Reverse a String in Python
Python is an object-oriented high-level programming language. Python has dynamic semantics and has high-level built-in data structures which support dynamic typing and dynamic binding. Python provides rapid development. It has an English-like syntax that is very easy to write and understand which also reduces the maintenance cost. Python is an interpreted language which means that it uses an interpreter instead of the compiler to run the code. The interpreted language is processed at the run time thus takes less time to run. Python also has third parties modules and libraries which encourage development and modifying the code.
In this post, we are going to discuss how to reverse a string in Python. In Python, strings are ordered sequences of characters. You may not get this problem directly in an interview, but you may find its application in some other questions in which you want to reverse the string or do a similar operation. It also has some real-world applications.
String reversal is not so common, so strings do not support the inbuilt function reverse() like lists or other containers. We are going to use four approaches to reverse a string in Python, they are:
- Slicing
- While loop
- Reversed() function
- Recursion
1. Slicing
Python has an amazing feature called Slicing. With slicing, we can get a specific part of strings, tuples, and lists. Slicing can also be used to modify or delete items from mutable containers in python lists. Slicing provides very clean and short code which is easier to read.
Slicing is similar to indexing but it returns a range of elements instead of just one. The syntax of slicing is [start:stop:step]. The start and stop define the elements from where we are going to slice and till where we are slicing. Step determines the gap between the elements.
For reversing a string in Python the code is:
Code
def reverse_str(string):
string = string[::-1]
return string
str = input(“Enter a string:”)
print ("The string is : ",str)
print ("The reversed string using slicing is : ",reverse_str(str))
Output
Enter a string: python
The string is:python
The reversed string using slicing is:nohtyp
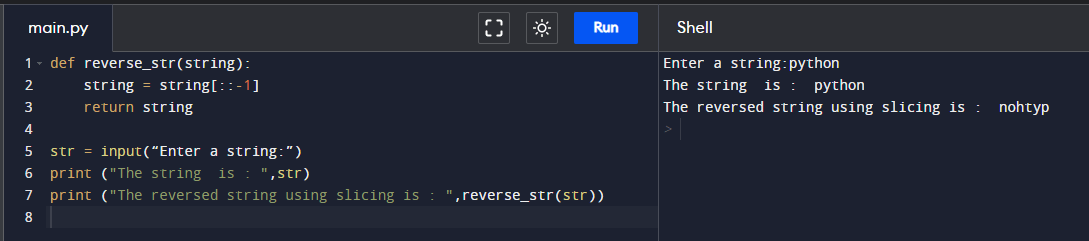
Explanation
Here we have not written anything in the start and step in the syntax of slicing which is similar to writing 0 and string length respectively. The -1 in step indicates that the interpreter has to process the string in the backward direction, henceforth reversing the string.
2. While loop
While the loop in Python is like in any other language, the statements inside the loop will run as long as the condition of the loop is true. Here, we will first take the input of the string from the user. Then we will create an empty string and store the characters in reverse order.
Code:
def reverse_str(string):
reversed_string=[]
length = len(string)
while length > 0:
reversed_string += string[ length - 1 ]
length = length - 1
returnreversed_string
str = input(“Enter a string:”)
print ("The string is: ",str)
print ("The reversed string using While loop is: ",reverse_str(str))
Output
Enter a string:python
The string is: python
The reversed string using While loop is: nohtyp
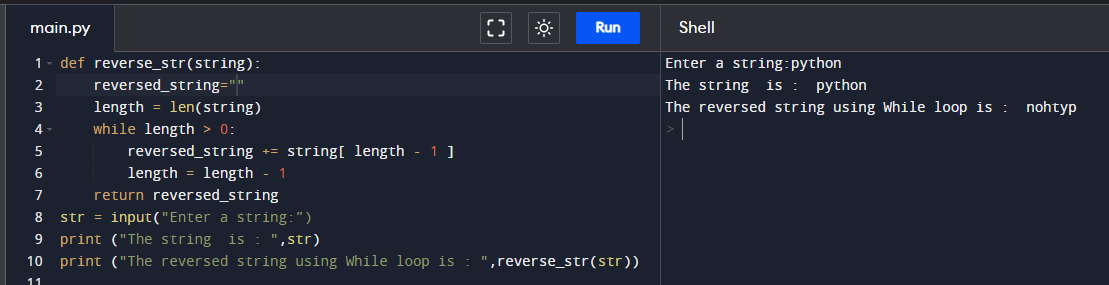
Explanation
In the code above, the logic is implemented in the reverse_str function. We have initialized an empty string called reversed_string which stores the final reversed string. Then we are looping the original string in reverse order. We are storing the last element first in our reversed_string variable. After completing the iteration and appending all the characters it will return the string.
3. Reversed() function
This is a very powerful approach in which we are taking advantage of various advanced functions in Python. In this case we are using the .join() function with reversed() function. Reversed() function returns the reverse iteration of the string. The following python code demonstrates the process to reverse a string:
Code
def reverse_str(string):
reversed_str = "".join(reversed(string))
return reversed_str
str = input(“Enter a string:”)
print ("The string is: ",str)
print ("The reversed string using Reversed function loop is: ",reverse_str(str))
Output
Enter a string: python
The string is: python
The reversed string using Reversed function is: nohtyp
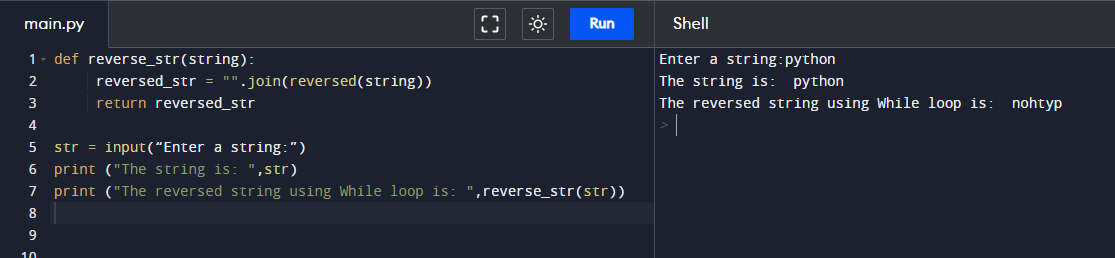
Explanation
We have defined the function in which we are using the built-in function reversed() to traverse through all the elements in the string in reverse order. Then we are joining all the elements given by the .reversed() function by using a .join() function and storing them in a variable called reversed_str and finally, we are returning the variable.
4. Recursion
The final approach we are going to discuss in this post is recursion. Recursion is a method of solving a problem when the problem is defined in terms of itself. When a function calls itself, then it is termed a recursive function. Let us look at the code to reverse a string using recursion.
Code
def reverse_str(string):
if len(string) == 0: # Checking the lenght of string
return string
else:
return reverse_str(string[1:]) + string[0]
str = input(“Enter a string:”)
print ("The string is: ",str)
print ("The reversed string using Recursion is: ",reverse_str(str))
Output
Enter a string: python
The string is: python
The reversed string using Recursion is: nohtyp
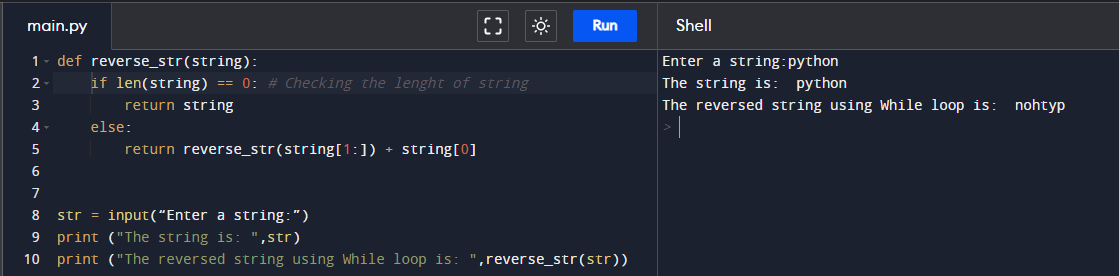
Explanation
In the code above, we have taken the string as an input from the user and we are passing that string to the function reverse_str which is returning us the reversed string. In the function, the logic is implemented.
We are using slicing here, but instead of just slicing one element we are taking the whole list except one element. There is a base condition defined in the function which is if the length of the string is 0, it will return the string else we will pass the string by removing the first element from it and concatenates that letter to the returned string.