Sys Module in Python
What are Modules?
The Modules are the kind of files that contain Python statements and definitions. The module is known by the name of the file followed by the suffix “.py”. It can define variables, functions, and classes. It helps in making code easy to use and easier to understand by grouping all code related to each other into a common module. Thus, the code appears organized logically.
Advantages of using Modules
- These can be easily renamed and used freely anywhere in our code.
- It reduces code redundancy.
- A code fragment can be used multiple times without writing it again and again.
- The modules can be built-in or the user can also create them. For example, math, Tkinter, random, etc. are built-in modules; and you can create different modules according to your comfort.
What is sys Module?
Whenever we want to make changes in the different parts of the Python run time environment, we require some different functions and variables, The Sys module provides the same and makes our task easy. This module helps us to operate on the interpreter, as it provides different functions and variables that help in interacting with the interpreter.
Example:
importsys
a = sys.version
print( a )
Output:
3.10.0 (tags/v3.10.0:b494f59, Oct 4 2021, 19:00:18) [MSC v.1929 64 bit (AMD64)]
As we can see in the output of the above-given example, the sys.version returns the version of the Python interpreter along with some additional information, and this all information is returned in form of a string. This shows the way the sys module interconnect with the interpreter and returns the version of Python.
Input and Output using sys module
Using the sys module, we can use the input and output on our own terms due to the variables provided by this module. The following three variables can be used to do so:
- stdin
- stdout
- stderr
stdin
This variable is used to take input directly from the command line. It stands for “standard input”, this variable calls the input() method. This variable automatically adds the “\n” after every sentence.
Example:
importsys
forxinsys.stdin:
if'q'==x.rstrip():
break
print(f'Input : {line}')
print("Exit")
Output:
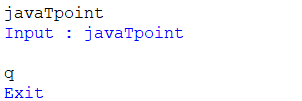
stdout
It is a built-in file object which is almost similar to the output stream in Python. It is used to return output directly to the screen console. The returned output may have any form, it can be output from an expression statement, a print statement, or a prompt direct for input. The streams are in text mode by default. When we want to print something, we simply use the print function, but internally, first, the required expression is written to sys.stdout and then displayed on the output screen.
Example:
importsys
sys.stdout.write('How are you?')
Output:
How are you?
stderr
In Python, all the encountered exceptions are written sys.stderr.
Example:
importsys
defprtStderr(*x ):
# In this function, x is an array that stores the object
# that is passed as the argument to the function
print(*x, file=sys.stderr)
prtStderr("Hey!! How are you?")
Output:
Hey!! How are you?
Command Line Arguments
Arguments passed during calling a program along with the calling statement is called command line argument. The sys module can be used to achieve this too, as this module has a variable known as sys.argv which helps in doing so. The main aim of this variable is:
- It returns the list of command line arguments.
- len(sys.argv) is used to get the number of command line arguments.
- The name of the current Python script is given by: sys.argv[0].
Example:
# A code in Python to illustrate
# command line arguments
importsys
# Total number arguments
l=len(sys.argv)
print("Total number of arguments passed:", l)
print("\nName of Python script:", sys.argv[0])
# Name of thearguments passed
print("\nArguments passed:", end =" ")
forjinrange(1, l):
print(sys.argv[ j ], end =" ")
# Sum of numbers passed as arguments
sum=0
forkinrange(1, l):
sum+=int(sys.argv[ k ])
print("\n Final result:", sum)
Output:
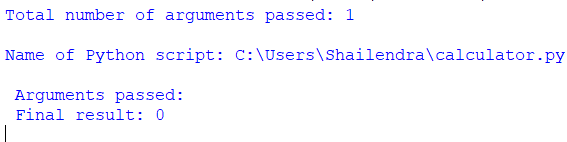
Exiting the program
To exit any program, we use sys.exit([arg]). In this statement, the argument “arg” can be taken as an integer giving the exit or any other type of object. A zero is considered as a successful termination if the argument passed is an integer.
Note: You can also pass a string as an argument in this statement.
Example:
# A code in Python to illustrate
# the sys.exit() statement
importsys
age =15
ifage <18:
# Exits the program
sys.exit("Age entered is less than 18")
else:
print("Age entered is not less than 18")
Output:
Age entered is not less than 18
Working with modules
To return the list of directories that will be searched by the interpreter for the required module can be accessed with the help of sys.path which is a built-in variable in the sys module.
When we import any module in a Python file, first, the specified module is searched within the built-in modules by the interpreter, if found good. If not then it searches among the list of directories provided by sys.path.
Note: sys.path is an ordinary list, and it can be manipulated according to the requirement of the user.
Example 1: Printing all paths
importsys
x = sys.path
print(x)
Output:
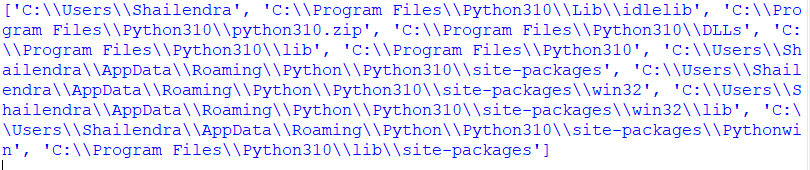
Example 2: Removing all the values present in sys.path
importsys
# Removing the values
sys.path =[]
# Importing numpy after removing all
# the values from sys.path
importnumpy
Output:

sys.module
This statement is used to display the names of the modules imported by the current Python shell.
Example:
importsys
x = sys.modules
print(x)
Output:
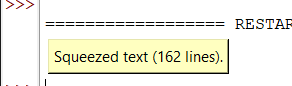
Reference Count
To know about the reference count of any object, we use the sys.getrefcount() method. Python use this value to perform operations, and when this value becomes 0, the memory for that particular object is freed (deleted).
Example:
importsys
a ='How are you'
b =sys.getrefcount(a)
print( b )
Output:
3
Some more functions in the Python sys module
- sys.setrecursionlimit() method: This method is used to set the limit of the maximum depth of the stack of the Python interpreter.
- sys.getrecursionlimit() method: This method is used to find the maximum depth of the Python interpreter stack, and it can also be used to get the current recursion limit of the interpreter.
- sys.settrace () method: This method is used to implement profilers, coverage tools, and debuggers.
- sys.switchinterval () method: This method is used to set the thread switch interval (in seconds).
- sys.maxsize () method: This method is used to get the largest value that a variable can store of data type Py_ssize_t.
- sys.maxint () method: This method is used to denote the highest value that the integer is capable to represent.
- sys.getdefaultencoding () method: This method is used to fetch the default string encoding that is currently being used by the Unicode Implementation.