Unit Testing in Python
The process of testing whether a particular unit is working properly or not is called “UNIT TESTING”. A unit test will check small components in your application. The first and foremost level of software testing is unit testing; it will test the smallest part of testing, and it will test it. Unit testing is used to evaluate every single unit of software that will perform as we designed.
In other words, Unit testing is defined as a technique in which a single module is tested, and it will be checked by the developer whether there may be any errors. The main reason to check and test individual parts of a system is to detect, fix, and analyze errors.
The unit test module in Python is used to test the individual source code unit. This
Unit test plays an important role when we write large code, and it also checks whether the result is correct or not.
Most of the time, we prefer to print values, check the expected output manually, and check whether there is a match with the expected output. This type of manual checking takes more time. So, to overcome such types of methods, Python provides a unit test module. Here by using this, we can also redirect the performance of applications.
Here by learning this unit test module, we will learn to know like creating a code, finding bugs, and it will execute the code before it delivers to users.
Example 1:
def test_sum1():
assert sum([3,4,5])==12,"it must be 12"
def test_sum2():
assert sum([3,4,5])==12,"it should be 12"
if __name__=="__main__":
test_sum1()
test_sum2()
print("sum is correct")
OUTPUT

From the above example, there are two functions, test_sum1() and test_sum2(). It gives the correct output because we have mentioned the correct sum in the assert method.
Example 2:
def test_sum1():
assert sum([3,4,5])==12,"it must be 12"
def test_sum2():
assert sum([3,4,5])==11,"it should be 11"
if __name__=="__main__":
test_sum1()
test_sum2()
print("sum is correct")
OUTPUT
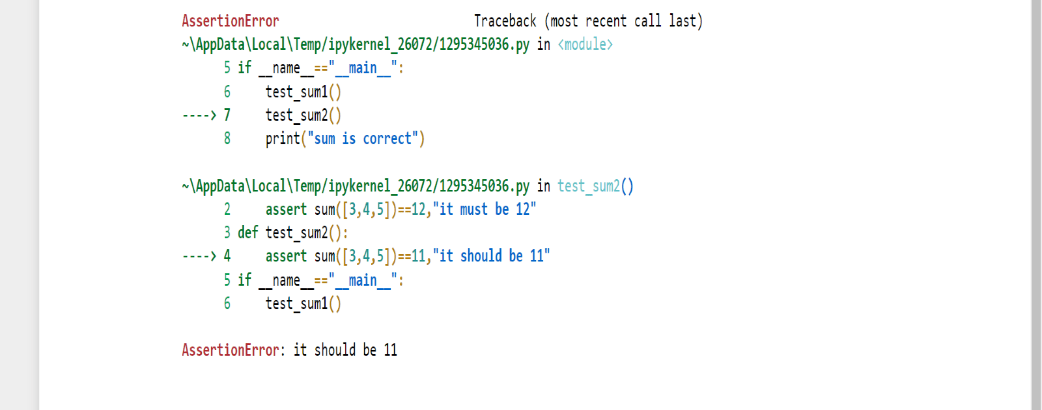
Here we had declared the wrong assert method; the sum is incorrect in the function test_sum2(). So, it gives an error called assertion error.
We use test runners to remove multiple errors. Python interpreter will give an error when there is an error in the first message if there are multiple errors.
“Test runner” is mainly used and designed for testing the output, used for running tests, and also gives tools for keeping and checking tests and applications.
Choosing the Test Runner
In Python, there are many test runners for testing and checking whether the code is correct or not and for checking the errors. Unit test is most popularly used and is a built-in python library. Unit-test test runner has a long life compared to other frameworks.
Here are some of the test-runners given below.
- Unit test
- Nose
- Py test
According to our requirements, we can choose any of them.
What is Unit Test?
Unit test is a built-in python standard library, and it is more useful because it consists of both a test framework and a test runner. Unit test has some requirements for the writing and execution of code. They are:
- For the unit test, the code should be written using functions and classes.
- In Testcase class, there must be a sequence of different assertion methods apart from built-in assertion statements.
Steps involved in Unit Testing
- Import unit test from library
- Create a class for the test, which is inherited from that class, the unit test. Testcase
- Write the test functions in the class
- Call unit test. main() or running the Testcases.
Example
import unittest
class exampleTest(unittest.TestCase):
# It will Return either True or False.
def test(self):
self.assertTrue(True)
if __name__ == '__main__':
unittest.main()
The above example is the example code using a unit test framework with only a single test. It returns fail if TRUE becomes FALSE.
To run a test
The last two blocks of code are useful and helps for running of the file and to run the test through a command line.
if __name__ == '__main__':
unittest.main()
OUTPUT
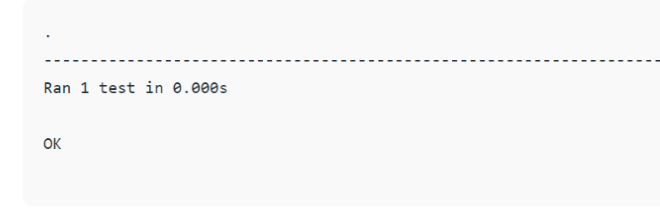
From the above result, we observed a dot (.) on the first line, which means the above test was passed and gave “OK”.
From the unit test code, there are three possibilities for test outcomes:
- OK: When we see OK in the resultant output, all the tests are passed.
- ERROR: It gives an exception error rather than giving assertion error.
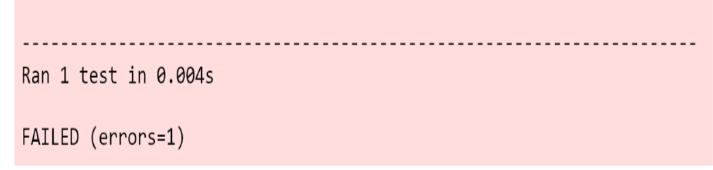
The above picture shows an error because an exception will be raised in the code.
- FAIL: When this FAIL message occurs, the test is not passed, and an assertion error exception will be raised.
Unit Test supports OOPS Concepts
Test case: A test case is a set of actions to test a system, application, or software. These sets of actions are used to perform on a system because they must satisfy the requirements of software and functions exactly.
A test case gives instructions to the tester to identify the system's performance and functions of an application. For suppose, if a test case fails, the result might be a fault of a software system, and a software organization can solve this.
Professional testers write test cases, and these test cases will run only after completing the features and requirements that a software needs. It will check the complete requirements of software for a product to design.
The test suite contains a group of test cases that will check the logical parts of the application.
Different Types of Test Cases
To check the product's front and back end, the software organization will take multiple face outcomes and evaluate them. Here we discuss two types of test cases:
- Formal Test Case: Formal test cases are test cases that are given in detail with a set of preconditions and test data. With this set of instructions and inputs, the tester writes a test in detail.
The input is predefined in formal test cases, which means there will be an expected output that satisfies the input given, and it tests the attempts to validate. - Informal Test Cases: It is the reverse of legal test cases. It does not have known inputs and outputs. In this case, testers will execute to discover or implement new things, and they reveal interesting facts about new digital things.
Mostly, the test cases are formal and will be advanced and planned according to the software's requirements.
Examples of Test Cases
- UI
- Performance
- Database
- Functionality
- User acceptance
Test Suite
As we discussed above, a test suite is a group of test cases used for testing a software program, and it shows that it contains some set of instructions and behaviors by executing the formal tests jointly.
Test Runner
A test runner has defined a class that will run a test or combination. It is also called the library, which will pick a group of unit tests and a group of settings, and these will execute them and will write the test results to the platform or console.
Test Fixture
The tests do not run as simply as we know; the text fixture is a baseline for test runners to find fixed environments so that there will be a chance of repeatable results.
Examples
- This is used for the creation of databases temporarily
- Also used for starting a server
How to Perform Unit Testing in Python
Module name: unit test
Class name: Test Case
Instance methods: three methods
1. setUp()
2. test ()
3. tearDown ()
Py Test framework
It is a next-level framework. Testers especially use the Py Test framework. Based on the unit test, a new advanced framework developed was Py Test. In other words, Py Test is an advanced level unit test.
Example
import unittest
def sum(a,b):
return a+b
class SumTest(unittest.TestCase):
def setUp(self):
print("SETUP CALLED")
self.a=10
self.b=20
def tearDown(self):
print("TEARDOWN CALLED...")
def test_sumfunc_1(self):
print("TEST 1 CALLED")
#arrange
a=10
b=20
#act
result=sum(self.a,self.b)
#assert
self.assertEqual(result,self.a+self.b)
def test_sumfunc_2(self):
print("TEST 2 CALLED")
#arrange
a=10
b=20
#act
Result=sum(self.b,self.a)
#assert
self.assertEqual(result,self.a+selfb)
if __name__=="__main__":
unittest.main()
OUTPUT
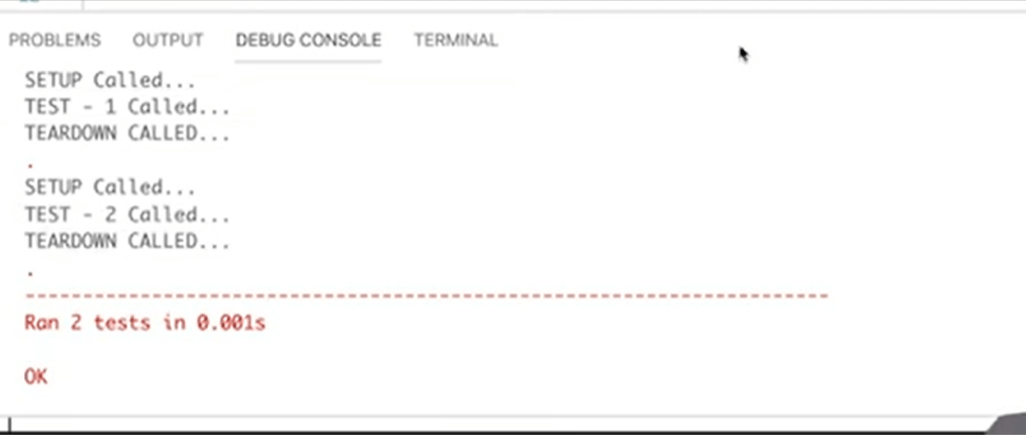
- assertEqual(): This statement is used to test whether the result gained from the code is equal to the expected result.
- assertTrue() and assertFalse(): This statement is used to test the statement either is true or not.
- assertRaises(): This statement is used to raise the exception if needed.
- Unit test.main(): It is the main function that provides a command-line interface
- The above example has a class named SumTest and two sum functions, test_sumfunc_1 and test_sumfunc_2.
- setUp(): open chrome browser
- test1(): test login functionality in google chrome
- tearDown(): close chrome browser
Advantages of Unit Testing
- Only less number of errors occurs when a problem is identified earlier.
- Debugging processes will be made easier.
- Testers and developers will make changes to the code base.
- Developers will re-use the code and migrate these codes for a new project.
- The problem must be fixed before the code is written. Otherwise, the cost of fixing the problem will increase.
Limitations of Unit Testing
- Test results will only be displayed to the console, and generating reports is impossible.
- The unit test framework executes test methods in alphabetical order, and it is impossible to customize execute the order.
- As a part of batch execution(TestSuite), all test methods from the specified TestCase classes will be executed, and it is impossible to specify particular methods.
- In unit testing, only limited setUp and tearDown methods are available.
- setUpClass()----> Before executing all test methods of a TestCase class.
- tearDownClass()----->After executing all test methods of a TestCase class.
- setUp---->Before every test method execution.
- tearDown()---->After every test method execution.