How to write a program in python?
How to write a program in python?
In python, writing programs is not a big deal. What we need to work on is our logic and some basic rules of this language.
So, let’s start with some examples that will help us to understand the fundamentals of this language.
The following code shows how we can print Hello World in Python.
print("Hello World")
INPUT-

OUTPUT-

In the next program, we will see how we can input functions and ask the user to provide us a value.
Check out the program for the same.
a=input(“Enter age: ”) print(“The age is {}”.format(a))
INPUT-

OUTPUT-

Now we will look at some more examples where the input() function is incorporated with arithmetic operators.
The first program shows how we can calculate simple interest in Python
p=int(input("Enter the principal: ")) r=int(input("Enter the rate: ")) t=int(input("Enter the time: ")) si=p*r*t/100 print("The value for simple interest is {}".format(si))
INPUT-

OUTPUT-
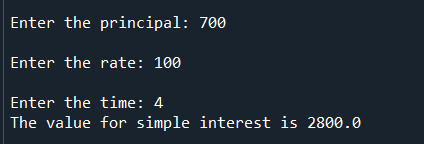
On executing the given program, we will be asked to enter the values of p,r, and t and then the expected result would be displayed.
In the next program, we will use the pow() and calculate the volume of a cylinder.
The following program shows how we can calculate the volume of a cylinder.
pi=3.14 r=int(input("Enter the value of radius: ")) h=int(input("Enter the value of height: ")) vol=pi*pow(r,2)*h print("Volume of cylinder is {}".format(vol))
INPUT-
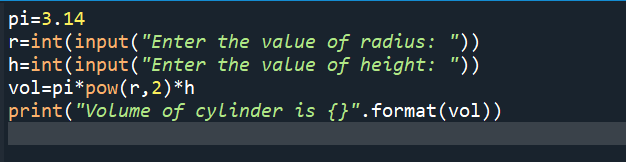
OUTPUT-
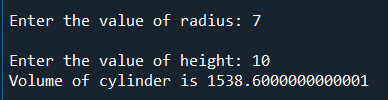
On executing the given program, we will be asked to enter the values of r and h and then the expected result would be displayed.
In the next program, we will see how basic arithmetic operators work in Python.
The following program shows how we can perform addition, subtraction, division, and multiplication.
num1=float(input("Enter number1: ")) num2=float(input("Enter number2: ")) add=num1+num2 subt=num1-num2 mult=num1*num2 div=num1/num2 print("Addition of two float numbers is {}".format(add)) print("Subtraction of two float numbers is {}".format(subt)) print("Multiplication of two float numbers is {}".format(mult)) print("Division of two float numbers is {}".format(div))
INPUT-
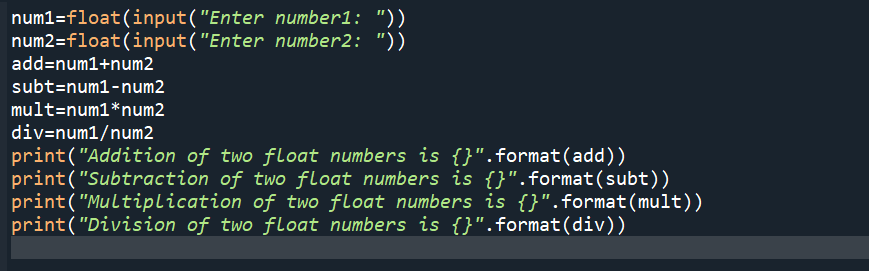
OUTPUT-
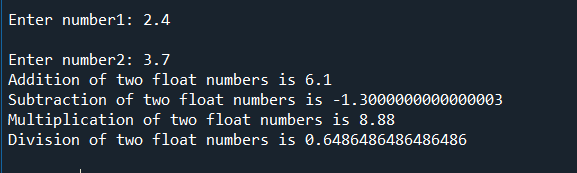
On executing the given program, we will be asked to enter the values of num1 and num2 and then the expected result would be displayed.
In the next program, we will calculate the area of a circle, we'll pass the parameters r and h and then we will obtain the value of the area in the output.
The following program illustrates the same-
pi=3.14 r=int(input("Enter the value of radius: ")) area=pi*r*r print("Area of circle is {}".format(area))
INPUT-

OUTPUT-
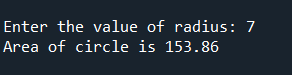
In the program given below, we'll use decision-making statements and find out the greatest among the three numbers.
The following program illustrates the same-
a=int(input("Enter the value of 1st number: ")) b=int(input("Enter the value of 2nd number: ")) c=int(input("Enter the value of 3rd number: ")) if(a>b and a>c): print("{} is greatest".format(a)) elif(b>a and b>c): print("{} is greatest".format(b)) else: print("{} is greatest".format(c))
INPUT-

OUTPUT-
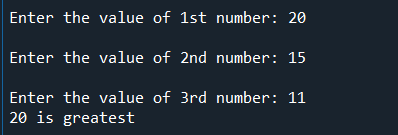
On executing the given program, we will be asked to enter the values of a,b, and c, and then the expected result would be displayed based on the comparison of values the objects hold.
In the next program, we will see what will be the output when strings and numbers are added.
The following program illustrates the same-
x=input("Enter the first number: ") y=input("Enter the second number: ") print(x+y) #result is the addition of two strings x=int(input("Enter the first number: ")) y=int(input("Enter the second number: ")) print(x+y) #result is addition of two numbers
INPUT-
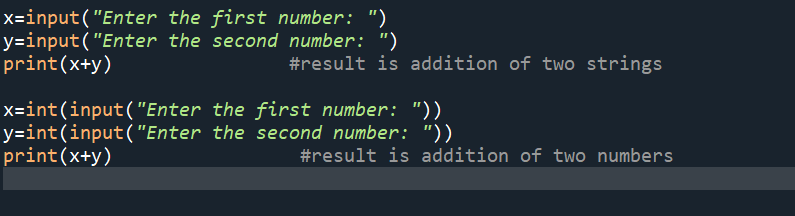
OUTPUT-
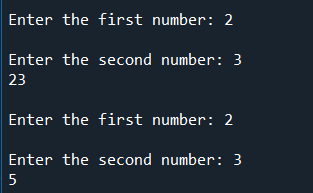
The program which is given below shows how different mathematical operators can be used.
Here we will calculate the distance between two points.
The following program shows the same-
x1=int(input("Enter the x-coordinate of first point: ")) y1=int(input("Enter the y-coordinate of first point: ")) x2=int(input("Enter the x-coordinate of second point: ")) y2=int(input("Enter the x-coordinate of second point: ")) dist=((x2-x1)**2+(y2-y1)**2)**0.5 print("The calculated distance is {}".format(dist))
INPUT-

OUTPUT-

On executing the given program, we will be asked to enter the values of x1,y1, and x2,y2, and then the expected result would be displayed after dist is computed.
In the next program we will look at simple examples related to creating functions in Python.
The first example shows how we can add two numbers with the help of functions.
Here we have defined the function and then called it.
Following program illustrates the same-
def sum(x,y): res=x+y print("Sum of {} and {} is {}".format(x,y,res)) x=int(input("Enter the value of x: ")) y=int(input("Enter the value of y: ")) sum(x,y)
INPUT-
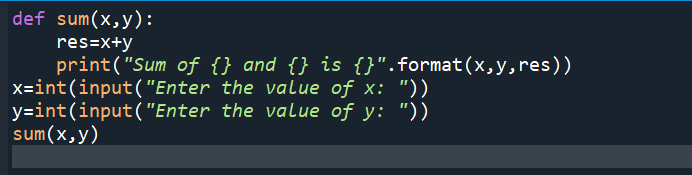
OUTPUT-

The last program shows how we can use lambda while defining functions in Python.
Lambda functions help us to reduce the size of the program. Let us see how it works-
The following program shows how to find a cube of a number
cube=lambda x:x*x*x print("Cube is {}".format(cube(9)))
INPUT-

OUTPUT-

In this article, we have seen how we can write programs in Python.