ComboBox in Python
Python includes several graphical user interface (GUI) libraries, including PyQT, Tkinter, Kivy, WxPython, and PySide. Tkinter is the most commonly used GUI module in Python because it is simple and easy to understand.
Tkinter is a fork of the Tk interface. The tkinter module is included in the Python standard library and must be imported when writing a Python programme
that generates a graphical user interface.
A Combobox is a cross between a list box and a text entry field. It's a Tkinter widget with a down arrow for choosing from a list of options.
It allows users to select from a list of displayed options. When the user clicks on the drop-down arrow in the entry field, a pop-up of the scrolled Listbox appears down the entry field. Only after selecting an option from the Listbox will the selected option be displayed in the entry field.
The combobox widget's syntax is as follows:
combobox = ttk.Combobox(master, option=value, ...)
Example 1: Combobox widget without setting a default value.
We can create a combobox widget without setting a default value. In this example we will look at the approach for the same.
import tkinter as tk
from tkinter import ttk
window = tk.Tk()
window.title('Combobox')
window.geometry('500x250')
ttk.Label(window, text = "JTP Combobox Widget",
background = 'black', foreground ="white",
font = ("Times New Roman", 15)).grid(row = 0, column = 1)
# label
ttk.Label(window, text = "Select the day :",
font = ("Times New Roman", 10)).grid(column = 0,
row = 5, padx = 10, pady = 25)
# Combobox creation
n = tk.StringVar()
daychoosen = ttk.Combobox(window, width = 27, textvariable = n)
# Adding combobox drop down list
daychoosen['values'] = (' Monday','Tuesday','Wedenesday','Thursday','Friday','Saturday','Sunday')
daychoosen.grid(column = 1, row = 5)
daychoosen.current()
window.mainloop()
Output for the above program is as follows:
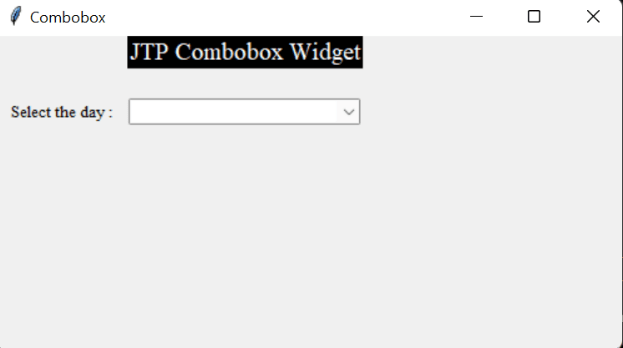
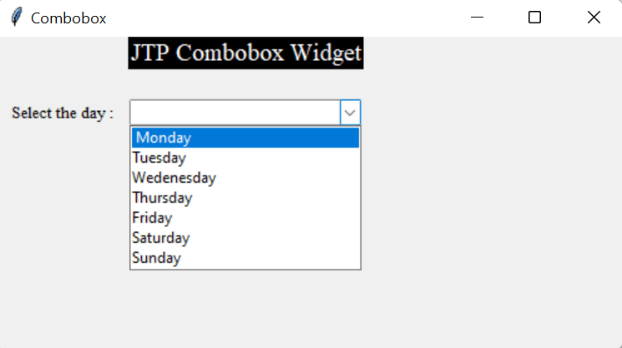
Example 2: Combobox with initial default values.
We can also set the initial default values in the Combobox widget as shown in the below sample code.
import tkinter as tk
from tkinter import ttk
window = tk.Tk()
window.title('Combobox')
window.geometry('500x250')
ttk.Label(window, text = "JTP Combobox Widget",
background = 'black', foreground ="white",
font = ("Times New Roman", 15)).grid(row = 0, column = 1)
# label
ttk.Label(window, text = "Select the day :",
font = ("Times New Roman", 10)).grid(column = 0,
row = 5, padx = 10, pady = 25)
# Combobox creation
n = tk.StringVar()
daychoosen = ttk.Combobox(window, width = 27, textvariable = n)
# Adding combobox drop down list
daychoosen['values'] = (' Monday','Tuesday','Wedenesday','Thursday','Friday','Saturday','Sunday')
daychoosen.grid(column = 1, row = 5)
daychoosen.current(0)
window.mainloop()
