Python Syntax
Python is a strong object-oriented programming language that is simple to learn. Python was created to be a very readable programming language. The syntax of the Python programming language is a set of guidelines that specify how a Python program should be constructed.
Comments
# Indicates that a comment has been made.
A comment starts with a hash character (#) that is absent from the literal String and finishes at the end of the actual line. The Python interpreter ignores any characters after the # character to the end of the line that is part of the remark. It's worth noting that Python doesn't support multi-line or block comments. Consider the following scenario;
A=10989
# A is initialized with a numeric value of 11.
if(A!=200000):
print(“Your number is not equal to 200000”)
- You can have stand-alone comments like the one above and inline comments appearing after a statement. Consider the following scenario:
A=11000 #A is initialized with a numeric value of 11.
- Python does not have a syntax for multi-line comments like C and C++'s /*... */, yet multi-line strings are frequently used in place of multi-line comments.
Indentation
A block of code is a group of statements in a programming language that should be handled as a unit. Curly brackets are used to indicate code blocks in C, while indentation is used to indicate code blocks in Python, as shown in the example below;
C program:
for(doublei=11.0;i<50;i++)
{
Total_price+=i;
// curly braces are indicating the code block in this C code.
}
Python program:
foriinrange(50):
Total_price+=i #The indentation indicates the code block in this python code.
Note: Indented code blocks in Python are always preceded by a colon (:) on the preceding line.
- The use of indentation aids in enforcing the uniform, legible style that many Python programmers prefer. However, for the uninformed, it may be perplexing; for example, the following two snippets will provide different outcomes.
>>>ifx!=100:>>>ifx!=4:
...y=x%2...y=x%2
...print(y)...print(y)
In the excerpt on the left, print(y) is indented, and it will only be run if x is less than 100. In the excerpt on the right, print(y) is outside the block and will be run regardless of the value of x.
Finally, remember that the amount of whitespace used to indent code blocks is completely up to the user, as long as it's consistent throughout the script.
Most style guidelines recommend indenting code blocks by four spaces, and we'll follow that recommendation in this report.
Coding Style in Python
- There should be four spaces between each indentation and no tabs.
- Do not use tabs and spaces in the same sentence. Because tabs can be confusing, sticking to utilizing only spaces is preferable.
- Users with a small display will appreciate the maximum line length of 79 characters.
- Separate top-level function and class declarations by blank lines and bigger chunks of code within functions should be separated by a single blank line.
- Inline comments should be used (they should be complete sentences).
- Around expressions and assertions, use spaces.
Keywords
The Python keywords are listed below. A keyword is a word used in programming languages with a specific meaning. Because these words are reserved, you cannot use them as constants, variables, or other identifier names.
- As we know that Python is a powerful tool, but this language is still being developed. As a result, the company's keywords will continue to evolve and increase.
Here are some of its keywords:
False class finallyisreturn
Nonecontinueforlambdatry
Truedeffromnonlocalwhile
anddelglobalnotwith
aselififoryield
assertelseimportpass
breakexceptinraise
- The keyword is a module in Python that allows one to list all of your keywords. To get the most recent keyword list, use the code below;
import keyword
print(keyword.kwlist)
Identifiers (Names)
Identifiers are fundamental building blocks of a program that serve as a naming convention for various program elements like variables, objects, classes, functions, lists, dictionaries, and so on.
The following are the rules that makeup Python Identifier:
- A string of letters and numbers that can be any length is an identifier.
- Lowercase and uppercase letters are distinct.
All of the characters are important.
- A letter must be the initial character; the underscore (_) qualifies as a letter.
- Except for the initial letter, the digits 0-9 can be part of the identifier.
- An identifier cannot be a Python keyword.
- Except for the underscore (_), an identifier cannot contain any special characters.
Some examples of valid identifiers are listed below:
Myidenti Date_21_22
MYIDENTI _beauti
ABC file_date_22
A10BCDE _invita_01
Some examples of invalid identifiers are listed below:
My-identi False
23MYIDENTI My.logo
from easypeesylemonsqegy@
Continuation of the Statements
- As in Python, every statement is written on its line; therefore, to separate statements in Python, the newline character is used.
- However, using the backslash (/) character, a long statement can span multiple lines.
if (x==120) and (y>120) or \
(y<300):
print(“This was an example to illustrate the use of backslash (\) character”)
Please note that the backslash character can span multiple physical lines and a single statement on one logical line, but not two different statements in one logical line.
- Expressions enclosed in parentheses (), square brackets (), or curly braces can span multiple lines without using backslashes.
Look at the code below for better understanding:
my_list = [100, 200, 300, 400
500, 600, 700, 800,
900, 1000, 1100, 1200]
End-of-Line Marks the End of a Statement
Item_price=180
We've established a variable called Item price and given it the value 180 in this assignment operation. The end of this sentence is simply signalled by the end of the line, as you can see. This differs from languages such as C and C++, which require every statement to end with a semicolon (;).
Semicolon Can Optionally Terminate a Statement
To divide numerous statements on a single line, use the semicolon as shown in the code below:
print('Rollno : ', 1);print(‘Name: ', 'Harry Potter');print('Age : ', '23');print(‘gender : ’, ‘Male’)
Here, the above code is equivalent to;
print('Rollno : ', 1)
print(‘Name: ', 'Harry Potter')
print('Age : ', '23')
print(‘gender : ’, ‘Male’)
Most Python style guides advise against using a semicolon to group many statements on a single line; however, it can be useful occasionally.
It doesn’t matter if there's whitespace between the lines.
While meaningful whitespace before lines (which signify a code block) is important, whitespace within lines of Python code is unimportant. All four of these formulations, for example, are equivalent:
A=12*5
A= 12 * 5
A= 12 * 5
A=12 * 5
One should note that When operators follow one another, effectively using whitespace can result in significantly more readable code, as you can see below: -
A=12*-9
Vs
A=12* -9
String Literal
A String literal is a sequence of characters surrounded by quotation marks.
A string literal in Python is denoted by single quotes ('), double quotes ("), triple single quotes ("'), and triple-double quotes (""").
If a string in Python began with a double quote, then it should end with a double quote too. The reason is that the String Literal in Python must be surrounded by the same types of quotes.
The following are some string literal examples:
A=” This is an example of String literal "
B=” String literal are surrounded by quotations “
print(A)
print(B)
Output in Python
- In Python, print() is used as an output statement.
- It displays the value of any Python expression on the Python shell.
- The single print() method can display several values separated by commas.
Using the single print() method.
- A single space ‘ ’ default acts as a separator between values. Any other character can be used with a sep argument.
- Example:
>>>Rollno=123
>>>print(Rollno)
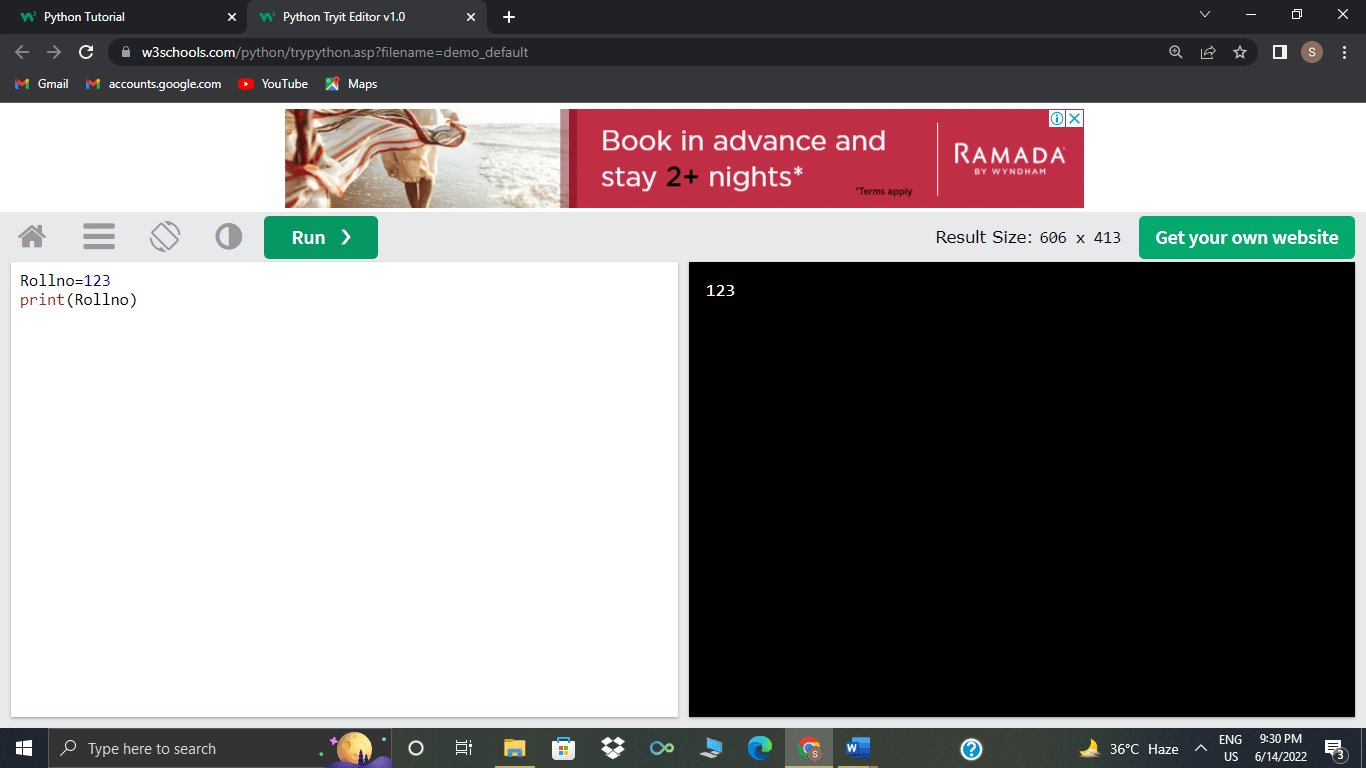
>>>name=”Unknown”
>>>print(name)
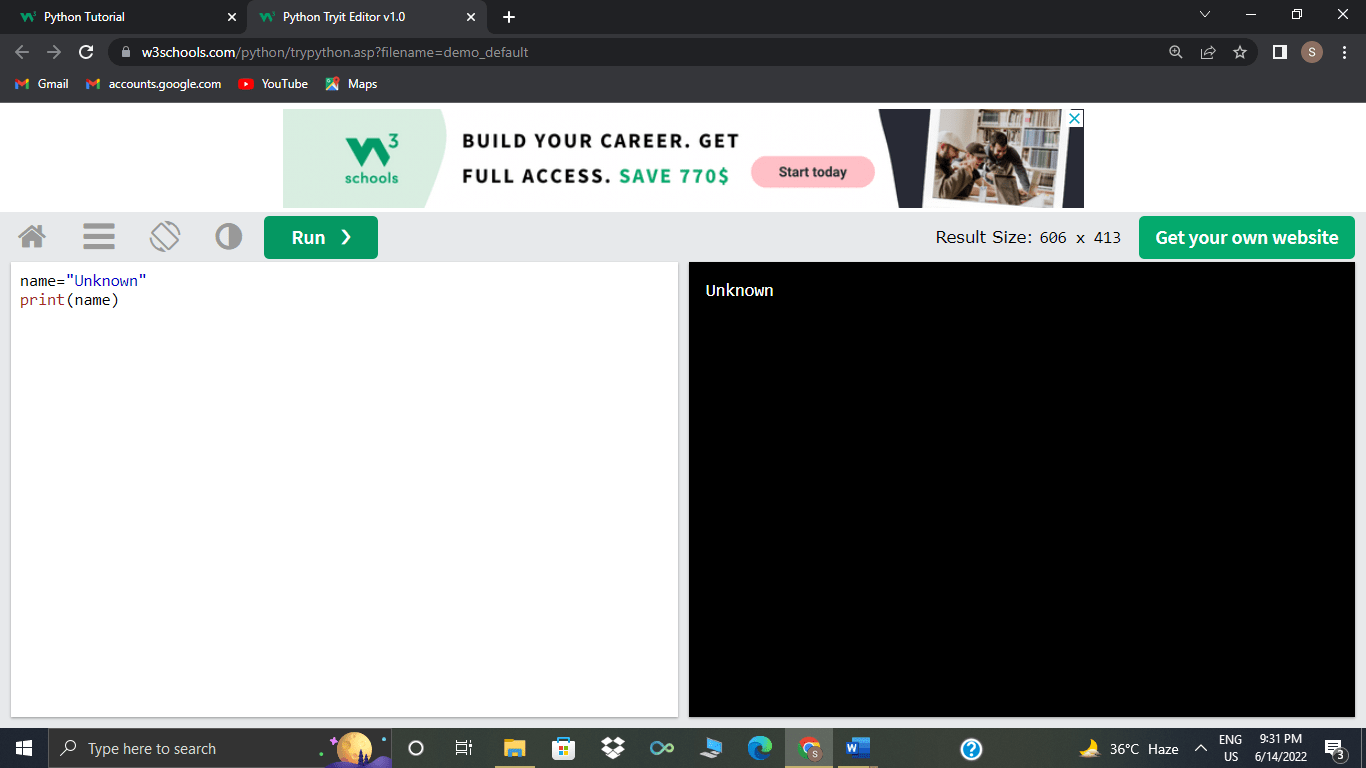
>>>print(“Roll no: “, Rollno,”,Name :”, name)
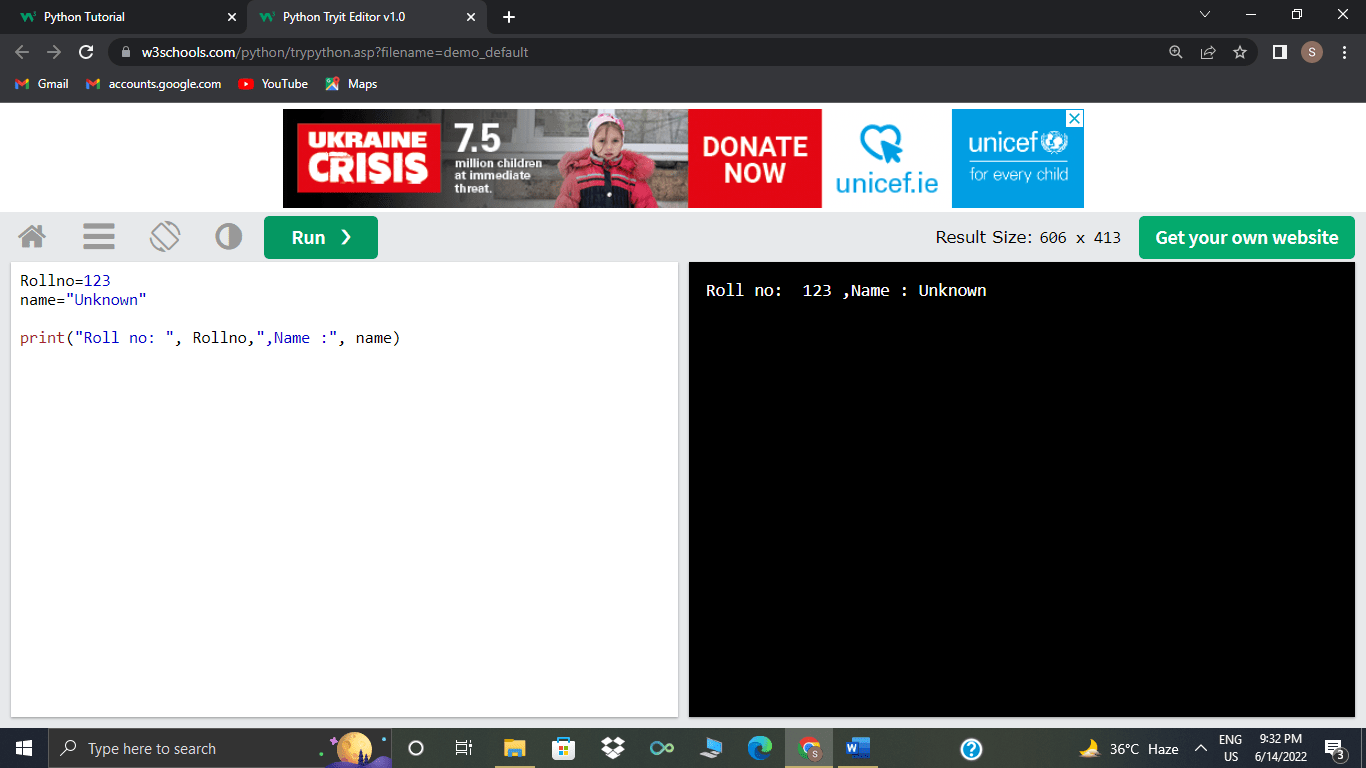
Obtaining User Input
- The input() function is included in the standard Python distribution's core library. It interprets the keystrokes as a string object that a variable of the same name may reference.
>>>a=int(input(“enter your rollno : “ ))
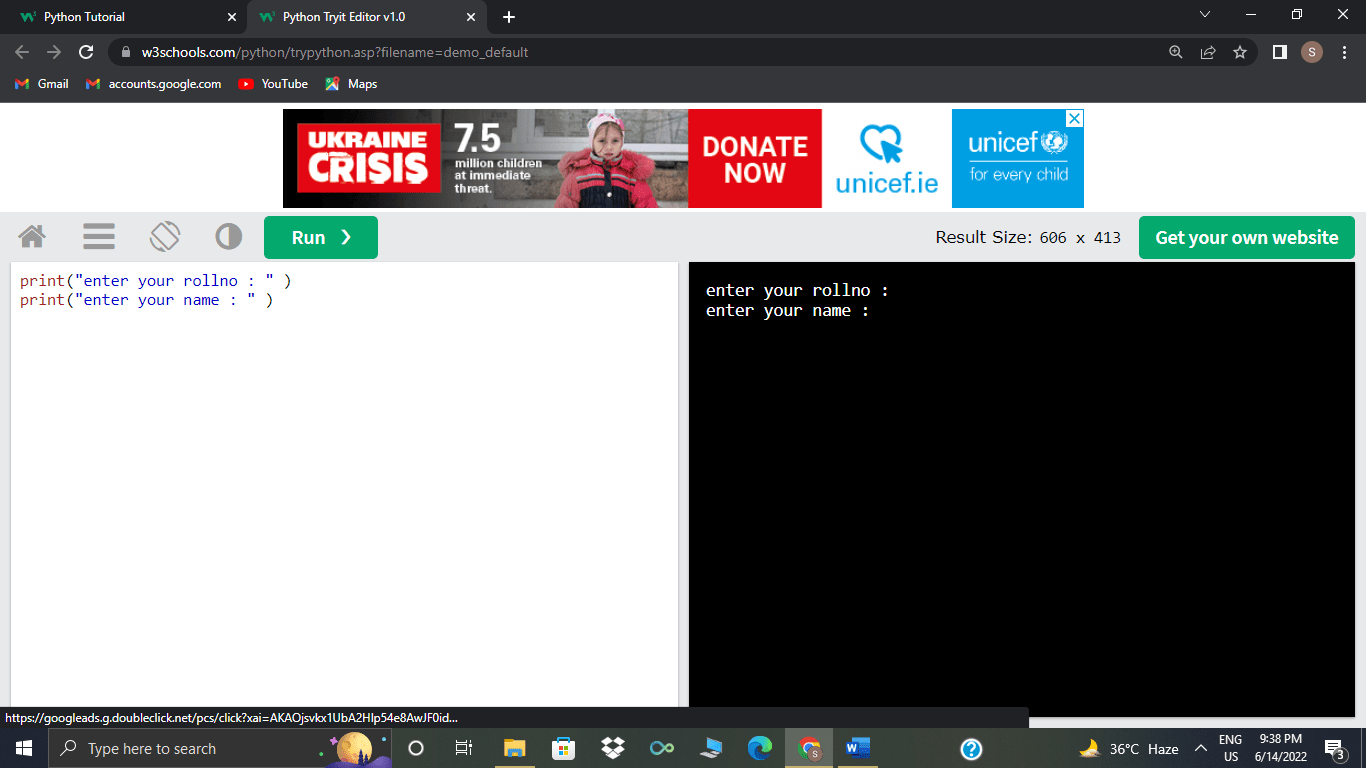
>>>b=input(“enter your name : “ )
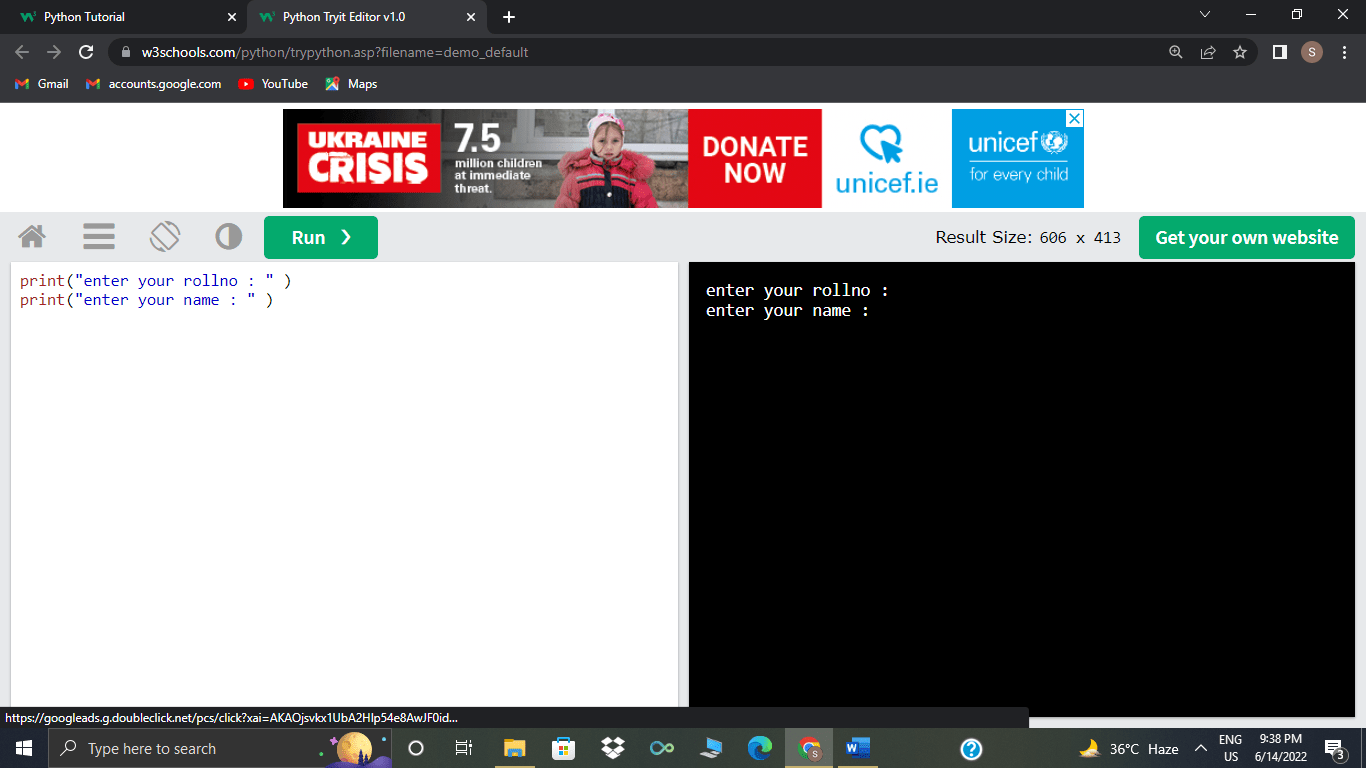
Summary
- A newline character is used to finish a Python statement.
- Python organizes its code structure with spaces and identifiers.
- The names given to variables, functions, modules, classes and other objects in Python are called identifiers.
- The code's functionality is explained in the comments. The Python interpreter ignores them.
- To designate a string, single, double, triple, or triple-double quotation marks can be used.