What is the re.sub() function in Python
There. sub () function is used to return a string by replacing the occurrences of a specific character or pattern with a replacement string. To use this function, import the re- module first. It is generally used to replace the substrings in strings. The inbuilt module of python named regex supports the sub () method for searching and replacing specified patterns. This can also be used to split a string or pattern into one or more substrings or sub-patterns.
A module can be imported by the following statement
import re
Not a single element; multiple elements can be replaced using this function by using a list.
The general syntax for using this function is:
re.sub(old, new, string, [count=x,flags=])
Where the terms can be described as:
- The first one, old, represents the string or pattern that needs to be replaced.
- The second one, new, represents a string or pattern with which the old must be replaced.
- The third term, string, denotes a string upon which the function or operation is to be executed (target string).
- The fourth term, count, represents the count of replacements that must occur.
- The fifth term, flags, is to shorten the code whose function is quite similar to that of a split operation.
After calling the function, it replaces a string by replacing the corresponding old one with a new pattern. If the one to be replaced(old) has not been found the string remains unchanged.
Function | Details |
re.sub (old, new, string) | All the occurrences of ‘old’ will be replaced by ‘new’. |
re.sub (old, new, string, count=1) | Only the first occurrence of ‘old’ be replaced by ‘new’ in the corresponding ‘string’. |
re.sub (old, new, string, count=x) | The first x occurrences of ‘old’ will be replaced by ‘new’. |
Example 1: To replace all the white spaces in the string “India is one among the countries in the world which has powerful Intelligence agency" with "^".
import re
str= “India is one among the countries in the world which has powerful Intelligence agency”
new_str=re.sub(r “\s”, “^”,str)
print(new)
Output:
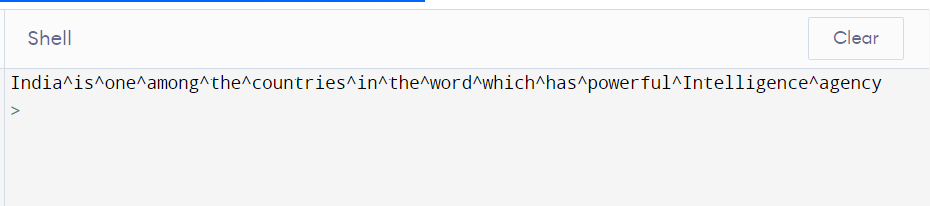
Here, we observe that all the occurrences of white spaces are replaced by "^", irrespective of the count of the number of white spaces.
Example 2: Replace the first occurrence of white space with "^" in the same above string.
import re
str= “India is one among the countries in the word which has powerful Intelligence agency”
new_str= re.sub(r “\s”, “^”,str, count=1)
print(new_str)
Output:
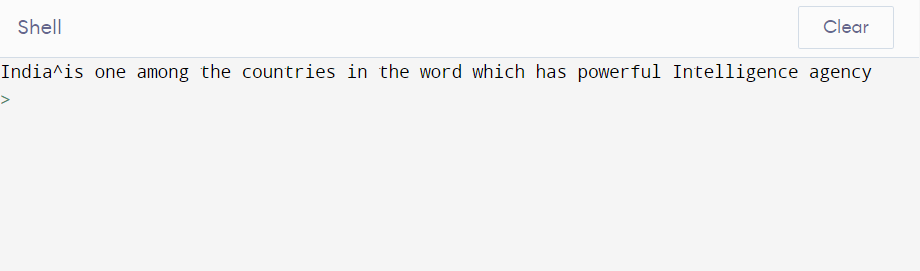
You can observe that only the first occurrence of white space is replaced by “^,” and other occurrences remain the same.
Example 3: Replace the first three occurrences of white spaces with “^” in the same string mentioned above.
import re
str= “India is one among the countries in the word which has powerful Intelligence agency”
new_str=re.sub(r “\s”, “^”,str,count=3)
print(new_str)
Output
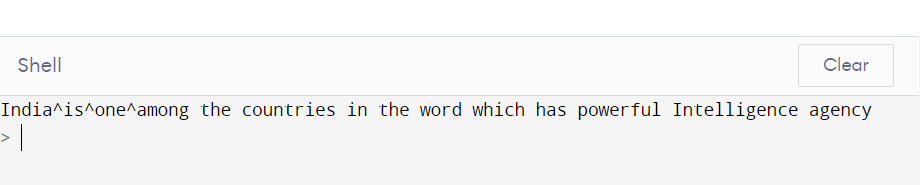
Here, you can observe that only the first three occurrences of white space are replaced by the specified character.
Example 4: Replace the occurrences of “r” using the re.sub() function with “l” in the string “ragging”.
import re
string= “chugging”
new_str=re.sub(“r”, “l”, string)
print(new_str)
Output:

Since there are no occurrences of “r,” we observe that there is no change in the corresponding string (String remained unchanged).
A character set or number set can also be replaced by a single specified character.
Example: Replace all the lower-case letters in a string “Financial Year Is From 1 April to 31 March Of Its Successive Year” by “0”
import re
str= “Financial Year Is From 1 April to 31 March Of Its Successive Year”
new_str=re.sub(r “[a-z]”, “0”, str)
print(new_str)
Output:

Here all the lower cases [a-z] got replaced by the specified character “0”.
We can also replace lower- and upper-case letters in a single shot or program using the parameter “flags.”
Example: Replacing all the lower case and upper-case letters in the above same string with “0” will be as follows
import re
str= “Financial Year Is From 1 April to 31 March Of Its Successive Year”
new=re.sub(r “[a-z]”, “0”, str, flags=re.I)
print(new)
Output

You can observe that all the characters, whether the lowercase or upper case, are replaced by the specified character “0” in the corresponding string.