How to Write a Configuration file in Python
This article will discuss How to write a configuration file in python, why we need config files in Python, the format of the configuration file, file extensions, and how to make a good configuration file in python, etc. So before starting, we should know what a configuration file in Python is.
Configuration File in Python
Config files have a wide range of applications primarily used by operating systems to customize an environment. For programmers or developers, configuring initial parameters for software without hard coding data into the program is one of the most frequent use cases. It enables you to modify such settings without accessing the program's source code.
In other words, the software can be instructed to go to a configuration file for the precise information it needs for those variables that may vary over time, such as IP addresses, host names, user names, passwords, and database names.
Writing a separate file with Python code is the simplest way to create configuration files. You may give it a name like databaseconfig.py. Then, to prevent unintentionally uploading it, you might add the line *config.py to your .gitignore file.
Why do we need config Files in Python?
One need not even touch the code to update or save settings because the Python program has a configuration file that can be used to read setting values. Key-value pairs or other configurable information is stored in configuration files so that they can be read or retrieved by the code at a later time.
Developers don't have to worry about altering the code if the configuration changes; they can simply make the change in the configuration file instead of having to recompile and deploy the code. Additionally, configuration files make your settings and code more reusable and keep the settings data organized and separated.
It is best to keep important data more securely stored in cloud vaults, such as passwords, secrets, and certifications. However, the application's default settings may be in a configuration file.
Format of the Configuration File
YAML, JSON, TOML, and INI are the popular and standardized formats of configuration files.
Most Programs in python and libraries readily accept YAML and INI. INI has only 1 hierarchical level, making it the simplest solution. INI, on the other hand, has no data types; all is encoded as a string.
Firstly choose any format in these, followed by documentation of that decision. It's time to parse the configuration now that the simple phase has been completed.
File Ending Extensions of these Formats
Extension extensions give the user and system a clue about a file's contents. Reasonable configuration file ends included are:
- config.py extension is for the Python files.
- .yaml / .yml extension is for if the configuration is done in the YAML format.
- .json extension is for the configuration files written in JSON format.
- .ini extension file is a particular file that stores configuration data in a straightforward, preset format.
- .CFG or A configuration file with the extension "CONFIG" is used by several programs to hold settings unique to their software. While some configuration files are saved as plain text files, others may be in a program-specific format.
- ~/.[my app name] extension On Linux systems, the naming convention for configuration files is "RC." RC is "run common" and is an ancient computer system.
How to Make a Good Configuration File
Following these guidelines will help you create a good configuration file given below:
- It should be written in text and have an understandable structure. Anyone who can read should be able to code.
- Developers won't have been the only ones reading configuration files. It is necessary when non-developers attempt to know the procedure and alter the software's behavior. The config file becomes more expressive as a result of the ability to explain specific concepts through the use of comments.
- All operating systems and applications should be capable of accepting configuration files. Additionally, it must be simple to ship to the server using a CDaaS pipeline.
Create a config File in Python
Configparser.py is a Python module that makes it simple to write, read, and modify .ini files.
To install this module, you have to open your command prompt and write the given command:
pip install configparser
The primary purpose of Configureparser is to read and write .ini files, although it also accepts dictionary and iterable file objects as inputs. Each .ini file is divided into parts that include several key-value pairs.
Steps to create a config file
Let’s discuss how to create a config file in Python. By following the steps given below, we can create a config file:
- First, import the configparser module.
- Create an object of configparser.ConfigParser() store it in a variable.
- Create sections according to need using .add_section("section_name") method.
- Add key-value pairs to section, using .set("section","key","value") method.
- To save config :
with open (path/"file_name.ini", "w") as fileName:
config object.write(fileName)
Let’s understand How to create a configuration file in Python by taking an example given below:
Example
# Code goes from here
# importing config parser
import configparser
# creating object of configparser
config = config parser.ConfigParser()
# creating a section
config.add_section("database")
# adding key-value pairs
config.set("database", "host", "local host")
config.set("database", "admin", "local admin")
config.set("database", "password", "qwerty@123")
config.set("database", "port no", "2253")
config.set("database", "database", "SQL")
config.set("database", "version", "1.1.0")
# creating another section
config.add_section("user")
# adding key-value pairs
config.set("user", "name", "test_name")
config.set("user", "e-mail", "[email protected]")
config.set("user", "id", "4759422")
with open("example.ini", 'w') as example:
config.write(example)
Output:
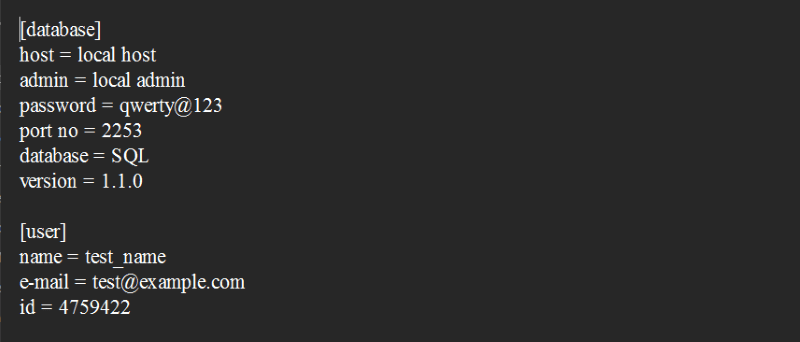
In the above example, to create an INI configuration file, we need to import configparser.
First, we have created an object of config parser using config parser.ConfigParser() method. To make sections in INI file we used .add_section(“section name”) method.
To fill values in section we have .set() method. It takes 3 parameters first is section name, the second is key or option name, and the third is value.
After filing sections, we need to create a file of .ini. To create a file, we use “with open()(). In these round brackets, we give the file's name so it will create a file in the same directory. To write value, we will use the .write() method.
Read a config File in Python.
We have learned how to construct and update the configuration file in Python .now that it has been created, in our main class, let's read the values from the configuration file. The easiest and clearest part comes next.
Every config is kept as a string because the Configureparser does not attempt to guess the data types in the config file. However, it offers a few ways to change a string's data type to the appropriate one. The most intriguing type is Boolean since it can distinguish between Boolean values such as "yes" and "no," "on" and "off," "true" and "false," and "1" and "0."
It could also read from an iterable file object, a dictionary, or a string using read_dict(), read_string(), or read_file ().
Let’s understand How to read configuration files in Python by taking an example given
below:
Example
# Code goes from here
# importing config parser
import configparser
# creating the object of configparser
config_data = configparser.ConfigParser()
# reading data
config_data.read("example.ini")
# app configuration data
database = config_data["database"]
# admin data
user = config_data["user"]
print("database data")
for database_data in database:
print(f"{database_data} = {database.get(database_data)}")
print("\nuser data")
for user_data in user:
print(f"{user_data} = {user.get(user_data)}")
Output:
database data
host = local host
admin = local admin
password = qwerty@123
port no = 2253
database = SQL
version = 1.1.0
user data
name = test_name
e-mail = [email protected]
id = 4759422
In the above example, we have a configuration file “example.ini”. Let’s try to read it. To read an INI file, we need to import the configparser.py module. Now we made an object of config parser using config parser.ConfigParser() method. At this time, this object does not have any data. We can read an out-source file using .read.
We have 2 sections in the ini file. One is the database section, and another is the user section. Let’s store these sections in different variable names as database and users.
Now by using for loop, we iterate in these sections and print these values to check if we get what we want.
YAML
YAML = “Yet Another Markup Language.”
A JSON extension called YAML was created to be simpler to write by hand. It does this in part because of the lengthy specification. Some data types, including string, integer, double, boolean, list, dictionary, etc., are natively encoded in YAML. Installing a third-party package will be necessary for Python to parse this. PyYAML.
To install this module, you have to open your command prompt and write the given command:
pip install pyyaml
The built-in Python data types that the YAML parser also returns can be sent to configuration from dict.
Example
database:
host: local host
admin: local admin
password: qwerty@123
port no: 2253
database: SQL
version : 1.1.0
user:
name : test_name
e-mail: [email protected]
id: 4759422
How to Read YAML File in Python
Let’s understand how to read the YAML file in Python by the example given below:
Example
# importing pyyaml module
import yaml
# to read a file
with open("exampleYaml.yaml", 'r') as file:
my_file = yaml.safe_load(file)
database = my_file["database"]
user = my_file["user"]
print("database data")
for database_data in database:
print(f"{database_data} = {database.get(database_data)}")
print("\nuser data")
for user_data in user:
print(f"{user_data} = {user.get(user_data)}")
Output:
database data
host = local host
admin = local admin
password = qwerty@123
port no = 2253
database = SQL
version = 1.1.0
user data
name = test_name
e-mail = [email protected]
id = 4759422
We have a YAML file “exampleYaml.yaml” in the above example. Let’s try to read it. To read a YMAL file, we need to import the YAML module. It is advised to use yaml.safe_load() rather than yaml.load() due to security concerns to prevent code injection in the configuration file.
We have 2 sections in the yaml file. One is the database section, and another is the user section. Let’s store these sections in different variable names as database and users.
Now by using for loop, we iterate in these sections and print these values to check if we get what we want. By following this way, we can read the YAML file.
JSON
JSON = “JavaScript Object Notation”
A format similar to JavaScript is called JSON.
Although JSON is quite popular and is very similar to YAML, comments cannot be added to JSON files.
The json module is used by the parsing logic to convert the JSON into Python's built-in data structures (dictionaries, lists, and strings). The dictionary is then used to generate the class. It also supports nested structures.
Example
{
"database":{
"host": "local host",
"admin": "local admin",
"password": "qwerty@123",
"port no": 2253,
"database": "SQL",
"version": "1.1.0"
},
"user":{
"name": "test_name",
"e-mail": "[email protected]",
"id": 4759422
}
}
How to Read JSON File in Python
Let’s understand how to read the JSON file in Python by the example given below:
Example
# importing json module
import json
with open("exampleJSON.json") as file:
my_file = json.load(file)
database = my_file["database"]
user = my_file["user"]
print("database data")
for database_data in database:
print(f"{database_data} = {database.get(database_data)}")
print("\nuser data")
for user_data in user:
print(f"{user_data} = {user.get(user_data)}")
Output:
database data
host = local host
admin = local admin
password = qwerty@123
port no = 2253
database = SQL
version = 1.1.0
user data
name = test_name
e-mail = [email protected]
id = 4759422
In the above example, we have a JSON file “exampleJSON.json”. To read it, we need to import the JSON module. We use json.load() to prevent code injection in the configuration file.
We have 2 sections in the json file. One is the database section, and another is the user section. Let’s store these sections in different variable names as database and users.
Now by using for loop, we iterate in these sections and print these values to check if we get what we want. By following this way, we can read the JSON file.
TOML
TOML = “Tom's Own Markup Language.”
The goal of TOML is to offer a simpler alternative to YAML.
We need to install a package to parse TOML. The most well-known one is simply referred to as toml.
It supports additional data types and has a set syntax for nested structures. Python package managers like pip and poetry frequently use it. But YAML is a better option if the configuration file has a lot of nested structures.
Example
[database]
host = "local host"
admin = "local admin"
password = "qwerty@123"
"port no" = 2253
database = "SQL"
version = "1.1.0"
[user]
name = "test_name"
e-mail = "[email protected]"
id = 4759422
How to Read TOML File in Python
# importing toml module
import toml
with open("exampleTOML.toml") as file:
my_file = toml.load(file)
database = my_file["database"]
user = my_file["user"]
print("database data")
for database_data in database:
print(f"{database_data} = {database.get(database_data)}")
print("\nuser data")
for user_data in user:
print(f"{user_data} = {user.get(user_data)}")
Output:
database data
host = local host
admin = local admin
password = qwerty@123
port no = 2253
database = SQL
version = 1.1.0
user data
name = test_name
e-mail = [email protected]
id = 4759422
We need to import the TOML module. In the above example, we have a TOMLfile “exampleTOML.TOML” file. In this, we use toml.load() to prevent code injection in the configuration file.
We have 2 sections in the toml file. One is the database section, and another is the user section. Let’s store these sections in different variable names as database and users.
Now by using for loop, we iterate in these sections and print these values to check if we get what we want. By following this way, we can read the TOMLfile.
INI
Using the included configparser module, Python can parse it. It is possible to provide the parser directly to configuration from dict because it functions like a dict-like object.
In addition to supporting dictionary and iterable file objects as inputs, Configureparser is generally used for reading and writing INI files.
There are numerous key-value pairs in each of the sections that make up an INI file.
Example
[database]
host = local host
admin = local admin
password = qwerty@123
port no = 2253
database = SQL
version = 1.1.0
[user]
name = test_name
e-mail = [email protected]
id = 4759422
Advantages
Let’s understand the advantages of making a config file in Python by following the points given below:-
- We don't have to code private data into a file hard that everybody may access.
- No matter how often we use the parameters in our code, changing their values is as easy as editing the configuration file.
- Suppose we want to publish our program on a repository like GitHub. In that case, we can hide the configuration file in the .gitignore file to ensure that no one can access our confidential data, and we can then add a readme file to show others how to make a new configuration file with their data.
Conclusion
This tutorial covered the Why, What, and How to create and read config files in Python. A comprehensive framework isn't necessarily preferable to a simple package, depending on the use case. Whatever option we select, we should always consider its readability, maintainability, and ability to detect errors as early as possible. Config files are simply another sort of code.