Difference between python list and tuple
In this tutorial, we will understand the key differences between python lists and tuples in python.
Firstly, let us see the similarities between Lists and Tuples. Both are two data types popular in python. They are used to accumulate one or more Python objects or data types serially. Both are capable of storing data such as integers, floats, strings, and dictionaries.
Now, let us understand the difference between both.
1. Difference in Representation
The Lists and tuples are represented differently.
The square bracket [] is taken into account to enclose a list, and elements therein are separated by a comma.
The parenthesis () is taken into account to enclose a tuple and elements therein are separated by the comma.
Let us see an example to understand this better.
Example:
# online python-3 complier (Interpreter)
# python program to mark the representational difference between list and tuple
list_1=[‘tutorials and example’, 15, 92, 984.70, {‘Name: “Python”}]
print(type(list_1))
tuple_1=(‘Tutorials and example’ ,95, 78, 31.9, [16, 82,n93])
print(type(tuple_1))
Output:
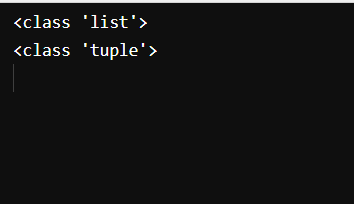
Explanation: In the above code, the representational difference between the data types – list and tuple are being addressed.
We can see that list named list_1 is represented using a square bracket and the tuple named tuple_1 is represented using parenthesis.
2. Mutability and Immutability in python
It is the most significant variance between list and tuple. The lists are mutable, and tuples are immutable. By the mutability of the list, we mean the objects in python can be modified after creation, whereas tuples once created can't be modified.
Example to show the mutable nature of the list.
# Python program to illustrate the mutable nature of lists in python
list1 = ["Anthropology", "Geography", "Hindi", "Polity", "History"]
print("list before modification: ")
print(list1)
list1[0] = "English"
print("list after modification: ")
print(list1)
Output:
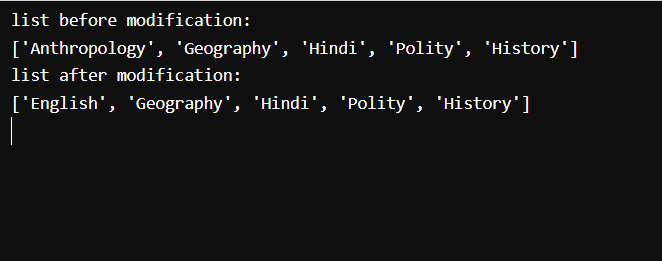
Explanation: The above code shows the mutable nature of the list. The first line of code shows the unmodified list. In the second line, we are trying to modify the 0th index of the list. We are able to successfully modify the list. The previous element ‘anthropology’ is being replaced with the word English. the modified list is shown. Here, we can modify the list.
Example to show the immutable nature of the tuple.
#Python program to illustrate the immutable nature of tuple in python
tuple1 = ("Anthropology", "Geography", "Hindi", "Polity", "History")
print("tuple before modification: ")
print(tuple1)
tuple1[0] = "English"
print("tuple after modification: ")
print(tuple1)
Output:
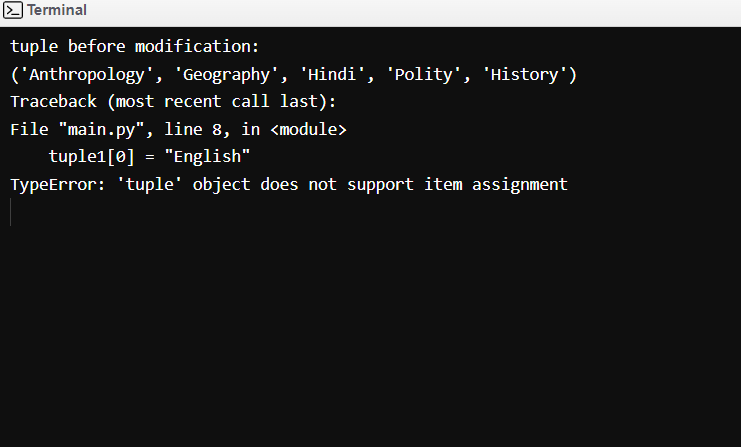
Explanation: The above code shows the immutable nature of the tuple. The first line of code shows the unmodified tuple. In the second line, we are trying to modify the 0th index of the list but failed. We are unable to modify the tuple. Hence, it proves that the tuple cannot be modified.
3. Debugging
It is easier to debug the tuples when working on a larger project as they are immutable in nature. If the size of the project is small or the number of data is less, then lists play a vital role. Now, let us understand this through an example.
#python program to illustrate the debugging in a list
lt1 = [236, 95,443, 83, 17, 890, 3211]
# Copying address of a in b
lt2 = lt1
lt1[6] = "Tutorial and example"
print(lt1)
Output:
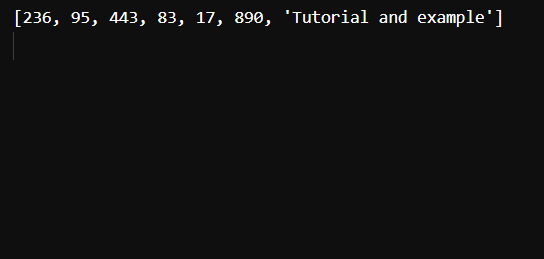
Explanation: In the above code, we tried to do lt2 = lt1; here we are not copying the list object from blt2 to lt1. The lt2 referred to the address of the list lt1. It means if we make the changes in the lt2 then it will also get reflected in list lt1, and it makes debugging easy. But it becomes tough for the substantial project where Python objects may have several references.
It will thus become very complicated to track those fluctuations in lists but it is not possible to change the immutable object tuples after it gets created.
Thus, it is easy to debug a tuple.
4. Supported functions
Fewer operations are supported in tuples than in the list. The inbuilt function named dir(object) is used to fetch all the supported functions for the lists and tuples.
List Functions
dir(list)
Output:
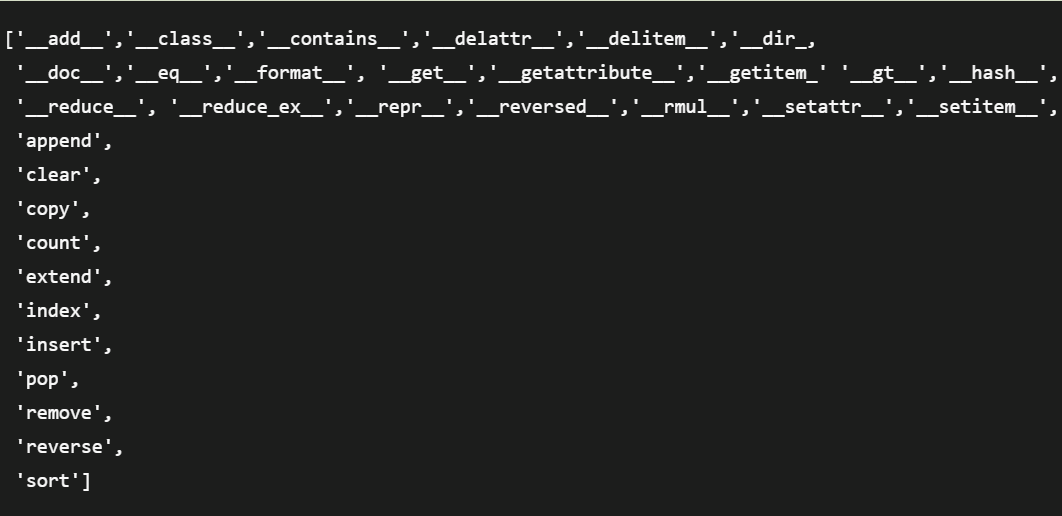
Tuple Functions
dir(tuple)
Output:
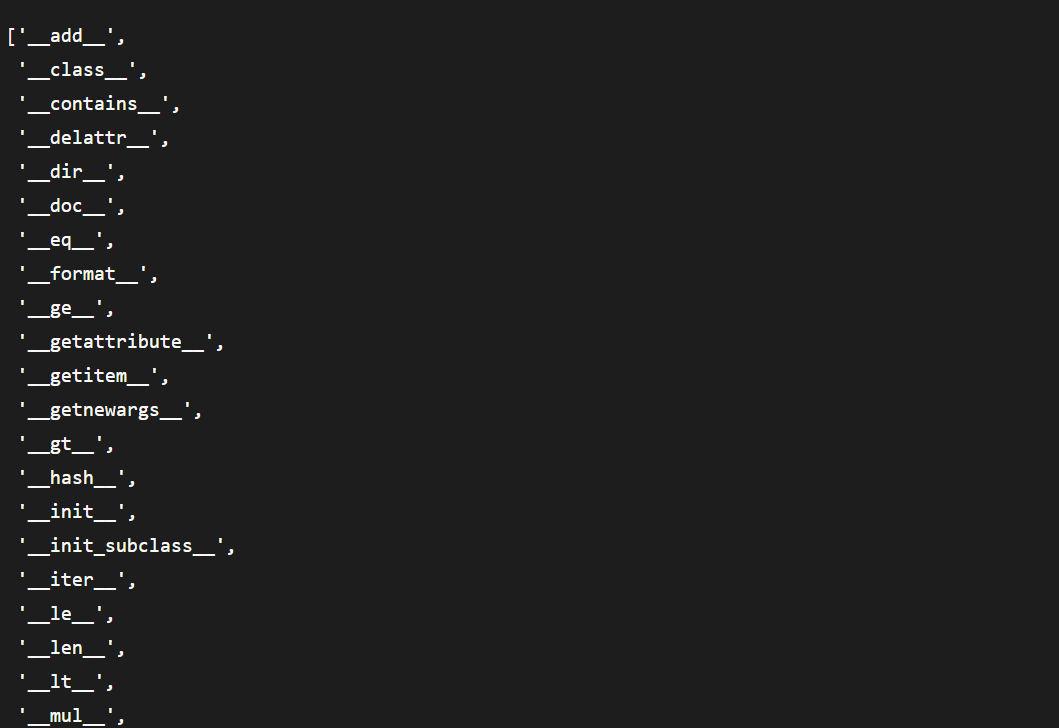
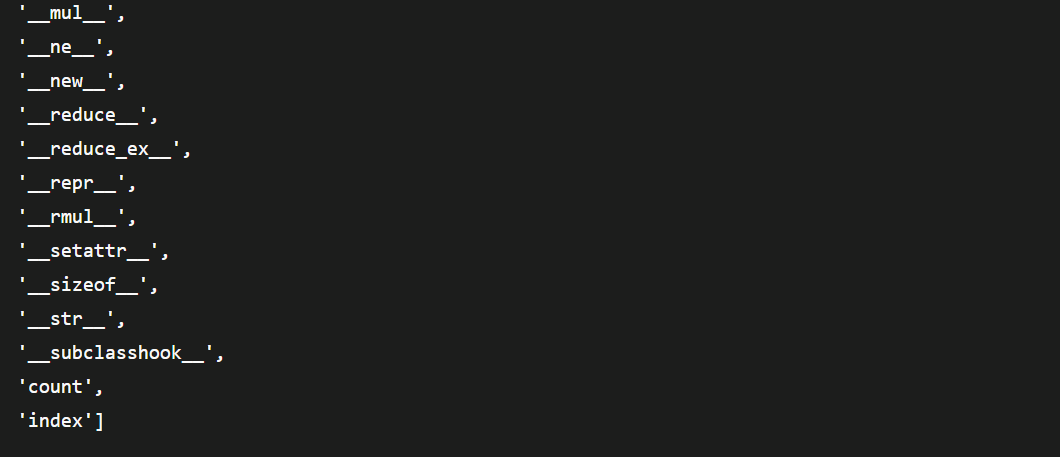
5. Efficiency in memory
The lists are less efficient in terms of memory than the tuple as the tuple has fewer built-in operations. Lists are appropriate for the smaller number of elements whereas tuples are a bit faster than the list for the enormous amount of data.
Example:
#python program to analyze the memory efficiency
Tuple = (145, 42, 32, 894, 65, 126, 897, 138, 909, 30, 7805, 1222, 815, 831, 8234,9208, 234, 56, 234, 535)
List = [11, 325, 245, 903, 14, 75, 76, 17, 88, 19, 40, 178, 84, 743, 832, 343, 555, 74, 941, 302, 723, 784, 74, 532 ]
print('The size of tuple =', Tuple.__sizeof__())
print('The size of list =', List.__sizeof__())
Output:
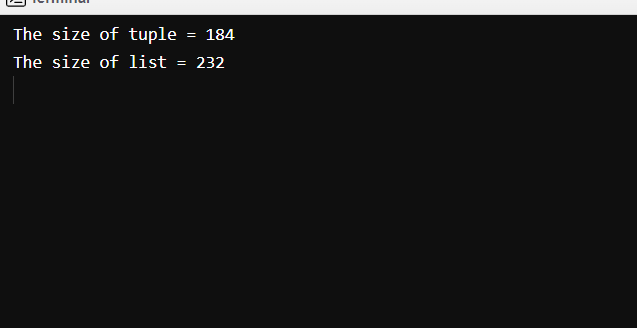
Difference Between Python List and Tuple
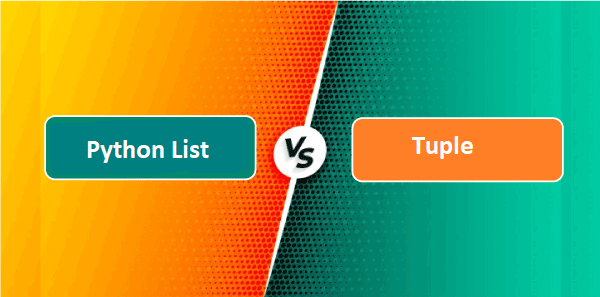
Parameter | List | Tuple |
Mutability | Mutable | Immutable |
speed | Slow | Fast |
Area of performance | Insertion and deletion | Accessing elements |
Memory | Consumes more memory | Consumes less memory |
Stored element | Capable of storing homogeneous as well as heterogeneous elements. | Capable of storing only heterogeneous elements. |
Built-in methods | Many built-in methods | Lesser in-built methods |
Representation | Square braces [] | Parenthesis () |