Ternary operators in python
Starting from Python version 2.5, ternary operators are also known as Conditional operators. Using these operators, we can evaluate any problem based on a condition. It is an alternative and sophisticated form of the if-else statements. Rather than using if-else statements that take multiple lines, we can check if a particular condition is true or false and evaluate based on the condition in a single line using these operators.
Syntax:
[code_if_true] if [condition] else [code_if_false]
Here,
- code_if_true: If the expression in the if [condition] is true, this code will be executed.
- condition: Expression based on which the code will be executed.
- code_if_false: If the expression in the if [condition] is false, this code will be executed.
Example:
Let us write a program to check the minimum number in the given two numbers:
a = int (input ("Enter the first number: "))
b = int (input ("Enter the second number: "))
min = a if (a < b) else b
print ("The minimum number in a and b is: ", min)
Output:
Enter the first number: 7
Enter the second number: 89
The minimum number in a and b is: 7
- Printing directly using Ternary Operator
We can place a print statement in the place of expression to be printed if the condition is true or false. This way, we can further decrease the length of the program as we need not to write another line for printing the result.
a = int (input ("Enter the first number: "))
b = int (input ("Enter the second number: "))
print (a, "is smaller") if (a < b) else print (b, "is smaller")
Output:
Enter the first number: 4
Enter the second number: 3
3 is smaller
- Using Tuples, dictionary, and lambda, we can simplify it. Let us take a simple example:
a = int (input ("Enter the first number: "))
b = int (input ("Enter the second number: "))
min1 = (b, a) [a < b]
print("Minimum value by using tuples: ", min1)
min2 = {True: a, False: b} [a < b]
print ("Minimum value by using a dictionary: ", min2)
min3 = (lambda: b, lambda: a) [a < b] ()
print ("Minimum value by using lambda: ", min3)
Output:
Enter the first number: 5
Enter the second number: 9
Minimum value by using tuples: 5
Minimum value by using a dictionary: 5
Minimum value by using lambda: 5
Understanding:
- Tuples:
In the line:
min1 = (b, a) [a < b]
The two possible outputs for true and false conditions must be placed in a tuple in the order: (False, True), and the condition to check is placed after the tuple in square braces.
We gave the input
a = 5
b = 9
The condition a is less than b is true; hence, the second value in the tuple is printed.
- Dictionary:
In the line:
min2 = {True: a, False : b} [a < b]
Dictionary is a collection of keys with corresponding values. Hence, we placed the possible outcomes of true and false conditions as values to True and False keys. Hence, when the condition becomes true, the value of the True key will be executed, and when the condition is false, the value of the False key will be executed. The condition to be checked should be written next.
We gave the input
a = 5
b = 9
The condition a is less than b is true; hence, the True key value: "a," which is equal to 5, is printed.
- Lambda function:
This method is more efficient than tuples and dictionaries because, here, we can be assured that only one expression will be executed.
In the line:
min3 = (lambda: b, lambda: a) [a < b] ()
We used two lambda functions with no arguments. Hence, according to the result of the expression, if a is less than b is true, then the second lambda function is executed; else, the first value will be printed. The condition is placed next.
We gave the input
a = 5
b = 9
The condition a is less than b is true; hence, the second lambda function is executed, and the value of a is printed.
Nested if-else:
We can write nested if-else statements in this format. Generally, writing nested if-else statements takes multiple lines, but using this format, we can make it in less number of lines.
Let us first take the normal nested if-else statement program to see the difference:
a = int (input ("Enter the first number: "))
b = int (input ("Enter the second number: "))
if a == b:
print ("a and b are equal==",a)
else:
if a > b:
print (a, " is greater than ", b)
else:
print (b, " is greater than", a)
Output:
Enter the first number: 4
Enter the second number: 4
a and b are equal== 4
- As you can observe in the above program, writing nested if-else took 7 lines. The same code in ternary form can be written in 2 lines:
a = int (input ("Enter the first number: "))
b = int (input ("Enter the second number: "))
print ("Equal" if a == b else (a, "is greater") if a > b else (b, "is greater"))
Output:
Enter the first number: 4
Enter the second number: 4
Equal
Understanding:
We need to place an if-else statement inside another if statement. Instead of using the traditional nested if-else statements, we used the ternary form. It took 1 line making the program simpler.
- Python Ternary operators are different from programming languages like C and C++.
- Ternary operators have the lowest priority in the operators’ hierarchy.
Short-hand Ternary:
Python also provides us with a way to make the code even smaller. This operator is the more shrunk version of the normal ternary operators.
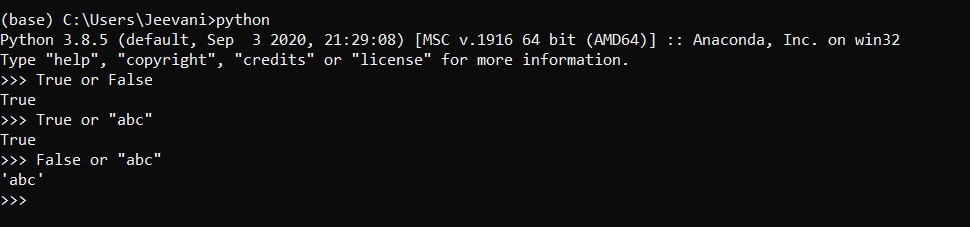
This method is useful when we need to quickly check the output of a function and display a message if the output is empty.
>>> Output = None
>>> Message = Output or "Output is empty"
>>> print (Message)
Output is empty