Nested for Loop in Python
"Loop" is one of the foundation concepts to learn programming in any language. Nested loops are significant steps in solving many types of problems, ranging from basic to complex scenarios. In almost all the languages, we can nest loops like we nest if-else statements. There are 2 types of loops in Python; while loops and for loops. We can nest a while loop inside a for loop and vice versa. This article will see several examples of nesting a for loop. In this article, we are going to discuss the below topics:
- Syntax
- Use of nested for loops
- Addition of two matrices example
- Creating a star semi pyramid example
- Nesting a while loop inside a for loop
- Using the control flow statements-break and continue.
- Writing in one line sing list comprehension.
Nesting a loop means writing a loop inside the body of another loop. The inner loop is nested inside the outer loop. We can nest any number of loops inside a loop.
Syntax
1. for loop nested inside a for loop
for iterating_element in sequence:
#body of the outer loop
for iterating_element in sequence:
#body of the inner loop
#body of the outer loop
2. while loop nested inside a for loop
for iterating_element in sequence:
#body of the outer loop
while (Test condition):
#body of the inner loop
#body of the outer loop
- First, the outer loop will start executing; for an iteration of the outer loop, the inner loop will be executed totally (all iterations) i.e. For every iteration of the outer loop, the inner loop will be un-touched and starts all new and ends completely then, the next iteration will be proceeded.
- Mathematically, the total number of iterations will be equal to the product of the number of iterations of the outer loop and the number of iterations of the inner loop.
Usage of Nested Loops
- Nested for loops are mostly used to perform execution on multi-dimensional data structures in Python like printing, iterating, etc.
- Nested looping has a major part in the control flow statements in Python.
- At the basic level, we use nested for loops to iterate a sequence like a list or a tuple using objects like range.
Let us take a scenario, analyze it and write a code for it:
Example-1: Addition of two 3 * 3 matrices
Analysis:
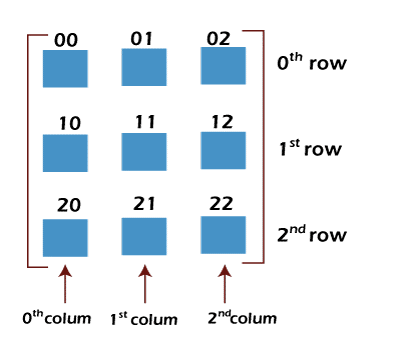
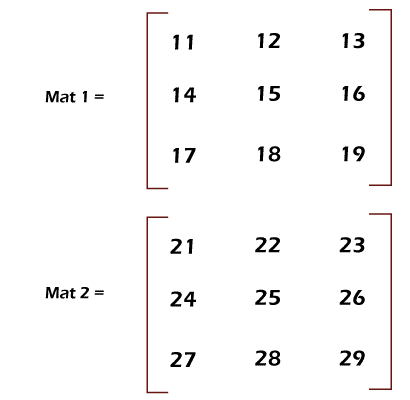
We need 2 for loops to iterate the 2 matrices to corresponding indices.
Code:
Mat1 = [[11, 12, 13],
[14, 15, 16],
[17, 18, 19]]
Mat2 = [[21, 22, 23],
[24, 25, 26],
[27, 28, 29]]
sum12 = [[0, 0, 0],
[0, 0, 0],
[0, 0, 0]]
for i in range (len (Mat1)):
for j in range (len (Mat1 [0])):
sum12 [i][j] = Mat1 [i][j] + Mat2 [i][j]
for i in sum12:
print (i)
Output:
[32, 34, 36]
[38, 40, 42]
[44, 46, 48]
Understanding:
We used list comprehension to represent the matrices – we kept three lists inside a list representing the three rows, the first element in the three lists representing the first column, and so on. We initialized the sum as a zero matrix to update it in the next part of the program. In the outer for loop, i iterates through the length of the matrix-1: 3, which means 0, 1, and 2-represents the rows. In the inner for loop, j iterates through the length of the 0th index of matrix 1-length of the first row-3- represent the number of columns.
- The outer loop iterates through the rows, and the inner loop iterates through each element in the row.
- In the above example,
First iteration:
i = 0: j = 0, 1, 2
sum12 [0][0] = Mat1 [0][0] + Mat2 [0][0] -> 11 + 21 = 32
sum12 [0][1] = Mat1 [0][1] + Mat2 [0][1] -> 12 + 22 = 34
sum12 [0][2] = Mat1 [0][2] + Mat2 [0][2] -> 13 + 23 = 36
[11, 12, 13] + [21, 22, 23] = [32, 34, 36]
Second iteration:
i = 1: j = 0, 1, 2
sum12 [1][0] = Mat1 [1][0] + Mat2 [1][0] -> 14 + 24 = 38
sum12 [1][1] = Mat1 [1][1] + Mat2 [1][1] -> 15 + 25 = 40
sum12 [1][2] = Mat1 [1][2] + Mat2 [1][2] -> 16 + 26 = 42
[14, 15, 16] + [24, 25, 26] = [38, 40, 42]
Third iteration:
i = 2: j = 0, 1, 2
sum12 [2][0] = Mat1 [2][0] + Mat2 [2][0] -> 17 + 27 = 44
sum12 [2][1] = Mat1 [2][1] + Mat2 [2][1] -> 18 + 28 = 46
sum12 [2][2] = Mat1 [2][2] + Mat2 [2][2] -> 19 + 29 = 48
[17, 18, 19] + [27, 28, 29] = [44, 46, 48]
Example 2: Creating a star semi-pyramid
Analysis:
*
* *
* * *
* * * *
* * * * *
This is the pattern we need to create. We need a for loop to iterate the rows and another for loop to print stars in every single row.
Code:
rows = int (input ("Enter the number of rows for the pyramid: "))
for row in range (1, rows + 1):
for star in range (1, row + 1):
print ("*", end = " ")
print ()
Output:
Enter the number of rows for the pyramid: 8
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * * *
Understanding:
We took the input for the number of rows from the user. The outer for loop is to iterate the rows of the pyramid, and the inner for loop is to print the stars in the row.
If number of rows is given as 8 like in the above case:
rows = 8
row = 1 -> stars = 1 *
row = 2 -> stars = 1, 2 * *
row = 3 -> stars = 1, 2, 3 * * *
row = 4 -> stars = 1, 2, 3, 4 * * * *
row = 5 -> stars = 1, 2, 3, 4, 5 * * * * *
row = 6 -> stars = 1, 2, 3, 4, 5, 6 * * * * * *
row = 7 -> stars = 1, 2, 3, 4, 5, 6, 7 * * * * * * *
row = 8 -> stars = 1, 2, 3, 4, 5, 6, 7, 8 * * * * * * * *
Inside the inner for loop, we printed a star and specified end = “ “ to stop the print statement from jumping to a new line as we need the stars to be printed in the same row. After completing the inner loop execution, one row will be completed. We need to go to a new line now. Hence, we gave an empty print statement as, by default, it goes to a new line after printing.
- Not only this pyramid, nested for loops are used in building many types of pyramids of stars and numbers in Python.
Note about range () in for loops:
- In the above example, you can observe that in the range function, rather than using range (0, rows), we used range (1, rows + 1), and in the inner loop, too, rather than giving the range (0, i), we used range (1, i + 1).
- We would get only 7 rows if we give (0, rows) and (0, i). When i = 0, in the inner loop, it becomes: range (0, 0). Here, nothing will be printed because the end index and the starting index are the same.
- Here is an example:
for i in range (0, 0):
print(i)
Nothing will be printed.
While loop nested inside a for loop:
This is a quite common and most used nested loop. These loops are simpler than other nested loops.
Scenario: Printing every element in the list for the given number of times in the same line.
Analysis: We need a loop to iterate through the list and another loop to print every element 5 times.
Code:
num = int (input ("Enter how many times to repeat the elements: "))
list1 = [1, 2, 3, 4]
for i in list1:
count = 0
while (count < num):
print (i, end = " ")
count = count + 1
print ()
Output:
Enter how many times to repeat the elements: 4
1 1 1 1
2 2 2 2
3 3 3 3
4 4 4 4
Understanding:
We used the for loop to iterate through the elements of list1, and the inner while loop is used to print every element for "num" number of times. In each loop iteration, one element is selected, and the count is set to 0. In the while loop, we print the element and increment the variable count by 1. While loop prints the element till count becomes equal to num.
Control statements in nested for loop:
1. break statement
Generally, when we use a break statement inside a loop, the loop gets stopped then and there, and the control is given to the next statement of the loop. How does it work in a nested for loop?
- Suppose we keep a break statement in the body of the outer for loop above the inner loop when the condition is met. In that case, the outer loop will be terminated without executing the inner loop for that iteration.
rows = 4
for row in range (1, rows + 1):
if (row == 2):
break
for star in range (1, row + 1):
print ("*", end = " ")
print ()
Output:
*
Understanding:
When the row is equal to 2, without executing the inner loop, it will be terminated then and there. Hence, we got only one star when row = 1.
- Suppose we keep a break statement in the body of the outer loop below the inner loop when the condition is met. In that case, the outer loop will be terminated after executing the inner loop for that iteration.
rows = 4
for row in range (1, rows + 1):
for star in range (1, row + 1):
print ("*", end = " ")
if (row == 2):
break
print ()
Output:
*
* *
Understanding:
When row = 2, the inner loop is also executed, and hence the second row is printed, and then the loop will be terminated.
- If we keep a break statement inside the inner loop when the condition is met, the inner loop will be terminated, and the next iteration of the outer loop will be executed.
rows = 4
for row in range (1, rows + 1):
for star in range (1, row + 1):
print ("*", end = " ")
if (star == 2):
break
print ()
Output:
*
* *
* *
* *
Understanding:
In each row, the stars will be printed only till star = 2, the inner loop will be terminated, and the control goes to the next iteration of the outer loop and continues.
2. Continue statement:
Generally, a continue statement is used to skip one loop iteration. Let us see how it is used in nested for loops.
- When a continue statement is used in the body of the outer loop above the inner loop, when the condition is met, the inner loop will be skipped for that iteration of the outer loop.
rows = 4
for row in range (1, rows + 1):
if (row == 2):
continue
for star in range (1, row + 1):
print ("*", end = " ")
print ()
Output:
*
* * *
* * * *
Understanding:
When row = 2, the inner loop is not executed. Hence, in the pyramid, the second row is missing.
- When a continue statement is used in the body of the outer loop after the inner loop when the condition is met, the inner loop is executed, and the control goes to the next iteration of the outer loop.
rows = 4
for row in range (1, rows + 1):
for star in range (1, row + 1):
print ("*", end = " ")
if(row == 2):
continue
print ()
Output:
*
* * * * *
* * * *
Understanding:
When row = 2, the two stars will be printed in the second row, but the iteration is skipped before printing a new line. Hence, we got the third row in the second row.
- When a continue statement is used in the body of the inner loop; when the condition is met, that iteration of the inner loop is skipped. The outer loop won’t get affected.
rows = 4
for row in range (1, rows + 1):
for star in range (1, row + 1):
if (star == 2):
continue
print ("*", end = " ")
print ()
Output:
*
*
* *
* * *
Understanding:
When the star is equal to 2 in every row, that star is not printed, and the iteration is skipped. Hence, from the second row, one star is missing.
Using list comprehension to print in one line:
When working with lists, we have the concept of list comprehension that we can use to keep the whole nested for loop in one single line. For example, if we have two lists and want to create a new list with the sum of all combinations of elements from the two lists. If we want to do it normally:
list1 = [10, 20, 30, 40]
list2 = [50, 60, 70, 80]
list3 = []
for i in list1:
for j in list2:
list3. append (i + j)
print (list3)
Output:
[60, 70, 80, 90, 70, 80, 90, 100, 80, 90, 100, 110, 90, 100, 110, 120]
Using list comprehension:
list1 = [10, 20, 30, 40]
list2 = [50, 60, 70, 80]
list3 = [i + j for i in list1 for j in list2]
print (list3)
Output:
[60, 70, 80, 90, 70, 80, 90, 100, 80, 90, 100, 110, 90, 100, 110, 120]
Understanding:
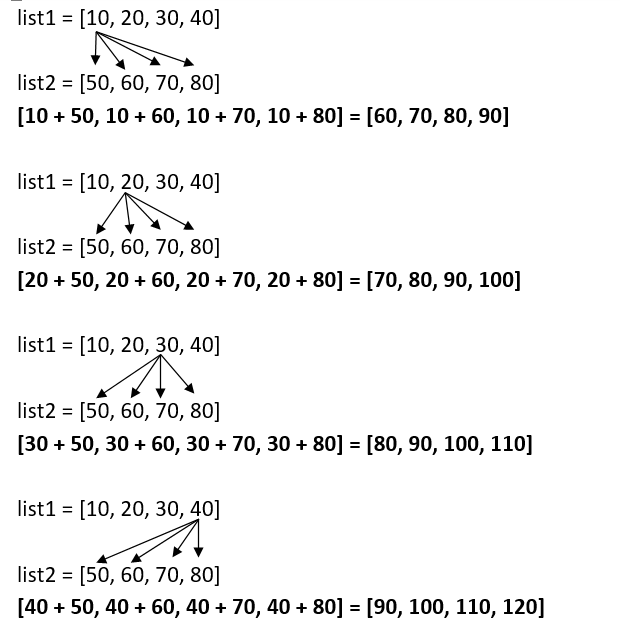
The output is the combination of all the items of the lists.