))
def calculate_action(self):
annualInterestRate = self.rate.text()
if len(annualInterestRate) == 0 or annualInterestRate == '0':
return
numberOfYears = self.years.text()
if len(numberOfYears) == 0 or numberOfYears == '0':
return
loanAmount = self.amount.text()
if len(loanAmount) == 0 or loanAmount == '0':
return
annualInterestRate = int(annualInterestRate)
numberOfYears = int(numberOfYears)
loanAmount = int(loanAmount)
monthlyInterestRate = annualInterestRate / 1200
monthlyPayment = loanAmount * monthlyInterestRate / (1 - 1 / (1 + monthlyInterestRate) ** (numberOfYears * 12))
monthlyPayment = "{:.2f}".format(monthlyPayment)
self.m_payment.setText("Monthly Payment : " + str(monthlyPayment))
totalPayment = float(monthlyPayment) * 12 * numberOfYears
totalPayment = "{:.2f}".format(totalPayment)
self.y_payment.setText("Total Payment : " + str(totalPayment))
App = QApplication(sys.argv)
window = Window()
sys.exit(App.exec())
Explanation of code:
Importing all the required modules to get processed, the constructor is used as init function, which is used to inherit the properties. After the function, we used to set the title for the window page, and the page dimensions are applied here, i.e., height and width, using geometry with the calling method. Showing all the widgets may look attractive for the page with a geometry head and using different fonts to set a different color to the head. Then after we create an interesting label by setting properties to the interesting label and then creating a QLineEdit object to get the interest, accepting only the number as input and setting properties to the rate line edit, we create several years label to ask the user for the loan years, interest, and total amount with the QLineEdit object to get the years accepting only number as input the setting properties to the rate line edit. PTO pushes a button to the user, which can conclude the final amount paid by monthly payment and total payment as output.
OUTPUT:
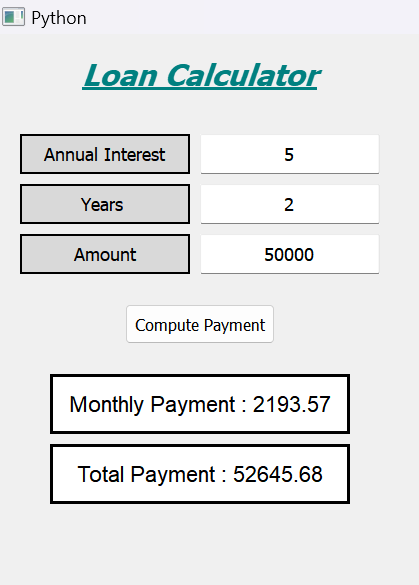