Is Python Object Oriented Programming language
In this tutorial, we will see whether python also belongs to an object-oriented programming language like several others. We will also see the advantages of using OOPs. Further, we will understand the real-world entities used in OOPs.
To answer the very first question in this tutorial, yes, Python is an Object-Oriented Programming language that makes use of classes and objects. Some of the prevalent object-oriented programming languages are- Java, C++, JavaScript, C#, PHP, MATLAB, PYTHON, etc.
Benefits of Object-oriented programming language:
- Use of objects has made the code reusability easier.
- It is easier to debug when objects are in use and an issue in coding arises.
- Objects can be deployed without revealing implementation details.
Now, let us understand the concepts of classes and objects
Classes
Class is like a blueprint that helps to create an object. It describes the properties and behaviors. It includes the contents of objects such as variables, methods, and functions.
For example- let us consider a class “Flower” now the properties of a Flower could be color, aroma, size, and season of flourishing.
Syntax of writing a class:
class Class Name
Example of writing a class name:
class Flower
Objects: Objects are defined as an instance of a class. The method and function of a class can be accessed with the help of an object only.
Now, let us understand the creation of classes and objects in Python:
In Python, the creation of classes is easy, it can be created with the help of a keyword called “class” along with the class name. Functions / Methods and variables are declared inside the class itself.
Way to create an object in python:
Syntax:
Object name = class_name( )
obj = Flower( )
The functions written within the class can be accessed with the aid of class with its class name and with the “.” dot operator calls its function.
We can access the class in two following ways:
- Without creating the object
To access the functions inside the class, the class name along with the dot operator is written.
Example-
Class Myclass():
Myclass.flower()
Rafflesia
Myclass.define()
largest flower
- Without creating an object.
p = Myclass
p.flower()
Rafflesia
p.define()
largest flower
Now creating an object is easier. It can be created by using a variable named “p” in this case and assigning it to the class “Myclass” and with the help of a dot operator the functions can be invoked inside the class.
Real-world entities used in python are:
- Inheritance
2.Polymorphism
3.Abstraction
4. Encapsulation
Inheritance
Inheritance is the ability of one class to originate or inherit the properties from another class. The class deriving properties from other classes is called the derived class or child class and the class from which the properties are being derived is called the base class or parent class.
Below are some of the benefits of inheritance are:
- Inheritance is capable of representing real-world relationships well.
- It provides the code reusability. Users can be escaped from the trouble of writing the same code repeatedly.it also allows users to add additional features to a class without the need of altering it.
- Its nature is transitive, which means that if class B inherits properties from another class let’s say A, then all the subclasses of B would automatically inherit properties from class A.
Example- Program showing the implementation of inheritance in python
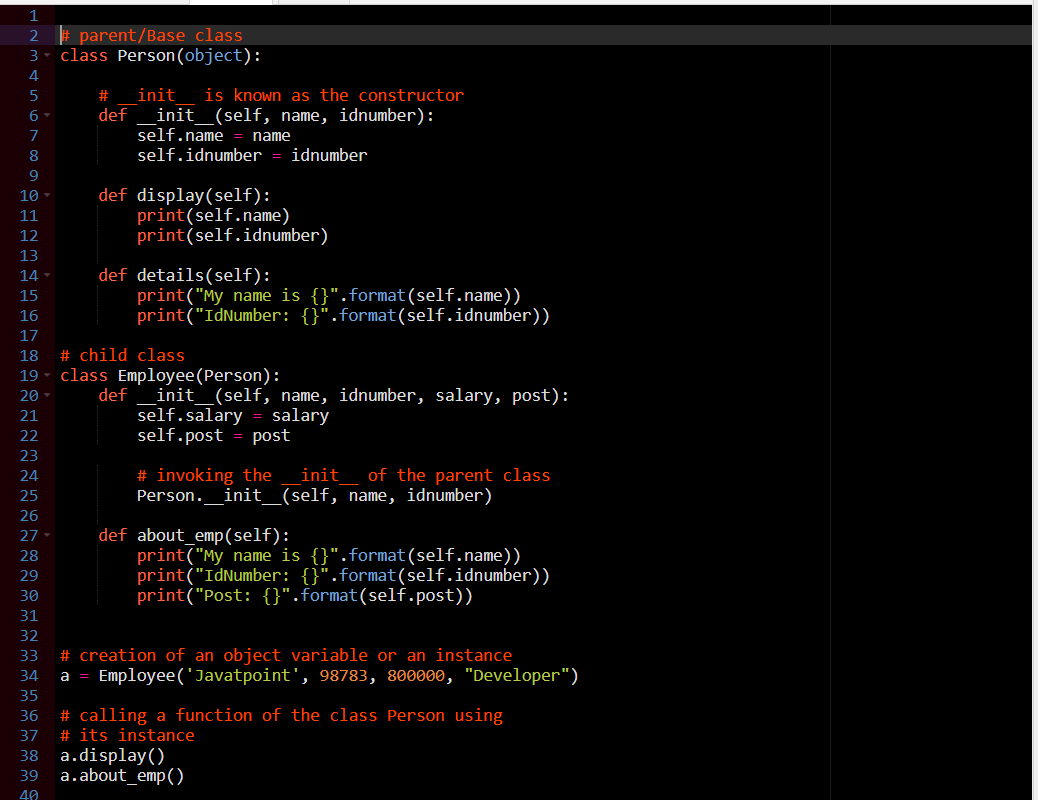
Output:
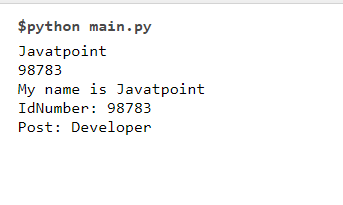
Polymorphism
It means having numerous forms.
Example: showing implementation of polymorphism in python
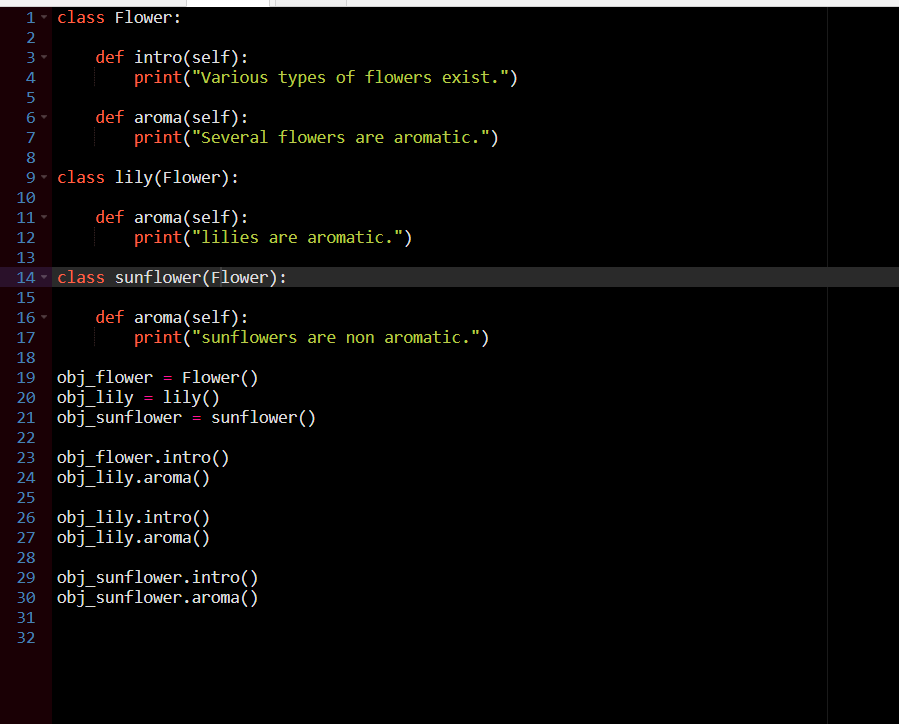
Output:
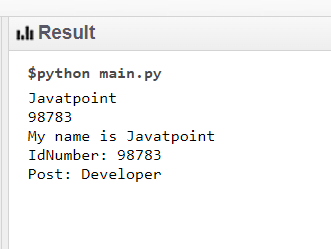
Encapsulation
Encapsulation is one of the vital concepts in object-oriented programming (OOP). It describes the idea of covering data and the methods that work on data within a single unit.
Example: Showing the implementation of encapsulation in python
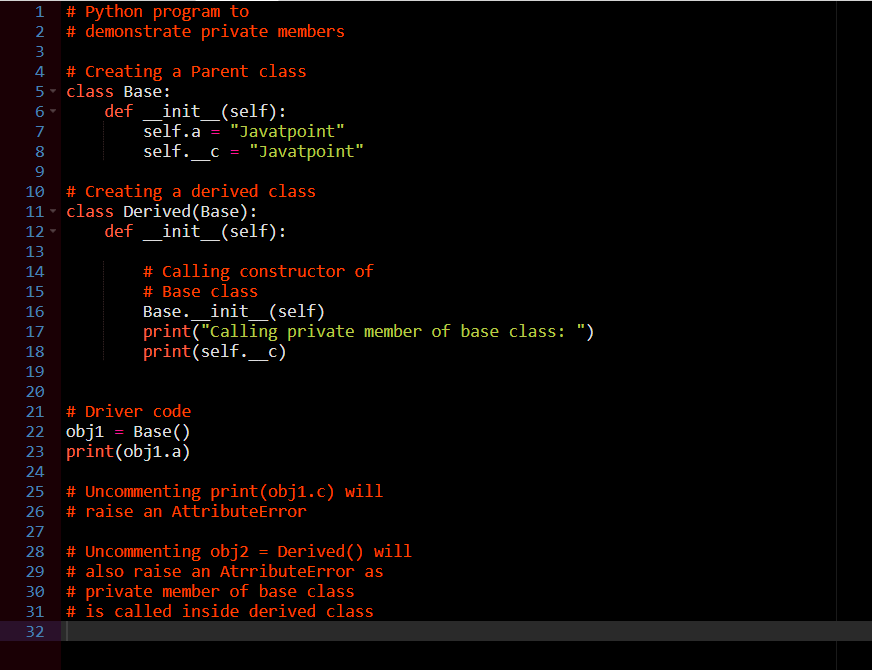
Output:
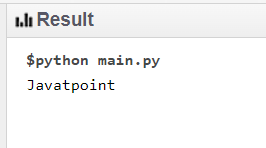
Abstraction
It is defined as a process of managing complexity by hiding redundant information and data from the user. It also means showing only the required data and results to users and not the processes.
Example- We can talk over phones, chat on the internet, etc.
Do we know all the processes working at the backend which allow us to perform these functions? NO
This is only termed an abstraction. We can act but background details have been hidden by the developers.
In Python, data hiding is achieved by using a double underscore (Or __) symbol before the name of an attribute and the user won’t be able to access those attributes outside the class.
Example: Showing implementation of Abstraction
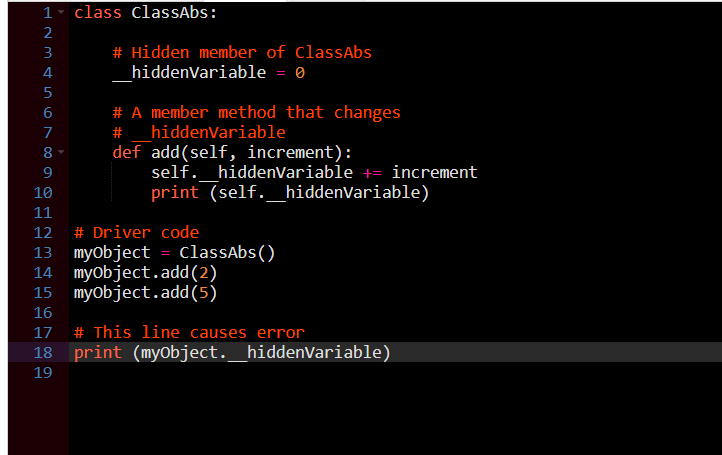
Output:
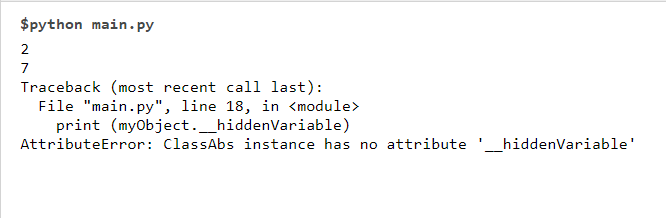
Explanation- Uncommenting print statement written at the results in an error.
We have tried to access the variable written outside the class which is against the principle of abstraction. So, the error is thrown.
Example: Showing the implementation of the abstraction
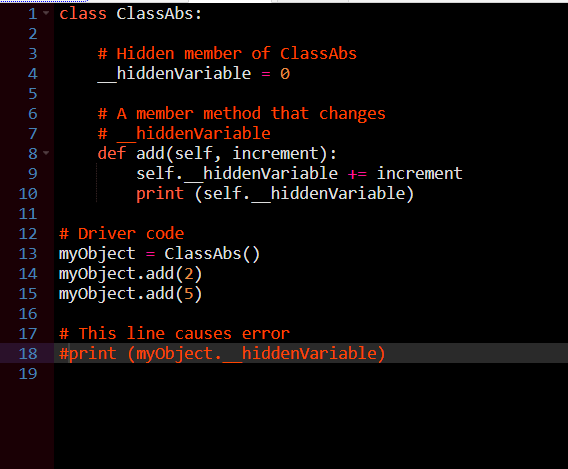
Output:
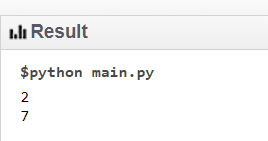
Explanation: We have Commented on the print statement written at the end thus, the error gets resolved.