Create a Table Using Tkinter in Python
Tkinter:
The standard Python technique for building Graphical User Interfaces (GUIs) is Tkinter, which is included in all popular Python distributions. The only framework included in the Python standard library is this one. On top of Tk, this Python framework serves as a thin object-oriented layer that provides access to the Tk toolkit. The Tk toolkit is a cross-platform set of "graphical control elements," also called widgets, used to build application interfaces. This framework gives Python users a quick and easy way to build GUI elements with the Tk toolkit's widgets. In a Python application, Tk widgets can be used to create buttons, menus, data fields, etc.
Once created, these graphic elements can be connected to or work with other widgets, features, functionality, processes, or data.
Tkinter bridges the gap between Python's execution model and Tcl's, built around cooperative multitasking. Like Python, Tcl is an interpreted dynamic programming language. Although it is an overall programming language that can be used independently, it is most frequently used in C programs as a scripting motor or as an interaction with the Tk toolbox. The Tcl library has a C interface that enables users to add custom Tcl or C commands, run Tcl commands and scripts, and manage one or more Tcl interpreter instances. Each interpreter has a queue for events that can be used to send and process events.
Syntax:
from tkinter import *
from tkinter import ttk
Advantages of Tkinter:
Qt is more than just a framework; it uses a variety of native platform APIs for networking, database development, and other uses. Through a unique API, it gives them direct access. This promotes flexibility in handling GUI incidents, which makes the code base run more smoothly.
Disadvantages of Tkinter:
Tkinter doesn't have any advanced widgets. There isn't a tool like Qt Designer for Tkinter in it. Its user interface is unreliable. Tkinter can be challenging to debug at times. It's not entirely in Python.
Creating tables using Tkinter:
When displaying information in the format of rows and columns, a table is helpful. Sadly, Tkinter does not include a Table widget for building tables. But there are other ways we can make a table. For instance, we can create a table by repetitively showing admittance widgets in the format of rows and columns.
Consider a scenario in which an application has a table GUI, and we can manipulate the information using other Python libraries like Numpy, Pandas, and Matplotlib. pyplot, etc. The user can draw a table and add data using Tkinter’s TreeView widget. Data items are presented in tables as rows and columns.
CODE:
from tkinter import *
parent = Tk()
redbutton = Button(parent, text = "Red", fg = "red")
redbutton.pack( side = LEFT)
greenbutton = Button(parent, text = "Black", fg = "black")
greenbutton.pack( side = RIGHT )
bluebutton = Button(parent, text = "Blue", fg = "blue")
bluebutton.pack( side = TOP )
blackbutton = Button(parent, text = "Green", fg = "red")
blackbutton.pack( side = BOTTOM)
parent.mainloop()
OUTPUT:
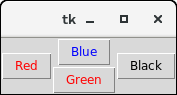
CODE:
from tkinter import *
parent = Tk()
name = Label(parent,text = "Name").grid(row = 0, column = 0)
e1 = Entry(parent).grid(row = 0, column = 1)
password = Label(parent,text = "Password").grid(row = 1, column = 0)
e2 = Entry(parent).grid(row = 1, column = 1)
submit = Button(parent, text = "Submit").grid(row = 4, column = 0)
parent.mainloop()
OUTPUT:
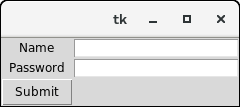
CODE:
from tkinter import *
class Table:
def __init__(self,root):
# creating a table
for i in range(total_rows):
for j in range(total_columns):
self.e = Entry(root, width=20, fg='blue',
font=('Arial',16,'bold'))
self.e.grid(row=i, column=j)
self.e.insert(END, lst[i][j])
# input of table data
lst = [(1,'Raj','Mumbai',19),
(2,'Aaryan','Pune',18),
(3,'Vaishnavi','Mumbai',20),
(4,'Rachna','Mumbai',21),
(5,'Shubham','Delhi',21)]
# finding the total number of rows and columns in the list
total_rows = len(lst)
total_columns = len(lst[0])
# createing an root window
root = Tk()
t = Table(root)
root.mainloop()
OUTPUT:

CODE:
# Importing the required python libraries
from tkinter import *
from tkinter import ttk
win = Tk()
win.geometry("700x350")
s = ttk.Style()
s.theme_use('clam')
tree = ttk.Treeview(win, column=("c1", "c2", "c3"), show='headings', height=5)
tree.column("# 1", anchor=CENTER)
tree.heading("# 1", text="ID")
tree.column("# 2", anchor=CENTER)
tree.heading("# 2", text="FName")
tree.column("# 3", anchor=CENTER)
tree.heading("# 3", text="LName")
tree.insert('', 'end', text="1", values=('1', 'Joe', 'Nash'))
tree.insert('', 'end', text="2", values=('2', 'Emily', 'Mackmohan'))
tree.insert('', 'end', text="3", values=('3', 'Estilla', 'Roffe'))
tree.insert('', 'end', text="4", values=('4', 'Percy', 'Andrews'))
tree.insert('', 'end', text="5", values=('5', 'Stephan', 'Heyward'))
tree.pack()
win.mainloop()
OUTPUT:
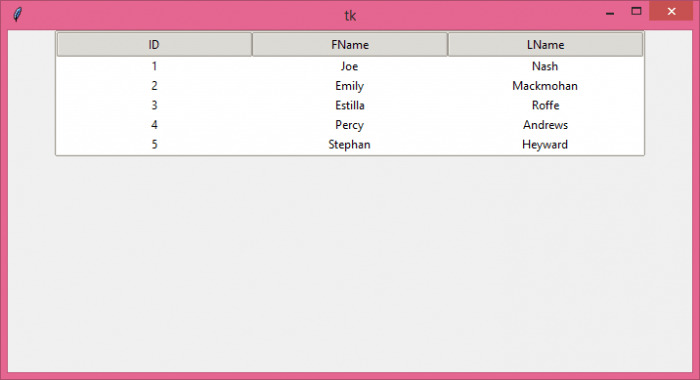