PyPi TensorFlow
It is a free open-source library for high-performative numerical computations. The architecture of TensorFlow allows us for easy deployment and computing across a varied number of platforms and from desktops to clusters servers of mobile and edge devices.
It is developed by the Google Brain team of engineers and researchers from Google's AI organization. It comes along with strong support for deep learning, machine learning, and varied numerical computation is used across many domains.
Features
- It has features that define, optimize and calculate mathematical expressions using multi-dimensional arrays called tensors.
- It includes good support for the development of machine learning and deep learning neural networks.
- It has high scalable features for computation with various datasets.
- It uses GPU computing automating management and a unique feature for optimization of memory and dataset.
Installation of TensorFlow
"python" is mandatory to install TensorFlow in our system.
In the windows operating system, follow the below steps to install TensorFlow.
Step 1: python version presented in the system should be verified.
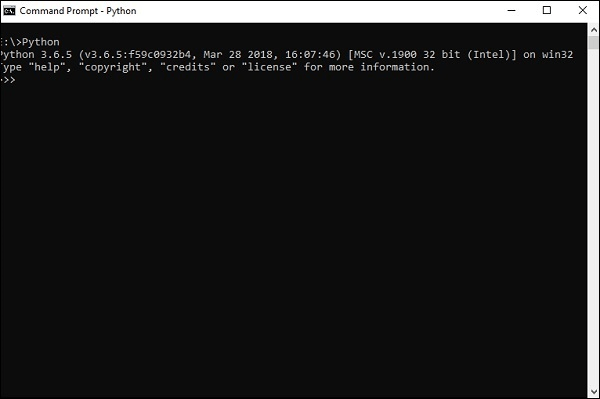
Step 2: Any mechanism can be used to install TensorFlow but it is advised to use "Anaconda" and "pip". The command "pip" is used to install modules in python.
Anaconda framework should be installed previously to TensorFlow.
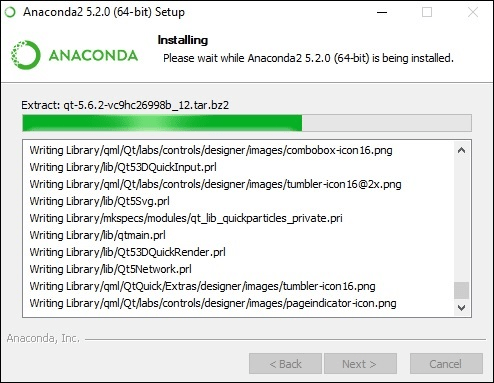
After installation, check with the "conda" command. The command execution is displayed as:
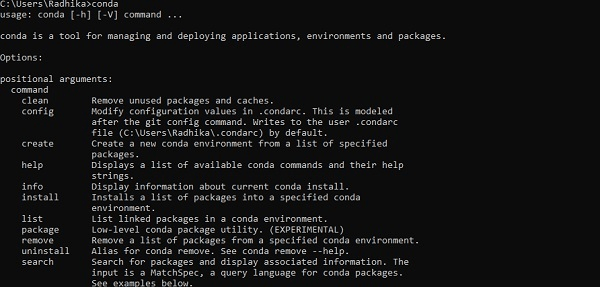
Step 3: To install TensorFlow execute the below command.
conda create --name tensorflow python = 3.5
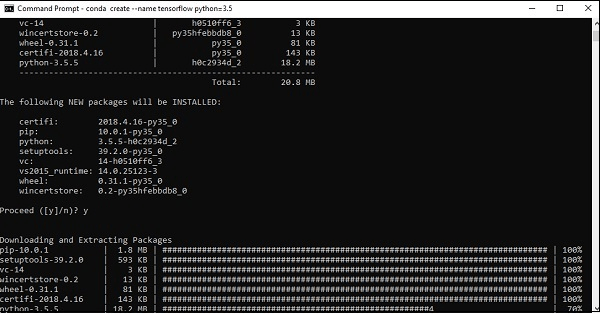
It downloads all the necessary packages for the setup
Step 4: After successful set up of the environment we should activate TensorFlow.
activate tensorflow

Step 5: We use pip for installing TensorFlow in the system.
pip install tensorflow

TensorFlow
TensorFlow is a basic software library for numerical computing using dataflow graphs where,
- Node: It represents mathematical operations.
- Edge: It represents a multi-dimensional array called Tensors.
Note: A tensor is a central unit of data in TensorFlow
Consider the below diagram:

Here, mul is a node that represents multiplication operation. Here, a and b are called as input (or) incoming tensors, and c is the resulting (or) output tensor.
The flexible architecture of tensors allows us to compute on more than one CPU or GPU in a system or server or a mobile application with a single API.
APIs in TensorFlow
TensorFlow provides us with many APIs (Application Programming Interface). But they can be classified majorly into two categories:
- Low-level API
- High-level API
1. Low-level API
- It has complete programming control.
- It is recommended mostly for machine learning researchers.
- It provides us with fine levels of control over the modules.
- TensorFlow Core is an example of a low-level API.
2. High-level API
- It is built on top of the other low-level APIs.
- It is easier to learn and use than other low-level APIs.
- Between multiple users, it makes repetition easier and more consistent.
Let us consider an example program in python using TensorFlow.
Example
# importing tensorflow
import tensorflow as tf
# creating nodes of computational graphs
node_a = tf.constant ( 4, dtype = tf.int32 )
node_b = tf.constant ( 5, dtype = tf.int32 )
node_c = tf.add ( node_a, node_b )
# creating object for tensorflow session
s = tf.Session()
# evaluating node_c and printing of result
print( " addtion of node_a and node_b is : ", s.run(node_c) )
# closing the session object
s.close()
Output
sum of node_a and node_b is : 9
Explanation of above code
Step 1: Creating a computational graph.
Creating a computational graph means defining the nodes. TensorFlow provides us with different types of nodes for different types of tasks. Each node takes zero or more tensors as inputs and produces an output as a tensor.
- In the above program, the nodes node_a and node_b are of tf.constant type. A node that takes no inputs is called a constant node. It outputs value is stored internally.
NOTE: dtype argument is used to specify the data type of the output
node_a = tf.constant ( 4, dtype = tf.int32 )
node_b = tf.constant ( 5, dtype = tf.int32 )
- The node node_c is of tf.add type which, takes two tensors as input and gives the resultant value as the output tensor.
node_c = tf.add ( node_a, node_b )
Step 2: Running of a computational graph
A session must be created to run a computational graph.
s = tf.Session()
Now, we should use run method for performing computations
print( " addtioon of node_a and node_b is : ", s.run(node_c) )
Finally, we should use close method to close the method.
s.close()