Python Deep Copy and Shallow Copy
Assignment statements in Python create bindings between a target and an object, not copies of them. The = operator only makes a new variable that shares the reference to the original object when it is used. The Python copy module can be used to make "true copies" or "clones" of these objects.
In essence, we might occasionally only want to change the new values and leave the original values alone. There are two techniques for making copies in Python:
- Deep Copy
- Shallow Copy
We employ the copy module to make these copies functional.
However, this approach only generates shallow copies and is ineffective for custom objects. There is a significant distinction between shallow and deep copying for compound objects like dicts, lists and sets:
Creating a new selection object and then inhabiting it with references to the child items found in the original is known as a "shallow copy." A shallow copy is essentially one level deep. Since the copying procedure does not recurse, copies of the child objects themselves will not be produced.
The copying procedure is iterative when using a deep copy. It entails first building a fresh collection object, which is then recursively filled with duplicates of the original collection object's children. By copying an object in this way, the original object and all of its children become completely independent clones.
Shallow Copy in Python:
A shallow copy builds a brand-new collection object and thereafter populates it with references to the child objects located in the original. It then references the objects contained in the original within the new compound object that it creates. The copying procedure doesn't recurse, so the child objects themselves won't be duplicated. When an object is copied, its reference is incorporated into another object. In other words, any modifications made to a copy of an object will be reflected in the original object. The "copy()" function in Python is used to carry out this.
Example:
The fact that another list was impacted by the change made in this example shows that the list was only superficially copied. Important Information The distinction between deep and shallow copying only applies to compound objects (i.e., items like lists or instances of classes that contain other items):
To the greatest extent possible, a shallow copy creates a new compound object and then references the objects found in the original within it.
A deep copy creates a new compound object before inserting copies of the objects found in the original into it in a recursive manner.
Sample Code:
# importing "copy" for copy operations
import copy
# initializing list 1
list1 = [1, 2, [3,5], 4]
# using shallow copy to copy
list2 = copy.copy(list1)
# original elements of list
print ("The original elements before shallow copying")
for i in range(0,len(list1)):
print (list1[i],end=" ")
print("\r")
# adding a new list element
list2[2][0] = 7
# determining whether a change is reflected
print ("The original elements after shallow copying")
for i in range(0,len( list1)):
print (list1[i],end=" ")
Output:

Deep Copy in Python:
In a deep copy, a new compound object is first created, and then copies of the components found in the original are recursively inserted into it. It entails creating a new collection object first, then populating it recursively with copies of the child objects discovered in the original. When using deep copy, an object is duplicated inside of another object. In other words, any modifications made to a copy of the object that do not affect the original object.
Example:
The fact that other lists were unaffected by the change made to the list in the example above shows that the list is heavily copied.
Sample Code:
# importing "copy" for copy operations
import copy
# initializing list 1
list1 = [1, 2, [3,5], 4]
# using deepcopy to deep copy
list2 = copy.deepcopy(list1)
# original elements of list
print ("The original elements before deep copying")
for i in range(0,len(list1)):
print (list1[i],end=" ")
print("\r")
# adding a new list element
list2[2][0] = 7
print ("The new list of elements after deep copying ")
for i in range(0,len( list1)):
print (list2[i],end=" ")
print("\r")
#Original list does not reflect the change.
# since it's a deep copy
print ("The original elements after deep copying")
for i in range(0,len( list1)):
print (list1[i],end=" ")
Output:
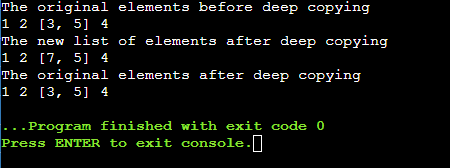