os.stat() method in Python
In Python, the os module provides the capacity to interact with the operating system. The operating system comes under the Python module. In this module, Python provides a specific feature that depends on the Operating System. This module's main feature is interacting with the file and path directory. In this article, we are going to learn about os.path.stat features of Python. So, before understanding what Python's stat() module is, we need to understand a bit about the directory tree.
What is the directory tree?
The files in the UNIX system are organized similarly to in a tree data structure, and that's why it is known as a directory tree. At the very top of the file system, there is a directory called "root," which is represented by "/," and all other files come under this.
The os.stat() method
In Python, the os.stat() method provides the feature to make a system call for the specific path. With the help of this method, we can get the status of the specific path.
Syntax for os.stat() method
We can implement the os.stat() method with the help of the following syntax.
os.stat(path)
Parameter for os.stat() method
There are some parameters that are used in the os.stat() method. These parameters are as follows:
- Path: It represents the valid path for the file.
- Return type: With this return type's help, the program returns stat_result for the object class. The os.stat_results shows the status for the specific path. There are some attributes that are provided by the stat_result.
- St_mode: It shows the permission and type of the file.
- St_ino: It shows the file index number on the window.
- St_dev: It shows the identifier number of the device in which the file is stored.
- St_nlink: It shows the number of hard links present in it.
- St_uid: It shows the user identification number of the user file.
- St_gid: It shows the group identification number of the user file.
- St_size: It shows the size of the file in bytes.
- St_atime: It shows the most access time of the file in seconds.
- St_mtime: It shows the most file modification time of the file in seconds.
- St_ctime: It shows the most recent time of metadata change of the file UNIX and file creation time on the window in seconds.
- St_atime_ns: The function of st_atime_ns is similar to st_atime, but it displays all the information in nanoseconds.
- st_mtime_ns: The function of st_mtime_ns is similar to st_atime, but it displays all the information in nanoseconds.
- st_ctime_ns: The function of st_ctime_ns is similar to st_atime, but it displays all the information in nanoseconds.
- St_blocks: It shows the number of allocations to the file.
- St_rdev: It shows the type of the device if the device type is an inode device.
- St_flags: It shows the user define flag for system files.
Example 1:
# Python program to explain os.stat() method
# importing os module
import os
# path
path = '/home/User/Documents/demo.txt'
# Get the status of
# the specified path
status = os.stat(path)
# Print the status
# of the specified path
print(status)
Output:

Example 2:
# !/usr/bin/python
import os, sys
# showing stat information of file "a2.py"
statinfo = os.stat('a2.py')
print statinfo
Output:
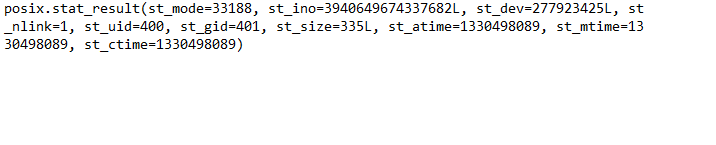
Example 3:
import stat
import os, time
st = os.stat("samples/sample.txt")
print "mode", "=>", oct(stat.S_IMODE(st[stat.ST_MODE]))
print "type", "=>",
if stat.S_ISDIR(st[stat.ST_MODE]):
print "DIRECTORY",
if stat.S_ISREG(st[stat.ST_MODE]):
print "REGULAR",
if stat.S_ISLNK(st[stat.ST_MODE]):
print "LINK",
print
print "size", "=>", st[stat.ST_SIZE]
print "last accessed", "=>", time.ctime(st[stat.ST_ATIME])
print "last modified", "=>", time.ctime(st[stat.ST_MTIME])
print "inode changed", "=>", time.ctime(st[stat.ST_CTIME])
Output:
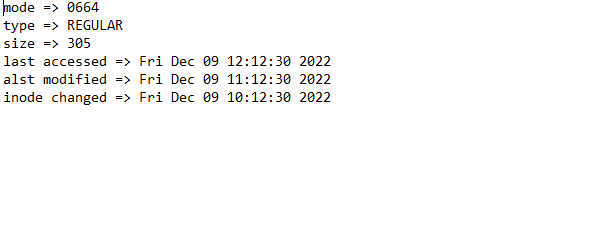
Example 4:
import os
import sys
path ="/home/File.txt"
fd = os.open(path, os.O_RDWR|os.O_CREAT)
os.close(fd)
file_info = os.stat(path)
print ("The device ID is:", file_info[2])
print ("The user ID is:", file_info[4])
Output:
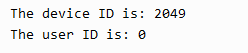
Explanation:
The information returned by the os.stat() method is in the form of an array, starting from the index number 0. Some elements of this method can be retrieved by specifying the index number.